Latest Courses

We provides tutorials and interview questions of all technology like java tutorial, android, java frameworks
Contact info
G-13, 2nd Floor, Sec-3, Noida, UP, 201301, India
[email protected] .

Latest Post
PRIVACY POLICY
Online Compiler
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Concatante PHP variables inside loop by a "Concatenating Assignment Operator", and Echo each output loop separately
With the "Concatenating Assignment Operator" I assigned in the loop two variables. I need to get each loop result separately. The problem is that I don't know why each next loop result is copied to each next loop result.
Using this code, I get the output:
I'm working on making the output look like this:

- 7 You need to reset $output at the top of the loop. – aynber Commented Dec 1, 2021 at 14:14
You are always concatenating values to the $output, you never clear it, so the numbers are just continually added. All you need to do is change the first $output .= "1"; in to a $output = "1"; and that will have the effect of resetting $output to the one character ready to be concatenated with the second.

Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged php arrays while-loop or ask your own question .
- The Overflow Blog
- Masked self-attention: How LLMs learn relationships between tokens
- Deedy Das: from coding at Meta, to search at Google, to investing with Anthropic
- Featured on Meta
- User activation: Learnings and opportunities
- Preventing unauthorized automated access to the network
- Feedback Requested: How do you use the tagged questions page?
Hot Network Questions
- Does history possess the epistemological tools to establish the occurrence of an anomaly in the past that defies current scientific models?
- Why do evacuations result in so many injuries?
- Is there an error in the dissipation calculation of a mosfet?
- When does derived tensor product commute with arbitrary products?
- What is a "derivative security"?
- what is the proper order for three verbs at the end of a sentence when there is no ersatz infinitive?
- Can you find a grand tour of the rooms in this 12x12 grid?
- How can I draw the intersection of a plane with a dome tent?
- In big band horn parts, should I write double flats (sharps) or the enharmonic equivalent?
- How do you measure exactly 31 minutes by burning the ropes?
- Are logic and mathematics the only fields in which certainty (proof) can be obtained?
- Do mathematicians care about the validity ("truth") of the axioms?
- Why is my Lenovo ThinkPad running Ubuntu using the e1000e Ethernet driver?
- How to jointly estimate range and delay of a target?
- On a glassed landmass, how long would it take for plants to grow?
- Estimating an upper bound of hyperbolicity constants in Gromov-hyperbolic groups
- Code editor-script console missing
- Story where the main character is hired as a FORTH interpreter. We pull back and realise he is a computer program living in a circuit board
- How can I make second argument path relative to the first on a command?
- Onto group actions and symmetries.
- Is it ethical to edit grammar, spelling, and wording errors in survey questions after the survey has been administered, prior to publication?
- What is an "axiomatic definition"? Every definition is an axiomatic definition?
- How to Organise/Present Built Worlds?
- Easily unload gravel from pickup truck

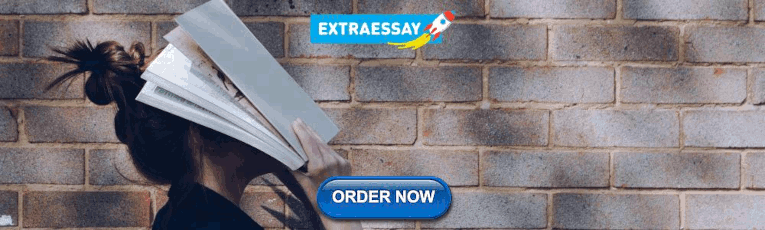
COMMENTS
There are two string operators. The first is the concatenation operator ('.'), which returns the concatenation of its right and left arguments. The second is the concatenating assignment operator ('.= '), which appends the argument on the right side to the argument on the left side. Please read Assignment Operators for more information.
PHP Compensation Operator is used to combine character strings. Operator. Description. . The PHP concatenation operator (.) is used to combine two string values to create one string. .=. Concatenation assignment.
W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more.
In this article, we will concatenate two strings in PHP. There are two string operators. The first is the concatenation operator ('.'), which returns the concatenation of its right and left arguments. The second is the concatenating assignment operator ('.='), which appends the argument on the right side to the argument on the left side ...
Here are some more advanced examples demonstrating the use of both the concatenation operator (.) and the concatenation assignment operator (.=) in PHP: ... while the concatenation assignment operator (.=) provides a convenient means of appending content to existing strings. This versatility is demonstrated through various examples, including ...
Assignment Operators. The basic assignment operator is "=". Your first inclination might be to think of this as "equal to". Don't. It really means that the left operand gets set to the value of the expression on the right (that is, "gets set to").
The concatenation assignment operator .= in PHP simplifies the process of appending one string to another by modifying the original string directly. This operator is especially useful in scenarios where a string needs to be built incrementally, such as in loops or when constructing complex messages dynamically.
In this article, we will concatenate two strings in PHP. There are two string operators. The first is the concatenation operator ('.'), which returns the concatenation of its right and left arguments. The second is the concatenating assignment operator ('.='), which appends the argument on the right side to the argument on the left side. Examples:
Use the Concatenation Assignment Operator to Concatenate Strings in PHP. In PHP, we can also use the concatenation assignment operator to concatenate strings. The concatenation assignment operator is .=. The difference between .= and . is that the concatenation assignment operator .= appends the string on the right side. The correct syntax to ...
String concatenation refers to joining two or more strings to create a new string. It is a typical and helpful operation in PHP, allowing developers to create customized and dynamic strings by combining different sources. The dot (.) operator performs this operation in PHP by joining the strings and creating a new string.
Use PHP assignment operator (=) to assign a value to a variable. The assignment expression returns the value assigned. Use arithmetic assignment operators to carry arithmetic operations and assign at the same time. Use concatenation assignment operator (.=)to concatenate strings and assign the result to a variable in a single statement.
Two string operands are concatenated returning the updated contents of string on the left side −. It will produce the following output −. PHP - String Operators - There are two operators in PHP for working with string data types: concatenation operator (.) and the concatenation assignment operator (.=). Read this chapter to learn how ...
3. Only slightly, since PHP has to parse the entire string looking for variables, while with concatenation, it just slaps the two variables together. So there's a tiny performance hit, but it's not noticeable for most things. It's a lot easier to concatenate variables like $_SERVER['DOCUMENT_ROOT'] using the concatenation operator (with quotes ...
There are two String operators in PHP. 1. Concatenation Operator "." (dot) 2. Concatenation Assignment Operator ".=" (dot equals) Concatenation Operator Concatenation is the operation of joining two character strings/variables together. In PHP we use . (dot) to join two strings or variables. Below are some examples of string concatenation:
PHP » Operators » .= Syntax: $var .= expressionvarA variable.expressionA value to concatenate the variable with.Concatenation assignment operator.
PHP Assignment Operators. The PHP assignment operators are used with numeric values to write a value to a variable. The basic assignment operator in PHP is "=". It means that the left operand gets set to the value of the assignment expression on the right.
There are two types of string operators provided by PHP. 1. Concatenation Operator ("."): In PHP, this operator is used to combine the two string values and returns it as a new string. Let's take the various examples of how to use the Concatenation Operator (".") to concatenate the strings in PHP. Example 1: <!
No way to do this with concatenation assignment operator. Share. Improve this answer. Follow answered Jul 13, 2015 at 12:29. Jordi Martín Jordi Martín. 519 4 4 silver ... Concatenation-assignment in PHP. 0. PHP Concatenation assignment. 0. PHP - Concatenate text to 2 variables in 1 operation. 1.
With the "Concatenating Assignment Operator" I assigned in the loop two variables. I need to get each loop result separately. The problem is that I don't know why each next loop result is ... PHP 'foreach' array concatenate echo. 0. How to print multiple variables values using loop. 0. PHP Combine arrays in a loop. 1. Concatenate array on loop ...