Variables in Pseudocode
Variables are a very easy topic to understand with pseudocode. I cannot stress how easy using, assigning, and comparing variables are. It is really easy to grasp this, so we will be going over how-to assigning variables, how to use them (for conditionals, recursive functions with parameters and more), how to override variables in pseudocode and finally how to some basics about them!
We follow the pseudocode standard set by the main computer science exam board in the UK, AQA, allowing a universal set of functions and operators to be used. This makes it much easier for programmers to understand each other's code without comments!
- Variables store values and data of each different data type. This means we can then later access this data by referencing the variable name.
- Variables can store all 5 different types of data in pseudocode. Boolean, Integer, Character, String, Float/Decimal/Real. This allows us to do a lot of things in our algorithms.
- We can reference the variables anywhere AFTER we have declared them, allowing us to pick out the value inside of them, to then use for conditional statements, loops, or even mathematical operations.
- The value of a constant never changes during program runtime, meaning that while the programming is running, the value cannot be reassigned. So when you first assign a value to a constant, make sure it is correct.
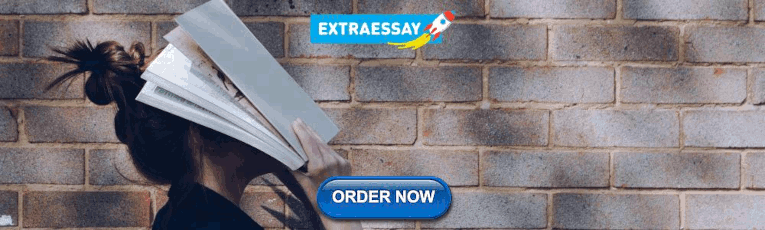
How to Use Variables in Pseudocode
Variables are the best way to hold values in pseudocode, which can then be used throughout the program by just referencing the name of that variable! Cool, right?
Variable Declaration
In pseudocode, it is very easy to declare variables! We just need to mention the variable name then assign a value to it. For example, if we were making a calculator app, we would make a variable called number . We could then use this later to perform our calculations, which we will get into later!
For constants it is very similar, however when declaring them, we must make sure to put their name in capital letters. For example if we were storing the user's input as a constant to then perform calculations on, we would say INPUT and then assign our value to it. This is to distinguish both variables and constants from each other in pseudocode, which helps wen working in teams of multiple developers! The value of a constant never changes during the run-time of the program
In pseudocode, when assigning values to a variable or constant, it is best practice to use the assignment arrow (←).
So, say we have just declared the variable number , we will want to assign a value to that. So, if we were taking the user input, we would use number ← USERINPUT, as seen in the example above. This assigns the user's input from an output or prompt field and then allow us to use that data. We can also just assign regular data to it, for example a string. ExampleString ← "pseudoeditor.com is awesome" , would be a valid assignment.
Using Variables
It is really easy to access the data within a variable. Say we were performing some calculations on an integer that the user entered, we would use the variable name then the operation we will be performing. We would use the number variable we assigned a value to earlier and say, number * 5 , if it was an integer.
It can just be a simple thing like outputting the variable back to the user, in that case we would just use the output feature and say, OUTPUT number !
.css-1v0lc0l{color:var(--chakra-colors-blue-500);} Try our pseudocode editor.
Give our pseudocode editor a go today for free - with a built-in compiler , tools to convert pseudocode to code , and project saving , PseudoEditor makes writing pseudocode easier than ever!
That is it! You have successfully declared, assigned a value to, and then used variables and constants in pseudocode. It was easy wasn't it? Now, lets move you onto a more advanced guide! You can read more of our guides and then get even better at pseudocode, without even leaving our site!
Read Our Guide on .css-vzy2dl{font-family:'Montserrat',sans-serif!important;color:var(--chakra-colors-yellow-500);} Subroutines in Pseudocode
Read our guide on file handling in pseudocode.
© 2024 PseudoEditor.com | All rights reserved
Useful Links
- Analysis of Algorithms
- Backtracking
- Dynamic Programming
- Divide and Conquer
- Geometric Algorithms
- Mathematical Algorithms
- Pattern Searching
- Bitwise Algorithms
- Branch & Bound
- Randomized Algorithms
How to write a Pseudo Code?
Pseudo code is a term which is often used in programming and algorithm based fields. It is a methodology that allows the programmer to represent the implementation of an algorithm. Simply, we can say that it’s the cooked up representation of an algorithm. Often at times, algorithms are represented with the help of pseudo codes as they can be interpreted by programmers no matter what their programming background or knowledge is. Pseudo code, as the name suggests, is a false code or a representation of code which can be understood by even a layman with some school level programming knowledge. Algorithm : It’s an organized logical sequence of the actions or the approach towards a particular problem. A programmer implements an algorithm to solve a problem. Algorithms are expressed using natural verbal but somewhat technical annotations. Pseudo code: It’s simply an implementation of an algorithm in the form of annotations and informative text written in plain English. It has no syntax like any of the programming language and thus can’t be compiled or interpreted by the computer.
Advantages of Pseudocode
- Improves the readability of any approach. It’s one of the best approaches to start implementation of an algorithm.
- Acts as a bridge between the program and the algorithm or flowchart. Also works as a rough documentation, so the program of one developer can be understood easily when a pseudo code is written out. In industries, the approach of documentation is essential. And that’s where a pseudo-code proves vital.
- The main goal of a pseudo code is to explain what exactly each line of a program should do, hence making the code construction phase easier for the programmer.
How to write a Pseudo-code?
- Arrange the sequence of tasks and write the pseudocode accordingly.
- Start with the statement of a pseudo code which establishes the main goal or the aim. Example:
- The way the if-else, for, while loops are indented in a program, indent the statements likewise, as it helps to comprehend the decision control and execution mechanism. They also improve the readability to a great extent.
- Use appropriate naming conventions. The human tendency follows the approach to follow what we see. If a programmer goes through a pseudo code, his approach will be the same as per it, so the naming must be simple and distinct.
- Use appropriate sentence casings, such as CamelCase for methods, upper case for constants and lower case for variables.
- Elaborate everything which is going to happen in the actual code. Don’t make the pseudo code abstract.
- Use standard programming structures such as ‘if-then’, ‘for’, ‘while’, ‘cases’ the way we use it in programming.
- Check whether all the sections of a pseudo code is complete, finite and clear to understand and comprehend.
- Don’t write the pseudo code in a complete programmatic manner. It is necessary to be simple to understand even for a layman or client, hence don’t incorporate too many technical terms.
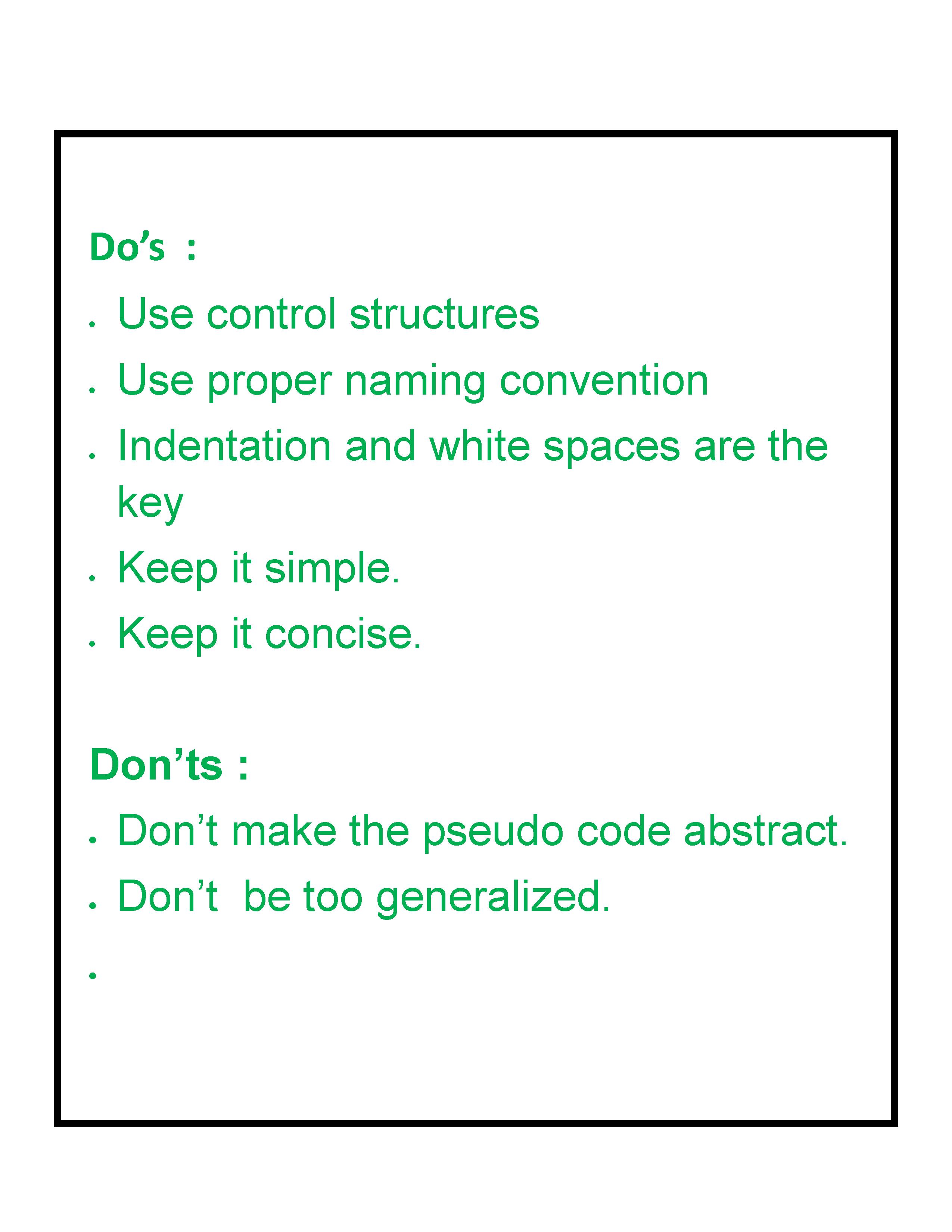
Dos and Don’ts to Pseudo Code Writing
Let’s have a look at this code
Similar Reads
- Advanced Data Structure
- Computer Subject
- Algorithms-Misc
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
- Create account
- Contributions
- Discussion for this IP address
GCSE Computer Science/Pseudocode
Pseudocode is a non-language-specific way of writing code. It is used during the design phase of a project as a quick way of devising algorithms before the language to be used is known and without needing to spend too much time using the exact syntax correct. Once pseudocode algorithms have been written it should then be easier to use this to help write the program code. Pseudocode is a structured way of writing algorithms which looks very much like program code.
Since pseudocode is an informal way of writing code there are different ways of writing it even at GCSE level. You should be aware both of what pseudo you are expected to be able to read and how you should write code for your course. Also you should be aware of whether or not a pseudocode fact sheet is provided.
- AQA: understand their pseudocode; use any reasonable pseudocode to answer exam question; no pseudocode fact sheet in exam.
Defining Variables
When devising an algorithm the programmer will need to use variables and assign values to them. Many languages require a variable to be defined and given a data type before use.
The five basic data types that can be used here include:
Variable Assignments
Assigning a value to a variable is indicated in pseudocode using an arrow symbol (←). The arrow points from the value being assigned towards the variable it is being assigned to.
The following line of pseudocode should be read as 'a becomes equal to 34'.
Totalling and Counting
The assignment operator can also be used when finding totals, as shown in the following example where x becomes equal to a plus b.
Similarly we can also use the assignment operator for counting, by assigning a variable to become equal to the value of itself plus 1.
Input and Output
Output is the act or returning some data to the user of the program. This could be in the form of a written message, a numerical value, an image, video or sound. This is shown in pseudocode by writing OUTPUT followed by what the output will be. The example below shows how we would output the message 'Hello World':
Sometimes the word PRINT may be used instead of OUTPUT, as in the following example:
Input on the other hand is when we expect some value to be provided by the user. The following example will output a message asking the user to input their name and assign it to a variable called 'name'.
The word READ can also be used instead of INPUT.
The use of selection allows decisions to be made within an algorithm at run-time based on certain conditions.
IF THEN ELSE
An IF statement starts with a condition which is tested. If the condition evaluates as TRUE then the THEN code block will be run. If the condition evaluates as false then the ELSE block will run. In the following example "Hello" will be printed if x = 1, otherwise "Good night" will be printed.
Often ELSE IF is used when there is more than one condition to check. The following example would print "Hello" if x = 1, or "How are you?" if x = 2 or will otherwise print "Goodbye".
CASE OF OTHERWISE ENDCASE
This structure is used when there are many possible outcomes to a condition. For instance, to extend the above example, suppose you wanted the program to print a different message depending on the value of a variable, xː
In this example if x = 1 then we print "Hello", if x =2 then "How are you?", if x = 3 then "I am fine." or if x = 4 then we print "Have a good dayǃ". If x is equal to anything else then we print "Goodbye".
Iteration is used when we need to repeat a block of code. We can make a code block repeat either for a given number of repetitions or continuously either for as long as a condition continues to be true or until a condition becomes true.
FOR TO NEXT
The FOR loop is used to repeat code for a given number of repetitions. We specify a counter variable and set it to an initial value and then specify an end value. After every iteration of the loop the counter variable is automatically incremented by 1. In the following example x starts at 1 and increases by 1 every time the code repeats until it reaches a value of 10, then the loop will terminate.
REPEAT UNTIL
A REPEAT loop will repeat the code block until the given condition is true. The condition is not checked until after the code has run once, so regardless of whether the condition is true or not the code will always run at least once. The following example will continue to take user input until the user inputs a value less than 10. This structure is often used for validation checks.
WHILE DO ENDWHILE
In a WHILE loop the code block will run, and continue to run, until the given condition is no longer true. A WHILE loop is similar to the REPEAT loop in that it decides when to terminate based on a condition. However the while loop checks the condition prior to running the first time. If the condition is not true then the code block will never run.
The below example is also used for input validation.
- Book:GCSE Computer Science
Home > What Is Pseudocode?
Pseudocode: What Is It and How Do You Write It?
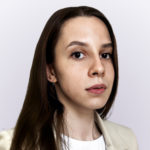
Table of Contents
What is pseudocode, how to write pseudocode, the do’s of writing pseudocode, the don’ts of writing pseudocode, how to solve programming problems by using pseudocode, expert opinion.
You might have already heard of such a term as pseudocode, especially if you are a software engineer. Pseudocode enables developers and non-developers to write the logic of code in a clear and free style. In spite of the fact that it does not contain real code, it is more than just a rough sketch of a software project. Pseudocode is a valuable tool to express algorithms and navigate the intricacies of coding independently of any specific programming language syntax. This is not all.
In this article, we will answer the “ What is pseudocode ?” question in detail. Moreover, we will discuss how to write it and how it can be helpful for addressing various programming issues.
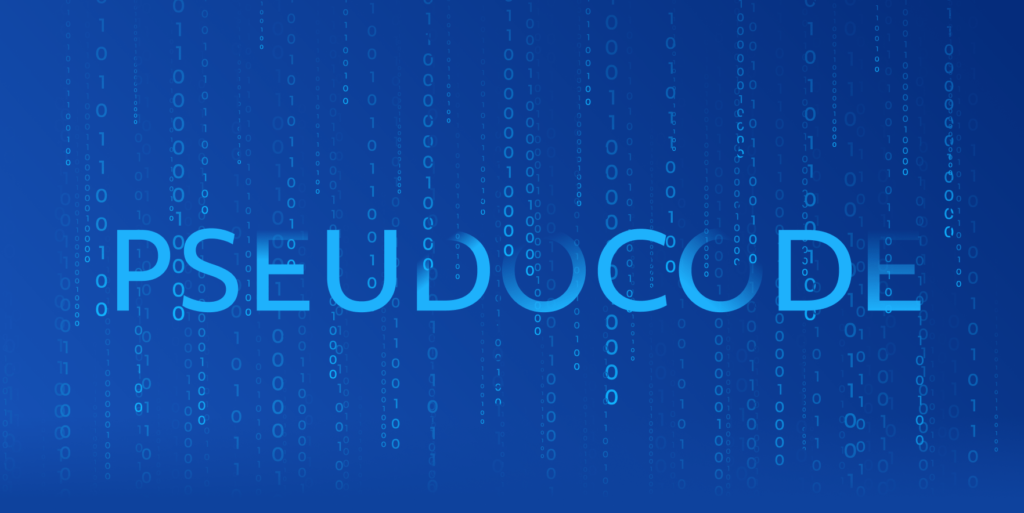
Pseudocode is a versatile concept that extends beyond programming and is used in numerous fields, such as mathematics or design. As the name implies, pseudocode is “fake” code. In other words, it is an informal way of designing the logic and the structure of an application or algorithm. Unlike specific programming languages with rigid syntax rules, pseudocode uses human-readable language to describe logical steps of specific actions. For this reason, it does not have a standard writing format. So, it may vary widely in style from person to person.
Pseudocode consists of essential elements common across various algorithms:
- Variables : represented using simple names to denote data storage;
- Operations : basic operations such as assignment, arithmetic, and conditional statements;
- Loops : described using intuitive language like “while” or “for”;
- Functions/Procedures : represented as named blocks of code with parameters and returns.
Software developers often use it to formulate and build algorithms before the coding stage. Then, to turn it into machine-readable code, they must translate it into their preferred coding language. By creating it first, programmers can focus on the algorithm’s design (as well as how it will function) rather than worrying about the quality of syntax.
Often, developers use both flowcharts and pseudocode in conjunction. While pseudocode is a text-based format that provides a detailed description of the algorithm, flowcharts use a graphical format (diagram) to illustrate the flow of decisions. Thus, developers can plan an algorithm’s logic effectively. So, among other advantages, it promotes a more thought-out approach to programming.
Advantages of pseudocode
It is highly beneficial to design a program in pseudocode for a wide range of reasons, including but not limited to the following:
- Easy to write, understand, and read : pseudocode uses natural language structures and basic concepts, making it easy to read and understand;
- Easy to convert : since it is written in a free style, it can be translated into any coding language;
- Easy to collaborate : unlike scripting language that can be difficult for understanding, it provides a common ground for team members (both tech and non-tech specialists) to work together effectively;
- Easy to change : it is more concise, less complex, and readable than actual code, thus offering a simpler way to incorporate changes before the product is developed;
- Reduces the number of bugs : it allows developers to identify potential bugs before they occur in real programs, thus increasing the speed and quality of the development process;
- Better problem understanding : using pseudocode first, developers can see the logic behind a solution before designing the actual code.
The main challenges of pseudocode
When it comes to the disadvantages of pseudocode, keep in mind the following:
- Lack of a formal standard: pseudocode can differ from one organization to another because there are no standard formats to write it. This can result in inconsistency and ambiguity in its usage, especially if it’s shared between the teams;
- Time-consuming: it can be time-consuming, as the process of translating the pseudocode into actual code may require additional effort and meticulous planning;
- Hard to verify: computers cannot compile or execute pseudocode, so its correctness cannot be verified;
- Complex to map process: due to the lack of visual representation of the process, it can be challenging to convey complicated procedural logic clearly.
In general, pseudocode is a valuable tool that helps the team understand how the final product is supposed to function. And despite the lack of a standard format for pseudocode, there are several universal tips on how to create it – let’s take a look.
As we said above, there is no definite and universal way to write pseudocode due to the lack of a standard format. Although pseudocode can be generated in many ways, it has certain common and structured elements that must be included. They are:
- Sequence: consists of a series of steps performed in a specific order;
- While: a conditional loop that runs until a certain condition is met;
- If-Then-Else: a conditional statement that takes different actions depending on whether it is true or false;
- Repeat-Until: a loop that continues until a specified condition becomes true;
- For: a loop that repeats for a specific number of times;
- Case: the generalization form of If-Then-Else.
Let’s look at a pseudocode example for a better explanation.
A pseudocode example
Before diving into coding, make sure you know what your pseudocode is supposed to accomplish. Let’s say your intended program will allow users to determine whether a number is even or odd. So, pseudocode may look something like this:
Explanation: the first step prompts the user to input a number. The number serves as the initial data for the subsequent calculations. Then, we calculate the remainder by dividing the number by 2. After that, we use an “If-Else” statement to check if the remainder equals 0. If true, the program outputs “The number is even.” If false, the program outputs “The number is odd.” Thus, it shows a simple decision-making process and describes how an even number is determined.
Remember, it was just an example for creating this program; the approaches may differ greatly.
Pseudocode effectiveness comes from the ability to clearly explain the algorithm logic. So, for clear and readable pseudocode, be sure to follow these tips:
- Start with a description of the purpose of the process;
- Write only one statement per line;
- Focus on logic, not syntax;
- Use clear terminology;
- Keep the proper order of your pseudocode;
- Use standard programming structures, like “if-then” and “while,” else”;
- Organize your pseudocode sections;
- Double-check your pseudocode.
To ensure clarity, conciseness, and effectiveness of pseudocode, it is equally important to know pitfalls to prevent when designing pseudocode. Here are a few of the main considerations:
- Avoid extra details that are better for actual code;
- Do not skip indentation;
- Do not use ambiguous or vague terms;
- Do not overcomplicate;
- Do not ignore structure;
- Do not use programming language keywords;
- Do not skip testing.
Now that we better understand the main do’s and don’ts, let’s review the steps that developers may find helpful during coding.
For developers, pseudocode may simplify the development process. Rather than focusing solely on technical details like coding syntax and structure, developers can prioritize the logical part. Here are some steps that developers can take during pseudocoding:
Step 1. Understand the problem When it comes to coding, the understanding of the problem is vital (a task you need to accomplish). Pseudocode allows developers to describe their interpretation of the problem in natural language. In other words, developers will identify the problem’s inputs, outputs, and specific conditions before moving forward.
Step 2. Understand the question If programmers understand the issue(s), they outline the steps necessary to solve the problem and hence comes to a solution. Thus, a clear understanding of all aspects of the issue is the next step towards a solution.
Step.3 Break down the problem Divide the problem into smaller sub-problems or tasks to allow you to solve it more easily. By solving a small problem, you are getting closer to solving a big one. After solving each step of the problem, check the result to see if you are on the right track. Keep solving these small problems until you find a solution to the problem.
Step.4 Bring in actual code and tools When the problem is thoroughly explored and broken down into manageable steps, developers can smoothly transition from pseudocode to real coding. Pseudocode serves as a template for implementation, easing the coding process. This way, programmers can choose the most appropriate software constructs, logic flow, and algorithmic solutions. Many websites are available to help with this, such as Mozilla Developer Network, W3Schools, Stack Overflow, and others.
By following these steps, developers can leverage pseudocode as an effective tool to conceptualize, design, and explain programming solutions before diving into more detailed coding procedure.
Pseudocode in programming is not an indispensable solution for solving programming issues across developers. Instead, it is a helpful method for uncovering ambiguous decisions regarding a specific problem in web development, data science, design, and other fields. Therefore, the understanding of “what is pseudocode and how to deal with it” lays the foundation for crafting code that works and is concise.
Pseudocode is an excellent way to describe the process without spending much time writing code. Nowadays, there are more options for describing the steps of a program. It is also a good solution to use flowcharts with pseudocode together, if time is not a problem, to help individuals see the code parts more clearly. Aside from that, I would like to emphasize that writing pseudocode can be a good way to train young developers as well.
.NET Developer at SoftTeco
Sergey Ostapuk
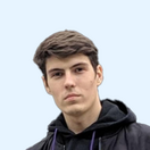
We will be happy to hear your thoughts Cancel reply
I agree with Privacy Policy and Terms of Services
Read More in Our Blog
Mvp vs mlp: what to choose.
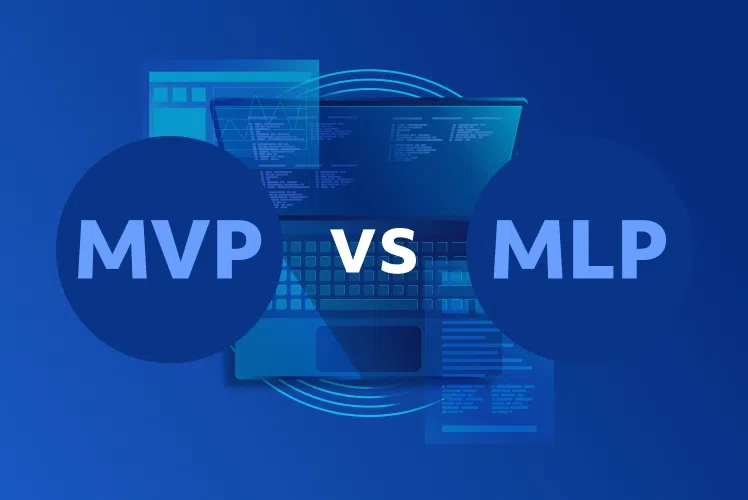
In the world of software development, a Minimum Viable Product (MVP) is considered a surefire way to start a project and test the idea. However, many believe that you can take it a step further and create a Minimum Lovable Product (MLP) instead. So is MVP really outdated, and when should you ...
Minimum Viable Product (MVP) Development 101: The Main Do’s and Don’Ts
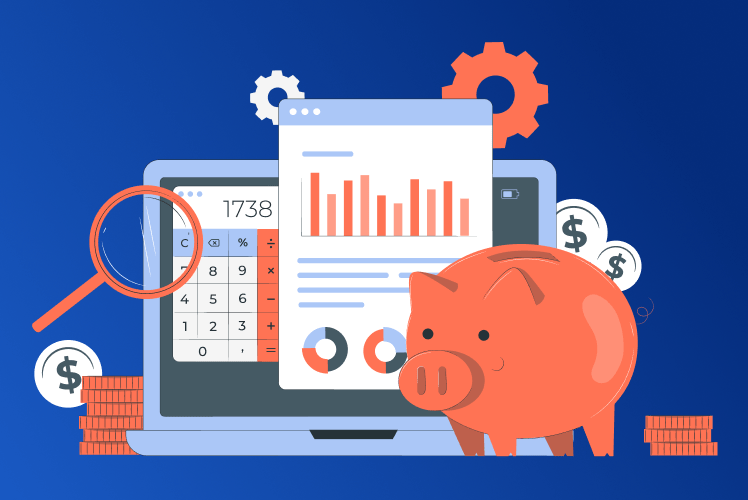
1The MVP is not dead and here is why2The main steps of MVP development3Best practices for creating an MVP4Summing up Say, you have this amazing idea for a software product but you are not too sure about whether it’s going to be a success or not. How do you test your idea with real users without ...
Want to stay updated on the latest tech news?
Sign up for our monthly blog newsletter in the form below.
Headquarters
82 Laisves al., Kaunas, 44250, Lithuania
- IT Consulting
- Software Development
- Web Development
- Mobile App Development
- UI/UX Design
- Quality Assurance
- Machine Learning, AI, Data Science, Big Data
- Salesforce Development
- DevOps Services
- Support and Maintenance
- Technologies
Reviews on other sites
Copyright © 2008-2024 SoftTeco ®
- Privacy Policy
- Terms of Services
- Cookie Settings

- Discover All Services
- Discover IT Consulting
- Software Development Consulting
- Web Development Consulting
- Digital Transformation Consulting Services
- Cloud Migration Consulting
- Big Data Consulting
- Machine Learning Consulting
- Salesforce Consulting
- UX Consulting
- Ecommerce Consulting
- Discover Software Development
- Custom Software Development
- API Development
- Nearshore Software Development
- Cloud Integration
- Outsourcing Software Development
- IT Outsourcing
- IT Outstaffing
- Healthcare Software Development
- MVP Development
- MEAN Stack Development
- MERN Stack Development
- Cyber Security
- Database Development
- IoT Software Development
- Software Development for Startups
- Enterprise Software Development
- Code Review
- GDPR Compliance
- HR Software Development
- Neobank Development Company
- Discover Web Development
- Magento Development
- Ecommerce Web Development
- Medical Website Development
- WordPress Development Services
- Drupal Development
- Shopify Development Services
- BigCommerce Development
- Discover Mobile App Development
- Fitness App Development
- Healthcare Mobile Development
- Ecommerce App Development
- Mobile Banking App Development
- White Label Applicationfor IoT Device Management
- Discover UI/UX Design
- Mobile App Design
- Website Design
- Discover Quality Assurance
- Security Testing
- Penetration Testing
- Stress Testing
- Performance Testing
- Discover Machine Learning, AI, Data Science, Big Data
- Machine Learning Development
- AI Development
- Data Visualization Services
- Big Data Services
- Discover All Technologies
- Discover Backend
- Discover Frontend
- Discover Mobile
- Discover iOS
- Objective-C
- Discover Android
- Discover Cross-Platform
- React Native
- Discover Cloud Solutions
- DigitalOcean
- Google Cloud
- Machine Learning, AI, Data Science, Big Data Services & Technologies
- QA Automation
- Discover All Industries
- Financial and Banking
- Media and Entertainment
- Elearning and Education
- Telecommunications
- Manufacturing
- Transport and Logistics
- Agriculture
- Sports and Lifestyle
- Tourism and Hospitality
- Oil and Gas
- Professional Services
- IT Services
- About SoftTeco
- Information Security
- Referral Program
- Corporate Responsibility
- Working at SoftTeco
- Current Job Openings
- Career Start
- PRO Courses Guides New Tech Help Pro Expert Videos About wikiHow Pro Upgrade Sign In
- EDIT Edit this Article
- EXPLORE Tech Help Pro About Us Random Article Quizzes Request a New Article Community Dashboard This Or That Game Forums Popular Categories Arts and Entertainment Artwork Books Movies Computers and Electronics Computers Phone Skills Technology Hacks Health Men's Health Mental Health Women's Health Relationships Dating Love Relationship Issues Hobbies and Crafts Crafts Drawing Games Education & Communication Communication Skills Personal Development Studying Personal Care and Style Fashion Hair Care Personal Hygiene Youth Personal Care School Stuff Dating All Categories Arts and Entertainment Finance and Business Home and Garden Relationship Quizzes Cars & Other Vehicles Food and Entertaining Personal Care and Style Sports and Fitness Computers and Electronics Health Pets and Animals Travel Education & Communication Hobbies and Crafts Philosophy and Religion Work World Family Life Holidays and Traditions Relationships Youth
- Browse Articles
- Learn Something New
- Quizzes Hot
- Happiness Hub
- This Or That Game
- Train Your Brain
- Explore More
- Support wikiHow
- About wikiHow
- Log in / Sign up
- Computers and Electronics
- Programming
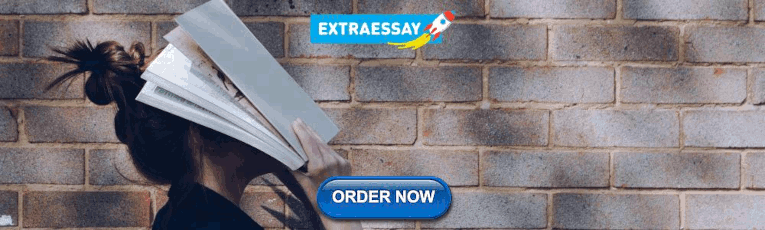
Learn to Write Pseudocode: What It Is and Why You Need It
Last Updated: February 23, 2023 Fact Checked
Pseudocode Basics
Writing good pseudocode, creating an example pseudocode document.
This article was co-authored by wikiHow staff writer, Kyle Smith . Kyle Smith is a wikiHow Technology Writer, learning and sharing information about the latest technology. He has presented his research at multiple engineering conferences and is the writer and editor of hundreds of online electronics repair guides. Kyle received a BS in Industrial Engineering from Cal Poly, San Luis Obispo. This article has been fact-checked, ensuring the accuracy of any cited facts and confirming the authority of its sources. This article has been viewed 1,090,798 times. Learn more...
Want to learn how to write pseudocode? Pseudocode is a step-by-step written outline of your code that you can transcribe into the programming language you’re working with. In other words, you’re writing the flow of your code in plain language rather than official coding syntax. Many programmers use pseudocode to plan out the logic of an algorithm before writing it in code. This wikiHow shows you how to write a pseudocode document for your computer program.
Things You Should Know
- Pseudocode is useful for planning your program and troubleshooting the logic.
- Use a simple plain-text editor or even pen and paper to write your pseudocode.
- Write a statement for each line of logic in your program.

- Describing how an algorithm should work. Pseudocode can illustrate where a particular construct, mechanism, or technique could or must appear in a program.
- Explaining a computing process to less-technical users. Computers need a very strict input syntax to run a program, but humans (especially non-programmers) may find it easier to understand a more fluid, subjective language that clearly states the purpose of each line of code.
- Designing code in a group setting. High-level software architects will often include pseudocode in their design process to help solve a complex problem they see their programmers running into. If you are developing a program with other coders, you may find that pseudocode helps make your intentions clear.
Pseudocode serves as a guide for thinking through programming problems, and a communication option that can help you explain your ideas to other people.

- If you are working with others on a project—whether they are your peers, junior programmers, or non-technical collaborators—it is important to use at least some standard structures so that everyone else can easily understand your intent.
- If you are enrolled in a programming course at a university, a coding camp, or a company, you will likely be tested against a taught pseudocode "standard". This standard often varies between institutions and teachers.
Clarity is a primary goal of pseudocode, and it may help if you work within accepted programming conventions. As you develop your pseudocode into actual code, you will need to transcribe it into a programming language – so it can help to structure your outline with this in mind.

- You can use pen and paper to write your pseudocode! Try different formats to find what works best for your creative programming process.
Plain-text editors include Notepad (Windows) and TextEdit (Mac).

- For example, a section of pseudocode that discusses entering a number should all be in the same "block", while the next section (e.g., the section that discusses the output) should be in a different block.

- For example, if you use "if" and "then" commands in your pseudocode, you might want to change them to read "IF" and "THEN" (e.g., "IF <condition> THEN <output result>").

You might even want to get rid of any coding commands altogether and just define each line's process in plain language. For example, "if input is odd, output 'Y'" might become "if user enters an odd number, display 'Y'".

- This will make writing the actual code easier, since your code will run top-down.

- if CONDITION then INSTRUCTION — This means that a given instruction will only be conducted if a given condition is true. "Instruction", in this case, means a step that the program will perform, while "condition" means that the data must meet a certain set of criteria before the program takes action. [3] X Research source
- while CONDITION do INSTRUCTION — This means that the instruction should be repeated again and again until the condition is no longer true. [4] X Research source
- do INSTRUCTION while CONDITION — This is very similar to "while CONDITION do INSTRUCTION". In the first case, the condition is checked before the instruction is conducted, but in the second case the instruction will be conducted first; thus, in the second case, INSTRUCTION will be conducted at least one time.
- function NAME (ARGUMENTS): INSTRUCTION — This means that every time a certain name is used in the code, it is an abbreviation for a certain instruction. "Arguments" are lists of variables that you can use to clarify the instruction.

- Brackets—both square [code] and curly {code}—can help contain long segments of pseudocode.
- When coding, you can add comments by typing "//" on the left side of the comment (e.g., //This is a temporary step. ). You can use this same method when writing pseudocode to leave notes that don't fit into the coding text.

- Would this pseudocode be understood by someone who isn't familiar with the process?
- Is the pseudocode written in such a way that it will be easy to translate it into a computing language?
- Does the pseudocode describe the complete process without leaving anything out?
- Is every object name used in the pseudocode clearly understood by the target audience?
- If you find that a section of pseudocode needs elaboration or it doesn't explicitly outline a step that someone else might forget, go back and add the necessary information.

Community Q&A

- Pseudocode is optimal for complex programs that are hundreds to thousands of lines in length. Thanks Helpful 4 Not Helpful 0

- Pseudocode cannot be substituted for actual code when creating a program. Pseudocode can only be used to create a reference for what the code should do. Thanks Helpful 3 Not Helpful 0
You Might Also Like

- ↑ https://www.techopedia.com/definition/3946/pseudocode
- ↑ https://www.youtube.com/watch?v=D0qfR606tVo
- ↑ https://www.ibm.com/support/knowledgecenter/en/SSLTBW_2.3.0/com.ibm.zos.v2r3.ikjc300/ifthen.htm
- ↑ https://users.csc.calpoly.edu/~jdalbey/SWE/pdl_std.html
About This Article

1. Write the purpose of the process. 2. Write the initial steps that set the stage for functions. 3. Write only one statement per line. 4. Capitalize the initial keyword of each main direction. 5. Write what you mean, not how to program it. 6. Use standard programming structures. 7. Use blocks to structure steps. 8. Add comments if necessary. Did this summary help you? Yes No
- Send fan mail to authors
Is this article up to date?

Featured Articles

Trending Articles

Watch Articles

- Terms of Use
- Privacy Policy
- Do Not Sell or Share My Info
- Not Selling Info
wikiHow Tech Help Pro:
Level up your tech skills and stay ahead of the curve

Writing Pseudocode: A Beginner's Guide
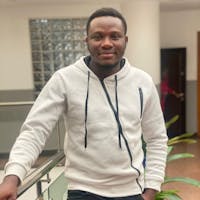
Pseudocode is a valuable tool for expressing algorithms in a high-level and abstract manner. It serves as a bridge between human understanding and actual programming languages. In this blog post, we will explore what pseudocode is, and its purpose, and provide examples of its usage in real-life scenarios.
What is Pseudocode?
Pseudocode is not an actual programming language but a representation of code that resembles a programming language without adhering to its strict syntax rules. The term pseudo refers to something false or fake, indicating that pseudocode is a simulated version of code. It allows programmers to focus on the logic and functionality of an algorithm rather than the specific language syntax.
The Purpose of Pseudocode
The primary purpose of pseudocode is to provide a high-level and abstract representation of an algorithm, independent of any particular programming language. By using pseudocode, programmers can simplify complex problems, avoid language-specific limitations, and facilitate understanding and implementation across different languages.
Pseudocode acts as a communication tool between programmers, enabling them to discuss and share algorithms without being constrained by specific programming languages. It allows for flexibility, as the same algorithm can be implemented in different languages based on the pseudocode's logic and structure.
Exam Board Pseudocode
In academic settings, exam boards often use standardized pseudocode in their questions and marking schemes. While these standardized versions of pseudocode may differ slightly between exam boards, they serve the purpose of ensuring consistency and ease of evaluation. However, it's important to note that these specific syntax rules are often only applicable within the context of exams and may not reflect real-world pseudocode usage.
Real-Life Examples
To understand how pseudocode is used in practice, let's examine some real-life examples. One such example can be found in university textbooks, where complex algorithms are explained using pseudocode. These textbooks often present machine learning algorithms, such as decision trees, and provide pseudocode representations to illustrate their functionality.
While the pseudocode in textbooks may appear different from what you encounter in exams, the core concepts remain the same. Pseudocode still utilizes familiar programming constructs like functions , parameters , conditionals ( if-else statements ), loops , and variables . The notation used in these examples simplifies the algorithm's implementation while excluding language-specific details.
Using pseudocode instead of actual programming languages has several advantages. Pseudocode condenses complex algorithms into concise representations, making them easier to understand. It also allows for algorithm portability, enabling programmers to implement the same logic across different programming languages. Additionally, pseudocode serves as a future-proof solution since it remains relevant even as programming languages evolve.
Understanding Pseudocode
Pseudocode is a simulated version of code that resembles a programming language but does not adhere to its strict syntax rules. It aims to focus on the logic and functionality of an algorithm, making it independent of any specific programming language. Pseudocode facilitates communication among programmers and enables the implementation of algorithms across different languages.
Writing Pseudocode
When writing pseudocode, remember that there is no one correct syntax. It's more important to focus on the algorithm's logic and functionality rather than adhering to specific formatting rules. Pseudocode should be clear and easily understandable by competent programmers familiar with the problem domain.
To illustrate the process of writing pseudocode, let's consider an example.
If I was to implement the logic in code using JavaScript, it would look like the example below:
In this example, we define a function calculateAverage that takes two numbers as input, calculates their sum, computes the average, and returns it. The main program obtains user inputs, calls the calculateAverage function, and displays the result.
Here is another example:
The pseudocode would look like this:
Someone else could write the code as shown below and they will mean the same:
The code snippets show that writing pseudocode is based on your pattern.
Note: Using this keyword like FOR , IF , ELSE IF ELSE , END , etc. are not mandatory because Pseudocode is flexible and allows for the use of alternative keywords or descriptive phrases as long as they effectively convey the intended logic and structure of the algorithm.
For example, instead of using FOR , you could use REPEAT or LOOP . Similarly, instead of END , you could use STOP or FINISH . The key is to maintain consistency within your pseudocode and ensure that the chosen keywords or phrases accurately represent the intended flow of the algorithm. The example below is the same as the pseudocode above but with a different approach.
The code in JavaScript would look like this:
As you can see, the pseudocode resembles a programming language but avoids specific syntax requirements.
Tips for Writing Pseudocode
Familiarize yourself with real code.
To effectively write and understand pseudocode, it is crucial to have a foundation in a real programming language. Learning a programming language such as Python or Java will provide you with the necessary knowledge and concepts to express algorithms in pseudocode.
Simplify and Generalize
When writing pseudocode, aim to simplify the code by excluding language-specific details and unnecessary complexities. Make it as general as possible while retaining clarity and readability. Remember, pseudocode is a high-level representation, so focus on expressing the algorithm's logic rather than the specific syntax.
Be Consistent
Maintain consistency in your pseudocode by using a uniform style and notation throughout. Choose a convention for variable assignment, function calls, and control structures (such as braces or indentation) and stick to it. Inconsistencies can lead to confusion and may result in lower marks in examinations.
Practice Problem Solving
To improve your pseudocode writing skills, engage in problem-solving exercises that require expressing solutions using pseudocode. Work through algorithmic questions and practice implementing the logic in pseudocode. This practice will enhance your ability to translate real-world problems into pseudocode representations.
Learn from Examples and Resources
Study examples of pseudocode from textbooks, online resources, and tutorial videos. Analyze how experts express complex algorithms in pseudocode and learn from their approach. Additionally, seek out resources specific to your academic curriculum or exam board for standardized pseudocode guidelines.
Remember, Pseudocode Is Not Executable Code
Always keep in mind that pseudocode is a simplified representation and is not meant to be executed as actual code. It is a tool for communicating algorithmic ideas, not a programming language itself. Therefore, don't stress over small syntax details or worry about the code's executability.
GitHub Repository
What is Pseudocode? How to Use Pseudocode to Solve Coding Problems
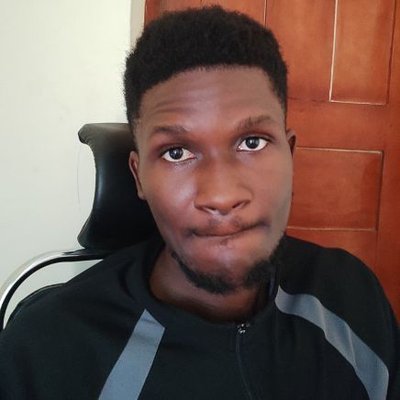
You might be wondering what pseudocode is and why it's so useful for writing computer programs.
But before we jump into pseudocode, let's refresh our memories about what programming and coding are, in the simplest sense.
Programming is the manifestation of logic. A program is a set of instructions that defines the behaviour of your software application. Writing code is how you implement it for the machine.
What is Pseudocode?
Pseudocode literally means ‘fake code’. It is an informal and contrived way of writing programs in which you represent the sequence of actions and instructions (aka algorithms) in a form that humans can easily understand.
You see, computers and human beings are quite different, and therein lies the problem.
The language of a computer is very rigid: you are not allowed to make any mistakes or deviate from the rules. Even with the invention of high-level, human-readable languages like JavaScript and Python, it’s still pretty hard for an average human developer to reason and program in those coding languages.
With pseudocode, however, it’s the exact opposite. You make the rules. It doesn’t matter what language you use to write your pseudocode. All that matters is comprehension.
In pseudocode, you don't have to think about semi-colons, curly braces, the syntax for arrow functions, how to define promises, DOM methods and other core language principles. You just have to be able to explain what you're thinking and doing.
Benefits of Writing Pseudocode
When you're writing code in a programming language, you’ll have to battle with strict syntax and rigid coding patterns. But you write pseudocode in a language or form with which you're very familiar.
Since pseudocode is an informal method of program design, you don’t have to obey any set-out rules. You make the rules yourself.
Pseudocode acts as the bridge between your brain and computer’s code executor. It allows you to plan instructions which follow a logical pattern, without including all of the technical details.
Pseudocode is a great way of getting started with software programming as a beginner. You won’t have to overwhelm your brain with coding syntax.
In fact, many companies organize programming tests for their interviewees in pseudocode. This is because the importance of problem solving supersedes the ability to ‘hack’ computer code.
You can get quality code from many platforms online, but you have to learn problem solving and practice it a lot.
Planning computer algorithms with pseudocode makes you meticulous. It helps you explain exactly what each line in a software program should do. This is possible because you are in full control of everything, which is one of the great features of pseudocode.
Example of Pseudocode
Pseudocode is a very intuitive way to develop software programs. To illustrate this, I am going to refer back to a very simple program I wrote in my last article :
When a user fills in a form and clicks the submit button, execute a ValidateEmail function. What should the function do?
Derive an email regular expression (regex) to test the user's email address against.
Access the user's email from the DOM and store it in a variable. Find and use the right DOM method for that task.
With the email value now accessed and stored, create a conditional statement:
If the email format doesn’t match the rule specified by the regex, access the element with the myAlert id attribute and pass in the “Invalid Email” message for the user to see.
Else, if the above condition isn’t true and the email address format actually matches with the regex, check to see if the database already has such an email address. If it already does, access the element with the myAlert id attribute and pass in the “Email exists!” message for the user to see.
Now, if both of these conditions aren’t met, (that is the email format matches the regex and the database doesn’t have such an email address stored yet), push the users email address into the database and pass in the “Successful!” message for the user to see.
Once you are done outlining the various steps you want your code to take, everything becomes easier and clearer. Now, let’s turn that psedocode into real JavaScript code:
All you have to do at this stage is find the programming language constructs that will help you achieve each of your steps. Noticed how seamless the transition from pseudocode to actual code became? That’s how effective writing pseudocode can be for program design.
Pseudocode is also a great way to solve programming-related problems when you're struggling with them. For those practising programming in coding challenge platforms like CodeWars , pseudocode can be of immense help.
How to Solve Programming Problems with Pseudocode
Solving programming problems can be hard. Not only do you have the logical part to reckon with, but also the technical (code forming) part as well. I recently uncovered a brilliant and effective formula for solving tricky coding problems.
Here are the steps you can follow to solving programming problems with pseudocode:
Step 1: Understand what the function does
First, you need to understand that all a function does is (optionally) accept data as input, work on the data little by little, and finally return an output. The body of the function is what actually solves the problem and it does so line by line.
Step 2: Make sure you understand the question
Next, you need to read and understand the question properly. This is arguably the most important step in the process.
If you fail to properly understand the question, you won’t be able to work through the problem and figure out the possible steps to take. Once you identify the main problem to be solved you'll be ready to tackle it.
Step 3: Break the problem down.
Now you need to break down the problem into smaller parts and sub-problems. With each smaller problem you solve, you'll get closer to solving the main problem.
It helps to represent these problem solving steps in the clearest and most easily understandable way you can – which is psedocode!
Start solving: open and use tools like Google, Stack Overflow, MDN, and of course freeCodeCamp! :)
For every step of the problem that you solve, test the output to make sure you’re on the right path. Keep solving these small problems until you arrive at the final solution.
I picked up this highly effective formula from Aaron Jack and I think you’ll benefit from it. Check out his video about how to solve coding problems:
As you can see, pseudocode is a very useful strategy for planning computer programs.
Of course, you have to remember that pseudocode is not a true representation of a computer program. While using pseudocode to plan your algorithm is great, you will ultimately have to translate it into an actual computer-readable program. This means that you'll eventually need to learn how to program in a real programming language.
Taking up coding challenges online is a great way to learn how to program because, as they say, practice makes perfect. But when you try your next challenge, don’t forget to implement pseudocode in the process!
You can check out some of my other programming-related posts on my personal blog . I am also available on Twitter .
Thank you for reading and see you soon.
P/S: If you are learning JavaScript, I created an eBook which teaches 50 topics in JavaScript with hand-drawn digital notes. Check it out here .
Technical writer | Backend web developer | Experienced in developing and deploying web and mobile applications. Writes for @Logrocket, @tutsplus and more
If this article was helpful, share it .
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
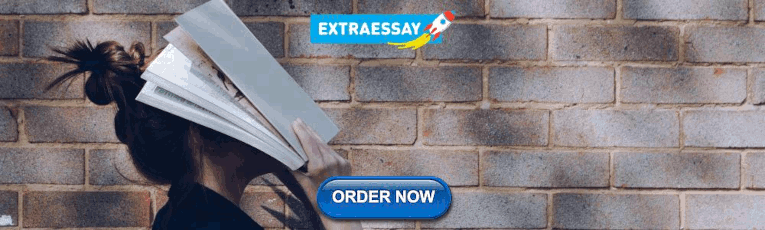
IMAGES
VIDEO
COMMENTS
Pseudocode is the intermediate state between an idea and its implementation (code) in a high-level language. Pseudocode is an important part of designing an algorithm, it helps the programmer in planning the solution to the problem as well as the reader in understanding the approach to the problem.
Pseudocode is a way to describe how to accomplish tasks using basic steps like those a computer might perform. The advantage of pseudocode over plain English is that it has a precise meaning that allows us to express a computation clearly and precisely.
Assignment. In pseudocode, when assigning values to a variable or constant, it is best practice to use the assignment arrow (←).
How to write a Pseudo-code? Arrange the sequence of tasks and write the pseudocode accordingly. Start with the statement of a pseudo code which establishes the main goal or the aim. Example: the number whether it's even or odd.
Assigning a value to a variable is indicated in pseudocode using an arrow symbol (←). The arrow points from the value being assigned towards the variable it is being assigned to. The following line of pseudocode should be read as 'a becomes equal to 34'.
Pseudocode consists of essential elements common across various algorithms: Variables: represented using simple names to denote data storage; Operations: basic operations such as assignment, arithmetic, and conditional statements; Loops: described using intuitive language like “while” or “for”;
Want to learn how to write pseudocode? Pseudocode is a step-by-step written outline of your code that you can transcribe into the programming language you’re working with. In other words, you’re writing the flow of your code in plain language rather than official coding syntax.
Pseudocode is a simulated version of code that resembles a programming language but does not adhere to its strict syntax rules. It aims to focus on the logic and functionality of an algorithm, making it independent of any specific programming language.
In assignments and exams for the course, you need to demonstrate your knowledge without obscuring the big picture with unneeded detail. Here are a few general guidelines for checking your pseudocode: 1. Mimic good code and good English. Using aspects of both systems means adhering to the style rules of both to some degree.
Pseudocode acts as the bridge between your brain and computer’s code executor. It allows you to plan instructions which follow a logical pattern, without including all of the technical details. Pseudocode is a great way of getting started with software programming as a beginner.