inputFilePath1 = Program.RootPath + "\\" + "1.pptx"; String inputFilePath2 = Program.RootPath + "\\" + "2.pptx"; String inputFilePath3 = Program.RootPath + "\\" + "3.pptx"; String outputFilePath = Program.RootPath + "\\" + "Output.pptx"; String[] inputFilePaths = new String[3] { inputFilePath1, inputFilePath2, inputFilePath3 }; // Combine three PowerPoint files and output. PPTXDocument.CombineDocument(inputFilePaths, outputFilePath);
Append PowerPoint Document in C#
In addition, C# users can append a PowerPoint file to the end of a current PowerPoint document and combine to a single PowerPoint file.
String inputFilePath1 = Program.RootPath + "\\" + "1.pptx"; PPTXDocument doc1 = new PPTXDocument(inputFilePath1); // get XLSXDocument object from another file String inputFilePath2 = Program.RootPath + "\\" + "2.pptx"; PPTXDocument doc2 = new PPTXDocument(inputFilePath2); // append the 2nd document doc1.AppendDocument(doc2); // save the document String outputFilePath = Program.RootPath + "\\" + "Output.pptx"; doc1.Save(outputFilePath);
|
- GroupDocs.Total Product Family
- GroupDocs.Viewer Product Solution
- GroupDocs.Annotation Product Solution
- GroupDocs.Conversion Product Solution
- GroupDocs.Comparison Product Solution
- GroupDocs.Signature Product Solution
- GroupDocs.Assembly Product Solution
- GroupDocs.Metadata Product Solution
- GroupDocs.Search Product Solution
- GroupDocs.Parser Product Solution
- GroupDocs.Watermark Product Solution
- GroupDocs.Editor Product Solution
- GroupDocs.Merger Product Solution
- GroupDocs.Redaction Product Solution
- GroupDocs.Classification Product Solution
- Pricing Information
- Free Trials
- Temporary License
- My Orders & Quotes
- Renew an Order
- Upgrade an Order
- API Reference
- Code Samples
- Free Support
- Free Consulting
- Paid Support
- Paid Consulting
- Knowledge Base
- New Releases
- aspose.cloud
- groupdocs.com
- groupdocs.cloud
- groupdocs.app
- conholdate.com
- conholdate.cloud
- conholdate.app
- containerize.com
- codeporting.com
- fileformat.com
- fileformat.app
- Acquisition
- GroupDocs Documentation
- GroupDocs.Merger Product Family
- GroupDocs.Merger for .NET
- Developer Guide
- Merge files
- Merge PowerPoint Presentations
Merge PowerPoint Presentations Leave feedback
On this page.
You must be familiar with PPTX and PPT extension files, these are Presentation file formats that store collection of records to accommodate presentation data such as: slides, shapes, text, animations, video, audio and embedded objects. A presentation can be saved/converted into other file formats as well such as PDF, BMP, PNG, JPEG, and XPS.
Common Presentation file extensions and their associated file formats include PPTX , PPT , PPSX and PPS .
How to merge PPSX presentations
PPSX, Power Point Slide Show, file are created using Microsoft PowerPoint 2007 and above for Slide Show purpose. It is an update to the PPS file format that was supported by Microsoft PowerPoint 97-2003 versions. When a PPSX file is shared with another user and opened, it starts as PowerPoint show unlike PPTX file that opens in editable mode. The sequence of slide show is the same as in the original presentation. All the slides accompany the images, sounds and other embedded media accompany the presentation slides to the PPSX during the slideshow.
- Create an instance of Merger class and pass source PPSX file path as a constructor parameter. You may specify absolute or relative file path as per your requirements.
- Add another PPSX file to merge with Join method. Repeat this step for other PPSX documents you want to merge.
- Call Merger class Save method and specify the filename for the merged PPSX file as parameter.
NOTE: You may merge other Presentation formats like PPTX, PPT and PPS in the same way as shown above. For this provide files by specifying their names with extension.
How to merge PPTX streams
Please read the following article: How to correctly merge PPTX streams
Code Examples
Please find more use-cases and complete C# sources of our backend and frontend examples and try them for free!
Merge PowerPoint Live Demo
GroupDocs.Merger for .NET provides an online PowerPoint Merger App , which allows you to try it for free and check its quality and accuracy.
Was this page helpful?
Any additional feedback you'd like to share with us, please tell us how we can improve this page., thank you for your feedback.
We value your opinion. Your feedback will help us improve our documentation.
- Privacy Policy
- Terms of use
- Spire.Office for .NET
- Spire.OfficeViewer for .NET
- Spire.Doc for .NET
- Spire.DocViewer for .NET
- Spire.XLS for .NET
- Spire.Spreadsheet for .NET
- Spire.Presentation for .NET
- Spire.PDF for .NET
- Spire.PDFViewer for .NET
- Spire.PDFViewer for ASP.NET
- Spire.DataExport for .NET
- Spire.Barcode for .NET
- Spire.Email for .NET
- Spire.OCR for .NET
- Free Spire.Office for .NET
- Free Spire.Doc for .NET
- Free Spire.DocViewer for .NET
- Free Spire.XLS for .NET
- Free Spire.Presentation for .NET
- Free Spire.PDF for .NET
- Free Spire.PDFViewer for .NET
- Free Spire.PDFConverter for .NET
- Free Spire.DataExport for .NET
- Free Spire.Barcode for .NET
- Spire.Office for WPF
- Spire.Doc for WPF
- Spire.DocViewer for WPF
- Spire.XLS for WPF
- Spire.PDF for WPF
- Spire.PDFViewer for WPF
- Order Online
- Download Centre
- Temporary License
- Purchase Policies
- Renewal Policies
- Find A Reseller
- Purchase FAQS
- Support FAQs
- How to Apply License
- License Agreement
- Privacy Policy
- Customized Demo
- Code Samples
- Unsubscribe
- API Reference
- Spire.Doc for Java
- Spire.XLS for Java
- Spire.Presentation for Java
- Spire.PDF for Java
- Become Our Reseller
- Paid Support
- Our Customers
- Login/Register

- Python APIs
- Android APIs
- AI Products
- .NET Libraries
- Free Products
- Free Spire.Email for .NET
- WPF Libraries
- Java Libraries
- Spire.Office for Java
- Spire.Barcode for Java
- Spire.OCR for Java
- Free Spire.Office for Java
- Free Spire.Doc for Java
- Free Spire.XLS for Java
- Free Spire.Presentation for Java
- Free Spire.PDF for Java
- Free Spire.Barcode for Java
- C++ Libraries
- Spire.Office for C++
- Spire.Doc for C++
- Spire.XLS for C++
- Spire.PDF for C++
- Spire.Presentation for C++
- Spire.Barcode for C++
- Python Libraries
- Spire.Office for Python
- Spire.Doc for Python
- Spire.XLS for Python
- Spire.PDF for Python
- Spire.Presentation for Python
- Spire.Barcode for Python
- Android Libraries
- Spire.Office for Android via Java
- Spire.Doc for Android via Java
- Spire.XLS for Android via Java
- Spire.Presentation for Android via Java
- Spire.PDF for Android via Java
- Free Spire.Office for Android via Java
- Free Spire.Doc for Android via Java
- Free Spire.XLS for Android via Java
- Free Spire.Presentation for Android via Java
- Free Spire.PDF for Android via Java
- Cloud Libraries
- Spire.Cloud.Office
- Spire.Cloud.Word
- Spire.Cloud.Excel
- Swift Libraries
- Spire.XLS for Swift
- Spire.XLS AI for .NET
- Newsletter Subscribe Unsubscribe
- Spire.Presentation
- Spire.Barcode
- Spire.Email
- Spire.DocViewer
- Spire.PDFViewer
- Spire.SpreadSheet
- Spire.Cloud
- Spire.Doc for CPP
- Spire.XLS for CPP
- Spire.Presentation for CPP
- Spire.PDF for CPP
- Document Operation
- Page Background
- Image and Shape
- Header and Footer
- Program Guide for WPF
- Quick Guide
- Find and Replace
- Quick Start for .NET
- Quick Start for .NET Core
- Quick Start for .NET Standard
- Quick Start for WPF
- View Word Documents
- Data Import/Export
- Conditional Formatting
- Pivot Table
- Smart Marker
- Marker Designer
- Silverlight
- Getting Started
- Program Guide
- Paragraph and Text
- Set the properties of the PowerPoint
- C#/VB.NET: Set Background Color or Picture for PowerPoint Slides
- C#/VB.NET: Add or Delete Slides in PowerPoint
- C#: Change Slide Size in PowerPoint
- Remove Slides from a Presentation
- Save and Load PowerPoint to|from Stream
- Change the Slide Order
- Set Transitions for Powerpoint files
- Set the layout of the slide
- C#: Split PowerPoint Presentations
- C#: Copy Slides in PowerPoint Presentations
- Merge Selected Slides to a Single Presentation
- Mark a Presentation as Final
- Hide slide in PowerPoint document
- Loop a PowerPoint Presentation in C#, VB.NET
- Set the presentation show type as kiosk (full screen)
- Get the Titles of All Slides with C#
- Obtain object's sound effect in PowerPoint
- Apply a Slide Master to a Presentation in C#, VB.NET
- Create Multiple Slide Maters and Apply Them to Individual Slides
- Detect the used themes in PowerPoint in C#
- C#/VB.NET: Add or Remove Sections in PowerPoint
- Remove Section in PowerPoint in C#, VB.NET
- C#/VB.NET: Add Math Equations to PowerPoint using LaTeX Code
- C#/VB.NET: Create a PowerPoint Document
- Image and Shapes
- Audio and Video
- Comment and Note
- Program Guide Content
- Getting started
- Page Setting
- Extract/Read
- Attachments
- Interaction
- Document Settings
- View PDF Documents
- PDF Document Viewer
- Datatable Export
- ListView Export
- Create and Scan Barcode
- Send, Receive, Extract Emails
- Manipulate Folders
- Recognize Text
- Form Fields
Merge Selected Slides to a Single Presentation in C#, VB.NET
Slide cloning feature provided by Spire.Presentation enables developers to clone one or several slides within one PowerPoint presentation or among multiple presentations. By cloning slide from source presentation to target document, we can easily split a large presentation into small ones and merge multiple presentations to one presentation in reverse. This article presents how to merge selected slides from multiple PowerPoint presentations into one single presentation.
Step 1 : Create a new PowerPoint document and remove the default blank slide.
Step 2 : Initialize two instances of Presentation class and load the sample PowerPoint file respectively.
Step 3 : Append all slides in sample_01 to the new PowerPoint document using method Append(ISlide slide).
Step 4 : Append the second slide in sample_02 to the new presentation.
Step 5 : Save and launch the file.
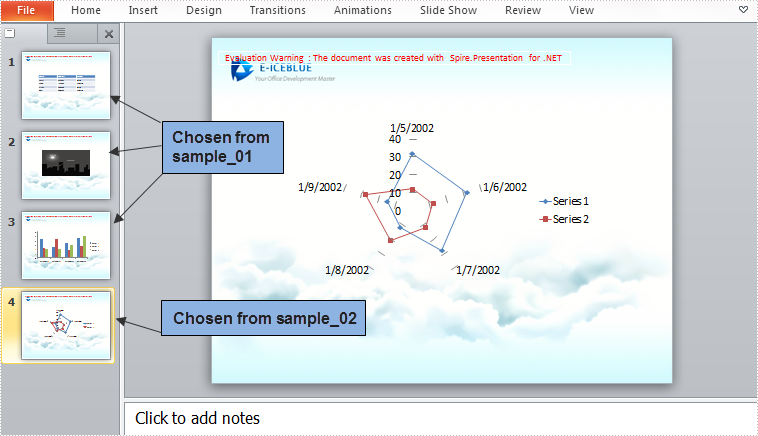
Entire Code
- Spire.Spreadsheet
- Purchase FAQs
- License Upgrade
- Believe The Users
- Our Service

How to merge or combine multiple PowerPoint files in C#, VB.NET
Syncfusion Essential Presentation is a .NET PowerPoint library used to create, open, read, edit, combine and convert PowerPoint presentations. The library supports combine/merge PowerPoint slides with below paste options equivalent to Microsoft PowerPoint.
- Keep Source Formatting – This implies that the slide will keep its original theme format by maintaining the theme of presentation you are copying from.
- Use Destination Theme - This will adapt the copied slide to match the destination theme by taking the theme of the destination presentation.
Steps to combine PowerPoint programmatically:
- Create a new C# console application.
- Install Syncfusion.Presentation.Base NuGet package to the console application project from nuget.org . For more information about adding a NuGet feed in Visual Studio and installing NuGet packages, refer to documentation
- Include the following namespace in the Program.cs file.
The following example demonstrates how to copy a PowerPoint slide from a PowerPoint presentation and paste it to another PowerPoint presentation.
You can download the working sample from Combine-PowerPoint.Zip.
Take a moment to peruse the documentation , where you can find options to combine the PowerPoint slides with code examples.
An online sample link to C ombine PowerPoint slides
Refer here to explore the rich set of Syncfusion Essential Presentation features.
Starting with v16.2.0.x, if you reference Syncfusion assemblies from trial setup or from the NuGet feed, include a license key in your projects. Refer to link to learn about generating and registering Syncfusion license key in your application to use the components without trail message.
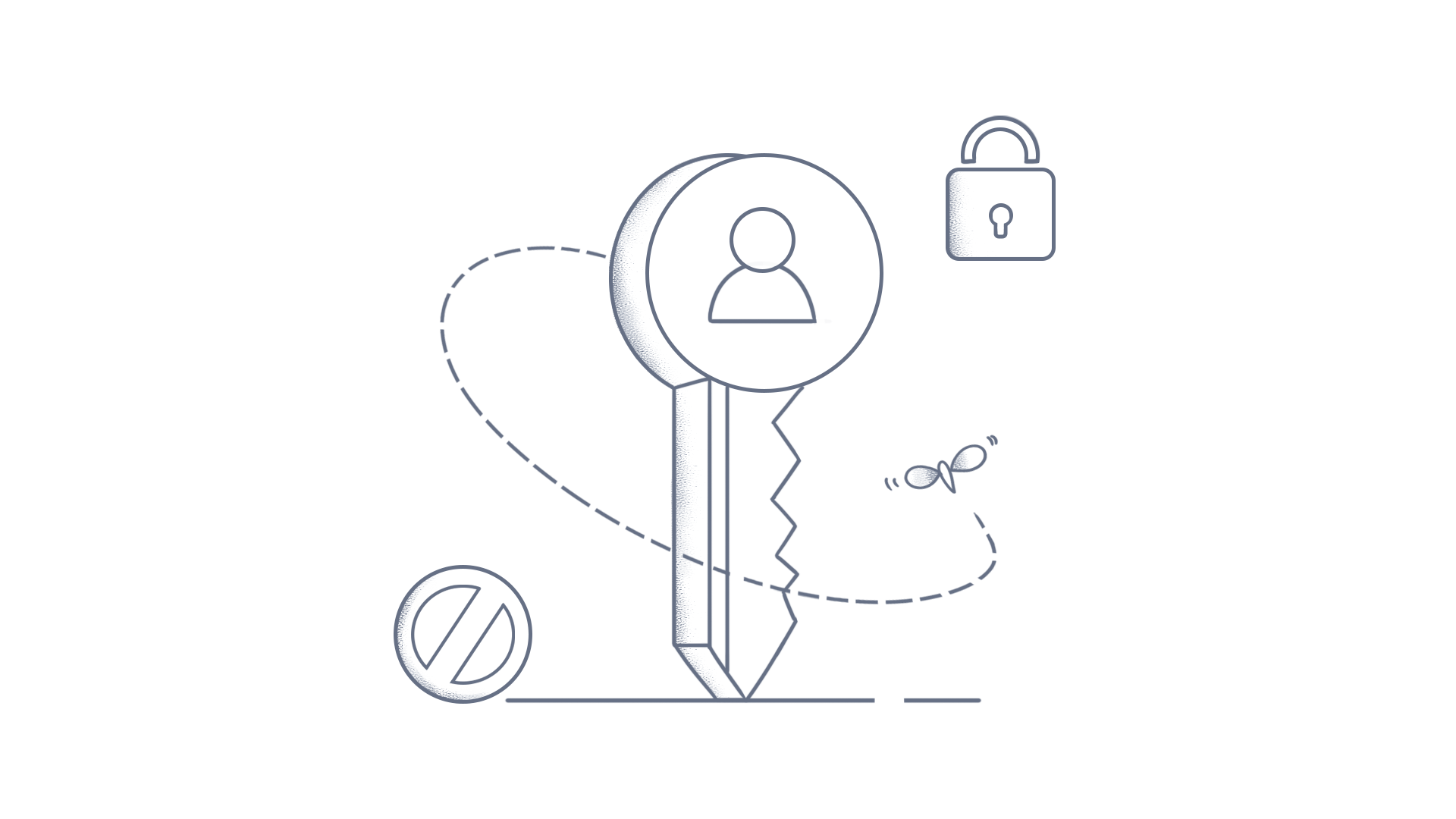
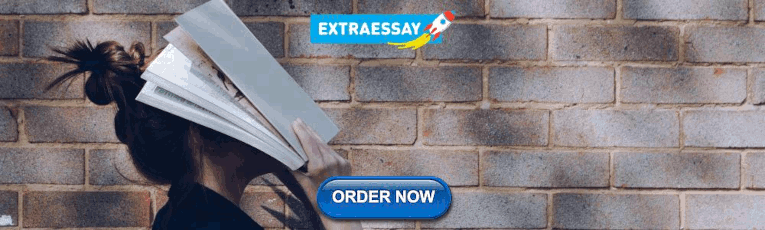
Access denied
- All Products
- Pricing Information
- Free Trials
- Temporary License
- My Orders & Quotes
- Renew an Order
- Upgrade an Order
- Paid Support
- Paid Consulting
- API Reference
- Code Samples
- Free Support
- Free Consulting
- Knowledge Base
- New Releases
- aspose.com aspose.cloud aspose.app aspose.ai
- groupdocs.com groupdocs.cloud groupdocs.app groupdocs.ai
- conholdate.com conholdate.cloud conholdate.app conholdate.ai
- Acquisition
Merge PowerPoint Presentations PPT, PPTX using C#
You may want to check out Aspose free online Merger app . It allows people to merge PowerPoint presentations in the same format (PPT to PPT, PPTX to PPTX, etc.) and merge presentations in different formats (PPT to PPTX, PPTX to ODP, etc.).

Presentation Merging
When you merge one presentation to another , you are effectively combining their slides in a single presentation to obtain one file.
Most presentation programs (PowerPoint or OpenOffice) lack functions that allow users to combine presentations in such manner.
Aspose.Slides for .NET , however, allows you merge to presentations in different ways. You get to merge presentations with all their shapes, styles, texts, formatting, comments, animations, etc. without having to worry about loss of quality or data.
Clone Slides .
What Can Be Merged
With Aspose.Slides, you can merge
- entire presentations. All the slides from the presentations end up in one presentation
- specific slides. Selected slides end up in one presentation
- presentations in one format (PPT to PPT, PPTX to PPTX, etc) and in different formats (PPT to PPTX, PPTX to ODP, etc) to one another.
Besides presentations, Aspose.Slides allows you to merge other files:
- Images , such as JPG to JPG or PNG to PNG
- Documents, such as PDF to PDF or HTML to HTML
- And two different files such as image to PDF or JPG to PDF or TIFF to PDF .
Merging Options
You can apply options that determine whether
- each slide in the output presentation retains a unique style
- a specific style is used for all the slides in the output presentation.
To merge presentations, Aspose.Slides provides AddClone methods (from the ISlideCollection interface). There are several implementations of the AddClone methods that define the presentation merging process parameters. Every Presentation object has a Slides collection, so you can call a AddClone method from the presentation to which you want to merge slides.
The AddClone method returns an ISlide object, which is a clone of the source slide. The slides in an output presentation are simply a copy of the slides from the source. Therefore, you can make changes the resulting slides (for example, apply styles or formatting options or layouts) without worrying about the source presentations becoming affected.
Merge Presentations
Aspose.Slides provides the AddClone (ISlide) method that allows you to combine slides while the slides retain their layouts and styles (default parameters).
This C# code shows you how to merge presentations:
Merge Presentations with Slide Master
Aspose.Slides provides the AddClone (ISlide, IMasterSlide, Boolean) method that allows you to combine slides while applying a slide master presentation template. This way, if necessary, you get to change the style for slides in the output presentation.
This code in C# demonstrates the described operation:
If you want the slides in the output presentation to have a different slide layout, use the AddClone (ISlide, ILayoutSlide) method instead when merging.
Merge Specific Slides From Presentations
This C# code shows you how to select and combine specific slides from different presentations to get one output presentation:
Merge Presentations With Slide Layout
This C# code shows you how to combine slides from presentations while applying your preferred slide layout to them to get one output presentation:
Merge Presentations With Different Slide Sizes
To merge 2 presentations with different slide sizes, you have to resize one of the presentations to make its size match that of the other presentation.
This sample code demonstrates the described operation:
Merge Slides to Presentation Section
This C# code shows you how to merge a specific slide to a section in a presentation:
The slide is added at the end of the section.
Subscribe to Aspose Product Updates
Get monthly newsletters & offers directly delivered to your mailbox.
© Aspose Pty Ltd 2001-2024. All Rights Reserved.
- Aspose.Slides
- .NET
- Merger
Merge PPT Formats in C#
Native and high performance ppt document merger using server-side aspose.slides for .net apis, without the use of any software like microsoft or open office, adobe pdf..
Aspose.Slides for .NET
Download from NuGet
Open NuGet package manager, search for and install. You may also use the following command from the Package Manager Console.
Merge PPT File Using C#
API which is a feature-rich, powerful and easy to use document manipulation and merging API for C# platform. Open
package manager, search for Aspose.Slides and install. You may also use the following command from the Package Manager Console.
Steps for Merging PPT Files in C#
A basic document merging and concatenating with Aspose.Slides for .NET APIs can be done with just few lines of code.
Load all the PPT files with full path.
Make one document as the base file
Call the relevant method for concatenating and merging files one by one.
Call the Save() method and pass the file name (full path) and format (PPT) as a parameter.
Now you can open and use the PPT file in Microsoft Office, Adobe PDF or any other compatible program.
System Requirements
Our APIs are supported on all major platforms and Operating Systems. Before executing the code below, please make sure that you have the following prerequisites on your system.
- Microsoft Windows or a compatible OS with .NET Framework, .NET Core, Windows Azure, Mono or Xamarin Platforms
- Development environment like Microsoft Visual Studio
- Aspose.Slides for .NET DLL referenced in your project - Install from NuGet using the Download button above
Merge PPT Files - C#
Merge pdf files online.
How to Merge PDF in Python
About Aspose.Slides for .NET API
Online ppt merger live demos, other supported merging formats.
Using C#, One can also merge many other file formats including.

- Latest Articles
- Top Articles
- Posting/Update Guidelines
- Article Help Forum

- View Unanswered Questions
- View All Questions
- View C# questions
- View C++ questions
- View Javascript questions
- View Visual Basic questions
- View .NET questions
- CodeProject.AI Server
- All Message Boards...
- Running a Business
- Sales / Marketing
- Collaboration / Beta Testing
- Work Issues
- Design and Architecture
- Artificial Intelligence
- Internet of Things
- ATL / WTL / STL
- Managed C++/CLI
- Objective-C and Swift
- System Admin
- Hosting and Servers
- Linux Programming
- .NET (Core and Framework)
- Visual Basic
- Web Development
- Site Bugs / Suggestions
- Spam and Abuse Watch
- Competitions
- The Insider Newsletter
- The Daily Build Newsletter
- Newsletter archive
- CodeProject Stuff
- Most Valuable Professionals
- The Lounge
- The CodeProject Blog
- Where I Am: Member Photos
- The Insider News
- The Weird & The Wonderful
- What is 'CodeProject'?
- General FAQ
- Ask a Question
- Bugs and Suggestions
Programmatically Merging Powerpoint Presentations in asp.net

Add your solution here
| |
Your Email | | ? | Optional Password | | - Read the question carefully.
- Understand that English isn't everyone's first language so be lenient of bad spelling and grammar.
- If a question is poorly phrased then either ask for clarification, ignore it, or edit the question and fix the problem. Insults are not welcome.
This content, along with any associated source code and files, is licensed under The Code Project Open License (CPOL)  Top Experts | Last 24hrs | This month | | 40 | | 30 | | 20 | | 15 | | 10 |  C# .NET PowerPoint API for processing presentation file formatsSupercharge your .net presentations by developing apps to generate, process, and manipulate microsoft powerpoint slides. manage different presentation file formats including ppt, pptx, pot, potx, pps, ppsx, and odp. convert powerpoint documents to pdf, html, raster images, and svg vectors., manipulate powerpoint slides using the .net presentation api. Aspose.Slides for .NET is a full-featured and flexible .NET PowerPoint API enabling software and app developers to programmatically generate, modify, manipulate, and export Microsoft PowerPoint presentations in the .NET platform. It supports the processing of various popular PowerPoint file formats such as PPT, POT, PPTX, PPS, POTX, and PPTM as well as OpenOffice (ODP) documents. Programmers can utilize the advanced formatting and presentation processing capabilities of this .NET library to add, remove, split, or merge presentation slides, apply text formatting, manage animations and slide transitions, work with images, shapes, tables, and charts, and do so much more. Boasting an intricate rendering engine, Aspose.Slides for .NET API helps you in immaculately converting PowerPoint presentations to multiple file formats such as PowerPoint to PDF, PowerPoint to JPG, and PowerPoint to HTML. Getting StartedBefore setting up the .NET PowerPoint presentations API, please check the System Requirements page for the prerequisites to help you install the API without any issues. Aspose.Slides for .NET InstallationYou can directly download the DLLs or the MSI installer of Aspose.Slides for .NET by visiting the downloads section . You can also use the NuGet package for installing the .NET PowerPoint API. The command for setting up the library in the package manager console is given below: How to Convert PowerPoint to PDF in .NET and Quickly Merge PresentationsAspose.Slides for .NET is the leading API for manipulating PowerPoint presentations that includes a great feature set with the ability to convert presentations. Seamlessly convert PowerPoint to PDF and other file formats in .NET and merge presentation slides using the .NET PowerPoint API. Convert PowerPoint to PDF in .NETPerform immaculate PowerPoint presentations to PDF conversions in C# .NET using Aspose.Slides for .NET API while setting different properties of the resultant PDF document in the process. Please refer to the following steps and the sample coding for converting PowerPoint PPT to PDF in the .NET platform: - Create an instance of the Presentation class object to load the source presentation file.
- Initialize the PdfOptions class object to set the desired image options.
- Set the desired PDF options for the resultant PDF file.
- Convert the presentation to PDF format using the Save method.
Merge Presentations in .NETMerging presentations into a unified PowerPoint document is a prominent feature of Aspose.Slides for .NET API. Please check the below-given steps and information to quickly and easily combine multiple PowerPoint PPTX presentations within your document merger apps using the .NET presentations API: - Create an instance of the Presentation class to load the source presentation.
- Initialize separate Presentation class objects for the required target presentations.
- In a loop, iterate through all the slides in the target presentations.
- Call the AddClone() method of the slides collection of the source presentation where other presentations are to be combined.
- Save the resultant presentation having all the slides from the target presentations.
More working examples and complete coding samples of Aspose.Slides for .NET API are available on the GitHub Examples page. Please check out the free online apps of Aspose.Slides that let you view, convert, parse, compare, watermark, redact, split, and edit PowerPoint presentation files from anywhere using your mobile or desktop devices. Secure and independent .NET presentation APIWith Aspose.Slides for .NET, you have access to some excellent security features allowing for dependable presentation processing in .NET. This includes the ability to password-protect the presentations and support for read-only mode. Additionally, installation of Microsoft PowerPoint is not needed while using the .NET presentation API giving you a fully independent and unique user experience. 1. How can I convert PowerPoint files in .NET?Converting Microsoft PowerPoint files in .NET is quick and easy using Aspose.Slides for .NET API. Only a few lines of .NET coding are needed to convert PPT, PPTX, PPS, PPSX, POT, POTX, PPTM, and ODP files to different file formats. 2. How long it takes to merge or convert PowerPoint slides?The .NET API for manipulating and processing presentation slides works fast and completes your presentation files conversion and merger requests in no time. 3. Is it safe to process presentations using the .NET API?You can be sure of the security of your PowerPoint presentation files while processing them using Aspose.Slides for .NET API. We ensure the privacy of your data and take all required measures to provide you with a secure user experience. 4. Can I process PowerPoint presentations on Mac OS, Windows, or Linux?Yes, the PowerPoint presentations processing API for .NET works across different OS, frameworks, and operating environments. You can use it on the platform of your choice and it does not require any additional software installation to function. PowerPoint Presentations Manipulation ToolsLooking for help. Checkout our support channels for help with your questions related to Aspose product API features and working. - Convert PPT to Video in C#
- Encrypt PowerPoint in C#
- Convert JPG to PPT in C# .NET
Documentation- Aspose.Slides for .NET Features
- Install Aspose.Slides for .NET NuGet Package
- Aspose.Slides for .NET Paid Support Helpdesk
Knowledge Base- How to Merge Slides in C#
- How to Insert Signature in PowerPoint using C#
- How to Create a Table in PowerPoint using C#
Ready to get started?Instantly share code, notes, and snippets.  aspose-com-gists / merge-powerpoint-presentation-selected-slides.cs- Download ZIP
- Star ( 0 ) 0 You must be signed in to star a gist
- Fork ( 0 ) 0 You must be signed in to fork a gist
- Embed Embed this gist in your website.
- Share Copy sharable link for this gist.
- Clone via HTTPS Clone using the web URL.
- Learn more about clone URLs
- Save aspose-com-gists/02b8b90a3b1b53e565530346be710916 to your computer and use it in GitHub Desktop.
| // Instantiate a Presentation object that represents a target presentation file | | using (Presentation presentation1 = new Presentation("presentation1.pptx")) | | { | | // Instantiate a Presentation object that represents a source presentation file | | using (Presentation presentation2 = new Presentation("presentation2.pptx")) | | { | | // Merge only even slides of presentation2 (first slide is at 0 index) | | for (int i = 1; i <= presentation2.Slides.Count; i = i + 2) | | { | | presentation1.Slides.AddClone(presentation2.Slides[i]); | | } | | } | | presentation1.Save("merged-presentation-even.pptx", Export.SaveFormat.Pptx); | | } | | // Instantiate a Presentation object that represents a target presentation file | | using (Presentation presentation1 = new Presentation("presentation1.pptx")) | | { | | // Instantiate a Presentation object that represents a source presentation file | | using (Presentation presentation2 = new Presentation("presentation2.pptx")) | | { | | // Merge first two slides only using slide master | | presentation1.Slides.AddClone(presentation2.Slides[0], presentation1.Masters[0], true); | | presentation1.Slides.AddClone(presentation2.Slides[1], presentation1.Masters[0], true); | | } | | presentation1.Save("merged-presentation-master.pptx", Export.SaveFormat.Pptx); | | } | | // Instantiate a Presentation object that represents a target presentation file | | using (Presentation presentation1 = new Presentation("presentation1.pptx")) | | { | | // Instantiate a Presentation object that represents a source presentation file | | using (Presentation presentation2 = new Presentation("presentation2.pptx")) | | { | | foreach (ISlide slide in presentation2.Slides) | | { | | // Merge slides from source to target | | presentation1.Slides.AddClone(slide); | | } | | } | | // Save the presentation | | presentation1.Save("merged-presentation.pptx", Export.SaveFormat.Pptx); | | } | - Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack OverflowFind centralized, trusted content and collaborate around the technologies you use most. Q&A for work Connect and share knowledge within a single location that is structured and easy to search. Get early access and see previews of new features. Merge PowerPoint Files in SharePointi want to merge 2 PowerPoint Files/Slides which are hosted within a SharePoint Env. It has to be done on the Server Side. I also found some Solutions, but all of them need Namespaces which arnt available for me: DocumentFormat.OpenXML // Has to be installed on the Server to be accessable. Impossible :S Microsoft.Office.Interop.PowerPoint // Even Office PP has to be installed on the Server, a absolute nogo. Does someone know another NameSpace which gives me the required functionality to merge two PowerPoint Slides? For example, in SharePoint you are able to "View" PPT files within the Browser, there is even a sp-service for this, but i did not find any information how to contact it and maybe do the merge over it. Thanks for any Help in advance :) Best Regards THO  The problem you are having is with the requirement to do this "server side". SharePoint is merely the storage, you should be able to use a server that allows you to install the required Office document manipulation tools and simply read the documents from the SharePoint server and web services. Even "server side" your code is going to consist of the following steps: - Download ppt files
- Merge ppt files
- Upload merged file to SharePoint
 Your AnswerReminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more Sign up or log inPost as a guest. Required, but never shown By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy . Not the answer you're looking for? Browse other questions tagged c# sharepoint powerpoint or ask your own question .- The Overflow Blog
- Where does Postgres fit in a world of GenAI and vector databases?
- Featured on Meta
- We've made changes to our Terms of Service & Privacy Policy - July 2024
- Bringing clarity to status tag usage on meta sites
- What does a new user need in a homepage experience on Stack Overflow?
- Feedback requested: How do you use tag hover descriptions for curating and do...
- Staging Ground Reviewer Motivation
Hot Network Questions- Optimal Bath Fan Location
- A very interesting food chain
- Do metal objects attract lightning?
- Do the amplitude and frequency of gravitational waves emitted by binary stars change as the stars get closer together?
- Is this a new result about hexagon?
- Could someone tell me what this part of an A320 is called in English?
- Does Vexing Bauble counter taxed 0 mana spells?
- How much payload could the Falcon 9 send to geostationary orbit?
- The answer is not wrong
- Using Thin Lens Equation to find how far 1972 Blue Marble photo was taken
- Where to donate foreign-language academic books?
- How can these humans cross the ocean(s) at the first possible chance?
- Completely introduce your friends
- How much missing data is too much (part 2)? statistical power, effective sample size
- Parody of Fables About Authenticity
- How does the summoned monster know who is my enemy?
- How would you say a couple of letters (as in mail) if they're not necessarily letters?
- Can I use a JFET if its drain current exceeds the Saturation Drain Current from the datasheet (or is my JFET faulty)?
- Using conditionals within \tl_put_right from latex3 explsyntax
- What prevents a browser from saving and tracking passwords entered to a site?
- Why is PUT Request not allowed by default in OWASP CoreRuleSet
- Is Intuition Indispensable in Mathematics?
- Worth replacing greenboard for shower wall
- How can I delete a column from several CSV files?
 PowerPoint is not printing correctly on Windows 11/101] test printing in another software, 2] reinstall the printer driver, 3] export the powerpoint presentation as a pdf, 4] change the page layout, 5] run sfc scan, 6] update or repair microsoft office, why is my powerpoint not printing correctly, how do i repair powerpoint in windows 11, nishantgola@twc. 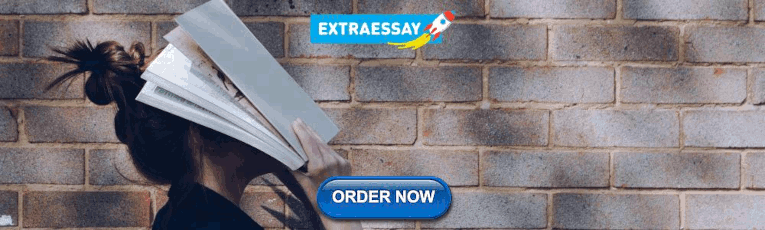 |
COMMENTS
How can I merge several presentations into one presentation? c#; powerpoint; gembox-presentation; Share. Improve this question. Follow edited Mar 31, 2020 at 2:59. Mario Z. 4,383 2 2 gold badges 26 26 silver badges 39 39 bronze badges. asked Mar 31, 2020 at 2:52.
Combining two or more presentations into a single one is just a few lines of code away. This article discusses different ways how to merge multiple PowerPoint presentations into a single one using C#.Further, it will also show how you can join specific slides of different presentations using a similar C# code.. The following topics are covered in this article:
Now the presentations are merged. Click on the Converted PDF button. Select a location for the converted PDF and give it a name that ends with .pdf and click on the Save button. Click on the Save as PDF button. Now the merged presentation has been converted to PDF. Following images show the merged presentation and converted PDF.
Merge PowerPoint Presentations in C## In this section, you will learn how to clone and merge all the slides from one PowerPoint presentation to another. For this, you can simply clone the slides from the source presentation and add them at the end of the target presentation. The following are the steps to merge two presentations.
How to merge two PowerPoint presentations in C#. Install NuGet package: Install the Syncfusion.Presentation.Net.Core NuGet package in your project. Open source and destination file: Initialize two IPresentation objects for the passing the FileStream objects. Clone slides from source presentation: Use the Clone method of the ISlide interface to ...
Merge PowerPoint Presentations using C#. Let's follow the steps given below to initiate the concatenation of Microsoft PowerPoint using C# .NET. First, we need to create an object of the Configuration class. Secondly, set client credentials to a Configuration instance. Thirdly, create an object of SlidesApi while passing the configuration ...
How to Combine PowerPoint Presentations in C# using Slide Range; C# REST API to Merge PowerPoint PPTs and SDK Installation# In order to merge PowerPoint files, I will be using .NET SDK of GroupDocs.Merger Cloud API. It is a secure, reliable and high-performance Cloud SDK to merge several documents into one and to split a single file into ...
Combine and Merge Multiple PowerPoint Files into One Using C#. This part illustrates how to combine three PowerPoint files into a new file in C# application. You may also combine more PowerPoint documents together. String inputFilePath1 = Program.RootPath + "\\" + "1.pptx" ; String inputFilePath2 = Program.RootPath + "\\" + "2.pptx" ;
Repeat this step for other PPSX documents you want to merge. Call Merger class Save method and specify the filename for the merged PPSX file as parameter. NOTE: You may merge other Presentation formats like PPTX, PPT and PPS in the same way as shown above. For this provide files by specifying their names with extension.
Step 1: Create a new PowerPoint document and remove the default blank slide. Step 2: Initialize two instances of Presentation class and load the sample PowerPoint file respectively. Step 3: Append all slides in sample_01 to the new PowerPoint document using method Append (ISlide slide). Step 4: Append the second slide in sample_02 to the new ...
Steps to combine PowerPoint programmatically: Create a new C# console application. Install Syncfusion.Presentation.Base NuGet package to the console application project from nuget.org. For more information about adding a NuGet feed in Visual Studio and installing NuGet packages, refer to documentation.
Merge PowerPoint Presentations using C# Raw. readme.md Related Article(s): Merge PowerPoint Presentations & Slides using C#; Raw. MergePresentations.cs This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
C#. ASP.NET. I am trying myself in coding at the moment very new to this and I have a small project at the moment where I have a website which has stored a few slides on a server and I want the user to be able to select some slides and those slides should be merged into one presentation. So many powerpoint files which contain 1 slide and I want ...
Info. Most presentation programs (PowerPoint or OpenOffice) lack functions that allow users to combine presentations in such manner. Aspose.Slides for .NET, however, allows you merge to presentations in different ways.You get to merge presentations with all their shapes, styles, texts, formatting, comments, animations, etc. without having to worry about loss of quality or data.
Steps for Merging PPT Files in C#. A basic document merging and concatenating with Aspose.Slides for .NET APIs can be done with just few lines of code. Load all the PPT files with full path. Call the relevant method for concatenating and merging files one by one. Call the Save () method and pass the file name (full path) and format (PPT) as a ...
programmatically how to use the excel chart or excel data linked to powerpoint presentation using vsto in c# code How to merge two powerpoint files slides into one presentation using JSOM, REST API in sharepoint online
Merge Presentations in .NET. Merging presentations into a unified PowerPoint document is a prominent feature of Aspose.Slides for .NET API. Please check the below-given steps and information to quickly and easily combine multiple PowerPoint PPTX presentations within your document merger apps using the .NET presentations API:
I want to merge multiple PointPoint decks, the merging works fine when every deck contains 30 or less slides, If any deck contains more than 30 slides, PowerPoint issues a Repair message. ... Using OpenXML to save a PowerPoint presentation as a PDF. ... Generating PowerPoint files in C# with OpenXML SDK; Validates, but opens with errors. 5 ...
Merge PowerPoint Presentations using C# Raw. merge-powerpoint-presentation-selected-slides.cs This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters. Learn more about bidirectional Unicode characters ...
0. i want to merge 2 PowerPoint Files/Slides which are hosted within a SharePoint Env. It has to be done on the Server Side. I also found some Solutions, but all of them need Namespaces which arnt available for me: DocumentFormat.OpenXML // Has to be installed on the Server to be accessable.
To export a PowerPoint presentation as a PDF, open the presentation and go to File > Export. Now, select the Create PDF/XPS Document option from the left side and then click on the Create PDF/XPS ...