- C++ Data Types
- C++ Input/Output
- C++ Pointers
- C++ Interview Questions
- C++ Programs
- C++ Cheatsheet
- C++ Projects
- C++ Exception Handling
- C++ Memory Management
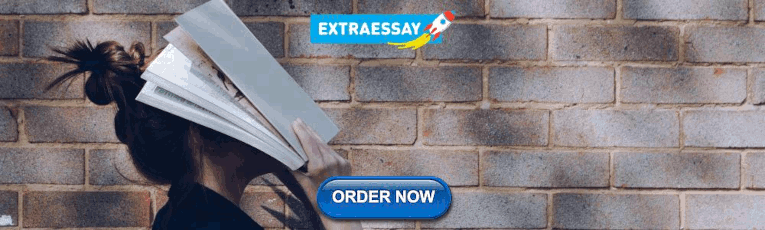
Left Shift and Right Shift Operators in C/C++
In C/C++, left shift (<<) and right shift (>>) operators are binary bitwise operators that are used to shift the bits either left or right of the first operand by the number of positions specified by the second operand allowing efficient data manipulation. In this article, we will learn about the left shift and right shift operators.
Let’s take a look at an example:
Left Shift (<<) Operators
The left shift(<<) is a binary operator that takes two numbers, left shifts the bits of the first operand, and the second operand decides the number of places to shift. In other words, left-shifting an integer “ a ” with an integer “ b ” denoted as ‘ (a<<b)’ is equivalent to multiplying a with 2^b (2 raised to power b).
- a is the integer value to be shifted.
- b specifies how many positions to shift the bits.
Example: Let’s take a=21 ; which is 10101 in Binary Form. Now, if “ a is left-shifted by 1 ” i.e a = a << 1 then a will become a = a * ( 2 ^ 1) . Thus, a = 21 * (2 ^ 1) = 42 which can be written as 10100.
But if the size of the data type of a is only 5 bits, then the first bit will be discarded we will be left with a = 10, which is 01010 in binary. It is shown in the below image.
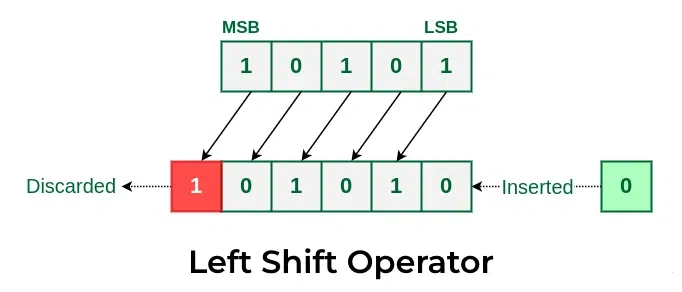
Example of Left Shift Operator
Applications of left shift operator.
- Multiplication by Powers of Two : Left shifting a number by n positions is equivalent to multiplying it by 2^n and is much faster than normal multiplication
- Efficient Calculations : Used in performance-critical applications where arithmetic operations need to be fast.
- Bit Manipulation : Common in low-level programming, such as embedded systems and hardware interfacing.
To gain a deeper understanding of bitwise operations and how they improve performance, our Complete C++ Course offers detailed tutorials on using operators efficiently in C++.
Right Shift(>>) Operators
Right Shift(>>) is a binary operator that takes two numbers, right shifts the bits of the first operand, and the second operand decides the number of places to shift. In other words, right-shifting an integer “ a ” with an integer “ b ” denoted as ‘ (a>>b) ‘ is equivalent to dividing a with 2^b.
Example: Let’s take a=21 ; which is 10101 in Binary Form. Now, if a is right shifted by 1 i.e a = a >> 1 then a will become a=a/(2^1) . Thus, a = a/(2^1) = 10 which can be written as 1010 .
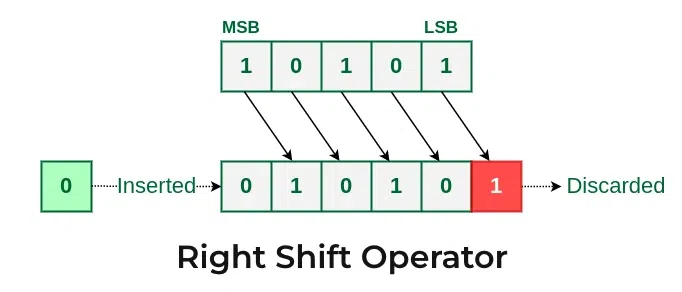
Example of Right Shift Operator
Applications of right shift operators.
- Division by Powers of Two : Right shifting a number by n positions is equivalent to dividing it by 2^n and it is very fast.
- Efficient Calculations : Used in performance-critical applications for fast division operations.
- Bit Manipulation : Useful in extracting specific bits from data, common in data compression and cryptography.
Important Points of Shift Operators
1. The left-shift and right-shift operators should not be used for negative numbers. The result of is undefined behavior if any of the operands is a negative number. For example, results of both 1 >> -1 and 1 << -1 is undefined.
2. If the number is shifted more than the size of the integer, the behavior is undefined. For example, 1 << 33 is undefined if integers are stored using 32 bits. For bit shift of larger values 1ULL<<62 ULL is used for Unsigned Long Long which is defined using 64 bits that can store large values.
3. The left-shift by 1 and right-shift by 1 are equivalent to the product of the first term and 2 to the power given element(1<<3 = 1*pow(2,3)) and division of the first term and second term raised to power 2 (1>>3 = 1/pow(2,3)) respectively.
Must Read: Bitwise Operators in C/C++
Similar Reads
- Left Shift and Right Shift Operators in C/C++ In C/C++, left shift (<<) and right shift (>>) operators are binary bitwise operators that are used to shift the bits either left or right of the first operand by the number of positions specified by the second operand allowing efficient data manipulation. In this article, we will learn 7 min read
- Result of comma operator as l-value in C and C++ Using the result of the comma operator as l-value is not valid in C. But in C++, the result of the comma operator can be used as l-value if the right operand of the comma operator is l-value. For example, if we compile the following program as a C++ program, then it works and prints b = 30. And if w 1 min read
- set operator= in C++ STL The ‘=’ is an operator in C++ STL which copies (or moves) a set to another set and set::operator= is the corresponding operator function. There are three versions of this function: The first version takes reference of an set as an argument and copies it to an set. Syntax: ums1.operator=(set &set 2 min read
- list::operator= in C++ STL Lists are containers used in C++ to store data in a non contiguous fashion, Normally, Arrays and Vectors are contiguous in nature, therefore the insertion and deletion operations are costlier as compared to the insertion and deletion option in Lists. list::operator= This operator is used to assign n 2 min read
- Increment (++) and Decrement (--) Operator Overloading in C++ Operator overloading is a feature in object-oriented programming which allows a programmer to redefine a built-in operator to work with user-defined data types. Why Operator Overloading? Let's say we have defined a class Integer for handling operations on integers. We can have functions add(), subtr 4 min read
- Types of Operator Overloading in C++ C++ provides a special function to change the current functionality of some operators within its class which is often called as operator overloading. Operator Overloading is the method by which we can change some specific operators' functions to do different tasks. Syntax: Return_Type classname :: o 4 min read
- C++ Increment and Decrement Operators Prerequisite: Operators in C++ What is a C++ increment Operator? The C++ increment operator is a unary operator. The symbol used to represent the increment operator is (++). The increment operator increases the value stored by the variable by 1. This operator is used for Numeric values only. There a 4 min read
- Overloading Subscript or array index operator [] in C++ The Subscript or Array Index Operator is denoted by '[]'. This operator is generally used with arrays to retrieve and manipulate the array elements. This is a binary or n-ary operator and is represented in two parts: Postfix/Primary ExpressionExpressionThe postfix expression, also known as the prima 6 min read
- reinterpret_cast in C++ | Type Casting operators reinterpret_cast is a type of casting operator used in C++. It is used to convert a pointer of some data type into a pointer of another data type, even if the data types before and after conversion are different.It does not check if the pointer type and data pointed by the pointer is same or not. Sy 3 min read
- forward_list::operator= in C++ STL Forward list in STL implements singly linked list. Introduced from C++11, forward lists are useful than other containers for insertion, removal and moving operations (like sort) and allows time constant insertion and removal of elements.It differs from the list by the fact that forward list keeps tr 2 min read
- array::operator[ ] in C++ STL Array classes are generally more efficient, light-weight, and reliable than C-style arrays. The introduction of array class from C++11 has offered a better alternative for C-style arrays. array::operator[] This operator is used to reference the element present at position given inside the operator. 2 min read
- Vector Operator = in C++ STL In C++, the vector operator = is used to assign the contents of one vector to another. It allows you to copy elements from one vector to another or initialize a vector with another vector's contents. Let’s take a look at a simple code example: [GFGTABS] C++ #include <bits/stdc++.h> using names 3 min read
- Overloading stream insertion (<>) operators in C++ In C++, stream insertion operator "<<" is used for output and extraction operator ">>" is used for input. We must know the following things before we start overloading these operators. 1) cout is an object of ostream class and cin is an object of istream class 2) These operators must be 2 min read
- What are the Operators that Can be and Cannot be Overloaded in C++? There are various ways to overload Operators in C++ by implementing any of the following types of functions: 1) Member Function 2) Non-Member Function 3) Friend Function List of operators that can be overloaded are: + - * ? % ? & | ~ ! = < > += -= *= ?= %= ?= &= |= << >> 4 min read
- Modulo Operator (%) in C/C++ with Examples In C or C++, the modulo operator (also known as the modulus operator), denoted by %, is an arithmetic operator. The modulo division operator produces the remainder of an integer division which is also called the modulus of the operation. Syntax of Modulus OperatorIf x and y are integers, then the ex 5 min read
- std::stoul and std::stoull in C++ std::stoul Convert string to unsigned integer. Parses str interpreting its content as an integral number of the specified base, which is returned as an unsigned long value. unsigned long stoul (const string& str, size_t* idx = 0, int base = 10); Parameters : str : String object with the represen 3 min read
- unordered_multiset operator = in C++ STL The ‘=’ is an operator in C++ STL which copies (or moves) an unordered_multiset to another unordered_multiset and unordered_multiset::operator= is the corresponding operator function. There are three versions of this function: The first version takes reference of an unordered_multiset as an argument 2 min read
- Relational Operators in C In C, relational operators are the symbols that are used for comparison between two values to understand the type of relationship a pair of numbers shares. The result that we get after the relational operation is a boolean value, that tells whether the comparison is true or false. Relational operato 4 min read
- How to sum two integers without using arithmetic operators in C/C++? Given two integers a and b, how can we evaluate the sum a + b without using operators such as +, -, ++, --, ...? Method 1 (Using pointers)An interesting way would be: C/C++ Code // May not work with C++ compilers and // may produce warnings in C. // Returns sum of 'a' and 'b' int sum(int a, int b) { 4 min read
- C++ Bit Manipulation
- cpp-operator
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
JS Tutorial
Js versions, js functions, js html dom, js browser bom, js web apis, js vs jquery, js graphics, js examples, js references, javascript assignment, javascript assignment operators.
Assignment operators assign values to JavaScript variables.
Shift Assignment Operators
Bitwise assignment operators, logical assignment operators, the = operator.
The Simple Assignment Operator assigns a value to a variable.
Simple Assignment Examples
The += operator.
The Addition Assignment Operator adds a value to a variable.
Addition Assignment Examples
The -= operator.
The Subtraction Assignment Operator subtracts a value from a variable.
Subtraction Assignment Example
The *= operator.
The Multiplication Assignment Operator multiplies a variable.
Multiplication Assignment Example
The **= operator.
The Exponentiation Assignment Operator raises a variable to the power of the operand.
Exponentiation Assignment Example
The /= operator.
The Division Assignment Operator divides a variable.
Division Assignment Example
The %= operator.
The Remainder Assignment Operator assigns a remainder to a variable.
Remainder Assignment Example
Advertisement
The <<= Operator
The Left Shift Assignment Operator left shifts a variable.
Left Shift Assignment Example
The >>= operator.
The Right Shift Assignment Operator right shifts a variable (signed).
Right Shift Assignment Example
The >>>= operator.
The Unsigned Right Shift Assignment Operator right shifts a variable (unsigned).
Unsigned Right Shift Assignment Example
The &= operator.
The Bitwise AND Assignment Operator does a bitwise AND operation on two operands and assigns the result to the the variable.
Bitwise AND Assignment Example
The |= operator.
The Bitwise OR Assignment Operator does a bitwise OR operation on two operands and assigns the result to the variable.
Bitwise OR Assignment Example
The ^= operator.
The Bitwise XOR Assignment Operator does a bitwise XOR operation on two operands and assigns the result to the variable.
Bitwise XOR Assignment Example
The &&= operator.
The Logical AND assignment operator is used between two values.
If the first value is true, the second value is assigned.
Logical AND Assignment Example
The &&= operator is an ES2020 feature .
The ||= Operator
The Logical OR assignment operator is used between two values.
If the first value is false, the second value is assigned.
Logical OR Assignment Example
The ||= operator is an ES2020 feature .
The ??= Operator
The Nullish coalescing assignment operator is used between two values.
If the first value is undefined or null, the second value is assigned.
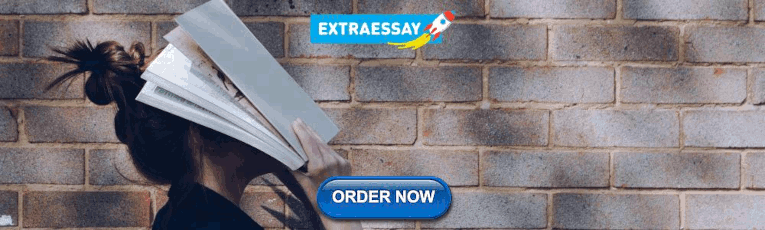
Nullish Coalescing Assignment Example
The ??= operator is an ES2020 feature .

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Specifications
Browser compatibility.
An assignment operator assigns a value to its left operand based on the value of its right operand.
The source for this interactive example is stored in a GitHub repository. If you'd like to contribute to the interactive examples project, please clone https://github.com/mdn/interactive-examples and send us a pull request.
The basic assignment operator is equal ( = ), which assigns the value of its right operand to its left operand. That is, x = y assigns the value of y to x . The other assignment operators are usually shorthand for standard operations, as shown in the following definitions and examples.
Simple assignment operator is used to assign a value to a variable. The assignment operation evaluates to the assigned value. Chaining the assignment operator is possible in order to assign a single value to multiple variables. See the example.
Addition assignment
The addition assignment operator adds the value of the right operand to a variable and assigns the result to the variable. The types of the two operands determine the behavior of the addition assignment operator. Addition or concatenation is possible. See the addition operator for more details.
Subtraction assignment
The subtraction assignment operator subtracts the value of the right operand from a variable and assigns the result to the variable. See the subtraction operator for more details.
Multiplication assignment
The multiplication assignment operator multiplies a variable by the value of the right operand and assigns the result to the variable. See the multiplication operator for more details.
Division assignment
The division assignment operator divides a variable by the value of the right operand and assigns the result to the variable. See the division operator for more details.
Remainder assignment
The remainder assignment operator divides a variable by the value of the right operand and assigns the remainder to the variable. See the remainder operator for more details.
Exponentiation assignment
The exponentiation assignment operator evaluates to the result of raising first operand to the power second operand. See the exponentiation operator for more details.
Left shift assignment
The left shift assignment operator moves the specified amount of bits to the left and assigns the result to the variable. See the left shift operator for more details.
Right shift assignment
The right shift assignment operator moves the specified amount of bits to the right and assigns the result to the variable. See the right shift operator for more details.
Unsigned right shift assignment
The unsigned right shift assignment operator moves the specified amount of bits to the right and assigns the result to the variable. See the unsigned right shift operator for more details.
Bitwise AND assignment
The bitwise AND assignment operator uses the binary representation of both operands, does a bitwise AND operation on them and assigns the result to the variable. See the bitwise AND operator for more details.
Bitwise XOR assignment
The bitwise XOR assignment operator uses the binary representation of both operands, does a bitwise XOR operation on them and assigns the result to the variable. See the bitwise XOR operator for more details.
Bitwise OR assignment
The bitwise OR assignment operator uses the binary representation of both operands, does a bitwise OR operation on them and assigns the result to the variable. See the bitwise OR operator for more details.
Left operand with another assignment operator
In unusual situations, the assignment operator (e.g. x += y ) is not identical to the meaning expression (here x = x + y ). When the left operand of an assignment operator itself contains an assignment operator, the left operand is evaluated only once. For example:
- Arithmetic operators
Document Tags and Contributors
- JavaScript basics
- JavaScript first steps
- JavaScript building blocks
- Introducing JavaScript objects
- Introduction
- Grammar and types
- Control flow and error handling
- Loops and iteration
- Expressions and operators
- Numbers and dates
- Text formatting
- Regular expressions
- Indexed collections
- Keyed collections
- Working with objects
- Details of the object model
- Using promises
- Iterators and generators
- Meta programming
- JavaScript modules
- Client-side web APIs
- A re-introduction to JavaScript
- JavaScript data structures
- Equality comparisons and sameness
- Inheritance and the prototype chain
- Strict mode
- JavaScript typed arrays
- Memory Management
- Concurrency model and Event Loop
- References:
- ArrayBuffer
- AsyncFunction
- Float32Array
- Float64Array
- GeneratorFunction
- InternalError
- Intl.Collator
- Intl.DateTimeFormat
- Intl.ListFormat
- Intl.Locale
- Intl.NumberFormat
- Intl.PluralRules
- Intl.RelativeTimeFormat
- ReferenceError
- SharedArrayBuffer
- SyntaxError
- Uint16Array
- Uint32Array
- Uint8ClampedArray
- WebAssembly
- decodeURI()
- decodeURIComponent()
- encodeURI()
- encodeURIComponent()
- parseFloat()
- Array comprehensions
- Bitwise operators
- Comma operator
- Comparison operators
- Conditional (ternary) operator
- Destructuring assignment
- Expression closures
- Generator comprehensions
- Grouping operator
- Legacy generator function expression
- Logical operators
- Object initializer
- Operator precedence
- (currently at stage 1) pipes the value of an expression into a function. This allows the creation of chained function calls in a readable manner. The result is syntactic sugar in which a function call with a single argument can be written like this:">Pipeline operator
- Property accessors
- Spread syntax
- async function expression
- class expression
- delete operator
- function expression
- function* expression
- in operator
- new operator
- void operator
- Legacy generator function
- async function
- for await...of
- for each...in
- function declaration
- import.meta
- try...catch
- Arrow functions
- Default parameters
- Method definitions
- Rest parameters
- The arguments object
- constructor
- element loaded from a different domain for which you violated the same-origin policy.">Error: Permission denied to access property "x"
- InternalError: too much recursion
- RangeError: argument is not a valid code point
- RangeError: invalid array length
- RangeError: invalid date
- RangeError: precision is out of range
- RangeError: radix must be an integer
- RangeError: repeat count must be less than infinity
- RangeError: repeat count must be non-negative
- ReferenceError: "x" is not defined
- ReferenceError: assignment to undeclared variable "x"
- ReferenceError: can't access lexical declaration`X' before initialization
- ReferenceError: deprecated caller or arguments usage
- ReferenceError: invalid assignment left-hand side
- ReferenceError: reference to undefined property "x"
- SyntaxError: "0"-prefixed octal literals and octal escape seq. are deprecated
- SyntaxError: "use strict" not allowed in function with non-simple parameters
- SyntaxError: "x" is a reserved identifier
- SyntaxError: JSON.parse: bad parsing
- SyntaxError: Malformed formal parameter
- SyntaxError: Unexpected token
- SyntaxError: Using //@ to indicate sourceURL pragmas is deprecated. Use //# instead
- SyntaxError: a declaration in the head of a for-of loop can't have an initializer
- SyntaxError: applying the 'delete' operator to an unqualified name is deprecated
- SyntaxError: for-in loop head declarations may not have initializers
- SyntaxError: function statement requires a name
- SyntaxError: identifier starts immediately after numeric literal
- SyntaxError: illegal character
- SyntaxError: invalid regular expression flag "x"
- SyntaxError: missing ) after argument list
- SyntaxError: missing ) after condition
- SyntaxError: missing : after property id
- SyntaxError: missing ; before statement
- SyntaxError: missing = in const declaration
- SyntaxError: missing ] after element list
- SyntaxError: missing formal parameter
- SyntaxError: missing name after . operator
- SyntaxError: missing variable name
- SyntaxError: missing } after function body
- SyntaxError: missing } after property list
- SyntaxError: redeclaration of formal parameter "x"
- SyntaxError: return not in function
- SyntaxError: test for equality (==) mistyped as assignment (=)?
- SyntaxError: unterminated string literal
- TypeError: "x" has no properties
- TypeError: "x" is (not) "y"
- TypeError: "x" is not a constructor
- TypeError: "x" is not a function
- TypeError: "x" is not a non-null object
- TypeError: "x" is read-only
- TypeError: 'x' is not iterable
- TypeError: More arguments needed
- TypeError: Reduce of empty array with no initial value
- TypeError: can't access dead object
- TypeError: can't access property "x" of "y"
- TypeError: can't assign to property "x" on "y": not an object
- TypeError: can't define property "x": "obj" is not extensible
- TypeError: can't delete non-configurable array element
- TypeError: can't redefine non-configurable property "x"
- TypeError: cannot use 'in' operator to search for 'x' in 'y'
- TypeError: cyclic object value
- TypeError: invalid 'instanceof' operand 'x'
- TypeError: invalid Array.prototype.sort argument
- TypeError: invalid arguments
- TypeError: invalid assignment to const "x"
- TypeError: property "x" is non-configurable and can't be deleted
- TypeError: setting getter-only property "x"
- TypeError: variable "x" redeclares argument
- URIError: malformed URI sequence
- Warning: -file- is being assigned a //# sourceMappingURL, but already has one
- Warning: 08/09 is not a legal ECMA-262 octal constant
- Warning: Date.prototype.toLocaleFormat is deprecated
- Warning: JavaScript 1.6's for-each-in loops are deprecated
- Warning: String.x is deprecated; use String.prototype.x instead
- Warning: expression closures are deprecated
- Warning: unreachable code after return statement
- X.prototype.y called on incompatible type
- JavaScript technologies overview
- Lexical grammar
- Enumerability and ownership of properties
- Iteration protocols
- Transitioning to strict mode
- Template literals
- Deprecated features
- ECMAScript 2015 support in Mozilla
- ECMAScript 5 support in Mozilla
- Firefox JavaScript changelog
- New in JavaScript 1.1
- New in JavaScript 1.2
- New in JavaScript 1.3
- New in JavaScript 1.4
- New in JavaScript 1.5
- New in JavaScript 1.6
- New in JavaScript 1.7
- New in JavaScript 1.8
- New in JavaScript 1.8.1
- New in JavaScript 1.8.5
- Documentation:
- All pages index
- Methods index
- Properties index
- Pages tagged "JavaScript"
- JavaScript doc status
- The MDN project
Learn the best of web development
Get the latest and greatest from MDN delivered straight to your inbox.
Thanks! Please check your inbox to confirm your subscription.
If you haven’t previously confirmed a subscription to a Mozilla-related newsletter you may have to do so. Please check your inbox or your spam filter for an email from us.
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
Left shift assignment (<<=)
The left shift assignment ( <<= ) operator performs left shift on the two operands and assigns the result to the left operand.
Description
x <<= y is equivalent to x = x << y , except that the expression x is only evaluated once.
Using left shift assignment
Specifications, browser compatibility.
- Assignment operators in the JS guide
- Left shift ( << )
- Skip to main content
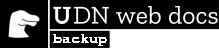
- Left shift assignment (<<=)
The left shift assignment operator ( <<= ) moves the specified amount of bits to the left and assigns the result to the variable.
The source for this interactive example is stored in a GitHub repository. If you'd like to contribute to the interactive examples project, please clone https://github.com/mdn/interactive-examples and send us a pull request.
Using left shift assignment
Specifications, browser compatibility.
- Assignment operators in the JS guide
- Left shift operator
- JavaScript basics
- JavaScript first steps
- JavaScript building blocks
- Introducing JavaScript objects
- Introduction
- Grammar and types
- Control flow and error handling
- Loops and iteration
- Expressions and operators
- Numbers and dates
- Text formatting
- Regular expressions
- Indexed collections
- Keyed collections
- Working with objects
- Details of the object model
- Using promises
- Iterators and generators
- Meta programming
- JavaScript modules
- Client-side JavaScript frameworks
- Client-side web APIs
- A re-introduction to JavaScript
- JavaScript data structures
- Equality comparisons and sameness
- Inheritance and the prototype chain
- Strict mode
- JavaScript typed arrays
- Memory Management
- Concurrency model and Event Loop
- References:
- AggregateError
- ArrayBuffer
- AsyncFunction
- BigInt64Array
- BigUint64Array
- FinalizationRegistry
- Float32Array
- Float64Array
- GeneratorFunction
- InternalError
- ReferenceError
- SharedArrayBuffer
- SyntaxError
- Uint16Array
- Uint32Array
- Uint8ClampedArray
- WebAssembly
- decodeURI()
- decodeURIComponent()
- encodeURI()
- encodeURIComponent()
- parseFloat()
- Addition (+)
- Addition assignment (+=)
- Assignment (=)
- Bitwise AND (&)
- Bitwise AND assignment (&=)
- Bitwise NOT (~)
- Bitwise OR (|)
- Bitwise OR assignment (|=)
- Bitwise XOR (^)
- Bitwise XOR assignment (^=)
- Comma operator (,)
- Conditional (ternary) operator
- Decrement (--)
- Destructuring assignment
- Division (/)
- Division assignment (/=)
- Equality (==)
- Exponentiation (**)
- Exponentiation assignment (**=)
- Function expression
- Greater than (>)
- Greater than or equal (>=)
- Grouping operator ( )
- Increment (++)
- Inequality (!=)
- Left shift (<<)
- Less than (<)
- Less than or equal (<=)
- Logical AND (&&)
- Logical AND assignment (&&=)
- Logical NOT (!)
- Logical OR (||)
- Logical OR assignment (||=)
- Logical nullish assignment (??=)
- Multiplication (*)
- Multiplication assignment (*=)
- Nullish coalescing operator (??)
- Object initializer
- Operator precedence
- Optional chaining (?.)
- Pipeline operator (|>)
- Property accessors
- Remainder (%)
- Remainder assignment (%=)
- Right shift (>>)
- Right shift assignment (>>=)
- Spread syntax (...)
- Strict equality (===)
- Strict inequality (!==)
- Subtraction (-)
- Subtraction assignment (-=)
- Unary negation (-)
- Unary plus (+)
- Unsigned right shift (>>>)
- Unsigned right shift assignment (>>>=)
- async function expression
- class expression
- delete operator
- function* expression
- in operator
- new operator
- void operator
- async function
- for await...of
- function declaration
- import.meta
- try...catch
- Arrow function expressions
- Default parameters
- Method definitions
- Rest parameters
- The arguments object
- Private class fields
- Public class fields
- constructor
- Error: Permission denied to access property "x"
- InternalError: too much recursion
- RangeError: argument is not a valid code point
- RangeError: invalid array length
- RangeError: invalid date
- RangeError: precision is out of range
- RangeError: radix must be an integer
- RangeError: repeat count must be less than infinity
- RangeError: repeat count must be non-negative
- ReferenceError: "x" is not defined
- ReferenceError: assignment to undeclared variable "x"
- ReferenceError: can't access lexical declaration "x" before initialization
- ReferenceError: deprecated caller or arguments usage
- ReferenceError: invalid assignment left-hand side
- ReferenceError: reference to undefined property "x"
- SyntaxError: "0"-prefixed octal literals and octal escape seq. are deprecated
- SyntaxError: "use strict" not allowed in function with non-simple parameters
- SyntaxError: "x" is a reserved identifier
- SyntaxError: JSON.parse: bad parsing
- SyntaxError: Malformed formal parameter
- SyntaxError: Unexpected token
- SyntaxError: Using //@ to indicate sourceURL pragmas is deprecated. Use //# instead
- SyntaxError: a declaration in the head of a for-of loop can't have an initializer
- SyntaxError: applying the "delete" operator to an unqualified name is deprecated
- SyntaxError: for-in loop head declarations may not have initializers
- SyntaxError: function statement requires a name
- SyntaxError: identifier starts immediately after numeric literal
- SyntaxError: illegal character
- SyntaxError: invalid regular expression flag "x"
- SyntaxError: missing ) after argument list
- SyntaxError: missing ) after condition
- SyntaxError: missing : after property id
- SyntaxError: missing ; before statement
- SyntaxError: missing = in const declaration
- SyntaxError: missing ] after element list
- SyntaxError: missing formal parameter
- SyntaxError: missing name after . operator
- SyntaxError: missing variable name
- SyntaxError: missing } after function body
- SyntaxError: missing } after property list
- SyntaxError: redeclaration of formal parameter "x"
- SyntaxError: return not in function
- SyntaxError: test for equality (==) mistyped as assignment (=)?
- SyntaxError: unterminated string literal
- TypeError: "x" has no properties
- TypeError: "x" is (not) "y"
- TypeError: "x" is not a constructor
- TypeError: "x" is not a function
- TypeError: "x" is not a non-null object
- TypeError: "x" is read-only
- TypeError: "x" is not iterable
- TypeError: More arguments needed
- TypeError: Reduce of empty array with no initial value
- TypeError: X.prototype.y called on incompatible type
- TypeError: can't access dead object
- TypeError: can't access property "x" of "y"
- TypeError: can't assign to property "x" on "y": not an object
- TypeError: can't define property "x": "obj" is not extensible
- TypeError: can't delete non-configurable array element
- TypeError: can't redefine non-configurable property "x"
- TypeError: cannot use "in" operator to search for "x" in "y"
- TypeError: cyclic object value
- TypeError: invalid "instanceof" operand "x"
- TypeError: invalid Array.prototype.sort argument
- TypeError: invalid arguments
- TypeError: invalid assignment to const "x"
- TypeError: property "x" is non-configurable and can't be deleted
- TypeError: setting getter-only property "x"
- TypeError: variable "x" redeclares argument
- URIError: malformed URI sequence
- Warning: -file- is being assigned a //# sourceMappingURL, but already has one
- Warning: 08/09 is not a legal ECMA-262 octal constant
- Warning: Date.prototype.toLocaleFormat is deprecated
- Warning: JavaScript 1.6's for-each-in loops are deprecated
- Warning: String.x is deprecated; use String.prototype.x instead
- Warning: expression closures are deprecated
- Warning: unreachable code after return statement
- JavaScript technologies overview
- Lexical grammar
- Enumerability and ownership of properties
- Iteration protocols
- Transitioning to strict mode
- Template literals
- Deprecated features
This page is Ready to Use
left shift assignment
Moves the specified number of bits to the left and assigns the result to result. The bits vacated by the operation are filled with 0.
Using the <<= operator is the same as specifying result = result << expression
Other articles
- Bitwise Left Shift Operator (<<)
- Bitwise Right Shift Operator (>>)
- Unsigned Right Shift Operator (>>>)
Attributions
Microsoft Developer Network: Article
- Accessibility
Left shift assignment (<<=)
The left shift assignment operator ( <<= ) moves the specified amount of bits to the left and assigns the result to the variable.
Using left shift assignment
Specifications, browser compatibility.
- Assignment operators in the JS guide
- Left shift operator
© 2005–2021 MDN contributors. Licensed under the Creative Commons Attribution-ShareAlike License v2.5 or later. https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Left_shift_assignment
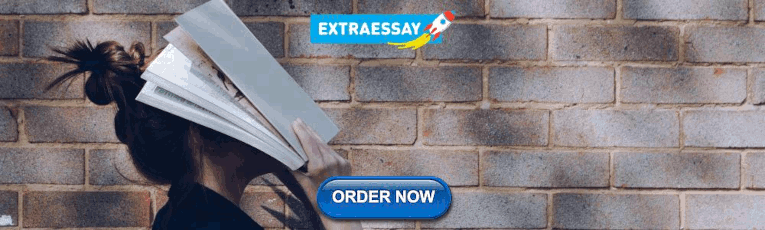
IMAGES
VIDEO
COMMENTS
The Left Shift Assignment Operator is represented by "<<=".This operator moves the specified number of bits to the left and assigns that result to the variable. We can fill the vacated place by 0. The left shift operator treats the integer stored in the variable to the operator's left as a 32-bit binary number.
Left Shift (<<) Operators. The left shift(<<) is a binary operator that takes two numbers, left shifts the bits of the first operand, and the second operand decides the number of places to shift. In other words, left-shifting an integer " a " with an integer " b " denoted as ' (a<<b)' is equivalent to multiplying a with 2^b (2 ...
Left Shift(<<): The left shift operator, shifts all of the bits in value to the left a specified number of times. Syntax: value << num. Here num specifies the number of position to left-shift the value in value. That is, the << moves all of the bits in the specified value to the left by the number of bit positions specified by num.
Learn how to use assignment operators to assign values to variables in JavaScript. See examples of simple, addition, subtraction, multiplication, division, remainder, shift, bitwise and logical assignment operators.
Left shift assignment. The left shift assignment operator moves the specified amount of bits to the left and assigns the result to the variable. See the left shift operator for more details. Syntax Operator: x <<= y Meaning: x = x << y Examples
The left shift assignment (<<=) operator performs left shift on the two operands and assigns the result to the left operand. Try it. Syntax. js. x <<= y Description. x <<= y is equivalent to x = x << y, except that the expression x is only evaluated once. Examples. Using left shift assignment. js.
The left shift assignment operator (<<=) moves the specified amount of bits to the left and assigns the result to the variable. The source for this interactive example is stored in a GitHub repository. If you'd like to contribute to the interactive examples project, ...
left shift assignment Summary. Moves the specified number of bits to the left and assigns the result to result. The bits vacated by the operation are filled with 0. Syntax result <<= expression result Any variable. expression The number of bits to move. Examples. Using the <<= operator is the same as specifying result = result << expression
Left shift assignment (<<=) The left shift assignment operator (<<=) moves the specified amount of bits to the left and assigns the result to the variable. Syntax x ...