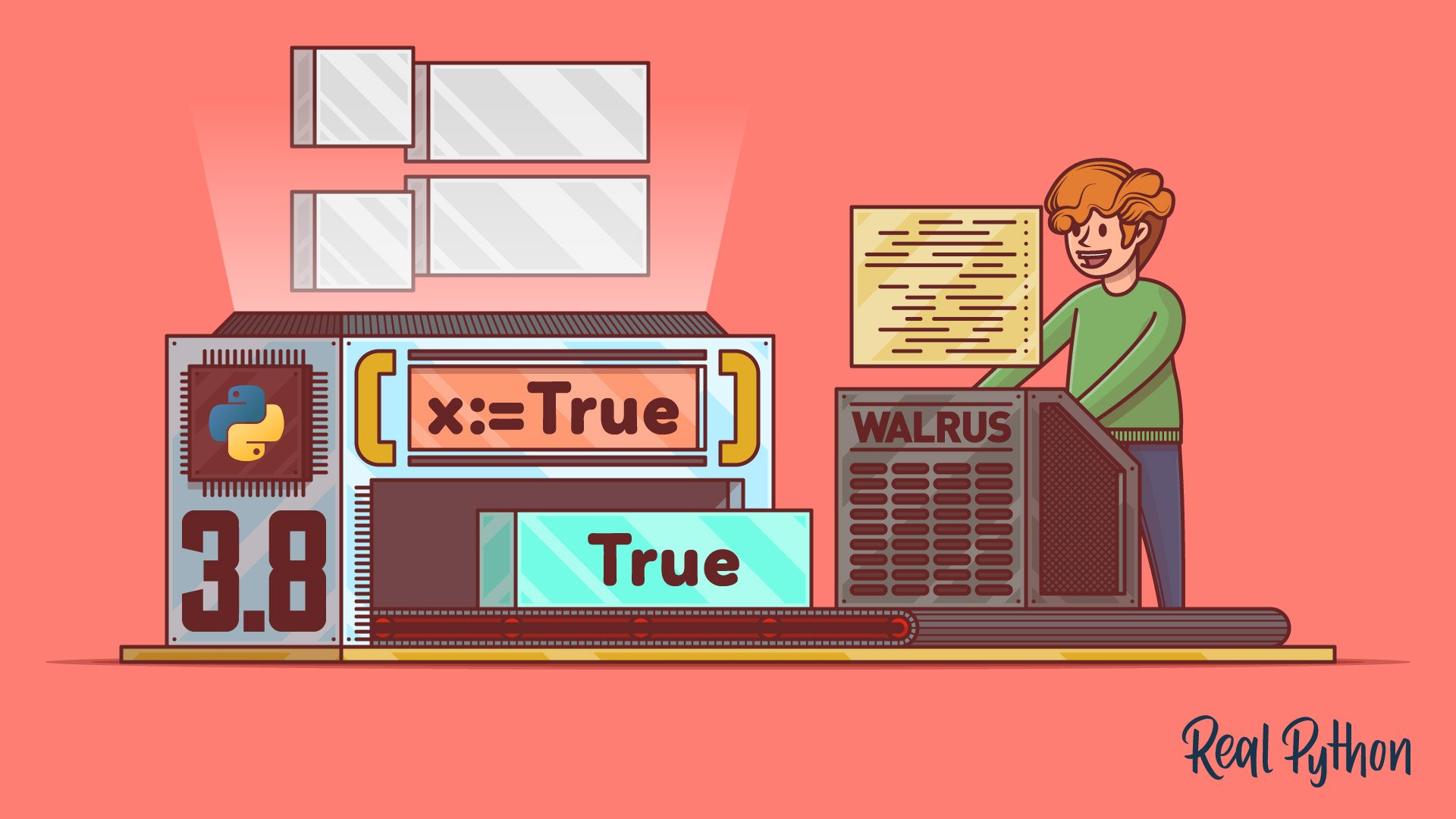
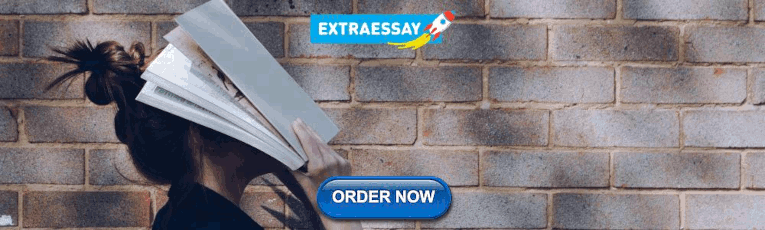
The Walrus Operator: Python 3.8 Assignment Expressions
Table of Contents
Hello, Walrus!
Implementation, lists and dictionaries, list comprehensions, while loops, witnesses and counterexamples, walrus operator syntax, walrus operator pitfalls.
Watch Now This tutorial has a related video course created by the Real Python team. Watch it together with the written tutorial to deepen your understanding: Python Assignment Expressions and Using the Walrus Operator
Each new version of Python adds new features to the language. For Python 3.8, the biggest change is the addition of assignment expressions . Specifically, the := operator gives you a new syntax for assigning variables in the middle of expressions. This operator is colloquially known as the walrus operator .
This tutorial is an in-depth introduction to the walrus operator. You will learn some of the motivations for the syntax update and explore some examples where assignment expressions can be useful.
In this tutorial, you’ll learn how to:
- Identify the walrus operator and understand its meaning
- Understand use cases for the walrus operator
- Avoid repetitive code by using the walrus operator
- Convert between code using the walrus operator and code using other assignment methods
- Understand the impacts on backward compatibility when using the walrus operator
- Use appropriate style in your assignment expressions
Note that all walrus operator examples in this tutorial require Python 3.8 or later to work.
Free Download: Get a sample chapter from Python Tricks: The Book that shows you Python’s best practices with simple examples you can apply instantly to write more beautiful + Pythonic code.
Walrus Operator Fundamentals
Let’s start with some different terms that programmers use to refer to this new syntax. You’ve already seen a few in this tutorial.
The := operator is officially known as the assignment expression operator . During early discussions, it was dubbed the walrus operator because the := syntax resembles the eyes and tusks of a sideways walrus . You may also see the := operator referred to as the colon equals operator . Yet another term used for assignment expressions is named expressions .
To get a first impression of what assignment expressions are all about, start your REPL and play around with the following code:
Line 1 shows a traditional assignment statement where the value False is assigned to walrus . Next, on line 5, you use an assignment expression to assign the value True to walrus . After both lines 1 and 5, you can refer to the assigned values by using the variable name walrus .
You might be wondering why you’re using parentheses on line 5, and you’ll learn why the parentheses are needed later on in this tutorial .
Note: A statement in Python is a unit of code. An expression is a special statement that can be evaluated to some value.
For example, 1 + 2 is an expression that evaluates to the value 3 , while number = 1 + 2 is an assignment statement that doesn’t evaluate to a value. Although running the statement number = 1 + 2 doesn’t evaluate to 3 , it does assign the value 3 to number .
In Python, you often see simple statements like return statements and import statements , as well as compound statements like if statements and function definitions . These are all statements, not expressions.
There’s a subtle—but important—difference between the two types of assignments seen earlier with the walrus variable. An assignment expression returns the value, while a traditional assignment doesn’t. You can see this in action when the REPL doesn’t print any value after walrus = False on line 1, while it prints out True after the assignment expression on line 5.
You can see another important aspect about walrus operators in this example. Though it might look new, the := operator does not do anything that isn’t possible without it. It only makes certain constructs more convenient and can sometimes communicate the intent of your code more clearly.
Note: You need at least Python 3.8 to try out the examples in this tutorial. If you don’t already have Python 3.8 installed and you have Docker available, a quick way to start working with Python 3.8 is to run one of the official Docker images :
This will download and run the latest stable version of Python 3.8. For more information, see Run Python Versions in Docker: How to Try the Latest Python Release .
Now you have a basic idea of what the := operator is and what it can do. It’s an operator used in assignment expressions, which can return the value being assigned, unlike traditional assignment statements. To get deeper and really learn about the walrus operator, continue reading to see where you should and shouldn’t use it.
Like most new features in Python, assignment expressions were introduced through a Python Enhancement Proposal (PEP). PEP 572 describes the motivation for introducing the walrus operator, the details of the syntax, as well as examples where the := operator can be used to improve your code.
This PEP was originally written by Chris Angelico in February 2018. Following some heated discussion, PEP 572 was accepted by Guido van Rossum in July 2018. Since then, Guido announced that he was stepping down from his role as benevolent dictator for life (BDFL) . Starting in early 2019, Python has been governed by an elected steering council instead.
The walrus operator was implemented by Emily Morehouse , and made available in the first alpha release of Python 3.8.
In many languages, including C and its derivatives, assignment statements function as expressions. This can be both very powerful and also a source of confusing bugs. For example, the following code is valid C but doesn’t execute as intended:
Here, if (x = y) will evaluate to true and the code snippet will print out x and y are equal (x = 8, y = 8) . Is this the result you were expecting? You were trying to compare x and y . How did the value of x change from 3 to 8 ?
The problem is that you’re using the assignment operator ( = ) instead of the equality comparison operator ( == ). In C, x = y is an expression that evaluates to the value of y . In this example, x = y is evaluated as 8 , which is considered truthy in the context of the if statement.
Take a look at a corresponding example in Python. This code raises a SyntaxError :
Unlike the C example, this Python code gives you an explicit error instead of a bug.
The distinction between assignment statements and assignment expressions in Python is useful in order to avoid these kinds of hard-to-find bugs. PEP 572 argues that Python is better suited to having different syntax for assignment statements and expressions instead of turning the existing assignment statements into expressions.
One design principle underpinning the walrus operator is that there are no identical code contexts where both an assignment statement using the = operator and an assignment expression using the := operator would be valid. For example, you can’t do a plain assignment with the walrus operator:
In many cases, you can add parentheses ( () ) around the assignment expression to make it valid Python:
Writing a traditional assignment statement with = is not allowed inside such parentheses. This helps you catch potential bugs.
Later on in this tutorial , you’ll learn more about situations where the walrus operator is not allowed, but first you’ll learn about the situations where you might want to use them.
Walrus Operator Use Cases
In this section, you’ll see several examples where the walrus operator can simplify your code. A general theme in all these examples is that you’ll avoid different kinds of repetition:
- Repeated function calls can make your code slower than necessary.
- Repeated statements can make your code hard to maintain.
- Repeated calls that exhaust iterators can make your code overly complex.
You’ll see how the walrus operator can help in each of these situations.
Arguably one of the best use cases for the walrus operator is when debugging complex expressions. Say that you want to find the distance between two locations along the earth’s surface. One way to do this is to use the haversine formula :

ϕ represents the latitude and λ represents the longitude of each location. To demonstrate this formula, you can calculate the distance between Oslo (59.9°N 10.8°E) and Vancouver (49.3°N 123.1°W) as follows:
As you can see, the distance from Oslo to Vancouver is just under 7200 kilometers.
Note: Python source code is typically written using UTF-8 Unicode . This allows you to use Greek letters like ϕ and λ in your code, which may be useful when translating mathematical formulas. Wikipedia shows some alternatives for using Unicode on your system.
While UTF-8 is supported (in string literals, for instance), Python’s variable names use a more limited character set . For example, you can’t use emojis while naming your variables. That is a good restriction !
Now, say that you need to double-check your implementation and want to see how much the haversine terms contribute to the final result. You could copy and paste the term from your main code to evaluate it separately. However, you could also use the := operator to give a name to the subexpression you’re interested in:
The advantage of using the walrus operator here is that you calculate the value of the full expression and keep track of the value of ϕ_hav at the same time. This allows you to confirm that you did not introduce any errors while debugging.
Lists are powerful data structures in Python that often represent a series of related attributes. Similarly, dictionaries are used all over Python and are great for structuring information.
Sometimes when setting up these data structures, you end up performing the same operation several times. As a first example, calculate some basic descriptive statistics of a list of numbers and store them in a dictionary:
Note that both the sum and the length of the numbers list are calculated twice. The consequences are not too bad in this simple example, but if the list was larger or the calculations were more complicated, you might want to optimize the code. To do this, you can first move the function calls out of the dictionary definition:
The variables num_length and num_sum are only used to optimize the calculations inside the dictionary. By using the walrus operator, this role can be made more clear:
num_length and num_sum are now defined inside the definition of description . This is a clear hint to anybody reading this code that these variables are just used to optimize these calculations and aren’t used again later.
Note: The scope of the num_length and num_sum variables is the same in the example with the walrus operator and in the example without. This means that in both examples, the variables are available after the definition of description .
Even though both examples are very similar functionally, a benefit of using the assignment expressions is that the := operator communicates the intent of these variables as throwaway optimizations.
In the next example, you’ll work with a bare-bones implementation of the wc utility for counting lines, words, and characters in a text file:
This script can read one or several text files and report how many lines, words, and characters each of them contains. Here’s a breakdown of what’s happening in the code:
- Line 6 loops over each filename provided by the user. sys.argv is a list containing each argument given on the command line, starting with the name of your script. For more information about sys.argv , you can check out Python Command Line Arguments .
- Line 7 translates each filename string to a pathlib.Path object . Storing a filename in a Path object allows you to conveniently read the text file in the next lines.
- Lines 8 to 12 construct a tuple of counts to represent the number of lines, words, and characters in one text file.
- Line 9 reads a text file and calculates the number of lines by counting newlines.
- Line 10 reads a text file and calculates the number of words by splitting on whitespace.
- Line 11 reads a text file and calculates the number of characters by finding the length of the string.
- Line 13 prints all three counts together with the filename to the console. The *counts syntax unpacks the counts tuple. In this case, the print() statement is equivalent to print(counts[0], counts[1], counts[2], path) .
To see wc.py in action, you can use the script on itself as follows:
In other words, the wc.py file consists of 13 lines, 34 words, and 316 characters.
If you look closely at this implementation, you’ll notice that it’s far from optimal. In particular, the call to path.read_text() is repeated three times. That means that each text file is read three times. You can use the walrus operator to avoid the repetition:
The contents of the file are assigned to text , which is reused in the next two calculations. The program still functions the same:
As in the earlier examples, an alternative approach is to define text before the definition of counts :
While this is one line longer than the previous implementation, it probably provides the best balance between readability and efficiency. The := assignment expression operator isn’t always the most readable solution even when it makes your code more concise.
List comprehensions are great for constructing and filtering lists. They clearly state the intent of the code and will usually run quite fast.
There’s one list comprehension use case where the walrus operator can be particularly useful. Say that you want to apply some computationally expensive function, slow() , to the elements in your list and filter on the resulting values. You could do something like the following:
Here, you filter the numbers list and leave the positive results from applying slow() . The problem with this code is that this expensive function is called twice.
A very common solution for this type of situation is rewriting your code to use an explicit for loop:
This will only call slow() once. Unfortunately, the code is now more verbose, and the intent of the code is harder to understand. The list comprehension had clearly signaled that you were creating a new list, while this is more hidden in the explicit for loop since several lines of code separate the list creation and the use of .append() . Additionally, the list comprehension runs faster than the repeated calls to .append() .
You can code some other solutions by using a filter() expression or a kind of double list comprehension:
The good news is that there’s only one call to slow() for each number. The bad news is that the code’s readability has suffered in both expressions.
Figuring out what’s actually happening in the double list comprehension takes a fair amount of head-scratching. Essentially, the second for statement is used only to give the name value to the return value of slow(num) . Fortunately, that sounds like something that can instead be performed with an assignment expression!
You can rewrite the list comprehension using the walrus operator as follows:
Note that the parentheses around value := slow(num) are required. This version is effective, readable, and communicates the intent of the code well.
Note: You need to add the assignment expression on the if clause of the list comprehension. If you try to define value with the other call to slow() , then it will not work:
This will raise a NameError because the if clause is evaluated before the expression at the beginning of the comprehension.
Let’s look at a slightly more involved and practical example. Say that you want to use the Real Python feed to find the titles of the last episodes of the Real Python Podcast .
You can use the Real Python Feed Reader to download information about the latest Real Python publications. In order to find the podcast episode titles, you’ll use the third-party Parse package. Start by installing both into your virtual environment :
You can now read the latest titles published by Real Python :
Podcast titles start with "The Real Python Podcast" , so here you can create a pattern that Parse can use to identify them:
Compiling the pattern beforehand speeds up later comparisons, especially when you want to match the same pattern over and over. You can check if a string matches your pattern using either pattern.parse() or pattern.search() :
Note that Parse is able to pick out the podcast episode number and the episode name. The episode number is converted to an integer data type because you used the :d format specifier .
Let’s get back to the task at hand. In order to list all the recent podcast titles, you need to check whether each string matches your pattern and then parse out the episode title. A first attempt may look something like this:
Though it works, you might notice the same problem you saw earlier. You’re parsing each title twice because you filter out titles that match your pattern and then use that same pattern to pick out the episode title.
Like you did earlier, you can avoid the double work by rewriting the list comprehension using either an explicit for loop or a double list comprehension. Using the walrus operator, however, is even more straightforward:
Assignment expressions work well to simplify these kinds of list comprehensions. They help you keep your code readable while you avoid doing a potentially expensive operation twice.
Note: The Real Python Podcast has its own separate RSS feed , which you should use if you want to play around with information only about the podcast. You can get all the episode titles with the following code:
See The Real Python Podcast for options to listen to it using your podcast player.
In this section, you’ve focused on examples where list comprehensions can be rewritten using the walrus operator. The same principles also apply if you see that you need to repeat an operation in a dictionary comprehension , a set comprehension , or a generator expression .
The following example uses a generator expression to calculate the average length of episode titles that are over 50 characters long:
The generator expression uses an assignment expression to avoid calculating the length of each episode title twice.
Python has two different loop constructs: for loops and while loops . You typically use a for loop when you need to iterate over a known sequence of elements. A while loop, on the other hand, is used when you don’t know beforehand how many times you’ll need to loop.
In while loops, you need to define and check the ending condition at the top of the loop. This sometimes leads to some awkward code when you need to do some setup before performing the check. Here’s a snippet from a multiple-choice quiz program that asks the user to answer a question with one of several valid answers:
This works but has an unfortunate repetition of identical input() lines. It’s necessary to get at least one answer from the user before checking whether it’s valid or not. You then have a second call to input() inside the while loop to ask for a second answer in case the original user_answer wasn’t valid.
If you want to make your code more maintainable, it’s quite common to rewrite this kind of logic with a while True loop. Instead of making the check part of the main while statement, the check is performed later in the loop together with an explicit break :
This has the advantage of avoiding the repetition. However, the actual check is now harder to spot.
Assignment expressions can often be used to simplify these kinds of loops. In this example, you can now put the check back together with while where it makes more sense:
The while statement is a bit denser, but the code now communicates the intent more clearly without repeated lines or seemingly infinite loops.
You can expand the box below to see the full code of the multiple-choice quiz program and try a couple of questions about the walrus operator yourself.
Full source code of multiple-choice quiz program Show/Hide
This script runs a multiple-choice quiz. You’ll be asked each of the questions in order, but the order of answers will be shuffled each time:
Note that the first answer is assumed to be the correct one. You can add more questions to the quiz yourself. Feel free to share your questions with the community in the comments section below the tutorial!
You can often simplify while loops by using assignment expressions. The original PEP shows an example from the standard library that makes the same point.
In the examples you’ve seen so far, the := assignment expression operator does essentially the same job as the = assignment operator in your old code. You’ve seen how to simplify code, and now you’ll learn about a different type of use case that’s made possible by this new operator.
In this section, you’ll learn how you can find witnesses when calling any() by using a clever trick that isn’t possible without using the walrus operator. A witness, in this context, is an element that satisfies the check and causes any() to return True .
By applying similar logic, you’ll also learn how you can find counterexamples when working with all() . A counterexample, in this context, is an element that doesn’t satisfy the check and causes all() to return False .
In order to have some data to work with, define the following list of city names:
You can use any() and all() to answer questions about your data:
In each of these cases, any() and all() give you plain True or False answers. What if you’re also interested in seeing an example or a counterexample of the city names? It could be nice to see what’s causing your True or False result:
Does any city name start with "H" ?
Yes, because "Houston" starts with "H" .
Do all city names start with "H" ?
No, because "Oslo" doesn’t start with "H" .
In other words, you want a witness or a counterexample to justify the answer.
Capturing a witness to an any() expression has not been intuitive in earlier versions of Python. If you were calling any() on a list and then realized you also wanted a witness, you’d typically need to rewrite your code:
Here, you first capture all city names that start with "H" . Then, if there’s at least one such city name, you print out the first city name starting with "H" . Note that here you’re actually not using any() even though you’re doing a similar operation with the list comprehension.
By using the := operator, you can find witnesses directly in your any() expressions:
You can capture a witness inside the any() expression. The reason this works is a bit subtle and relies on any() and all() using short-circuit evaluation : they only check as many items as necessary to determine the result.
Note: If you want to check whether all city names start with the letter "H" , then you can look for a counterexample by replacing any() with all() and updating the print() functions to report the first item that doesn’t pass the check.
You can see what’s happening more clearly by wrapping .startswith("H") in a function that also prints out which item is being checked:
Note that any() doesn’t actually check all items in cities . It only checks items until it finds one that satisfies the condition. Combining the := operator and any() works by iteratively assigning each item that is being checked to witness . However, only the last such item survives and shows which item was last checked by any() .
Even when any() returns False , a witness is found:
However, in this case, witness doesn’t give any insight. 'Holguín' doesn’t contain ten or more characters. The witness only shows which item happened to be evaluated last.
One of the main reasons assignments were not expressions in Python from the beginning is that the visual likeness of the assignment operator ( = ) and the equality comparison operator ( == ) could potentially lead to bugs. When introducing assignment expressions, a lot of thought was put into how to avoid similar bugs with the walrus operator. As mentioned earlier , one important feature is that the := operator is never allowed as a direct replacement for the = operator, and vice versa.
As you saw at the beginning of this tutorial, you can’t use a plain assignment expression to assign a value:
It’s syntactically legal to use an assignment expression to only assign a value, but only if you add parentheses:
Even though it’s possible, however, this really is a prime example of where you should stay away from the walrus operator and use a traditional assignment statement instead.
PEP 572 shows several other examples where the := operator is either illegal or discouraged. The following examples all raise a SyntaxError :
In all these cases, you’re better served using = instead. The next examples are similar and are all legal code. However, the walrus operator doesn’t improve your code in any of these cases:
None of these examples make your code more readable. You should instead do the extra assignment separately by using a traditional assignment statement. See PEP 572 for more details about the reasoning.
There’s one use case where the := character sequence is already valid Python. In f-strings , a colon ( : ) is used to separate values from their format specification . For example:
The := in this case does look like a walrus operator, but the effect is quite different. To interpret x:=8 inside the f-string, the expression is broken into three parts: x , : , and =8 .
Here, x is the value, : acts as a separator, and =8 is a format specification. According to Python’s Format Specification Mini-Language , in this context = specifies an alignment option. In this case, the value is padded with spaces in a field of width 8 .
To use assignment expressions inside f-strings, you need to add parentheses:
This updates the value of x as expected. However, you’re probably better off using traditional assignments outside of your f-strings instead.
Let’s look at some other situations where assignment expressions are illegal:
Attribute and item assignment: You can only assign to simple names, not dotted or indexed names:
This fails with a descriptive error message. There’s no straightforward workaround.
Iterable unpacking: You can’t unpack when using the walrus operator:
If you add parentheses around the whole expression, it will be interpreted as a 3-tuple with the three elements lat , 59.9 , and 10.8 .
Augmented assignment: You can’t use the walrus operator combined with augmented assignment operators like += . This raises a SyntaxError :
The easiest workaround would be to do the augmentation explicitly. You could, for example, do (count := count + 1) . PEP 577 originally described how to add augmented assignment expressions to Python, but the proposal was withdrawn.
When you’re using the walrus operator, it will behave similarly to traditional assignment statements in many respects:
The scope of the assignment target is the same as for assignments. It will follow the LEGB rule . Typically, the assignment will happen in the local scope, but if the target name is already declared global or nonlocal , that is honored.
The precedence of the walrus operator can cause some confusion. It binds less tightly than all other operators except the comma, so you might need parentheses to delimit the expression that is assigned. As an example, note what happens when you don’t use parentheses:
square is bound to the whole expression number ** 2 > 5 . In other words, square gets the value True and not the value of number ** 2 , which was the intention. In this case, you can delimit the expression with parentheses:
The parentheses make the if statement both clearer and actually correct.
There’s one final gotcha. When assigning a tuple using the walrus operator, you always need to use parentheses around the tuple. Compare the following assignments:
Note that in the second example, walrus takes the value 3.8 and not the whole tuple 3.8, True . That’s because the := operator binds more tightly than the comma. This may seem a bit annoying. However, if the := operator bound less tightly than the comma, it would not be possible to use the walrus operator in function calls with more than one argument.
The style recommendations for the walrus operator are mostly the same as for the = operator used for assignment. First, always add spaces around the := operator in your code. Second, use parentheses around the expression as necessary, but avoid adding extra parentheses that are not needed.
The general design of assignment expressions is to make them easy to use when they are helpful but to avoid overusing them when they might clutter up your code.
The walrus operator is a new syntax that is only available in Python 3.8 and later. This means that any code you write that uses the := syntax will only work on the most recent versions of Python.
If you need to support older versions of Python, you can’t ship code that uses assignment expressions. There are some projects, like walrus , that can automatically translate walrus operators into code that is compatible with older versions of Python. This allows you to take advantage of assignment expressions when writing your code and still distribute code that is compatible with more Python versions.
Experience with the walrus operator indicates that := will not revolutionize Python. Instead, using assignment expressions where they are useful can help you make several small improvements to your code that could benefit your work overall.
There are many times it’s possible for you to use the walrus operator, but where it won’t necessarily improve the readability or efficiency of your code. In those cases, you’re better off writing your code in a more traditional manner.
You now know how the new walrus operator works and how you can use it in your own code. By using the := syntax, you can avoid different kinds of repetition in your code and make your code both more efficient and easier to read and maintain. At the same time, you shouldn’t use assignment expressions everywhere. They will only help you in some use cases.
In this tutorial, you learned how to:
To learn more about the details of assignment expressions, see PEP 572 . You can also check out the PyCon 2019 talk PEP 572: The Walrus Operator , where Dustin Ingram gives an overview of both the walrus operator and the discussion around the new PEP.
🐍 Python Tricks 💌
Get a short & sweet Python Trick delivered to your inbox every couple of days. No spam ever. Unsubscribe any time. Curated by the Real Python team.
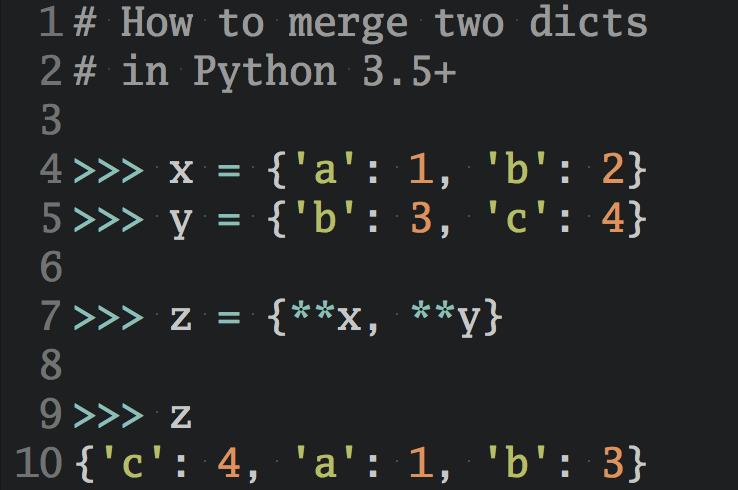
About Geir Arne Hjelle
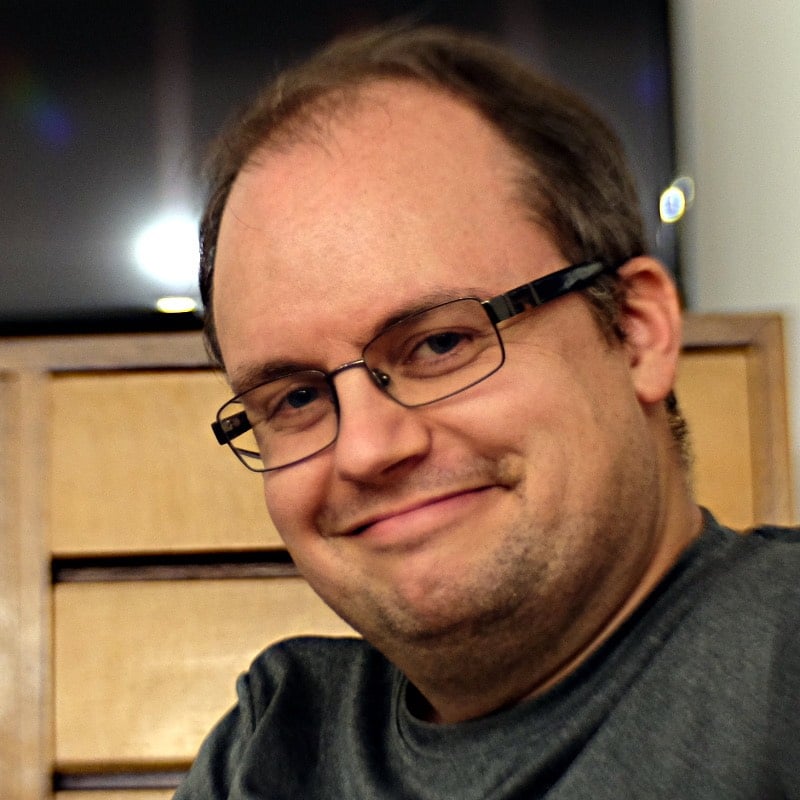
Geir Arne is an avid Pythonista and a member of the Real Python tutorial team.
Each tutorial at Real Python is created by a team of developers so that it meets our high quality standards. The team members who worked on this tutorial are:
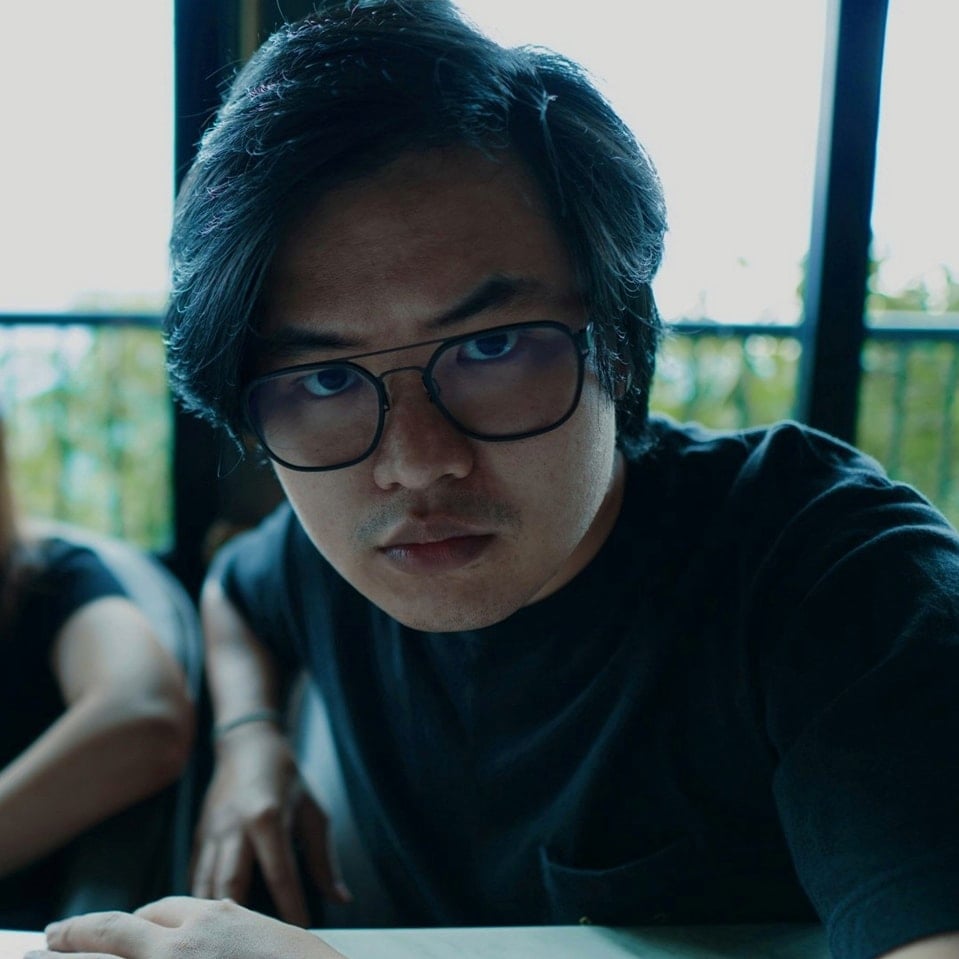
Master Real-World Python Skills With Unlimited Access to Real Python
Join us and get access to thousands of tutorials, hands-on video courses, and a community of expert Pythonistas:
Join us and get access to thousands of tutorials, hands-on video courses, and a community of expert Pythonistas:
What Do You Think?
What’s your #1 takeaway or favorite thing you learned? How are you going to put your newfound skills to use? Leave a comment below and let us know.
Commenting Tips: The most useful comments are those written with the goal of learning from or helping out other students. Get tips for asking good questions and get answers to common questions in our support portal . Looking for a real-time conversation? Visit the Real Python Community Chat or join the next “Office Hours” Live Q&A Session . Happy Pythoning!
Keep Learning
Related Topics: intermediate best-practices
Recommended Video Course: Python Assignment Expressions and Using the Walrus Operator
Keep reading Real Python by creating a free account or signing in:
Already have an account? Sign-In
Almost there! Complete this form and click the button below to gain instant access:
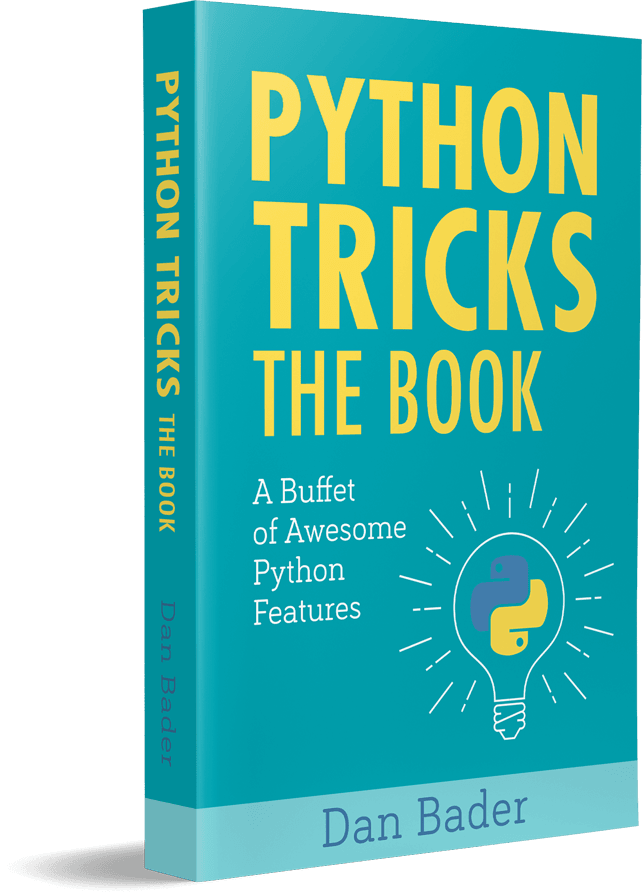
"Python Tricks: The Book" – Free Sample Chapter (PDF)
🔒 No spam. We take your privacy seriously.
Python Tutorial
File handling, python modules, python numpy, python pandas, python matplotlib, python scipy, machine learning, python mysql, python mongodb, python reference, module reference, python how to, python examples, python assignment operators.
Assignment operators are used to assign values to variables:
Operator | Example | Same As | Try it |
---|---|---|---|
= | x = 5 | x = 5 | |
+= | x += 3 | x = x + 3 | |
-= | x -= 3 | x = x - 3 | |
*= | x *= 3 | x = x * 3 | |
/= | x /= 3 | x = x / 3 | |
%= | x %= 3 | x = x % 3 | |
//= | x //= 3 | x = x // 3 | |
**= | x **= 3 | x = x ** 3 | |
&= | x &= 3 | x = x & 3 | |
|= | x |= 3 | x = x | 3 | |
^= | x ^= 3 | x = x ^ 3 | |
>>= | x >>= 3 | x = x >> 3 | |
<<= | x <<= 3 | x = x << 3 |
Related Pages

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
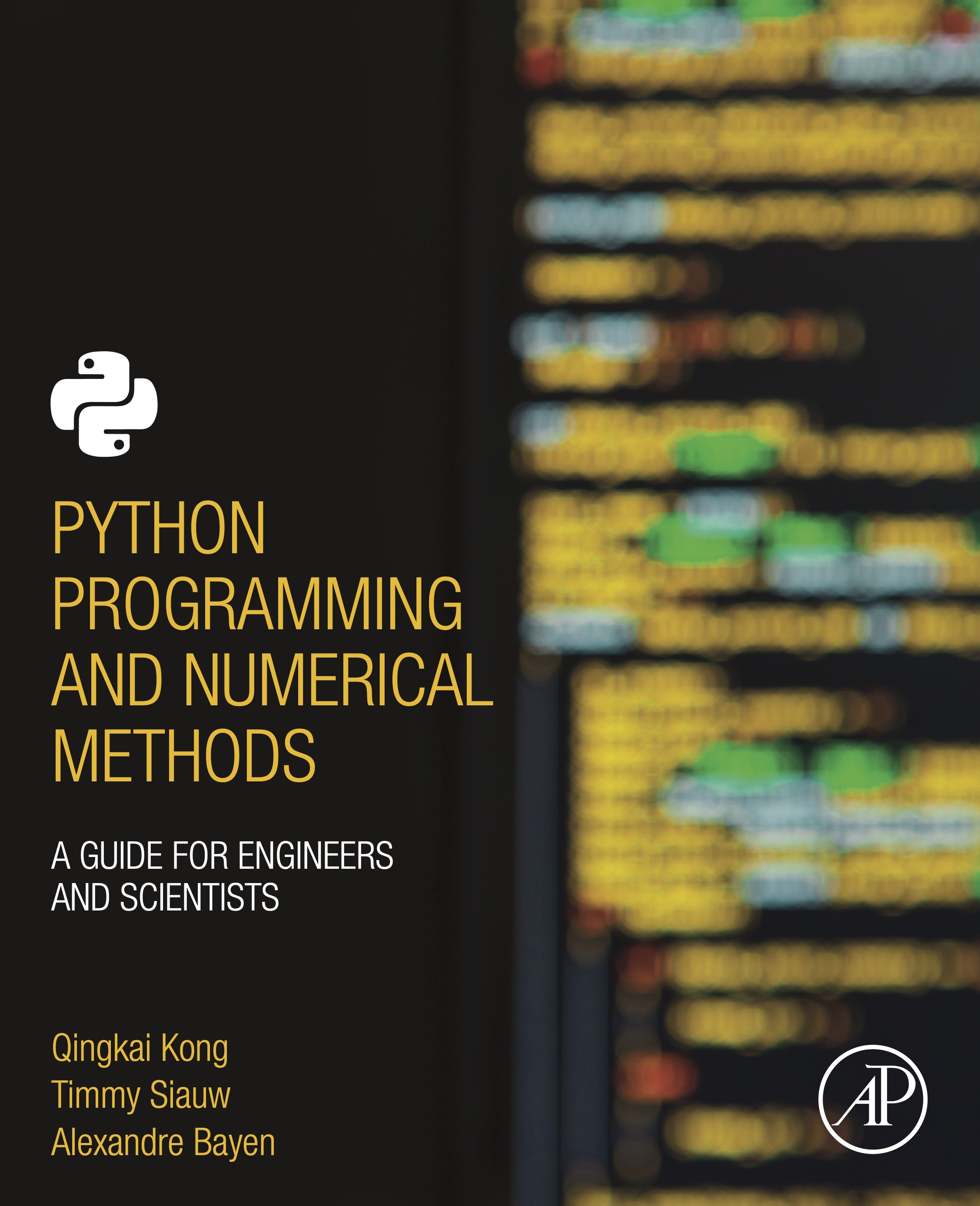
Python Numerical Methods

This notebook contains an excerpt from the Python Programming and Numerical Methods - A Guide for Engineers and Scientists , the content is also available at Berkeley Python Numerical Methods .
The copyright of the book belongs to Elsevier. We also have this interactive book online for a better learning experience. The code is released under the MIT license . If you find this content useful, please consider supporting the work on Elsevier or Amazon !
< 2.0 Variables and Basic Data Structures | Contents | 2.2 Data Structure - Strings >
Variables and Assignment ¶
When programming, it is useful to be able to store information in variables. A variable is a string of characters and numbers associated with a piece of information. The assignment operator , denoted by the “=” symbol, is the operator that is used to assign values to variables in Python. The line x=1 takes the known value, 1, and assigns that value to the variable with name “x”. After executing this line, this number will be stored into this variable. Until the value is changed or the variable deleted, the character x behaves like the value 1.
TRY IT! Assign the value 2 to the variable y. Multiply y by 3 to show that it behaves like the value 2.
A variable is more like a container to store the data in the computer’s memory, the name of the variable tells the computer where to find this value in the memory. For now, it is sufficient to know that the notebook has its own memory space to store all the variables in the notebook. As a result of the previous example, you will see the variable “x” and “y” in the memory. You can view a list of all the variables in the notebook using the magic command %whos .
TRY IT! List all the variables in this notebook
Note that the equal sign in programming is not the same as a truth statement in mathematics. In math, the statement x = 2 declares the universal truth within the given framework, x is 2 . In programming, the statement x=2 means a known value is being associated with a variable name, store 2 in x. Although it is perfectly valid to say 1 = x in mathematics, assignments in Python always go left : meaning the value to the right of the equal sign is assigned to the variable on the left of the equal sign. Therefore, 1=x will generate an error in Python. The assignment operator is always last in the order of operations relative to mathematical, logical, and comparison operators.
TRY IT! The mathematical statement x=x+1 has no solution for any value of x . In programming, if we initialize the value of x to be 1, then the statement makes perfect sense. It means, “Add x and 1, which is 2, then assign that value to the variable x”. Note that this operation overwrites the previous value stored in x .
There are some restrictions on the names variables can take. Variables can only contain alphanumeric characters (letters and numbers) as well as underscores. However, the first character of a variable name must be a letter or underscores. Spaces within a variable name are not permitted, and the variable names are case-sensitive (e.g., x and X will be considered different variables).
TIP! Unlike in pure mathematics, variables in programming almost always represent something tangible. It may be the distance between two points in space or the number of rabbits in a population. Therefore, as your code becomes increasingly complicated, it is very important that your variables carry a name that can easily be associated with what they represent. For example, the distance between two points in space is better represented by the variable dist than x , and the number of rabbits in a population is better represented by nRabbits than y .
Note that when a variable is assigned, it has no memory of how it was assigned. That is, if the value of a variable, y , is constructed from other variables, like x , reassigning the value of x will not change the value of y .
EXAMPLE: What value will y have after the following lines of code are executed?
WARNING! You can overwrite variables or functions that have been stored in Python. For example, the command help = 2 will store the value 2 in the variable with name help . After this assignment help will behave like the value 2 instead of the function help . Therefore, you should always be careful not to give your variables the same name as built-in functions or values.
TIP! Now that you know how to assign variables, it is important that you learn to never leave unassigned commands. An unassigned command is an operation that has a result, but that result is not assigned to a variable. For example, you should never use 2+2 . You should instead assign it to some variable x=2+2 . This allows you to “hold on” to the results of previous commands and will make your interaction with Python must less confusing.
You can clear a variable from the notebook using the del function. Typing del x will clear the variable x from the workspace. If you want to remove all the variables in the notebook, you can use the magic command %reset .
In mathematics, variables are usually associated with unknown numbers; in programming, variables are associated with a value of a certain type. There are many data types that can be assigned to variables. A data type is a classification of the type of information that is being stored in a variable. The basic data types that you will utilize throughout this book are boolean, int, float, string, list, tuple, dictionary, set. A formal description of these data types is given in the following sections.
Assignment Operators
Add and assign, subtract and assign, multiply and assign, divide and assign, floor divide and assign, exponent and assign, modulo and assign.
to | |||
to and assigns the result to | |||
from and assigns the result to | |||
by and assigns the result to | |||
with and assigns the result to ; the result is always a float | |||
with and assigns the result to ; the result will be dependent on the type of values used | |||
to the power of and assigns the result to | |||
is divided by and assigns the result to |
For demonstration purposes, let’s use a single variable, num . Initially, we set num to 6. We can apply all of these operators to num and update it accordingly.
Assigning the value of 6 to num results in num being 6.
Expression: num = 6
Adding 3 to num and assigning the result back to num would result in 9.
Expression: num += 3
Subtracting 3 from num and assigning the result back to num would result in 6.
Expression: num -= 3
Multiplying num by 3 and assigning the result back to num would result in 18.
Expression: num *= 3
Dividing num by 3 and assigning the result back to num would result in 6.0 (always a float).
Expression: num /= 3
Performing floor division on num by 3 and assigning the result back to num would result in 2.
Expression: num //= 3
Raising num to the power of 3 and assigning the result back to num would result in 216.
Expression: num **= 3
Calculating the remainder when num is divided by 3 and assigning the result back to num would result in 2.
Expression: num %= 3
We can effectively put this into Python code, and you can experiment with the code yourself! Click the “Run” button to see the output.
The above code is useful when we want to update the same number. We can also use two different numbers and use the assignment operators to apply them on two different values.
- Read Tutorial
- Watch Guide Video
If that is about as clear as mud don't worry we're going to walk through a number of examples. And one very nice thing about the syntax for assignment operators is that it is nearly identical to a standard type of operator. So if you memorize the list of all the python operators then you're going to be able to use each one of these assignment operators quite easily.
The very first thing I'm going to do is let's first make sure that we can print out the total. So right here we have a total and it's an integer that equals 100. Now if we wanted to add say 10 to 100 how would we go about doing that? We could reassign the value total and we could say total and then just add 10. So let's see if this works right here. I'm going to run it and you can see we have a hundred and ten. So that works.

However, whenever you find yourself performing this type of calculation what you can do is use an assignment operator. And so the syntax for that is going to get rid of everything here in the middle and say plus equals and then whatever value. In this case I want to add onto it.
So you can see we have our operator and then right afterward you have an equal sign. And this is going to do is exactly like what we had before. So if I run this again you can see total is a hundred and ten

I'm going to just so you have a reference in the show notes I'm going to say that total equals total plus 10. This is exactly the same as what we're doing right here we're simply using assignment in order to do it.
I'm going to quickly go through each one of the other elements that you can use assignment for. And if you go back and you reference the show notes or your own notes for whenever you kept track of all of the different operators you're going to notice a trend. And that is because they're all exactly the same. So here if I want to subtract 10 from the total I can simply use the subtraction operator here run it again. And now you can see we have 90. Now don't be confused because we only temporarily change the value to 1 10. So when I commented this out and I ran it from scratch it took the total and it subtracted 10 from that total and that's what got printed out.
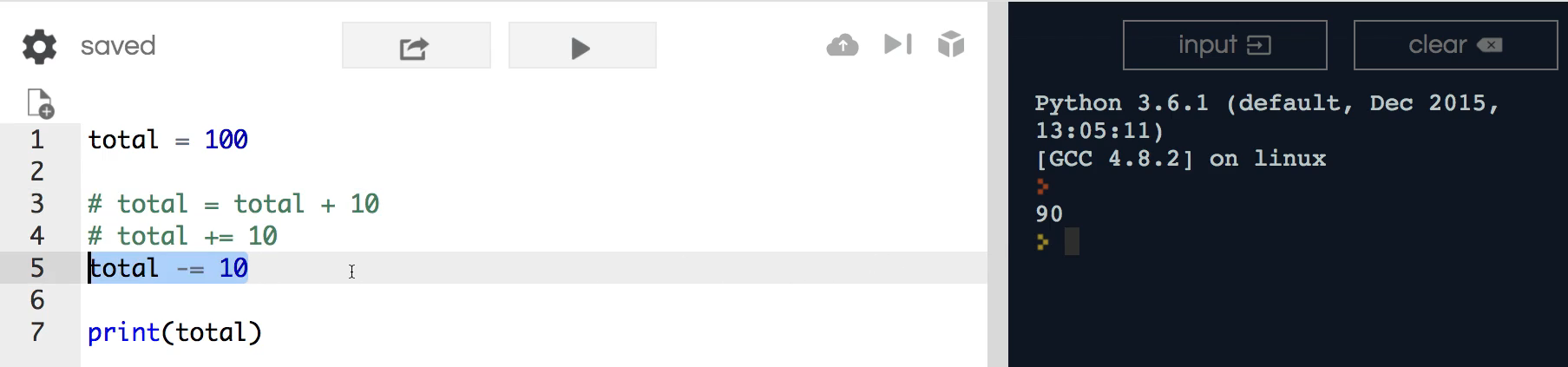
I'm going to copy this and the next one down the line is going to be multiplication. So in this case I'm going to say multiply with the asterisk the total and I'm just going to say times two just so we can see exactly what the value is going to be. And now we can see that's 200 which makes sense.
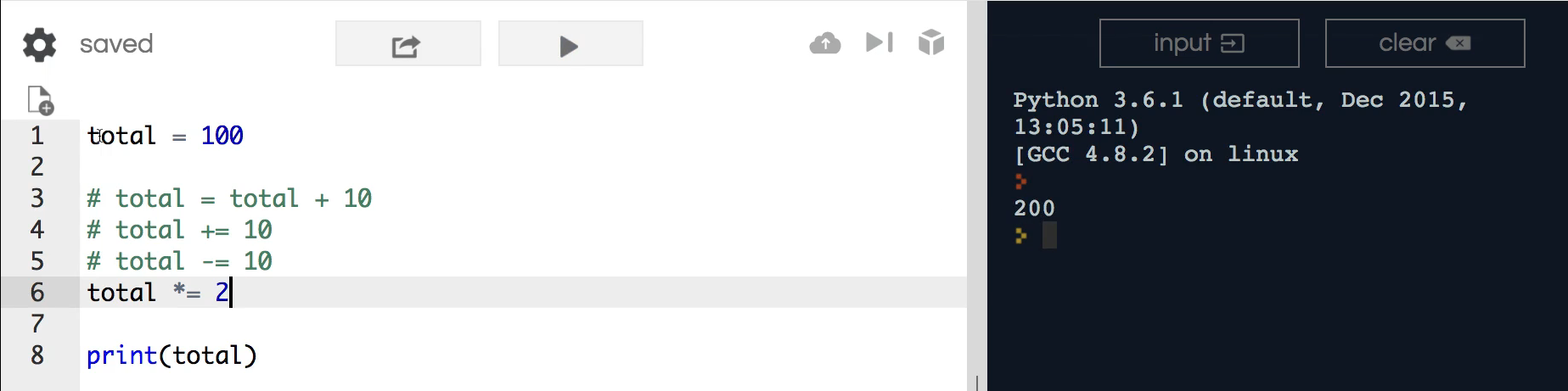
So we've taken total we have multiplied it by two and we have piped the entire thing into the total variable. So far so good. As you may have guessed next when we're going to do is division. So now I'm going to say total and then we're going to perform this division assignment and we're going to say divide this by 10 run it and you can see it gives us the value and it converts it to a float of ten point zero.
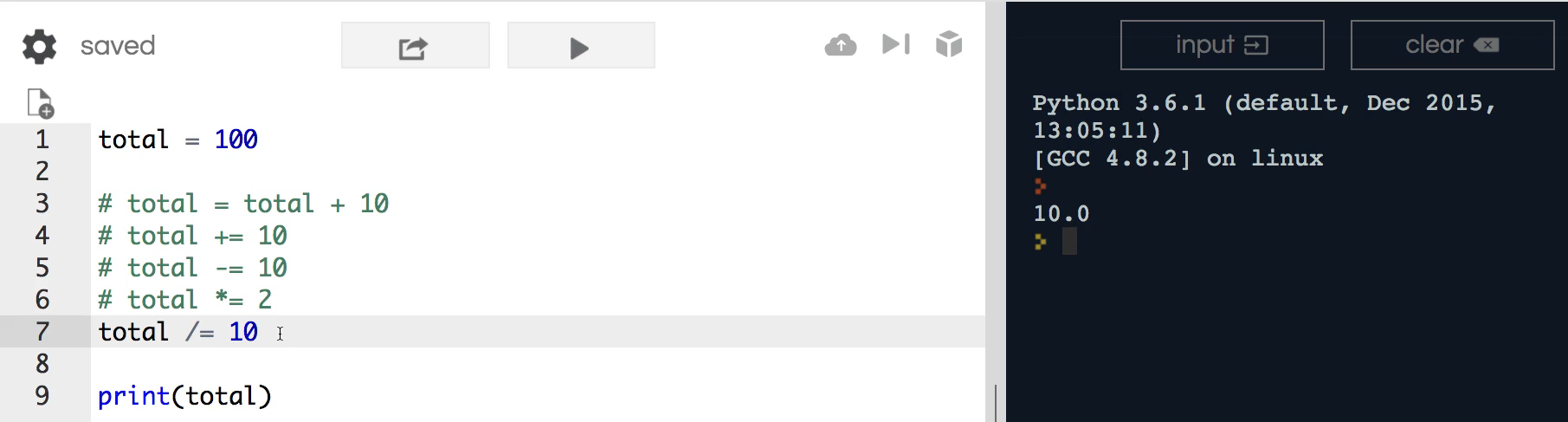
Now if this is starting to get a little bit much. Let's take a quick pause and see exactly what this is doing. Remember that all we're doing here is it's a shortcut. You could still perform it the same way we have in number 3 I could say total is equal to the total divided by 10. And if I run this you'll see we get ten point zero. And let's see what this warning is it says redefinition of total type from int to float. So we don't have to worry about this and this for if you're building Python programs you're very rarely ever going to see the syntax and it's because we have this assignment operator right here. So that is for division. And we also have the ability to use floor division as well. So if I run this you're going to see it's 10. But one thing you may notice is it's 10 it's not ten point zero. So remember that our floor division returns an integer it doesn't return a floating-point number. So if that is what you want then you can perform that task just like we did there.
Next one on the list is our exponents. I'm going to say the total and we're going to say we're going to assign that to the total squared. So going to run this and we get ten thousand. Just like you'd expect. And we have one more which is the modulus operator. So here remember it is the percent equals 2. And this is going to return zero because 100 is even if we changed 100 to be 101. This is going to return one because remember the typical purpose of the modulus operator is to let you know if you're working with an event or an odd value.
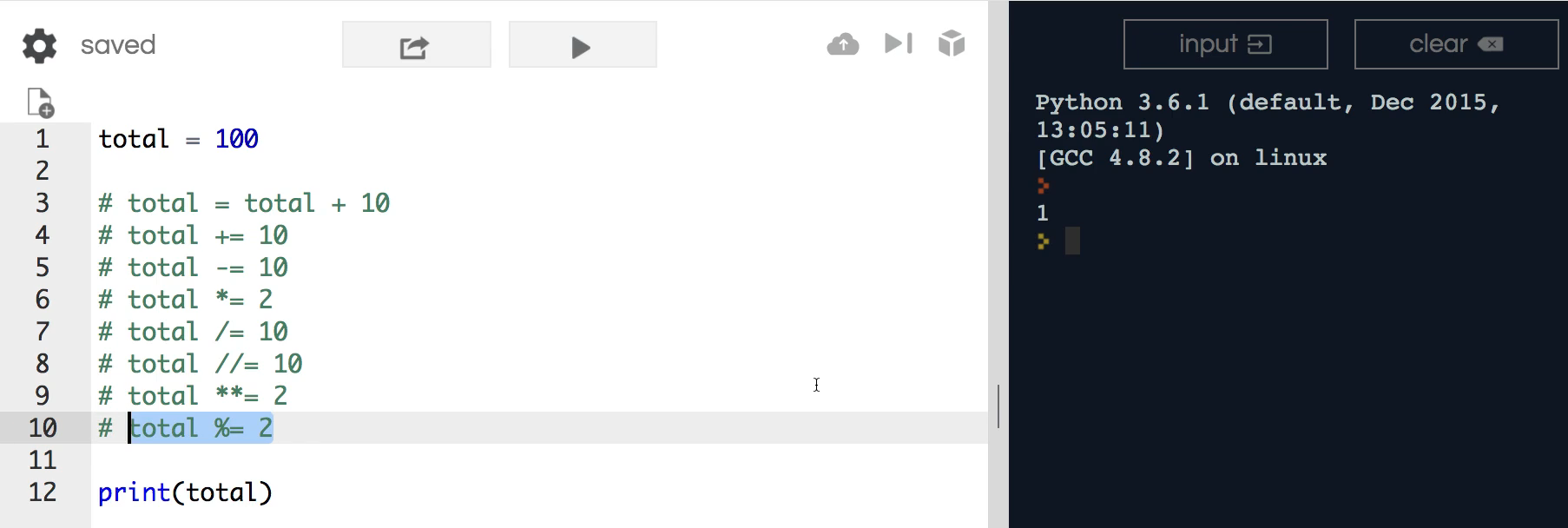
Now with all this being said, I wanted to show you every different option that you could use the assignment operator on. But I want to say that the most common way that you're going to use this or the most common one is going to be this one right here where we're adding or subtracting. So those are going to be the two most common. And what usually you're going to use that for is when you're incrementing or decrementing values so a very common way to do this would actually be like we have our total right here. So we have a total of 100 and you could imagine it being a shopping cart and it's 100 dollars and you could say product 2 and set this equal to 120. And then if I say product 3 and set this equal to 10. And so what I could do here is I could say total plus equals product to and then we could take the value and say product 3 and now if I run this you can see the value is 230.
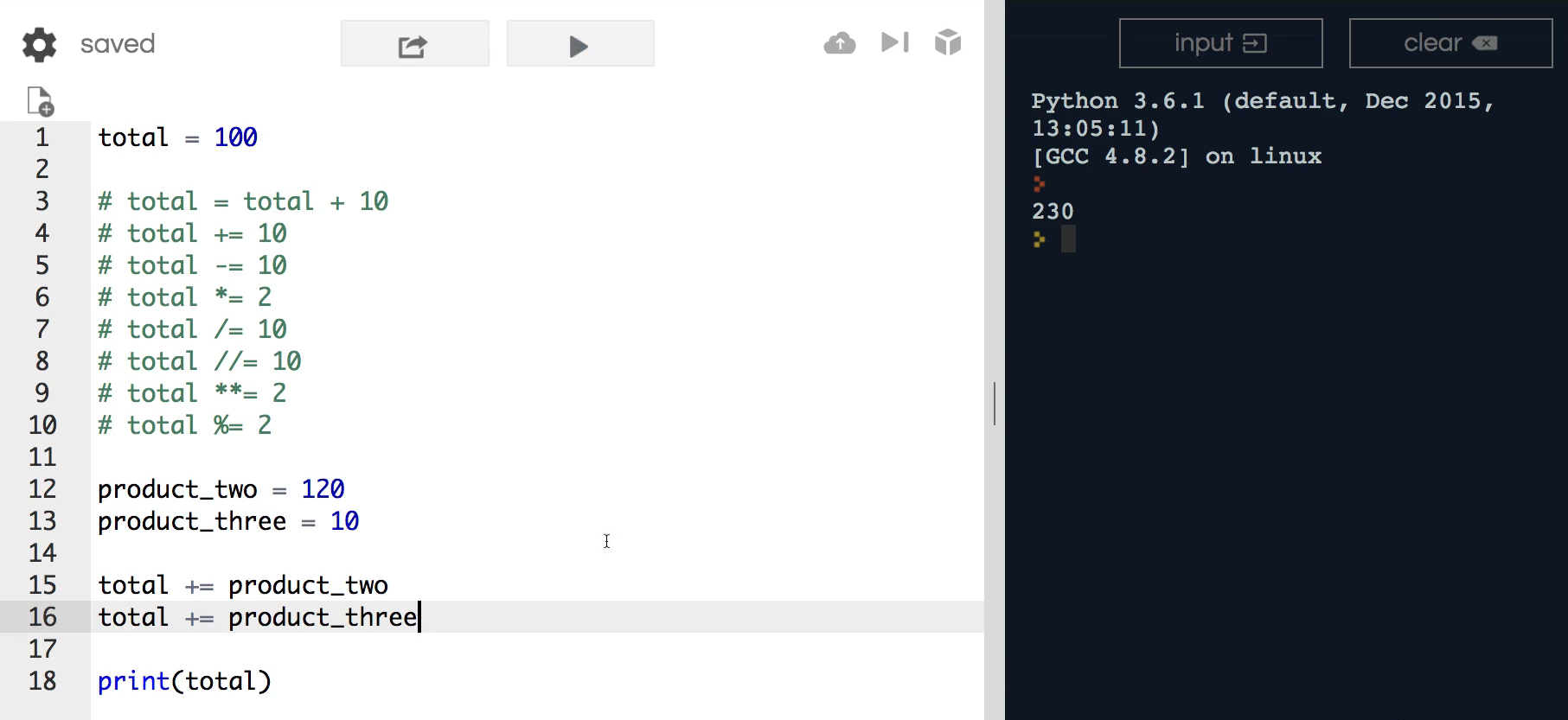
So that's a very common way whenever you want to generate a sum you can use this type of syntax which is much faster and it's also going to be a more pythonic way it's going to be the way you're going to see in standard Python programs whenever you're wanting to generate a sum and then reset and reassign the value.
So in review, that is how you can use assignment operators in Python.
devCamp does not support ancient browsers. Install a modern version for best experience.
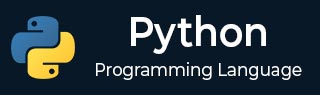
- Python Basics
- Python - Home
- Python - Overview
- Python - History
- Python - Features
- Python vs C++
- Python - Hello World Program
- Python - Application Areas
- Python - Interpreter
- Python - Environment Setup
- Python - Virtual Environment
- Python - Basic Syntax
- Python - Variables
- Python - Data Types
- Python - Type Casting
- Python - Unicode System
- Python - Literals
- Python - Operators
- Python - Arithmetic Operators
- Python - Comparison Operators
Python - Assignment Operators
- Python - Logical Operators
- Python - Bitwise Operators
- Python - Membership Operators
- Python - Identity Operators
- Python - Operator Precedence
- Python - Comments
- Python - User Input
- Python - Numbers
- Python - Booleans
- Python Control Statements
- Python - Control Flow
- Python - Decision Making
- Python - If Statement
- Python - If else
- Python - Nested If
- Python - Match-Case Statement
- Python - Loops
- Python - for Loops
- Python - for-else Loops
- Python - While Loops
- Python - break Statement
- Python - continue Statement
- Python - pass Statement
- Python - Nested Loops
- Python Functions & Modules
- Python - Functions
- Python - Default Arguments
- Python - Keyword Arguments
- Python - Keyword-Only Arguments
- Python - Positional Arguments
- Python - Positional-Only Arguments
- Python - Arbitrary Arguments
- Python - Variables Scope
- Python - Function Annotations
- Python - Modules
- Python - Built in Functions
- Python Strings
- Python - Strings
- Python - Slicing Strings
- Python - Modify Strings
- Python - String Concatenation
- Python - String Formatting
- Python - Escape Characters
- Python - String Methods
- Python - String Exercises
- Python Lists
- Python - Lists
- Python - Access List Items
- Python - Change List Items
- Python - Add List Items
- Python - Remove List Items
- Python - Loop Lists
- Python - List Comprehension
- Python - Sort Lists
- Python - Copy Lists
- Python - Join Lists
- Python - List Methods
- Python - List Exercises
- Python Tuples
- Python - Tuples
- Python - Access Tuple Items
- Python - Update Tuples
- Python - Unpack Tuples
- Python - Loop Tuples
- Python - Join Tuples
- Python - Tuple Methods
- Python - Tuple Exercises
- Python Sets
- Python - Sets
- Python - Access Set Items
- Python - Add Set Items
- Python - Remove Set Items
- Python - Loop Sets
- Python - Join Sets
- Python - Copy Sets
- Python - Set Operators
- Python - Set Methods
- Python - Set Exercises
- Python Dictionaries
- Python - Dictionaries
- Python - Access Dictionary Items
- Python - Change Dictionary Items
- Python - Add Dictionary Items
- Python - Remove Dictionary Items
- Python - Dictionary View Objects
- Python - Loop Dictionaries
- Python - Copy Dictionaries
- Python - Nested Dictionaries
- Python - Dictionary Methods
- Python - Dictionary Exercises
- Python Arrays
- Python - Arrays
- Python - Access Array Items
- Python - Add Array Items
- Python - Remove Array Items
- Python - Loop Arrays
- Python - Copy Arrays
- Python - Reverse Arrays
- Python - Sort Arrays
- Python - Join Arrays
- Python - Array Methods
- Python - Array Exercises
- Python File Handling
- Python - File Handling
- Python - Write to File
- Python - Read Files
- Python - Renaming and Deleting Files
- Python - Directories
- Python - File Methods
- Python - OS File/Directory Methods
- Python - OS.Path Methods
- Object Oriented Programming
- Python - OOPs Concepts
- Python - Object & Classes
- Python - Class Attributes
- Python - Class Methods
- Python - Static Methods
- Python - Constructors
- Python - Access Modifiers
- Python - Inheritance
- Python - Polymorphism
- Python - Method Overriding
- Python - Method Overloading
- Python - Dynamic Binding
- Python - Dynamic Typing
- Python - Abstraction
- Python - Encapsulation
- Python - Interfaces
- Python - Packages
- Python - Inner Classes
- Python - Anonymous Class and Objects
- Python - Singleton Class
- Python - Wrapper Classes
- Python - Enums
- Python - Reflection
- Python Errors & Exceptions
- Python - Syntax Errors
- Python - Exceptions
- Python - try-except Block
- Python - try-finally Block
- Python - Raising Exceptions
- Python - Exception Chaining
- Python - Nested try Block
- Python - User-defined Exception
- Python - Logging
- Python - Assertions
- Python - Built-in Exceptions
- Python Multithreading
- Python - Multithreading
- Python - Thread Life Cycle
- Python - Creating a Thread
- Python - Starting a Thread
- Python - Joining Threads
- Python - Naming Thread
- Python - Thread Scheduling
- Python - Thread Pools
- Python - Main Thread
- Python - Thread Priority
- Python - Daemon Threads
- Python - Synchronizing Threads
- Python Synchronization
- Python - Inter-thread Communication
- Python - Thread Deadlock
- Python - Interrupting a Thread
- Python Networking
- Python - Networking
- Python - Socket Programming
- Python - URL Processing
- Python - Generics
- Python Libraries
- NumPy Tutorial
- Pandas Tutorial
- SciPy Tutorial
- Matplotlib Tutorial
- Django Tutorial
- OpenCV Tutorial
- Python Miscellenous
- Python - Date & Time
- Python - Maths
- Python - Iterators
- Python - Generators
- Python - Closures
- Python - Decorators
- Python - Recursion
- Python - Reg Expressions
- Python - PIP
- Python - Database Access
- Python - Weak References
- Python - Serialization
- Python - Templating
- Python - Output Formatting
- Python - Performance Measurement
- Python - Data Compression
- Python - CGI Programming
- Python - XML Processing
- Python - GUI Programming
- Python - Command-Line Arguments
- Python - Docstrings
- Python - JSON
- Python - Sending Email
- Python - Further Extensions
- Python - Tools/Utilities
- Python - GUIs
- Python Useful Resources
- Python Compiler
- NumPy Compiler
- Matplotlib Compiler
- SciPy Compiler
- Python - Questions & Answers
- Python - Online Quiz
- Python - Programming Examples
- Python - Quick Guide
- Python - Useful Resources
- Python - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
Python Assignment Operator
The = (equal to) symbol is defined as assignment operator in Python. The value of Python expression on its right is assigned to a single variable on its left. The = symbol as in programming in general (and Python in particular) should not be confused with its usage in Mathematics, where it states that the expressions on the either side of the symbol are equal.
Example of Assignment Operator in Python
Consider following Python statements −
At the first instance, at least for somebody new to programming but who knows maths, the statement "a=a+b" looks strange. How could a be equal to "a+b"? However, it needs to be reemphasized that the = symbol is an assignment operator here and not used to show the equality of LHS and RHS.
Because it is an assignment, the expression on right evaluates to 15, the value is assigned to a.
In the statement "a+=b", the two operators "+" and "=" can be combined in a "+=" operator. It is called as add and assign operator. In a single statement, it performs addition of two operands "a" and "b", and result is assigned to operand on left, i.e., "a".
Augmented Assignment Operators in Python
In addition to the simple assignment operator, Python provides few more assignment operators for advanced use. They are called cumulative or augmented assignment operators. In this chapter, we shall learn to use augmented assignment operators defined in Python.
Python has the augmented assignment operators for all arithmetic and comparison operators.
Python augmented assignment operators combines addition and assignment in one statement. Since Python supports mixed arithmetic, the two operands may be of different types. However, the type of left operand changes to the operand of on right, if it is wider.
The += operator is an augmented operator. It is also called cumulative addition operator, as it adds "b" in "a" and assigns the result back to a variable.
The following are the augmented assignment operators in Python:
- Augmented Addition Operator
- Augmented Subtraction Operator
- Augmented Multiplication Operator
- Augmented Division Operator
- Augmented Modulus Operator
- Augmented Exponent Operator
- Augmented Floor division Operator
Augmented Addition Operator (+=)
Following examples will help in understanding how the "+=" operator works −
It will produce the following output −
Augmented Subtraction Operator (-=)
Use -= symbol to perform subtract and assign operations in a single statement. The "a-=b" statement performs "a=a-b" assignment. Operands may be of any number type. Python performs implicit type casting on the object which is narrower in size.
Augmented Multiplication Operator (*=)
The "*=" operator works on similar principle. "a*=b" performs multiply and assign operations, and is equivalent to "a=a*b". In case of augmented multiplication of two complex numbers, the rule of multiplication as discussed in the previous chapter is applicable.
Augmented Division Operator (/=)
The combination symbol "/=" acts as divide and assignment operator, hence "a/=b" is equivalent to "a=a/b". The division operation of int or float operands is float. Division of two complex numbers returns a complex number. Given below are examples of augmented division operator.
Augmented Modulus Operator (%=)
To perform modulus and assignment operation in a single statement, use the %= operator. Like the mod operator, its augmented version also is not supported for complex number.
Augmented Exponent Operator (**=)
The "**=" operator results in computation of "a" raised to "b", and assigning the value back to "a". Given below are some examples −
Augmented Floor division Operator (//=)
For performing floor division and assignment in a single statement, use the "//=" operator. "a//=b" is equivalent to "a=a//b". This operator cannot be used with complex numbers.
To Continue Learning Please Login
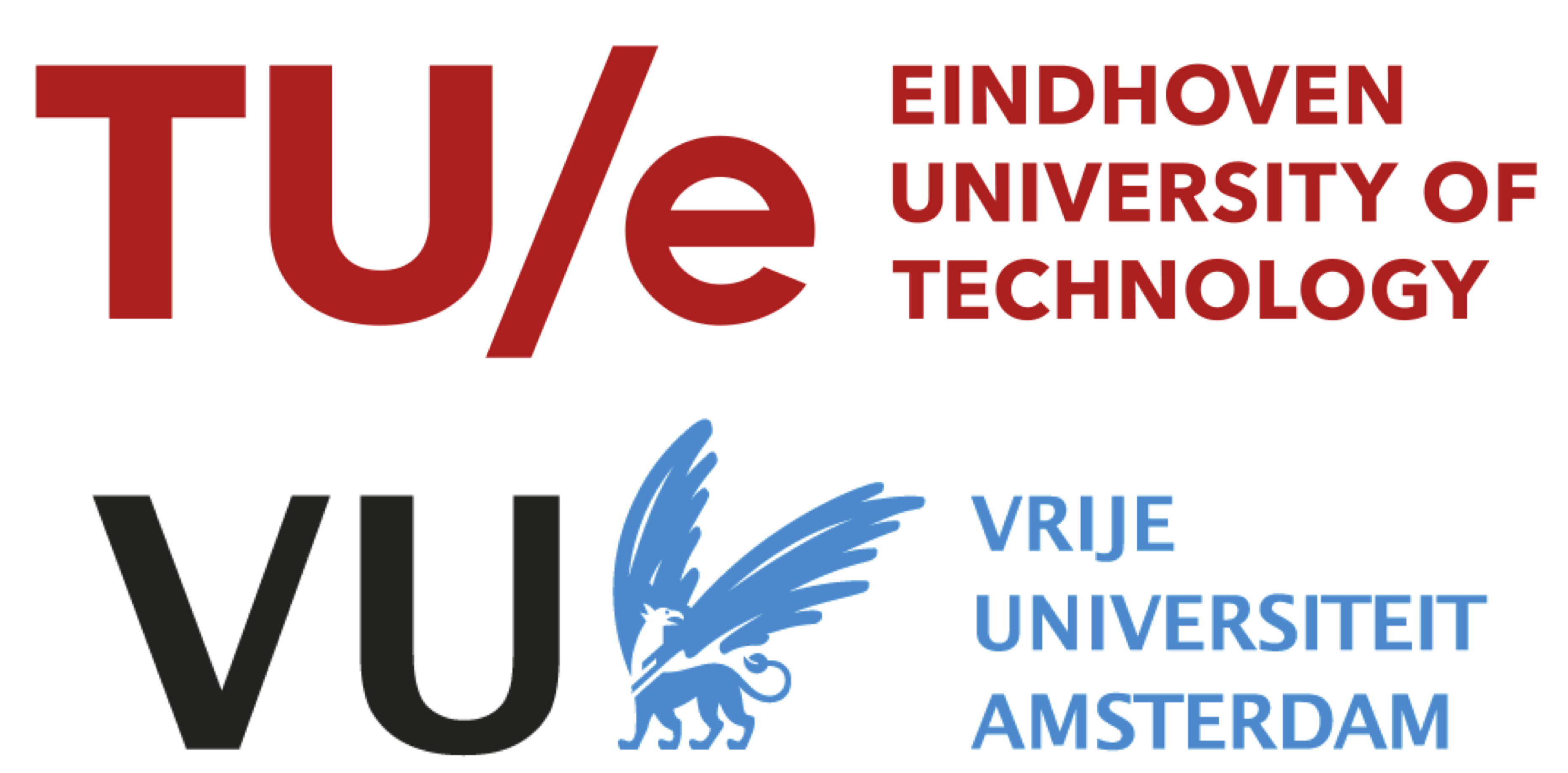
Learning Python by doing
- suggest edit
Variables, Expressions, and Assignments
Variables, expressions, and assignments 1 #, introduction #.
In this chapter, we introduce some of the main building blocks needed to create programs–that is, variables, expressions, and assignments. Programming related variables can be intepret in the same way that we interpret mathematical variables, as elements that store values that can later be changed. Usually, variables and values are used within the so-called expressions. Once again, just as in mathematics, an expression is a construct of values and variables connected with operators that result in a new value. Lastly, an assignment is a language construct know as an statement that assign a value (either as a constant or expression) to a variable. The rest of this notebook will dive into the main concepts that we need to fully understand these three language constructs.
Values and Types #
A value is the basic unit used in a program. It may be, for instance, a number respresenting temperature. It may be a string representing a word. Some values are 42, 42.0, and ‘Hello, Data Scientists!’.
Each value has its own type : 42 is an integer ( int in Python), 42.0 is a floating-point number ( float in Python), and ‘Hello, Data Scientists!’ is a string ( str in Python).
The Python interpreter can tell you the type of a value: the function type takes a value as argument and returns its corresponding type.
Observe the difference between type(42) and type('42') !
Expressions and Statements #
On the one hand, an expression is a combination of values, variables, and operators.
A value all by itself is considered an expression, and so is a variable.
When you type an expression at the prompt, the interpreter evaluates it, which means that it calculates the value of the expression and displays it.
In boxes above, m has the value 27 and m + 25 has the value 52 . m + 25 is said to be an expression.
On the other hand, a statement is an instruction that has an effect, like creating a variable or displaying a value.
The first statement initializes the variable n with the value 17 , this is a so-called assignment statement .
The second statement is a print statement that prints the value of the variable n .
The effect is not always visible. Assigning a value to a variable is not visible, but printing the value of a variable is.
Assignment Statements #
We have already seen that Python allows you to evaluate expressions, for instance 40 + 2 . It is very convenient if we are able to store the calculated value in some variable for future use. The latter can be done via an assignment statement. An assignment statement creates a new variable with a given name and assigns it a value.
The example in the previous code contains three assignments. The first one assigns the value of the expression 40 + 2 to a new variable called magicnumber ; the second one assigns the value of π to the variable pi , and; the last assignment assigns the string value 'Data is eatig the world' to the variable message .
Programmers generally choose names for their variables that are meaningful. In this way, they document what the variable is used for.
Do It Yourself!
Let’s compute the volume of a cube with side \(s = 5\) . Remember that the volume of a cube is defined as \(v = s^3\) . Assign the value to a variable called volume .
Well done! Now, why don’t you print the result in a message? It can say something like “The volume of the cube with side 5 is \(volume\) ”.
Beware that there is no checking of types ( type checking ) in Python, so a variable to which you have assigned an integer may be re-used as a float, even if we provide type-hints .
Names and Keywords #
Names of variable and other language constructs such as functions (we will cover this topic later), should be meaningful and reflect the purpose of the construct.
In general, Python names should adhere to the following rules:
It should start with a letter or underscore.
It cannot start with a number.
It must only contain alpha-numeric (i.e., letters a-z A-Z and digits 0-9) characters and underscores.
They cannot share the name of a Python keyword.
If you use illegal variable names you will get a syntax error.
By choosing the right variables names you make the code self-documenting, what is better the variable v or velocity ?
The following are examples of invalid variable names.
These basic development principles are sometimes called architectural rules . By defining and agreeing upon architectural rules you make it easier for you and your fellow developers to understand and modify your code.
If you want to read more on this, please have a look at Code complete a book by Steven McConnell [ McC04 ] .
Every programming language has a collection of reserved keywords . They are used in predefined language constructs, such as loops and conditionals . These language concepts and their usage will be explained later.
The interpreter uses keywords to recognize these language constructs in a program. Python 3 has the following keywords:
False class finally is return
None continue for lambda try
True def from nonlocal while
and del global not with
as elif if or yield
assert else import pass break
except in raise
Reassignments #
It is allowed to assign a new value to an existing variable. This process is called reassignment . As soon as you assign a value to a variable, the old value is lost.
The assignment of a variable to another variable, for instance b = a does not imply that if a is reassigned then b changes as well.
You have a variable salary that shows the weekly salary of an employee. However, you want to compute the monthly salary. Can you reassign the value to the salary variable according to the instruction?
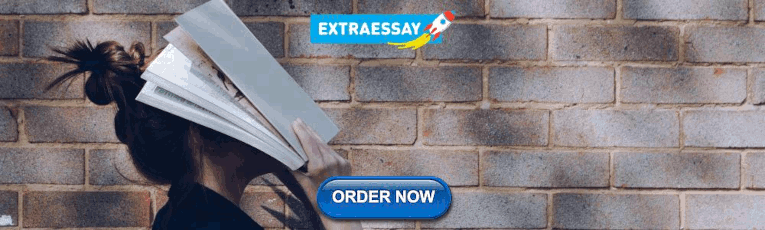
Updating Variables #
A frequently used reassignment is for updating puposes: the value of a variable depends on the previous value of the variable.
This statement expresses “get the current value of x , add one, and then update x with the new value.”
Beware, that the variable should be initialized first, usually with a simple assignment.
Do you remember the salary excercise of the previous section (cf. 13. Reassignments)? Well, if you have not done it yet, update the salary variable by using its previous value.
Updating a variable by adding 1 is called an increment ; subtracting 1 is called a decrement . A shorthand way of doing is using += and -= , which stands for x = x + ... and x = x - ... respectively.
Order of Operations #
Expressions may contain multiple operators. The order of evaluation depends on the priorities of the operators also known as rules of precedence .
For mathematical operators, Python follows mathematical convention. The acronym PEMDAS is a useful way to remember the rules:
Parentheses have the highest precedence and can be used to force an expression to evaluate in the order you want. Since expressions in parentheses are evaluated first, 2 * (3 - 1) is 4 , and (1 + 1)**(5 - 2) is 8 . You can also use parentheses to make an expression easier to read, even if it does not change the result.
Exponentiation has the next highest precedence, so 1 + 2**3 is 9 , not 27 , and 2 * 3**2 is 18 , not 36 .
Multiplication and division have higher precedence than addition and subtraction . So 2 * 3 - 1 is 5 , not 4 , and 6 + 4 / 2 is 8 , not 5 .
Operators with the same precedence are evaluated from left to right (except exponentiation). So in the expression degrees / 2 * pi , the division happens first and the result is multiplied by pi . To divide by 2π, you can use parentheses or write: degrees / 2 / pi .
In case of doubt, use parentheses!
Let’s see what happens when we evaluate the following expressions. Just run the cell to check the resulting value.
Floor Division and Modulus Operators #
The floor division operator // divides two numbers and rounds down to an integer.
For example, suppose that driving to the south of France takes 555 minutes. You might want to know how long that is in hours.
Conventional division returns a floating-point number.
Hours are normally not represented with decimal points. Floor division returns the integer number of hours, dropping the fraction part.
You spend around 225 minutes every week on programming activities. You want to know around how many hours you invest to this activity during a month. Use the \(//\) operator to give the answer.
The modulus operator % works on integer values. It computes the remainder when dividing the first integer by the second one.
The modulus operator is more useful than it seems.
For example, you can check whether one number is divisible by another—if x % y is zero, then x is divisible by y .
String Operations #
In general, you cannot perform mathematical operations on strings, even if the strings look like numbers, so the following operations are illegal: '2'-'1' 'eggs'/'easy' 'third'*'a charm'
But there are two exceptions, + and * .
The + operator performs string concatenation, which means it joins the strings by linking them end-to-end.
The * operator also works on strings; it performs repetition.
Speedy Gonzales is a cartoon known to be the fastest mouse in all Mexico . He is also famous for saying “Arriba Arriba Andale Arriba Arriba Yepa”. Can you use the following variables, namely arriba , andale and yepa to print the mentioned expression? Don’t forget to use the string operators.
Asking the User for Input #
The programs we have written so far accept no input from the user.
To get data from the user through the Python prompt, we can use the built-in function input .
When input is called your whole program stops and waits for the user to enter the required data. Once the user types the value and presses Return or Enter , the function returns the input value as a string and the program continues with its execution.
Try it out!
You can also print a message to clarify the purpose of the required input as follows.
The resulting string can later be translated to a different type, like an integer or a float. To do so, you use the functions int and float , respectively. But be careful, the user might introduce a value that cannot be converted to the type you required.
We want to know the name of a user so we can display a welcome message in our program. The message should say something like “Hello \(name\) , welcome to our hello world program!”.
Script Mode #
So far we have run Python in interactive mode in these Jupyter notebooks, which means that you interact directly with the interpreter in the code cells . The interactive mode is a good way to get started, but if you are working with more than a few lines of code, it can be clumsy. The alternative is to save code in a file called a script and then run the interpreter in script mode to execute the script. By convention, Python scripts have names that end with .py .
Use the PyCharm icon in Anaconda Navigator to create and execute stand-alone Python scripts. Later in the course, you will have to work with Python projects for the assignments, in order to get acquainted with another way of interacing with Python code.
This Jupyter Notebook is based on Chapter 2 of the books Python for Everybody [ Sev16 ] and Think Python (Sections 5.1, 7.1, 7.2, and 5.12) [ Dow15 ] .
- Python »
- 3.12.4 Documentation »
- The Python Tutorial »
- 3. An Informal Introduction to Python
- Theme Auto Light Dark |
3. An Informal Introduction to Python ¶
In the following examples, input and output are distinguished by the presence or absence of prompts ( >>> and … ): to repeat the example, you must type everything after the prompt, when the prompt appears; lines that do not begin with a prompt are output from the interpreter. Note that a secondary prompt on a line by itself in an example means you must type a blank line; this is used to end a multi-line command.
You can toggle the display of prompts and output by clicking on >>> in the upper-right corner of an example box. If you hide the prompts and output for an example, then you can easily copy and paste the input lines into your interpreter.
Many of the examples in this manual, even those entered at the interactive prompt, include comments. Comments in Python start with the hash character, # , and extend to the end of the physical line. A comment may appear at the start of a line or following whitespace or code, but not within a string literal. A hash character within a string literal is just a hash character. Since comments are to clarify code and are not interpreted by Python, they may be omitted when typing in examples.
Some examples:
3.1. Using Python as a Calculator ¶
Let’s try some simple Python commands. Start the interpreter and wait for the primary prompt, >>> . (It shouldn’t take long.)
3.1.1. Numbers ¶
The interpreter acts as a simple calculator: you can type an expression at it and it will write the value. Expression syntax is straightforward: the operators + , - , * and / can be used to perform arithmetic; parentheses ( () ) can be used for grouping. For example:
The integer numbers (e.g. 2 , 4 , 20 ) have type int , the ones with a fractional part (e.g. 5.0 , 1.6 ) have type float . We will see more about numeric types later in the tutorial.
Division ( / ) always returns a float. To do floor division and get an integer result you can use the // operator; to calculate the remainder you can use % :
With Python, it is possible to use the ** operator to calculate powers [ 1 ] :
The equal sign ( = ) is used to assign a value to a variable. Afterwards, no result is displayed before the next interactive prompt:
If a variable is not “defined” (assigned a value), trying to use it will give you an error:
There is full support for floating point; operators with mixed type operands convert the integer operand to floating point:
In interactive mode, the last printed expression is assigned to the variable _ . This means that when you are using Python as a desk calculator, it is somewhat easier to continue calculations, for example:
This variable should be treated as read-only by the user. Don’t explicitly assign a value to it — you would create an independent local variable with the same name masking the built-in variable with its magic behavior.
In addition to int and float , Python supports other types of numbers, such as Decimal and Fraction . Python also has built-in support for complex numbers , and uses the j or J suffix to indicate the imaginary part (e.g. 3+5j ).
3.1.2. Text ¶
Python can manipulate text (represented by type str , so-called “strings”) as well as numbers. This includes characters “ ! ”, words “ rabbit ”, names “ Paris ”, sentences “ Got your back. ”, etc. “ Yay! :) ”. They can be enclosed in single quotes ( '...' ) or double quotes ( "..." ) with the same result [ 2 ] .
To quote a quote, we need to “escape” it, by preceding it with \ . Alternatively, we can use the other type of quotation marks:
In the Python shell, the string definition and output string can look different. The print() function produces a more readable output, by omitting the enclosing quotes and by printing escaped and special characters:
If you don’t want characters prefaced by \ to be interpreted as special characters, you can use raw strings by adding an r before the first quote:
There is one subtle aspect to raw strings: a raw string may not end in an odd number of \ characters; see the FAQ entry for more information and workarounds.
String literals can span multiple lines. One way is using triple-quotes: """...""" or '''...''' . End of lines are automatically included in the string, but it’s possible to prevent this by adding a \ at the end of the line. The following example:
produces the following output (note that the initial newline is not included):
Strings can be concatenated (glued together) with the + operator, and repeated with * :
Two or more string literals (i.e. the ones enclosed between quotes) next to each other are automatically concatenated.
This feature is particularly useful when you want to break long strings:
This only works with two literals though, not with variables or expressions:
If you want to concatenate variables or a variable and a literal, use + :
Strings can be indexed (subscripted), with the first character having index 0. There is no separate character type; a character is simply a string of size one:
Indices may also be negative numbers, to start counting from the right:
Note that since -0 is the same as 0, negative indices start from -1.
In addition to indexing, slicing is also supported. While indexing is used to obtain individual characters, slicing allows you to obtain a substring:
Slice indices have useful defaults; an omitted first index defaults to zero, an omitted second index defaults to the size of the string being sliced.
Note how the start is always included, and the end always excluded. This makes sure that s[:i] + s[i:] is always equal to s :
One way to remember how slices work is to think of the indices as pointing between characters, with the left edge of the first character numbered 0. Then the right edge of the last character of a string of n characters has index n , for example:
The first row of numbers gives the position of the indices 0…6 in the string; the second row gives the corresponding negative indices. The slice from i to j consists of all characters between the edges labeled i and j , respectively.
For non-negative indices, the length of a slice is the difference of the indices, if both are within bounds. For example, the length of word[1:3] is 2.
Attempting to use an index that is too large will result in an error:
However, out of range slice indexes are handled gracefully when used for slicing:
Python strings cannot be changed — they are immutable . Therefore, assigning to an indexed position in the string results in an error:
If you need a different string, you should create a new one:
The built-in function len() returns the length of a string:
Strings are examples of sequence types , and support the common operations supported by such types.
Strings support a large number of methods for basic transformations and searching.
String literals that have embedded expressions.
Information about string formatting with str.format() .
The old formatting operations invoked when strings are the left operand of the % operator are described in more detail here.
3.1.3. Lists ¶
Python knows a number of compound data types, used to group together other values. The most versatile is the list , which can be written as a list of comma-separated values (items) between square brackets. Lists might contain items of different types, but usually the items all have the same type.
Like strings (and all other built-in sequence types), lists can be indexed and sliced:
Lists also support operations like concatenation:
Unlike strings, which are immutable , lists are a mutable type, i.e. it is possible to change their content:
You can also add new items at the end of the list, by using the list.append() method (we will see more about methods later):
Simple assignment in Python never copies data. When you assign a list to a variable, the variable refers to the existing list . Any changes you make to the list through one variable will be seen through all other variables that refer to it.:
All slice operations return a new list containing the requested elements. This means that the following slice returns a shallow copy of the list:
Assignment to slices is also possible, and this can even change the size of the list or clear it entirely:
The built-in function len() also applies to lists:
It is possible to nest lists (create lists containing other lists), for example:
3.2. First Steps Towards Programming ¶
Of course, we can use Python for more complicated tasks than adding two and two together. For instance, we can write an initial sub-sequence of the Fibonacci series as follows:
This example introduces several new features.
The first line contains a multiple assignment : the variables a and b simultaneously get the new values 0 and 1. On the last line this is used again, demonstrating that the expressions on the right-hand side are all evaluated first before any of the assignments take place. The right-hand side expressions are evaluated from the left to the right.
The while loop executes as long as the condition (here: a < 10 ) remains true. In Python, like in C, any non-zero integer value is true; zero is false. The condition may also be a string or list value, in fact any sequence; anything with a non-zero length is true, empty sequences are false. The test used in the example is a simple comparison. The standard comparison operators are written the same as in C: < (less than), > (greater than), == (equal to), <= (less than or equal to), >= (greater than or equal to) and != (not equal to).
The body of the loop is indented : indentation is Python’s way of grouping statements. At the interactive prompt, you have to type a tab or space(s) for each indented line. In practice you will prepare more complicated input for Python with a text editor; all decent text editors have an auto-indent facility. When a compound statement is entered interactively, it must be followed by a blank line to indicate completion (since the parser cannot guess when you have typed the last line). Note that each line within a basic block must be indented by the same amount.
The print() function writes the value of the argument(s) it is given. It differs from just writing the expression you want to write (as we did earlier in the calculator examples) in the way it handles multiple arguments, floating point quantities, and strings. Strings are printed without quotes, and a space is inserted between items, so you can format things nicely, like this:
The keyword argument end can be used to avoid the newline after the output, or end the output with a different string:
Table of Contents
- 3.1.1. Numbers
- 3.1.2. Text
- 3.1.3. Lists
- 3.2. First Steps Towards Programming
Previous topic
2. Using the Python Interpreter
4. More Control Flow Tools
- Report a Bug
- Show Source
- Docs »
- += Addition Assignment
- Edit on GitHub
+= Addition Assignment ¶
Description ¶.
Adds a value and the variable and assigns the result to that variable.
Return Value ¶
According to coercion rules.
Time Complexity ¶
Equivalent to A = A + B.
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
- Português (do Brasil)
JavaScript ( JS ) is a lightweight interpreted (or just-in-time compiled) programming language with first-class functions . While it is most well-known as the scripting language for Web pages, many non-browser environments also use it, such as Node.js , Apache CouchDB and Adobe Acrobat . JavaScript is a prototype-based , multi-paradigm, single-threaded , dynamic language, supporting object-oriented, imperative, and declarative (e.g. functional programming) styles.
JavaScript's dynamic capabilities include runtime object construction, variable parameter lists, function variables, dynamic script creation (via eval ), object introspection (via for...in and Object utilities ), and source-code recovery (JavaScript functions store their source text and can be retrieved through toString() ).
This section is dedicated to the JavaScript language itself, and not the parts that are specific to Web pages or other host environments. For information about APIs that are specific to Web pages, please see Web APIs and DOM .
The standards for JavaScript are the ECMAScript Language Specification (ECMA-262) and the ECMAScript Internationalization API specification (ECMA-402). As soon as one browser implements a feature, we try to document it. This means that cases where some proposals for new ECMAScript features have already been implemented in browsers, documentation and examples in MDN articles may use some of those new features. Most of the time, this happens between the stages 3 and 4, and is usually before the spec is officially published.
Do not confuse JavaScript with the Java programming language — JavaScript is not "Interpreted Java" . Both "Java" and "JavaScript" are trademarks or registered trademarks of Oracle in the U.S. and other countries. However, the two programming languages have very different syntax, semantics, and use.
JavaScript documentation of core language features (pure ECMAScript , for the most part) includes the following:
- The JavaScript guide
- The JavaScript reference
For more information about JavaScript specifications and related technologies, see JavaScript technologies overview .
Learn how to program in JavaScript with guides and tutorials.
For complete beginners
Head over to our Learning Area JavaScript topic if you want to learn JavaScript but have no previous experience with JavaScript or programming. The complete modules available there are as follows:
Answers some fundamental questions such as "what is JavaScript?", "what does it look like?", and "what can it do?", along with discussing key JavaScript features such as variables, strings, numbers, and arrays.
Continues our coverage of JavaScript's key fundamental features, turning our attention to commonly-encountered types of code blocks such as conditional statements, loops, functions, and events.
The object-oriented nature of JavaScript is important to understand if you want to go further with your knowledge of the language and write more efficient code, therefore we've provided this module to help you.
Discusses asynchronous JavaScript, why it is important, and how it can be used to effectively handle potential blocking operations such as fetching resources from a server.
Explores what APIs are, and how to use some of the most common APIs you'll come across often in your development work.
JavaScript guide
A much more detailed guide to the JavaScript language, aimed at those with previous programming experience either in JavaScript or another language.
Intermediate
JavaScript frameworks are an essential part of modern front-end web development, providing developers with proven tools for building scalable, interactive web applications. This module gives you some fundamental background knowledge about how client-side frameworks work and how they fit into your toolset, before moving on to a series of tutorials covering some of today's most popular ones.
An overview of the basic syntax and semantics of JavaScript for those coming from other programming languages to get up to speed.
Overview of available data structures in JavaScript.
JavaScript provides three different value comparison operations: strict equality using === , loose equality using == , and the Object.is() method.
How different methods that visit a group of object properties one-by-one handle the enumerability and ownership of properties.
A closure is the combination of a function and the lexical environment within which that function was declared.
Explanation of the widely misunderstood and underestimated prototype-based inheritance.
Memory life cycle and garbage collection in JavaScript.
JavaScript has a runtime model based on an "event loop".
Browse the complete JavaScript reference documentation.
Get to know standard built-in objects Array , Boolean , Date , Error , Function , JSON , Math , Number , Object , RegExp , String , Map , Set , WeakMap , WeakSet , and others.
Learn more about the behavior of JavaScript's operators instanceof , typeof , new , this , the operator precedence , and more.
Learn how do-while , for-in , for-of , try-catch , let , var , const , if-else , switch , and more JavaScript statements and keywords work.
Learn how to work with JavaScript's functions to develop your applications.
JavaScript classes are the most appropriate way to do object-oriented programming.
- Trending Now
- Foundational Courses
- Data Science
- Practice Problem
- Machine Learning
- System Design
- DevOps Tutorial
Default Arguments in Python
In this video, we will explore the concept of default arguments in Python. Default arguments allow you to define functions with default values for parameters, making your functions more flexible and easier to use. This tutorial is perfect for students, professionals, or anyone interested in enhancing their Python programming skills by understanding and utilizing default arguments effectively.
Why Use Default Arguments?
Default arguments enable you to call functions with fewer arguments than they are defined to accept. This makes your code more concise and provides a way to handle optional parameters without needing to overload functions or use complex logic.
Key Concepts
1. Default Arguments:
- Parameters that assume a default value if no argument is provided during the function call.
2. Function Definition:
- Default values are specified in the function definition using the assignment operator (=).
3. Function Call:
- When calling the function, if no argument is provided for a default parameter, the default value is used.
Steps to Use Default Arguments
Step 1: Define a Function with Default Arguments
- Define a function with parameters, some of which have default values assigned.
Step 2: Call the Function
- Call the function providing values for all parameters.
- Call the function providing values for only some of the parameters, allowing the default values to be used for others.
- Call the function without providing any arguments, using all default values.
Practical Example
Example: Function with Default Arguments
Define Function:
- Create a function greet with two parameters: name and greeting, where greeting has a default value.
Call Function:
- Call greet with both arguments, with only the name argument, and with no arguments to see how default values are applied.
Practical Applications
Optional Parameters:
- Use default arguments to handle optional parameters, making your functions more versatile and easier to call with varying numbers of arguments.
Simplifying Function Calls:
- Reduce the complexity of function calls by providing reasonable default values, especially for parameters that are often the same.
Flexible APIs:
- Design more flexible APIs and interfaces, allowing users to override default behavior when necessary while maintaining simplicity for common cases.
Additional Resources
For more detailed information and a comprehensive guide on default arguments in Python, check out the full article on GeeksforGeeks: https://www.geeksforgeeks.org/default-arguments-in-python/ . This article provides in-depth explanations, examples, and further readings to help you master the use of default arguments in Python functions.
By the end of this video, you’ll have a solid understanding of default arguments in Python, enhancing your ability to write flexible and concise functions.
Read the full article for more details: https://www.geeksforgeeks.org/default-arguments-in-python/ .
Thank you for watching!

- Stack Overflow Public questions & answers
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Talent Build your employer brand
- Advertising Reach developers & technologists worldwide
- Labs The future of collective knowledge sharing
- About the company
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
TypeError: '<' not supported between instances of 'float' and 'list'
I'm working on a homework assignment, and I've gotten stuck on this error. These are the functions I'm using, and where the problem seems to be is in the predict function. The predict and TPR FPR score functions work as expected up until I go to call them within the roc_curve_computer function. Then I get the TypeError: '<' not supported between instances of 'float' and 'list' message. I've tried multiple different things, but I'm missing something, and I can't put my finger on it yet. The problem arises with the threshold variable going from being a single float early in the assignment to an array of floats at the end.
Ive tried a different way of doing the predict function but ran into a similar issue. Any help is appreciated.
- machine-learning
- 2 Welcome to Stack Overflow. Please include the full traceback error, though I would hazard a guess that the error is in this line: if probs < threshold: . probs is a float. threshold is a list. What exactly are you trying to do? Are you certain it isn't supposed to be thresh and not threshold ? – ewokx Commented 2 days ago
- remember indentation is significant. It appears when you call predict that neither of the arguments appear to be defined – Geoduck Commented 2 days ago
- This wasn't your question, but I wouldn't recommended using the same name twice in the for probs in probs statement. It works, but it's a little confusing. for prob in probs might be easier to read and understand. – Andrew Yim Commented yesterday
- shouldn't this predict(pred_probs_check, threshold) be predict(pred_probs_check[labels], threshold[labels]) , and TPRR_FPR_score(labels, preds_new) be TPR_FPR_score(labels[labels], preds_new[labels]) – ticktalk Commented yesterday
Know someone who can answer? Share a link to this question via email , Twitter , or Facebook .
Your answer.
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Browse other questions tagged python arrays list machine-learning or ask your own question .
- Featured on Meta
- Upcoming sign-up experiments related to tags
- The 2024 Developer Survey Is Live
- The return of Staging Ground to Stack Overflow
- Policy: Generative AI (e.g., ChatGPT) is banned
Hot Network Questions
- How would wyrms develop culture, houses, etc?
- does the 401k 12 month loan limit reset with a new plan
- Is this crumbling concrete step salvageable?
- Wrappers around write() and read() and a function to copy file permissions
- How do I make an access hole in a chain link fence for chickens?
- Does flanking apply to the defending creature or the attackers?
- In general, How's a computer science subject taught in Best Universities of the World that are not MIT level?
- Looking for a story that possibly started "MYOB"
- Which CAS can do basic non-commutative differential algebra?
- Can my grant pay for a conference marginally related to award?
- Was Croatia the first country to recognize the sovereignity of the USA? Was Croatia expecting military help from USA that didn't come?
- why arrow has break?
- How can one be a monergist and deny irresistible grace?
- Is there any way to play Runescape singleplayer?
- Where is the documentation for the new apt sources format used in 22.04?
- Create sublists whose totals exceed a certain threshold and that are as short as possible
- How can non-residents apply for rejsegaranti with Nordjyllands Trafikselskab?
- Starship IFT-4: whatever happened to the fin tip camera feed?
- Is there a name for books in which the narrator isn't the protagonist but someone who know them well?
- Why in ordinary linear regression is no global test for lack of model fit unless there are replicate observations at various settings of X?
- Statement of Contribution in Dissertation
- Words in my bookshelf
- How are secret encodings not a violation of amateur radio regulations?
- Error relinking cloned line items to the cloned sobject record
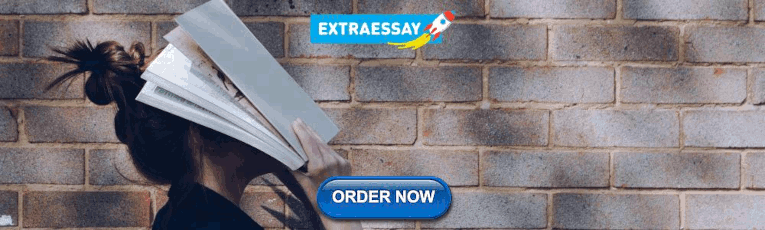
IMAGES
VIDEO
COMMENTS
Here, variable represents a generic Python variable, while expression represents any Python object that you can provide as a concrete value—also known as a literal—or an expression that evaluates to a value. To execute an assignment statement like the above, Python runs the following steps: Evaluate the right-hand expression to produce a concrete value or object.
x() is not the function, it's the call of the function. In python, functions are simply a type of variable, and can generally be used like any other variable. For example: def power_function(power): return lambda x : x**power power_function(3)(2) This returns 8. power_function is a function that
Assignment Operator. Assignment Operators are used to assign values to variables. This operator is used to assign the value of the right side of the expression to the left side operand. Python. # Assigning values using # Assignment Operator a = 3 b = 5 c = a + b # Output print(c) Output. 8.
Each new version of Python adds new features to the language. For Python 3.8, the biggest change is the addition of assignment expressions.Specifically, the := operator gives you a new syntax for assigning variables in the middle of expressions. This operator is colloquially known as the walrus operator.. This tutorial is an in-depth introduction to the walrus operator.
Implementation. Simply assign a function to the desired variable but without () i.e. just with the name of the function. If the variable is assigned with function along with the brackets (), None will be returned. Syntax: def func(): {. ..
Python Overview Python Built-in Functions Python String Methods Python List Methods Python Dictionary Methods Python Tuple Methods Python Set Methods Python File Methods Python Keywords Python Exceptions Python Glossary ... Python Assignment Operators. Assignment operators are used to assign values to variables: Operator Example Same As
Variables and Assignment¶. When programming, it is useful to be able to store information in variables. A variable is a string of characters and numbers associated with a piece of information. The assignment operator, denoted by the "=" symbol, is the operator that is used to assign values to variables in Python.The line x=1 takes the known value, 1, and assigns that value to the variable ...
For demonstration purposes, let's use a single variable, num.Initially, we set num to 6. We can apply all of these operators to num and update it accordingly.. Assignment. Assigning the value of 6 to num results in num being 6.. Expression: num = 6 Add and assign
And one very nice thing about the syntax for assignment operators is that it is nearly identical to a standard type of operator. So if you memorize the list of all the python operators then you're going to be able to use each one of these assignment operators quite easily.
Multiple- target assignment: x = y = 75. print(x, y) In this form, Python assigns a reference to the same object (the object which is rightmost) to all the target on the left. OUTPUT. 75 75. 7. Augmented assignment : The augmented assignment is a shorthand assignment that combines an expression and an assignment.
Since Python 3.6, in an async def function, ... assignment_expression::= [identifier ":="] expression. An assignment expression (sometimes also called a "named expression" or "walrus") assigns an expression to an identifier, while also returning the value of the expression.
Python Assignment Operator. The = (equal to) symbol is defined as assignment operator in Python. The value of Python expression on its right is assigned to a single variable on its left. The = symbol as in programming in general (and Python in particular) should not be confused with its usage in Mathematics, where it states that the expressions ...
Asking the User for Input#. The programs we have written so far accept no input from the user. To get data from the user through the Python prompt, we can use the built-in function input.. When input is called your whole program stops and waits for the user to enter the required data. Once the user types the value and presses Return or Enter, the function returns the input value as a string ...
Simple statements — Python 3.12.4 documentation. 7. Simple statements ¶. A simple statement is comprised within a single logical line. Several simple statements may occur on a single line separated by semicolons. The syntax for simple statements is: simple_stmt ::= expression_stmt. | assert_stmt.
The Python Operators are used to perform operations on values and variables. These are the special symbols that carry out arithmetic, logical, and bitwise computations. The value the operator operates on is known as the Operand. Here, we will cover Different Assignment operators in Python. Operators Sign Description SyntaxAssignment Operator = Assi
The print() function produces a more readable output, by omitting the enclosing quotes and by printing escaped and special characters: ... Simple assignment in Python never copies data. When you assign a list to a variable, the variable refers to the existing list. Any changes you make to the list through one variable will be seen through all ...
Description ¶. Adds a value and the variable and assigns the result to that variable.
JavaScript (JS) is a lightweight interpreted (or just-in-time compiled) programming language with first-class functions. While it is most well-known as the scripting language for Web pages, many non-browser environments also use it, such as Node.js, Apache CouchDB and Adobe Acrobat. JavaScript is a prototype-based, multi-paradigm, single-threaded, dynamic language, supporting object-oriented ...
Do you want to assign different return values from two different calls to your random function or a single value to two variables generated by a single call to the function. For the former, use tuple unpacking. ... Double assignment in python. 0. Python - assigning functions to variables. 2.
Default values are specified in the function definition using the assignment operator (=). 3. Function Call: When calling the function, if no argument is provided for a default parameter, the default value is used. ... examples, and further readings to help you master the use of default arguments in Python functions. By the end of this video ...
I suspect that upon calling a function, Python first establishes assignments of the local scope. Assignments then take higher precedence over or override inherited variables from the outer scopes. Thus without an assignment to s, the outer s is used. By contrast, with an assignment, s is redefined at the function call, and any reference before ...
For example, all variables assignable this way could be stored in a container of some kind maybe even in a separate module. No "tricks" would be required. After these warnings, here is an example. It can (re)assing any name in the current module. import sys this_module = sys.modules [__name__] def assign (name, value): setattr (this_module ...
The predict and TPR FPR score functions work as expected up until I go to call them within the roc_curve_computer function. Then I get the TypeError: '<' not supported between instances of 'float' and 'list' message.