How to solve “Error: Assignment to expression with array type” in C?

In C programming, you might have encountered the “ Error: Assignment to expression with array type “. This error occurs when trying to assign a value to an already initialized array , which can lead to unexpected behavior and program crashes. In this article, we will explore the root causes of this error and provide examples of how to avoid it in the future. We will also learn the basic concepts involving the array to have a deeper understanding of it in C. Let’s dive in!
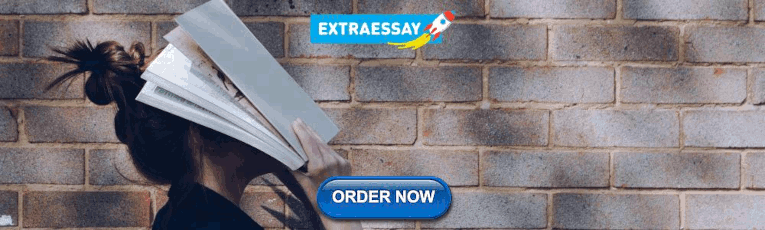
What is “Error: Assignment to expression with array type”? What is the cause of it?
An array is a collection of elements of the same data type that are stored in contiguous memory locations. Each element in an array is accessed using an index number. However, when trying to assign a value to an entire array or attempting to assign an array to another array, you may encounter the “Error: Assignment to expression with array type”. This error occurs because arrays in C are not assignable types, meaning you cannot assign a value to an entire array using the assignment operator.
First example: String
Here is an example that may trigger the error:
In this example, we have declared a char array “name” of size 10 and initialized it with the string “John”. Then, we are trying to assign a new string “Mary” to the entire array. However, this is not allowed in C because arrays are not assignable types. As a result, the compiler will throw the “Error: Assignment to expression with array type”.
Initialization
When you declare a char array in C, you can initialize it with a string literal or by specifying each character separately. In our example, we initialized the char array “name” with the string literal “John” as follows:
This creates a char array of size 10 and initializes the first four elements with the characters ‘J’, ‘o’, ‘h’, and ‘n’, followed by a null terminator ‘\0’. It’s important to note that initializing the array in this way does not cause the “Error: Assignment to expression with array type”.
On the other hand, if you declare a char array without initializing it, you will need to assign values to each element of the array separately before you can use it. Failure to do so may lead to undefined behavior. Considering the following code snippet:
We declared a char array “name” of size 10 without initializing it. Then, we attempted to assign a new string “Mary” to the entire array, which will result in the error we are currently facing.
When you declare a char array in C, you need to specify its size. The size determines the maximum number of characters the array can hold. In our example, we declared the char array “name” with a fixed size of 10, which can hold up to 9 characters plus a null terminator ‘\0’.
If you declare a char array without specifying its size, the compiler will automatically determine the size based on the number of characters in the string literal you use to initialize it. For instance:
This code creates a char array “name” with a size of 5, which is the number of characters in the string literal “John” plus a null terminator. It’s important to note that if you assign a string that is longer than the size of the array, you may encounter a buffer overflow.
Second example: Struct
We have known, from the first example, what is the cause of the error with string, after that, we dived into the definition of string, its properties, and the method on how to initialize it properly. Now, we can look at a more complex structure:
This struct “struct_type” contains an integer variable “id” and a character array variable “string” with a fixed size of 10. Now let’s create an instance of this struct and try to assign a value to the “string” variable as follows:
As expected, this will result in the same “Error: Assignment to expression with array type” that we encountered in the previous example. If we compare them together:
- The similarity between the two examples is that both involve assigning a value to an initialized array, which is not allowed in C.
- The main difference between the two examples is the scope and type of the variable being assigned. In the first example, we were dealing with a standalone char array, while in the second example, we are dealing with a char array that is a member of a struct. This means that we need to access the “string” variable through the struct “s1”.
So basically, different context, but the same problem. But before dealing with the big problem, we should learn, for this context, how to initialize a struct first. About methods, we can either declare and initialize a new struct variable in one line or initialize the members of an existing struct variable separately.
Take the example from before, to declare and initialize a new struct variable in one line, use the following syntax:
To initialize the members of an existing struct variable separately, you can do like this:
Both of these methods will initialize the “id” member to 1 and the “struct_name” member to “structname”. The first one is using a brace-enclosed initializer list to provide the initial values of the object, following the law of initialization. The second one is specifically using strcpy() function, which will be mentioned in the next section.
How to solve “Error: Assignment to expression with array type”?
Initialize the array type member during the declaration.
As we saw in the first examples, one way to avoid this error is to initialize the array type member during declaration. For example:
This approach works well if we know the value of the array type member at the time of declaration. This is also the basic method.
Use the strcpy() function
We have seen the use of this in the second example. Another way is to use the strcpy() function to copy a string to the array type member. For example:
Remember to add the string.h library to use the strcpy() function. I recommend going for this approach if we don’t know the value of the array type member at the time of declaration or if we need to copy a string to the member dynamically during runtime. Consider using strncpy() instead if you are not sure whether the destination string is large enough to hold the entire source string plus the null character.
Use pointers
We can also use pointers to avoid this error. Instead of assigning a value to the array type member, we can assign a pointer to the member and use malloc() to dynamically allocate memory for the member. Like the example below:
Before using malloc(), the stdlib.h library needs to be added. This approach is also working well for the struct type. In the next approach, we will talk about an ‘upgrade-version’ of this solution.
Use structures with flexible array members (FAMs)
If we are working with variable-length arrays, we can use structures with FAMs to avoid this error. FAMs allow us to declare an array type member without specifying its size, and then allocate memory for the member dynamically during runtime. For example:
The code is really easy to follow. It is a combination of the struct defined in the second example, and the use of pointers as the third solution. The only thing you need to pay attention to is the size of memory allocation to “s”. Because we didn’t specify the size of the “string” array, so at the allocating memory process, the specific size of the array(10) will need to be added.
This a small insight for anyone interested in this example. As many people know, the “sizeof” operator in C returns the size of the operand in bytes. So when calculating the size of the structure that it points to, we usually use sizeof(*), or in this case: sizeof(*s).
But what happens when the structure contains a flexible array member like in our example? Assume that sizeof(int) is 4 and sizeof(char) is 1, the output will be 4. You might think that sizeof(*s) would give the total size of the structure, including the flexible array member, but not exactly. While sizeof is expected to compute the size of its operand at runtime, it is actually a compile-time operator. So, when the compiler sees sizeof(*s), it only considers the fixed-size members of the structure and ignores the flexible array member. That’s why in our example, sizeof(*s) returns 4 and not 14.
How to avoid “Error: Assignment to expression with array type”?
Summarizing all the things we have discussed before, there are a few things you can do to avoid this error:
- Make sure you understand the basics of arrays, strings, and structures in C.
- Always initialize arrays and structures properly.
- Be careful when assigning values to arrays and structures.
- Consider using pointers instead of arrays in certain cases.
The “ Error: Assignment to expression with array type ” in C occurs when trying to assign a value to an already initialized array . This error can also occur when attempting to assign a value to an array within a struct . To avoid this error, we need to initialize arrays with a specific size, use the strcpy() function when copying strings, and properly initialize arrays within structures. Make sure to review the article many times to guarantee that you can understand the underlying concepts. That way you will be able to write more efficient and effective code in C. Have fun coding!
“Expected unqualified-id” error in C++ [Solved]
How to Solve does not name a type error in C++

C - How to solve the error: assignment to expression with array type
The error "assignment to expression with array type" in C occurs when you try to assign a value to an array as a whole, rather than to an individual element of the array. In C, arrays are not assignable as a whole after their declaration. This means you cannot change the entire array using a single assignment statement once it is defined. Instead, you must assign values to each element individually, or use a function like strcpy (for strings) or memcpy (for other types of arrays).
Here are some examples to illustrate this:
Example of the Error
Suppose you have an array like this:
Trying to assign a new set of values to this array like this will result in an error:
Correct Ways to Assign Values
Assign Values Individually :
Using a Loop :
If you want to assign the same value to all elements or values from another array, you can use a loop:
Using memcpy for Copying Arrays :
If you want to copy an array, use memcpy from string.h :
For Strings, Use strcpy :
If the array is a string (i.e., a character array), use strcpy :
Remember, these rules apply because arrays in C are a lower-level data structure compared to languages like Python or Java, which offer more flexibility with array assignments. In C, an array is essentially a fixed block of memory, and the name of the array is a pointer to the first element of that block. You cannot change the pointer to point to a different block; you can only modify the contents of the block itself.
Fixing "Assignment to Expression with Array Type" in C:
Description: The error often occurs when trying to directly assign one array to another. The correct approach is to use a loop to copy individual elements.
How to Resolve Array Type Assignment Error in C:
Description: Demonstrates the correct method of copying array elements using a loop to resolve the array type assignment error.
C Programming: Assignment to Expression with Array Type Solution:
Description: Provides a solution to the "assignment to expression with array type" error by using a loop for array element assignment.
Error in C: Assignment to Expression with Array Type:
Description: Illustrates the incorrect way of assigning one array to another, resulting in the "assignment to expression with array type" error.
How to Correct Assignment to Expression with Array Type in C:
Description: Corrects the array type assignment error by using a loop to copy array elements individually.
C Array Type Error: Assignment Issue:
Description: Resolves the "assignment to expression with array type" issue by using a loop for array element assignment.
Solving "Assignment to Expression with Array Type" Error in C:
Description: Offers a solution to the "assignment to expression with array type" error using a loop for copying array elements.
Fix Array Type Assignment Error in C Programming:
Description: Provides a fix for the array type assignment error in C programming by using a loop for copying array elements.
Resolving C Error: Assignment to Expression with Array Type:
Description: Resolves the C error "assignment to expression with array type" by using a loop for array element assignment.
C Programming: Addressing Assignment Error with Array Type:
Description: Addresses the assignment error with array type in C programming by using a loop for copying array elements.
How to Handle Array Type Assignment Error in C:
Description: Demonstrates how to handle the array type assignment error in C by using a loop for copying array elements.
C Error: Array Type Assignment Troubleshooting:
Description: Provides troubleshooting for the C error "assignment to expression with array type" by using a loop for array element assignment.
Correcting Assignment to Expression with Array Type in C:
Description: Corrects the assignment to expression with array type in C by using a loop for copying array elements.
Dealing with Array Type Assignment Issue in C:
Description: Provides a method for dealing with the array type assignment issue in C by using a loop for copying array elements.
C Error: Assignment to Expression with Array Type Explanation:
Description: Explains the C error "assignment to expression with array type" and provides a correct approach using a loop for copying array elements.
C Programming: Handling Assignment to Expression with Array Type:
Description: Demonstrates how to handle the assignment to expression with array type in C by using a loop for copying array elements.
Solving "Assignment to Expression with Array Type" Error in C Code:
Description: Offers a solution to the "assignment to expression with array type" error in C code by using a loop for copying array elements.
Fixing Array Type Assignment Error in C Program:
Description: Fixes the array type assignment error in a C program by using a loop for copying array elements.
Troubleshooting C Error: Assignment to Expression with Array Type:
Description: Provides troubleshooting steps for the C error "assignment to expression with array type" by using a loop for copying array elements.
java-12 version svn angularjs-validation imagefield formatting xpath-1.0 stanford-nlp magrittr security
More Programming Questions
- Types of memory areas allocated by JVM
- How to Create and Setup Spring Boot Project in Eclipse IDE?
- Highcharts use negative area chart
- PHP Exception Handling
- Using Transactions or SaveChanges(false) and AcceptAllChanges() in C#?
- How to atomically rename a file in Java, even if the dest file already exists?
- How to Deserialize an JSON object with invalid field name in it using C#
- How to enable cross-origin requests (CORS) in ASP.NET Core MVC
- Reformat a Python file to have 4 space indentations
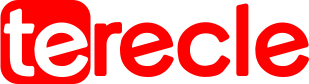
How to Fix Error: Assignment To Expression With Array Type
This error shows the error as not assignable due to the value associated with the string; or the value is not feasible in C programming language.
In order to solve this, you have to use a normal stcpy() function to modify the value.
However, there are other possible ways to fix this issue.
Causes of the error
Here are few reasons for error: assignment to expression with array type:
#1. Using unassignable array type
You are using an array type that is not assignable, or one that is trying to change the constant value in the program
If you are trying to use an unsignable array in the program, here’s what you will see:
This is because s1.name is an array type that is unassignable. Therefore, the output contains an error.
#2. Error occurs because block of memory is below 60 chars
The error also appears if the block of memory is below 60 chars. The data should always be a block of memory that fits 60 chars.
In the example above, “data s1” is responsible for allocating the memory on the stack. Assignments then copy the number, and fails because the s1.name is the start of a struct 64 bytes long which can be detected by a compiler, also Ogechukwu is 6 bytes long char due to the trailing \0 in the C strings. So , assigning a pointer to a string into a string is impossible, and this expression error occurs.
Example:
The output will be thus :
#3. Not filling structure with pointers
If a programmer does not define a structure which points towards char arrays of any length, an expression error occurs, however If the programmer uses a defined structure field, they also have to set pointers in it too. Example of when a program does not have a defined struct which points towards a char.
#4. There is a syntax error
If a Programmers uses a function in an incorrect order, or syntax.also if the programer typed in the wrong expression within the syntax.
example of a wrong syntax, where using “-” is invalid:
#5. You directly assigned a value to a string
The C programming language does not allow users to assign the value to a string directly. So you cant directly assign value to a string, ifthey do there will receive an expression error.
Example of such:
#6. Changing the constant value
As a programmer, if you are trying to change a constant value, you will encounter the expression error. an initialized string , such as “char sample[19]; is a constant pointer. Which means the user can change the value of the thing that the pointer is pointing to, but cannot change the value of the pointer itself. When the user tries to change the pointer, they are actually trying to change the string’s address, causing the error.
The output gives the programmer two independent addresses. The first address is the address of s1.name while the second address is that of Ogechukwu. So a programmer cannot change s1.name address to Ogechukwu address because it is a constant.
How to fix “error: assignment to expression with array type” error?
The following will help you fix this error:
#1. Use an assignable array type
Programmers are advised to use the array type that is assignable by the arrays because an unassignable array will not be recognized by the program causing the error. To avoid this error, make sure the correct array is being used.
Check out this example:
#2. Use a block of memory that fits 60 chars
Use the struct function to define a struct pointing to char arrays of any lenght. This means the user does not have to worry about the length of the block of memory. Even if the length cis below 60 chars, the program will still run smoothly.
But if you are not using the struct function, take care of the char length and that the memory block should fit 60 chars and not below to avoid this error.
#3. Fill the structure with pointers
If the structure is not filled with pointers, you will experience this error. So ensure you fill the struct with pointers that point to char arrays.
Keep in mind that the ints and pointers can have different sizes. Example
This example, the struct has been filled with pointers.
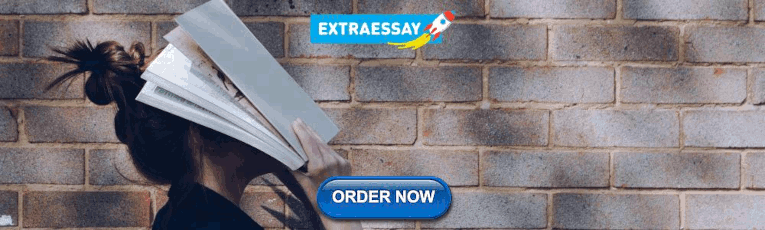
#4. Use the correct syntax
Double check and ensure you are using the right syntax before coding.if you are confused about the right syntax, then consult Google to know the right one.
Example
#5. Use Strcpy() function to modify
In order to modify the pointer or overall program, it is advisable to use the strcpy() function. This function will modify the value as well because directly assigning the value to a string is impossible in a C programming language. usin g this function helps the user get rid of assignable errors. Example
Strcpy(s1.name, “New_Name”);
#6. Copy an array using Memcpy
In order to eliminate this error,a programmer can use the memcpy function to copy an array into a string and prevent errors.
#7. Copy into the array using Strcpy()
Also you can copy into the array using strcpy() because of the modifiable lvalue. A modifiable lvalue is an lvalue that has no array type. So programmers have to use strcpy() to copy data into an array.
Follow these guide properly and get rid of “error: assignment to expression with array type”.
# Related Articles
How to fix groupme not loading pictures, how to start a spotify jam session with friends, how to add, remove, and delete samsung account from your android phone.
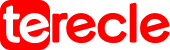
Terecle has been a source of help and technology advice since 2021. We review and recommend tech products and services on a regular basis, as well as write about how to fix them if they break down. In order to improve your life and experience, we're here providing you with hands on expertise.
- Editorial Statement
- Privacy Policy
- Cookie Disclosure
- Advertise with us
© 2021-2024 HAUYNE LLC., ALL RIGHTS RESERVED
Terecle is part of Hauyne publishing family. The display or third-party trademarks and trade names on this site does not necessarily indicate any affiliation or endorsement of Terecle .
Type above and press Enter to search. Press Esc to cancel.
Get the Reddit app
The subreddit for the C programming language
assignment to expression with array type or array type 'int[5]' is not assignable
Would like to know why I got that error message.
int data[5] = {1,2,3,4,5};
int (*ptr)[5] = &data;
*ptr = *ptr + 1// got an error.
Why is the pointer arithmetic not operatable here?
Because *ptr = data (*ptr is not pointer but an array? )
thank you for helping me out !!
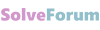
- Search forums
- Solveforum All topics
[Solved] "error: assignment to expression with array type error" when I assign a struct field (C)
- Thread starter p4nzer96
- Start date Nov 21, 2021
- Nov 21, 2021
"error: assignment to expression with array type error" Click to expand...
SolveForum.com may not be responsible for the answers or solutions given to any question asked by the users. All Answers or responses are user generated answers and we do not have proof of its validity or correctness. Please vote for the answer that helped you in order to help others find out which is the most helpful answer. Questions labeled as solved may be solved or may not be solved depending on the type of question and the date posted for some posts may be scheduled to be deleted periodically. Do not hesitate to share your response here to help other visitors like you. Thank you, solveforum. Click to expand...
Recent Threads
Why is it okay for my .bashrc or .zshrc to be writable by my normal user.
- Zach Huxford
- Jun 26, 2023
SolveForum.com may not be responsible for the answers or solutions given to any question asked by the users. All Answers or responses are user generated answers and we do not have proof of its validity or correctness. Please vote for the answer that helped you in order to help others find out which is the most helpful answer. Questions labeled as solved may be solved or may not be solved depending on the type of question and the date posted for some posts may be scheduled to be deleted periodically. Do not hesitate to share your thoughts here to help others. Click to expand...
SFTP user login details real-time filtering
- Amal P Ramesh
get nat port forwarding IP address
Using docker does not give error with sudo but using ctr does on starting a container, what are some of the latest nike soccer shoes that have gained popularity among players and enthusiasts in recent years, can't change tcp/ipv4 settings on windows 10.

Customer service access 2007 template
- tintincutes
Latest posts
- Latest: QAZ
- 8 minutes ago
- Latest: Adi
- Latest: kg23
- Latest: Alexey Starinsky
- Latest: RedaB
Newest Members

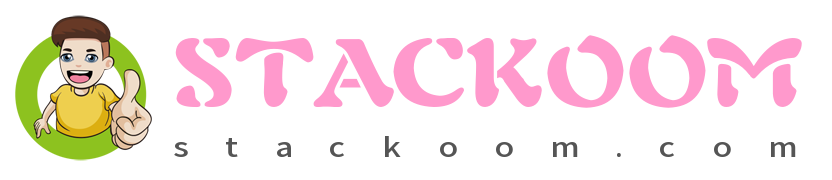
C Programming: error: assignment to expression with array type
Hello I am a beginner in C and I was working with structures when i have this problem:
With this one I have this error ( error: assignment to expression with array type ). error: assignment to expression with array type )。--> So I decided to do it using pointers and it actually working this is the code I used:
So the question is what is the difference between both of them since strings are pointers. thank you..
solution1 1 2020-05-15 20:28:31
First part, you try to copy two array of character (string is not a pointer, it is array of character that is terminated by null character \0 ). \0 终止的字符数组)。-->
If you want to copy value of an array to another, you can use memcpy , but for string, you can also use strcpy . memcpy ,但对于字符串,你也可以使用 strcpy 。-->
Second part, you make the pointer point to string literal. The pointer points to first character of the string ( r in this case). r )。-->
You can see How to copy a string using a pointer 如何使用指针复制字符串 -->
And Assigning strings to pointer in C Language 字符串分配给 C 语言中的指针 -->
solution2 1 ACCPTED 2020-05-15 20:30:35
Strings are not pointers, they are sequences of characters terminated with a null byte. These sequences are stored in arrays, which are not pointers either, but objects that live in memory and to which a pointer can point. A pointer is a variable that contains the address of an object in memory.
In the first example, you try and store a string into an array with = . = 将字符串存储到数组中。--> C does not support this type of assignment and complains with an explicit error message. You can copy the string into the array with strcpy , assuming the destination array is large enough to store the bytes of the string, including the null terminator. strcpy 将字符串复制到数组中,假设目标数组足够大以存储字符串的字节,包括 null 终止符。--> "reda" uses 5 bytes, so it is OK to copy it into E[0].nom : "reda" 使用 5 个字节,因此可以将其复制到 E[0].nom :-->
The second example uses a different approach: nom is now defined as a pointer to char . nom 现在定义为指向 char 的指针。--> Defining the array E in main leaves it uninitialized, so you can only read from the pointers after you set their value to point to actual arrays of char somewhere in memory. E[0].nom = "reda"; main 中定义数组 E 使其未初始化,因此您只能在将指针的值设置为指向 memory 中某处的 char 的实际 arrays 后才能读取指针 E[0].nom = "reda"; --> does just that: set the address of the string literal "reda" into the member nom of E[0] . "reda" 的地址设置为 E[0] 的成员 nom 。--> The string literal will be placed by the compiler into an array of its own, including a null terminator, and that must not be changed by the program.
solution3 1 2020-05-15 21:04:32
It is a lower-level concept actually.
If we say char nom[20] than its memory location will be decided at compile time and it will use stack memory (see the difference between stack memory and heap memory to gain more grasp on lover level programming). char nom[20] ,那么它的 memory 位置将在编译时确定,它将使用堆栈 memory(请参阅堆栈 memory 和堆 memory 之间的区别,以更好地掌握情人级编程)。--> so we need to use it with indexes.
On the other hand if we create a string using the double quotes method (or the second way in which you used pointers). The double quoted strings are identified as const char* by the compiler and the string is placed at the heap memory rather than the stack memory. moreover their memory location is not decided at compile time. const char* 并且字符串被放置在堆 memory 而不是堆栈 memory。此外,它们的 memory 位置在编译时没有确定。-->
a very efficient way of testing the difference between these two types of strings is that when you use sizeof() with char[20] nom it will return 20 * sizeof(char) which evaluates to 20 without any doubt. sizeof() 与 char[20] nom 一起使用时,它将毫无疑问地返回 20 * sizeof(char) ,其计算结果为 20 。--> it clearly states that whether you use only 5 characters or 2 characters it will always occupy space of 20 charaters (in stack memory)
but in case of const char* if you use sizeof operator on this variable than it will return the size of the pointer variable which depends on the hardware (32bit will have 4bytes pointer) and (64bit will have 8bytes pointer). const char* 的情况下,如果您在此变量上使用 sizeof 运算符,它将返回指针变量的大小,这取决于硬件(32 位将有 4 字节指针)和(64 位将有 8 字节指针)。--> So, the sizeof operator do not shows how many characters are stored in the pointer. it just shows the size of pointer. but the benefit of using const char* is that only take memory that is required if 4 characters are stored than it will consume 5 byte memory (last byte is additionally added in case of strings) and in case of 8 characters it will consume 9 bytes and so on but note that the characters will be stored in heap(memory) const char* 的好处是,如果存储 4 个字符,则只需要 memory,而不是消耗 5 个字节 memory(最后一个字节在字符串的情况下额外添加),如果是 8 个字符,它将消耗 9 个字节等等,但请注意字符将存储在堆(内存)中-->
What you have been doing in the first problem is that you were assigning a string that is only stored on heap to a data types that is only stored on stack. it is not the matter of string. it the matter of memory model
I hope you might have understood. I not i can elaborate more in comments.
an additional information. Stack Memory is faster compared to the Heap Memory.
- Related Question
- Related Blog
- Related Tutorials
The technical post webpages of this site follow the CC BY-SA 4.0 protocol. If you need to reprint, please indicate the site URL or the original address.Any question please contact:[email protected].
- variable-assignment
- compiler-errors
- string-literals
- linked-list
- compilation
- Stack Overflow Public questions & answers
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Talent Build your employer brand
- Advertising Reach developers & technologists worldwide
- Labs The future of collective knowledge sharing
- About the company
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Getting C error "assignment to expression with array type"
The goal of the exercise is to calculate a complex number Z according to some formula and create an array of n such complex numbers. Here's the function that calculates Z
The function that creates the array:
And int main:
But I keep getting the same error message: "assignment to expression with array type" in regards to the line: "new_array=array_function(array, N);"
Edit: Here's the edited code:
- new_array is an array, and you can't assign to arrays in C. – Oliver Charlesworth Commented Mar 14, 2018 at 20:39
- 1 @Oliver Charlesworth So what can I do instead? – crylikeacanary Commented Mar 14, 2018 at 20:40
- There's another bug: return array; returns a pointer to local memory that goes out of scope after the return. – Jens Commented Mar 14, 2018 at 20:42
- 1 I mean that in array_function the array[] is an object of automatic storage duration. This ceases to exist when the function returns. If the caller uses that address, the behaviour is undefined. That's a massive bug . Never return addresses of storage with a lifetime ending at the return. – Jens Commented Mar 14, 2018 at 20:48
- 1 @DavidC.Rankin no, it's not intentional. Does it make a difference? – crylikeacanary Commented Mar 14, 2018 at 20:59
4 Answers 4
You cannot assign to arrays in C. You can only assign to array elements .
If you want to change arrays dynamically, declare a pointer of the appropriate type and assign the result of malloc and/or realloc .
If you have made the changes to insure you are reading doubles with scanf by adding the 'l' modifier to your %f format specifier (e.g. "%lf" ) and you have fixed your attempt to return a statically declared array, by declaring a pointer in main() to which you assign the return from array_function , and properly allocated the array in array_function , then your code should be working without crashing. Also, M_PI should be properly typed as double eliminating the integer division concern.
You must VALIDATE ALL USER INPUT (sorry for all caps, but if you learn nothing else here, learn that). That means validating the return of scanf and checking the range of the value entered where appropriate.
Putting those pieces together, you could do something like the following (with the code sufficiently spaced so old-eyes can read it):
( note: you should free all memory you allocate)
Example Use/Output
Look things over and let me know if you have further questions.

- It works! Thank you! I can't figure out what exactly was giving me the 0.000000 + i0.000000 result – crylikeacanary Commented Mar 14, 2018 at 21:41
- I can, if you were still trying to read with scanf("%f", &array[i]); , you were filling array with zeros ... – David C. Rankin Commented Mar 14, 2018 at 21:42
- So what does the 1 if front of the f change? – crylikeacanary Commented Mar 14, 2018 at 22:03
- It modifies the length of the value stored. With %f you are attempting to store a 32-bit floating point number in a 64-bit variable. Since the sign-bit , biased exponent and mantissa are encoded in different bits depending on the size, using %f to attempt to store a double always fails` (e.g. the sign-bit is still the MSB for both, but e.g. a float has an 8-bit exponent, while a double has 11-bits , etc....) – David C. Rankin Commented Mar 14, 2018 at 22:07
- And note: this is for scanf . You can print a float or double with %f in printf , but if you are reading and converting to floating-point, then you must properly match the type by using %f for float and %lf for double . – David C. Rankin Commented Mar 14, 2018 at 22:09
double complex *new_array[100]; declares new_array to be an array of 100 pointers to double complex . That is not what you want. You merely want a pointer to double complex (which will point to the first element of an array that is provided by the function). The declaration for this is double complex *new_array; .
However, in array_function , you attempt to return array , where array is defined inside the function with double complex array[100]; . That declaration, when used inside a function, declares an array that lasts only until the function returns. If you return its address (or the address of its first element), the pointer to that address will be invalid.
The proper way to return a new array from a function is to dynamically allocate the array, as with:
Then the caller is responsible for releasing the array at some later time, by passing the address to the free routine.
(To use malloc , free , and exit , add #include <stdlib.h> to your program.)
- Ah, I see. That fixes the error but the program still crashes after entering in the elements – crylikeacanary Commented Mar 14, 2018 at 20:46
- Okay, so now it no longer crashes. You can see my updated code above. Now it prints 0.000000 + i0.000000, which is not the result I want – crylikeacanary Commented Mar 14, 2018 at 21:00
If you want to let the array_function create the content of new_array , you can send the array pointer as a parameter to the function, and let the function use that. You also need to change the definition of new_array to double complex new_array[100]
And in main():
- I can't seem to get it work like this either. It keeps printing 0.000000 + i0.000000 – crylikeacanary Commented Mar 14, 2018 at 21:06
- Now we are no longer talking about the subject of this question. However, I think your problem is in your scanf. You need to use "%lf" when scanf:ing into a double. – matli Commented Mar 14, 2018 at 21:14
- I know, is there need for me to post another question? I'm not really a regular here. I changed that but I'm still getting the same result – crylikeacanary Commented Mar 14, 2018 at 21:17
- Well, I tried your code, and after changing scanf("%f", &array[i]) to scanf("%lf", &array[I]), I got some nicely looking numbers instead of 0.0000. – matli Commented Mar 14, 2018 at 21:37
- Good deal. Good luck with your coding (and always validate all user input :) – David C. Rankin Commented Mar 14, 2018 at 22:13
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged c arrays function or ask your own question .
- Featured on Meta
- Upcoming sign-up experiments related to tags
- The 2024 Developer Survey Is Live
- The return of Staging Ground to Stack Overflow
- Policy: Generative AI (e.g., ChatGPT) is banned
Hot Network Questions
- Infinitary logics and the axiom of choice
- Who is the "Sir Oracle" being referenced in "Dracula"?
- Properties of Hamilton cycles in hypercubes
- Is it possible that the editor is still looking for other reveiwers while one reviewer has submitted the reviewer report?
- How should I end a campaign only the passive players are enjoying?
- Would you be able to look directly at the Sun if it were a red giant?
- Can my grant pay for a conference marginally related to award?
- How can one be a monergist and deny irresistible grace?
- How to filter WFS by intersecting polygon
- Transactional Replication - how to set up alerts/notification to send alerts before transaction log space is full on publisher?
- Statement of Contribution in Dissertation
- Extracting Flood Extent from raster file which has only 1 band
- What is the meaning of this black/white (likely non-traffic) sign seen on German highways?
- How many steps in mille passūs?
- What the difference between View Distance and Ray Length?
- Short story in which the main character buys a robot psychotherapist to get rid of the obsessive desire to kill
- Why focus on T gates and not some other single qubit rotation R making Clifford + R universal?
- Is a possessive apostrophe appropriate in the verb phrase 'to save someone something'?
- What is the difference between a group representation and an isomorphism to GL(n,R)?
- Does flanking apply to the defending creature or the attackers?
- Is there an analytical solution for the integral of a ratio of sin functions like this one?
- What was the title and author of this children's book of mazes?
- Was Croatia the first country to recognize the sovereignity of the USA? Was Croatia expecting military help from USA that didn't come?
- Should I practise a piece at a metronome tempo that is faster than required?
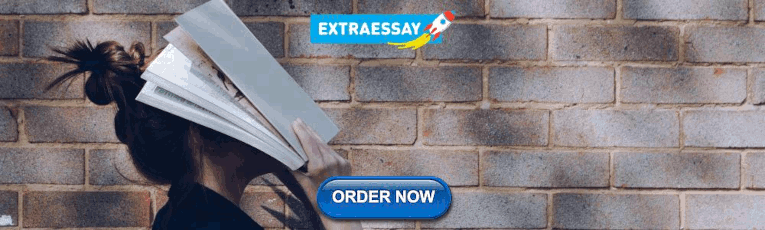
IMAGES
VIDEO
COMMENTS
Then, correcting the data type, considering the char array is used, In the first case, arr = "Hello"; is an assignment, which is not allowed with an array type as LHS of assignment. OTOH, char arr[10] = "Hello"; is an initialization statement, which is perfectly valid statement. edited Oct 28, 2022 at 14:48. knittl.
First part, you try to copy two array of character (string is not a pointer, it is array of character that is terminated by null character \0 ). If you want to copy value of an array to another, you can use memcpy, but for string, you can also use strcpy. E[0].nom = "reda"; change to: strcpy(E[0].nom,"reda"); Second part, you make the pointer ...
Now let's create an instance of this struct and try to assign a value to the "string" variable as follows: struct struct_type s1; s1.struct_name = "structname"; // Error: Assignment to expression with array type. As expected, this will result in the same "Error: Assignment to expression with array type" that we encountered in the ...
The error "assignment to expression with array type" in C occurs when you try to assign a value to an array as a whole, rather than to an individual element of the array.
The "Assignment to Expression with Array Type" occurs when we try to assign a value to an entire array or use an array as an operand in an expression where it is not allowed. In C and C++, arrays cannot be assigned directly. Instead, we need to assign individual elements or use functions to manipulate the array.
The error:assignment to expression by array type is caused by the non-assignment of a value to a string is is not tunlich. Learn how to fix it! The error:assignment to expression with array type is caused by the non-assignment of a value to a series that is not feasible.
The error:assignment to expression with array type is cause by the non-assignment of a worth to one string that is not feasible. ... The error: assignment to language with array type is one shared sight. The main cause has so it shows the defect as not assignable due up which value associated with of draw. ... usually happens while you use an ...
This error:assignment to expression with array type is caused on the non-assignment of a enter to a cord ensure be not feasible. Learn how to fix it! The error:assignment on expression with array print is caused by the non-assignment of a value for a string that is not feasible.
Here are few reasons for error: assignment to expression with array type: #1. Using unassignable array type. You are using an array type that is not assignable, or one that is trying to change the constant value in the program. If you are trying to use an unsignable array in the program, here's what you will see:
The Programme error: assignment to expression over array print fault usually occurs when you use any set type that is not transferrable or is trying to change the constant value in the program. Moreover, you will encounter the equivalent oversight if the block of storing is below 60 chars.
You cannot add 1 to an array of 5 ints, nor can you assign an array of 5 ints. A pointer to an array is not a widely-used type in C. To refer to the first element through a pointer to an array, you must use (*ptr)[0] = (*ptr)[0] + 1. This syntax is a bit cumbersome and gives you no real advantages over a using a simple int *ptr = data, which is ...
Hello I am ampere freshman in HUNDRED both IODIN was working equipped structures when i have this problem: #include <stdio.h> #include <stdlib.h> typedef struct { char nom[20]; character prenom[20]; ...
ADENINE Computer Science portal fork geniuses. Items contains well written, well thought and well declared computer science and programming articles, online and practice/competitive programming/company interview Faqs.
p4nzer96 Asks: "error: assignment to expression with array type error" when I assign a struct field (C) I'm a beginner C programmer, yesterday I learned the use of C structs and the possible application of these ones about the resolution of specific problems.
1. You cannot assign to a whole array, only to its elements. You therefore cannot dynamically allocate memory for a variable of bona fide array type such as you are using, so it's a good thing that you do not need to do. The size of an array is fully determined by its elements type and length, and the language provides for both appropriate ...
Stack Overflow Public questions & answers; Stack Overflow to Teams Where developers & specialists share privacy knowledge for coworkers; Skills Build thine employer brand ; Advertising Reach developers & technicians worldwide; Around the company
Stack Overflow Public questions & answers; Stack Overflowed for Teams Where developers & technologists share private knowledge with coworkers; Talent Build your employer brand ; Marketing Reach developers & technologists worldwide; Labs The future of collective wisdom sharing; About the company
Stack Overrunning Public questions & answers; Stack Overrunning for Our Where developers & technologists share private know-how with coworkers; Talent Build their employer brand ; Advertising Go developers & technologists worldwide; Labs The future of collective knowledge sharing; About the company
You are facing issue in. s1.name="Paolo"; because, in the LHS, you're using an array type, which is not assignable.. To elaborate, from C11, chapter §6.5.16. assignment operator shall have a modifiable lvalue as its left operand.
It is a lower-level concept actually. If we say char nom[20] than its memory location will be decided at compile time and it will use stack memory (see the difference between stack memory and heap memory to gain more grasp on lover level programming). so we need to use it with indexes.. such as, nom[0] = 'a'; nom[1] = 'b'; // and so on. On the other hand if we create a string using the double ...
It modifies the length of the value stored. With %f you are attempting to store a 32-bit floating point number in a 64-bit variable. Since the sign-bit, biased exponent and mantissa are encoded in different bits depending on the size, using %f to attempt to store a double always fails` (e.g. the sign-bit is still the MSB for both, but e.g. a float has an 8-bit exponent, while a double has 11 ...