
Home » Introduction and Basics » Typescript javascript one line if else else if statement
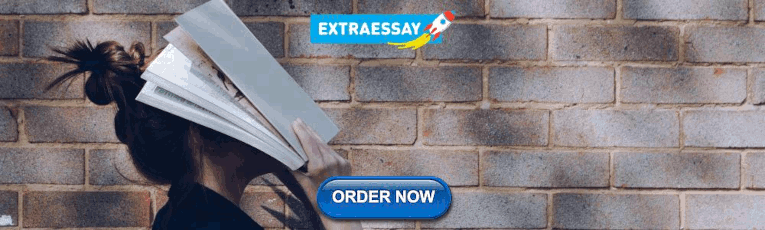
Typescript javascript one line if else else if statement
Introduction.
Typescript is a programming language that is a superset of JavaScript. It adds static typing to JavaScript, which helps catch errors at compile-time rather than runtime. One of the powerful features of Typescript is its ability to write concise and readable code. In this article, we will explore how to write a one-line if-else-else if statement in Typescript.
One-line if-else statement
In Typescript, we can write a one-line if-else statement using the ternary operator. The ternary operator is a shorthand way of writing an if-else statement in a single line. Here’s an example:
In the above example, we have a variable called “age” which is assigned a value of 18. We then use the ternary operator to check if the age is greater than or equal to 18. If it is, the variable “isAdult” is assigned the value true, otherwise it is assigned the value false.
One-line if-else if statement
Similarly, we can write a one-line if-else if statement using nested ternary operators. Here’s an example:
In the above example, we have a variable called "age" which is assigned a value of 18. We use nested ternary operators to check the age and assign the appropriate age group. If the age is less than 13, the variable "ageGroup" is assigned the value "Child". If the age is between 13 and 18, it is assigned the value "Teenager". Otherwise, it is assigned the value "Adult".
Typescript provides a concise and readable way to write one-line if-else and if-else if statements using the ternary operator. This allows developers to write clean and efficient code. By leveraging the power of Typescript, we can catch errors at compile-time and improve the overall quality of our code.
- No Comments
- javascript , statement , typescript
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
Table of Contents
Not stay with the doubts.

- Privacy Overview
- Strictly Necessary Cookies
This website uses cookies so that we can provide you with the best user experience possible. Cookie information is stored in your browser and performs functions such as recognising you when you return to our website and helping our team to understand which sections of the website you find most interesting and useful.
Strictly Necessary Cookie should be enabled at all times so that we can save your preferences for cookie settings.
If you disable this cookie, we will not be able to save your preferences. This means that every time you visit this website you will need to enable or disable cookies again.
Home » TypeScript Tutorial » TypeScript if else
TypeScript if else
Summary : in this tutorial, you will learn about the TypeScript if...else statement.
TypeScript if statement
An if statement executes a statement based on a condition. If the condition is truthy, the if statement will execute the statements inside its body:
For example, the following statement illustrates how to use the if statement to increase the counter variable if its value is less than the value of the max constant:
In this example, because the counter variable starts at zero, it is less than the max constant. The expression counter < max evaluates to true therefore the if statement executes the statement counter++ .
Let’s initialize the counter variable to 100 :
In this example, the expression counter < max evaluates to false . The if statement doesn’t execute the statement counter++ . Therefore, the output is 100.
TypeScript if…else statement
If you want to execute other statements when the condition in the if statement evaluates to false , you can use the if...else statement:
The following illustrates an example of using the if..else statement:
In this example, the expression counter < max evaluates to false therefore the statement in the else branch executes that resets the counter variable to 1 .
Ternary operator ?:
In practice, if you have a simple condition, you can use the ternary operator ?: rather than the if...else statement to make code shorter like this:
TypeScript if…else if…else statement
When you want to execute code based on multiple conditions, you can use the if...else if...else statement.
The if…else if…else statement can have one or more else if branches but only one else branch.
For example:
This example used the if...else if...else statement to determine the discount based on the number of items.
If the number of items is less than or equal to 5, the discount is 5%. The statement in the if branch executes.
If the number of items is less than or equal to 10, the discount is 10%. The statement in the else if branch executes.
When the number of items is greater than 10, the discount is 15%. The statement in the else branch executes.
In this example, the assumption is that the number of items is always greater than zero. However, if the number of items is less than zero or greater than 10, the discount is 15%.
To make the code more robust, you can use another else if instead of the else branch like this:
In this example, when the number of items is greater than 10, the discount is 15%. The statement in the second else if branch executes.
If the number of items is less than zero, the statement in the else branch executes.
- Use the if statement to execute code based on a condition.
- Use the else branch if you want to execute code when the condition is false. It’s good practice to use the ternary operator ?: instead of a simple if…else statement.
- Use if else if...else statement to execute code based on multiple conditions.
TypeScript - if else
An if statement can include one or more expressions which return boolean. If the boolean expression evaluates to true, a set of statements is then executed.
The following example includes multiple boolean expressions in the if condition.
In the above example, the if condition expression x < y is evaluated to true and so it executes the statement within the curly { } brackets.
if else Condition
An if else condition includes two blocks - if block and an else block. If the if condition evaluates to true, then the if block is executed. Otherwies, the else block is executed.
In the above example, the else block will be executed. Remember: else cannot include any condition and it must follow if or else if conditions.
The else if statement can be used after the if statement.
Ternary operator
A ternary operator is denoted by '?' and is used as a short cut for an if..else statement. It checks for a boolean condition and executes one of the two statements, depending on the result of the boolean condition.
In the above example, condition x > y is turned out be to false, so the second statement will be executed.
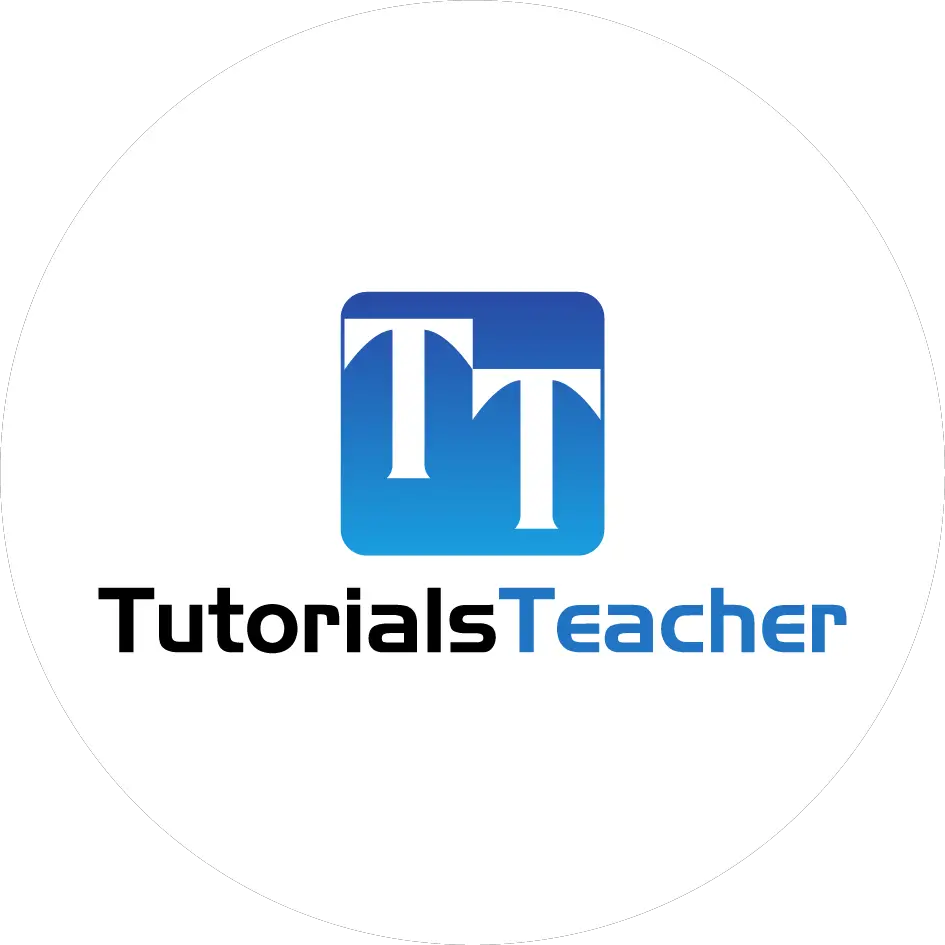
We are a team of passionate developers, educators, and technology enthusiasts who, with their combined expertise and experience, create in -depth, comprehensive, and easy to understand tutorials.We focus on a blend of theoretical explanations and practical examples to encourages hands - on learning. Visit About Us page for more information.
Popular Articles
- Typescript In Data Science: Career Prospects (Jun 24, 2024)
- Typescript In Iot Applications: Job Roles (Jun 24, 2024)
- Typescript In Cloud Computing: Career Growth (Jun 24, 2024)
- Typescript In Game Development: Job Opportunities (Jun 24, 2024)
- Typescript In Mobile App Development: Career Prospects (Jun 24, 2024)
TypeScript If
Switch to English
Table of Contents
Introduction
What is the typescript if statement, syntax of the typescript if statement, example of typescript if statement, common error-prone cases with typescript if statement, how to avoid errors, tips and tricks.
- The TypeScript If statement is a powerful control flow statement that lets you execute a block of code only when a specified condition is true.
- It's a crucial part of TypeScript as it allows the program to make decisions and execute statements based on those decisions.
- The 'condition' in the syntax can be any expression that evaluates to true or false. If the condition evaluates to true, then the code block within the if statement will be executed. Conversely, if the condition evaluates to false, then the program will skip over the if block.
- In this example, the If statement checks whether the age is greater than or equal to 18. If it is, then the program will print "You are eligible to vote".
- One common mistake is not wrapping the condition inside parentheses. This will throw a syntax error.
- Another common error is using a single equals sign (=) instead of double equals (==) or triple equals (===) for comparison in the if condition. The single equals sign is used for assignment, not comparison.
- Always wrap the condition inside parentheses.
- Use double equals (==) or triple equals (===) for comparisons. The triple equals (===) checks for both value and type, providing a stricter comparison than double equals (==).
- If you have multiple conditions to check, consider using 'Else If' and 'Else' statements in addition to the 'If' statement. They allow you to handle multiple conditions and provide a default action when none of the conditions are true.
- In TypeScript, you can also use the ternary operator (?:) as a shorthand for the If statement. However, it might not be as readable as a full If statement, especially when dealing with complex conditions.
I'm tired of these old-school single-line statements and looked up one-line solutions in TypeScript. The following one is pretty clean in my eyes:
How to set formControl default value but prevent to affect observable subscription
How to save csv data with filesaver js in typescript, how to solve error ts7031: binding element 'id' implicitly has an 'any' type., saved you some valuable time.
Buy me a drink 🍺 to keep me motivated to create free content like this!
Hello! I'm Stef, a software engineer near Antwerp, Belgium. For the past decade, I've built websites with Drupal and Angular for government and enterprise clients.
Since 2023, I've been captivated by generative art, especially with platforms like Midjourney.
Through this blog, I aim to share my code and projects with the community. Cheers and enjoy!

How to create one-line if statements in JavaScript

Kris Lachance
Head of Growth
One-line if statements in JavaScript are solid for concise conditional execution of code. They’re particularly good for simple conditions and actions that can be expressed neatly in a single line. We’ll go into more detail on all of this below.
What is the basic if statement?
Before diving into one-line if statements, you should know the basic if syntax:
Use the one-line if without curly braces
For a single statement following the if condition, braces can be omitted:
Employ the ternary operator for if-else
The ternary operator is the true one-liner for if-else statements:
Combine methods or operations
Chaining methods or operations in a one-liner if statement can keep your code terse:
Leverage short-circuit evaluation
Short-circuiting with logical operators allows if-else constructs in one line:
Handle assignment within one-line if
You can assign a value based on a condition in one line:
Use arrow functions for inline execution
Incorporate arrow functions for immediate execution within your one-liner:
How to handle multiple one-line if statements
When dealing with several conditions that require one-liners, ensure they remain readable:
Use one-liners in callbacks
One-liners can be effectively used within callback functions:
Know when to use if vs. ternary operator
The ternary operator is concise but use a regular if when the condition or actions are too complex for a ternary to remain clear.
Consider one-liners for default values
A one-liner if can set a default value if one isn't already assigned:
Be careful with one-liners and scope
Understand the scope of variables used in one-liners to avoid reference errors:
Remember operator precedence
When using logical operators in one-liners, keep operator precedence in mind to avoid unexpected results:
Avoid using one-liners for function declarations
Defining functions within one-liners can lead to readability and hoisting issues:
Use one-liners with template literals
Template literals can make your one-liners more readable when dealing with strings:
Understand limitations with const
Remember that const declarations cannot be used in traditional one-line if statements due to block-scoping:
Invite only
The next generation of charts.
Coming soon.
The next generation of charts. Coming soon.
Fast. Opinionated. Collaborative. Local-first. Keyboard centric. Crafted to the last pixel. We've got 50 slots for Alpha access.
Request access

Related posts

How to Remove Characters from a String in JavaScript
Jeremy Sarchet

How to Sort Strings in JavaScript

How to Remove Spaces from a String in JavaScript

Detecting Prime Numbers in JavaScript
Robert Cooper

How to Parse Boolean Values in JavaScript

How to Remove a Substring from a String in JavaScript
All JavaScript guides
Advisory boards aren’t only for executives. Join the LogRocket Content Advisory Board today →
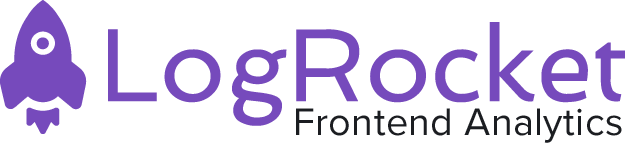
- Product Management
- Solve User-Reported Issues
- Find Issues Faster
- Optimize Conversion and Adoption
- Start Monitoring for Free
18 JavaScript and TypeScript shorthands to know
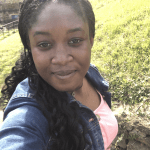
Editor’s note: This guide to the most useful JavaScript and TypeScript shorthands was last updated on 3 January 2023 to address errors in the code and include information about the satisfies operator introduced in TypeScript v4.9.
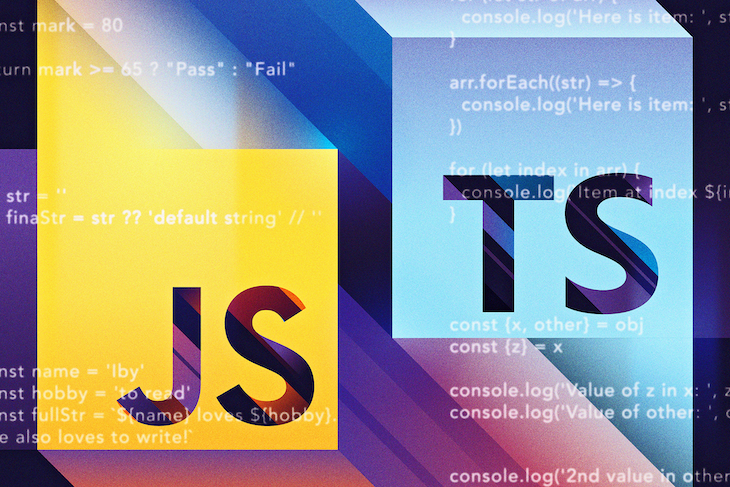
JavaScript and TypeScript share a number of useful shorthand alternatives for common code concepts. Shorthand code alternatives can help reduce lines of code, which is something we typically strive for.
In this article, we will review 18 common JavaScript and TypeScript and shorthands. We will also explore examples of how to use these shorthands.
Read through these useful JavaScript and TypeScript shorthands or navigate to the one you’re looking for in the list below.
Jump ahead:
JavaScript and TypeScript shorthands
Ternary operator, short-circuit evaluation, nullish coalescing operator, template literals, object property assignment shorthand, optional chaining, object destructuring, spread operator, object loop shorthand, array.indexof shorthand using the bitwise operator, casting values to boolean with , arrow/lambda function expression, implicit return using arrow function expressions, double bitwise not operator, exponent power shorthand, typescript constructor shorthand, typescript satisfies operator.
Using shorthand code is not always the right decision when writing clean and scalable code . Concise code can sometimes be more confusing to read and update. So, it is important that your code is legible and conveys meaning and context to other developers.
Our decision to use shorthands must not be detrimental to other desirable code characteristics. Keep this in mind when using the following shorthands for expressions and operators in JavaScript and TypeScript.
All shorthands available in JavaScript are available in the same syntax in TypeScript. The only slight differences are in specifying the type in TypeScript, and the TypeScript constructor shorthand is exclusive to TypeScript.
The ternary operator is one of the most popular shorthands in JavaScript and TypeScript. It replaces the traditional if…else statement. Its syntax is as follows:
The following example demonstrates a traditional if…else statement and its shorthand equivalent using the ternary operator:
The ternary operator is great when you have single-line operations like assigning a value to a variable or returning a value based on two possible conditions. Once there are more than two outcomes to your condition, using if/else blocks are much easier to read.
Another way to replace an if…else statement is with short-circuit evaluation. This shorthand uses the logical OR operator || to assign a default value to a variable when the intended value is falsy.
The following example demonstrates how to use short-circuit evaluation:
This shorthand is best used when you have a single-line operation and your condition depends on the falseness or non-falseness of a value/statement.
The nullish coalescing operator ?? is similar to short-circuit evaluation in that it assigns a variable a default value. However, the nullish coalescing operator only uses the default value when the intended value is also nullish.
In other words, if the intended value is falsy but not nullish, it will not use the default value.
Here are two examples of the nullish coalescing operator:
Example one
Example two, logical nullish assignment operator.
This is similar to the nullish coalescing operator by checking that a value is nullish and has added the ability to assign a value following the null check.
The example below demonstrates how we would check and assign in longhand and shorthand using the logical nullish assignment:
JavaScript has several other assignment shorthands like addition assignment += , multiplication assignment *= , division assignment /= , remainder assignment %= , and several others. You can find a full list of assignment operators here .
Template literals, which was introduced as part of JavaScript’s powerful ES6 features , can be used instead of + to concatenate multiple variables within a string. To use template literals, wrap your strings in `` and variables in ${} within those strings.
The example below demonstrates how to use template literals to perform string interpolation:
You can also use template literals to build multi-line strings without using \n . For example:
Using template literals is helpful for adding strings whose values may change into a larger string, like HTML templates. They are also useful for creating multi-line string because string wrapped in template literals retain all white spacing and indentation.
In JavaScript and TypeScript, you can assign a property to an object in shorthand by mentioning the variable in the object literal. To do this, the variable must be named with the intended key.
See an example of the object property assignment shorthand below:
Dot notation allows us to access the keys or values of an object. With optional chaining , we can go a step further and read keys or values even when we are not sure whether they exist or are set.
When the key does not exist, the value from optional chaining is undefined . This helps us avoid unneeded if/else check conditions when reading values from objects and unnecessary try/catch to handle errors thrown from trying to access object keys that don’t exist.
See an example of optional chaining in action below:
Besides the traditional dot notation, another way to read the values of an object is by destructuring the object’s values into their own variables.
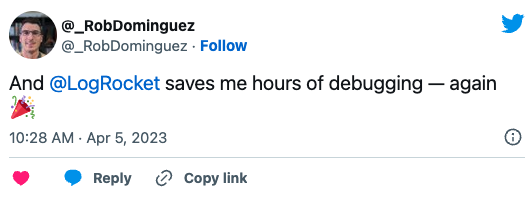
Over 200k developers use LogRocket to create better digital experiences

The following example demonstrates how to read the values of an object using the traditional dot notation compared to the shorthand method using object destructuring:
The spread operator … is used to access the content of arrays and objects. You can use the spread operator to replace array functions , like concat , and object functions, like object.assign .
Review the examples below to see how the spread operator can replace longhand array and object functions:
The traditional JavaScript for loop syntax is as follows:
We can use this loop syntax to iterate through arrays by referencing the array length for the iterator. There are three for loop shorthands that offer different ways to iterate through an array object:
- for…of : To access the array entries
- for…in : To access the indexes of an array and the keys when used on an object literal
- Array.forEach : To perform operations on the array elements and their indexes using a callback function
Please note, Array.forEach callbacks have three possible arguments, which are called in this order:
- The element of the array for the ongoing iteration
- The element’s index
- A full copy of the array
The examples below demonstrate these object loop shorthands in action:
We can look up the existence of an item in an array using the Array.indexOf method. This method returns the index position of the item if it exists in the array and returns -1 if it does not.
In JavaScript, 0 is a falsy value, while numbers less than or greater than 0 are considered truthy. Typically, this means we need to use an if…else statement to determine if the item exists using the returned index.
Using the bitwise operator ~ instead of an if…else statement allows us to get a truthy value for anything greater than or equal to 0 .
The example below demonstrates the Array.indexOf shorthand using the bitwise operator instead of an if…else statement:
In JavaScript, we can cast variables of any type to a Boolean value using the !![variable] shorthand.
See an example of using the !! [variable] shorthand to cast values to Boolean :
Functions in JavaScript can be written using arrow function syntax instead of the traditional expression that explicitly uses the function keyword. Arrow functions are similar to lambda functions in other languages .
Take a look at this example of writing a function in shorthand using an arrow function expression:
In JavaScript, we typically use the return keyword to return a value from a function. When we define our function using arrow function syntax, we can implicitly return a value by excluding braces {} .
For multi-line statements, such as expressions, we can wrap our return expression in parentheses () . The example below demonstrates the shorthand code for implicitly returning a value from a function using an arrow function expression:
In JavaScript, we typically access mathematical functions and constants using the built-in Math object. Some of those functions are Math.floor() , Math.round() , Math.trunc() , and many others.
The Math.trunc() (available in ES6) returns the integer part. For example, number(s) before the decimal of a given number achieves this same result using the Double bitwise NOT operator ~~ .
Review the example below to see how to use the Double bitwise NOT operator as a Math.trunc() shorthand:
It is important to note that the Double bitwise NOT operator ~~ is not an official shorthand for Math.trunc because some edge cases do not return the same result. More details on this are available here .
Another mathematical function with a useful shorthand is the Math.pow() function. The alternative to using the built-in Math object is the ** shorthand.
The example below demonstrates this exponent power shorthand in action:
There is a shorthand for creating a class and assigning values to class properties via the constructor in TypeScript . When using this method, TypeScript will automatically create and set the class properties . This shorthand is exclusive to TypeScript alone and not available in JavaScript class definitions.
Take a look at the example below to see the TypeScript constructor shorthand in action:
The satisfies operator gives some flexibility from the constraints of setting a type with the error handling covering having explicit types.
It is best used when a value has multiple possible types. For example, it can be a string or an array; with this operator, we don’t have to add any checks. Here’s an example:
In the longhand version of our example above, we had to do a typeof check to make sure palette.red was of the type RGB and that we could read its first property with at .
While in our shorthand version, using satisfies , we don’t have the type restriction of palette.red being string , but we can still tell the compiler to make sure palette and its properties have the correct shape.
The Array.property.at() i.e., at() method, accepts an integer and returns the item at that index. Array.at requires ES2022 target, which is available from TypeScript v4.6 onwards. More information is available here .
These are just a few of the most commonly used JavaScript and TypeScript shorthands.
JavaScript and TypeScript longhand and shorthand code typically work the same way under the hood, so choosing shorthand usually just means writing less lines of code. Remember, using shorthand code is not always the best option. What is most important is writing clean and understandable code that other developers can read easily.
What are your favorite JavaScript or TypeScript shorthands? Share them with us in the comments!
LogRocket : Debug JavaScript errors more easily by understanding the context
Debugging code is always a tedious task. But the more you understand your errors, the easier it is to fix them.
LogRocket allows you to understand these errors in new and unique ways. Our frontend monitoring solution tracks user engagement with your JavaScript frontends to give you the ability to see exactly what the user did that led to an error.
LogRocket records console logs, page load times, stack traces, slow network requests/responses with headers + bodies, browser metadata, and custom logs. Understanding the impact of your JavaScript code will never be easier!
Try it for free .
Share this:
- Click to share on Twitter (Opens in new window)
- Click to share on Reddit (Opens in new window)
- Click to share on LinkedIn (Opens in new window)
- Click to share on Facebook (Opens in new window)
- #typescript
- #vanilla javascript
Would you be interested in joining LogRocket's developer community?
Join LogRocket’s Content Advisory Board. You’ll help inform the type of content we create and get access to exclusive meetups, social accreditation, and swag.
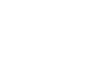
Stop guessing about your digital experience with LogRocket
Recent posts:.
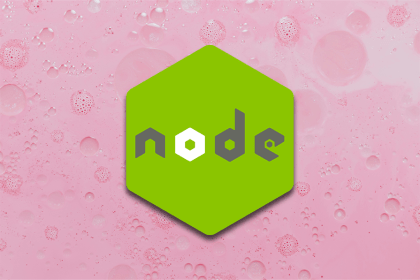
How to implement Coolify, the self-hosted alternative to Heroku
If you’ve been active on Twitter or Reddit lately, you’ve likely seen discussions surrounding Vercel’s pricing model, which has turned […]
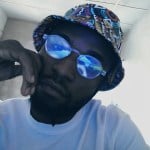
Zustand adoption guide: Overview, examples, and alternatives
Learn about Zustand’s simplistic approach to managing state and how it compares to existing tools such as Mobx and Redux.
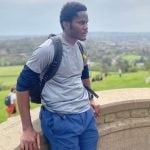
Working with custom elements in React
Dive into custom HTML elements, the challenges of using them with React, and the changes in React 19 that address these challenges.
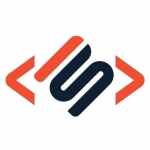
How to build a simple Svelte app
Developers can use both Svelte and React to effectively build web applications. While they serve the same overall purpose, there are distinct differences in how they work.
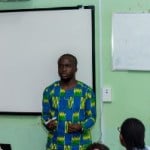
8 Replies to "18 JavaScript and TypeScript shorthands to know"
Thanks you for your article, I learn a lot with it.
But I think that I found a mistake in short circuit evaluation. When you show the traditional version with if…else statement you use logical && operator but I think you wanted use logical || operator.
I think that is just a wrting error but i prefer tell it to you.
Have a good day
Hi Romain thank you for spotting that! I’ll fix it right away
I was avoiding using logical OR to make clear the explanation of short circuit evaluation, so the if statement should be confirming “str” has a valid value. I have switched the assignment statements in the condition so it is correct now.
I think there is an error in the renamed variable of destructured object. Shouldn’t the line const {x: myVar} = object be: const {x: myVar} = obj
This code doesn’t work in Typescript? // for object literals const obj2 = { a: 1, b: 2, c: 3 }
for (let keyLetter in obj2) { console.log(`key: ${keyLetter} value: ${obj2[keyLetter]}`);
Gets error: error: TS7053 [ERROR]: Element implicitly has an ‘any’ type because expression of type ‘string’ can’t be used to index type ‘{ 0: number; 1: number; 2: number; }’. No index signature with a parameter of type ‘string’ was found on type ‘{ 0: number; 1: number; 2: number; }’. console.log(`key: ${keyLetter} value: ${obj2[keyLetter]}`);
Awesome information, thanks for sharing!!! 🚀🚀🚀
Good List of useful operators
~~x is not the same as Math.floor(x) : try it on negative numbers. You’ll find that ~~ is the same as Math.trunc(x) instead.
Leave a Reply Cancel reply
Stack Exchange Network
Stack Exchange network consists of 183 Q&A communities including Stack Overflow , the largest, most trusted online community for developers to learn, share their knowledge, and build their careers.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
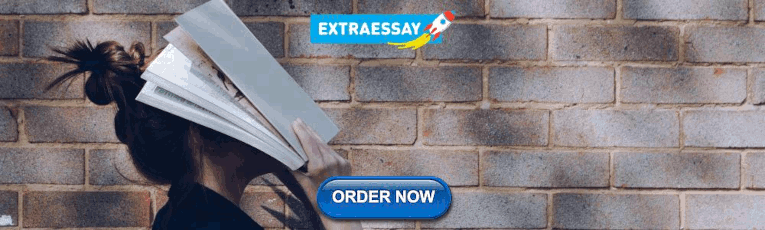
When to use single-line if statements? [closed]
When reviewing code, I prefer to read if statements that explicitly demonstrate that a fork in code execution may occur:
When scanning hundreds of lines of the nonindented version, it is not obvious that a conditional may or may not occur:
So when is it considered all right to single-line if statements? I'm willing to put up with it if the conditional is on a die(), return, or exception, because in those cases at least the current method ends.
Are there any other "good" uses for single-line if statements? How strictly should the practice be avoided? Note that I am explicitly asking about the lack of an indented line to indicate that a condition may or may not occur, I am not asking about the use of braces. Although I would appreciate all opinions in the comments, answers should address objective reasoning, such as maintainability or extensibility of code.
This is not a dupe of Single statement if block - braces or no because this question does not ask about braces. This is not a dupe of Single Line Statements & Good Practices because that question does not address the crux of this question: the ability to determine that some lines of code may or may not be run, thus leading to divergent code paths.
- coding-style
- 3 I see your point. However, the answers there might answer your question; this is something primarily opinion-based. – mike Commented Jul 1, 2015 at 13:54
- 2 Definitely subjective. I use them to check for nulls, and very simple/clear statements, just because I feel like conserving space. And who doesnt like elegant one liners: if(obj == null) break; – CodyF Commented Jul 1, 2015 at 14:41
- 2 "When to use single-line if statements?" Never. (please!) – utnapistim Commented Jul 1, 2015 at 14:44
- 3 @CodyF, And who doesnt like elegant one liners: if(obj == null) break; I don't; every time I find one in the current code base, it's a reading interruption (as in "WTF?! oh! same line!"). I have checked out more than a few files, just to fix the damn same-line ifs and commit again. – utnapistim Commented Jul 1, 2015 at 14:47
- 3 I'm not convinced either duplicate link is relevant when you read past their titles. – gbjbaanb Commented Jul 1, 2015 at 15:53
3 Answers 3
The only time to use a single-line if statement is when you have a lot of them and you can format your code to make it very clear what is happening. Anything else is not clear and will lead to errors.
is terrible. When mixed in with other code, it does not clearly show the conditional statement, and some people will confuse the following line as a badly-indented conditional. This applies to all statements, especially if they are die(), return or throw. It has nothing to do with the running of the code, its all about the readability.
this is much better than putting the conditionals on the next line as its clearer what is intended, there is no scope for confusion.
- 13 I would contend that even for single line if, to avoid future errors one should consider if (a) { do_a(); } with the braces. Yes, they're unnecessary but it will avoid static analysis warnings and make it harder to make a mistake in the future. – user40980 Commented Jul 1, 2015 at 13:31
- 1 that would usually be the argument to use a statemachine switch – Peter Commented Jul 11, 2023 at 6:20
I always prefer the version with braces.
- If I don't always use the braces, then I may forget to put them when there is more than one statement in the block.
- IDEs used by other team members can reformat the code automatically to move the statement to the new line, thus making it much more error prone in the future.
- Merging the code across the branches is more error prone if the if statement is a conflicting spot. The probability for error is even higher if the person doing the merge is not completely familiar with the merged changes.
- A statement not-belonging to the block may be included in it later by mistake (I add new statements to the block, so I need to add braces, but because of indentation or similar visual effects I also include a statement that should be left out of the block).

I would propose an alternative answer. I prefer single liners when the condition inside is really a single line and is relatively isolated from the rest of the conditions.
One great example is:
A bad example(in my opinion) would be what @gbjbaanb mentioned:
To me, this is a switch statement and should be written as such:
Much more readable IMHO and more efficient as variable needs to be evaluated only once VS running through all the if statements although gains are negligible.
- 1 Thank you. The OP also mentions that return statements are an exception that should be allowed, as the method ends there. – dotancohen Commented Jul 2, 2015 at 16:41
- 4 Your example assumes that a,b, and c are mutually exclusive. – Brian Commented Jul 2, 2015 at 16:54
- 2 @Brian You are correct, because if they are not, I would start looking at the design because your method is responsible for a bunch of operations, and that's not a good sign. – Alexus Commented Jul 2, 2015 at 17:21
- 2 Ugh... you prefer 13 verbose lines to 3? (Which, by the way, are far more readable due to the obvious repetition structure. And there's no chance of misplaced break s.) Efficiency is a non-issue since optimizers are usually smart enough for something this simple. – Mateen Ulhaq Commented Nov 9, 2018 at 21:30
- 1 They're logically equivalent. This is simple enough that I'm sure an optimizing compiler would generate the same assembly for both. Maybe I'll test it out when I get home. – Mateen Ulhaq Commented Nov 15, 2018 at 2:38
Not the answer you're looking for? Browse other questions tagged coding-style or ask your own question .
- The Overflow Blog
- Where does Postgres fit in a world of GenAI and vector databases?
- Featured on Meta
- We've made changes to our Terms of Service & Privacy Policy - July 2024
- Bringing clarity to status tag usage on meta sites
Hot Network Questions
- "TSA regulations state that travellers are allowed one personal item and one carry on"?
- Why there is no article after 'by'?
- Integral concerning the floor function
- The meaning of "by" in "swear by God"
- Where to donate foreign-language academic books?
- Regression techniques for a “triangular” scatterplot
- Completely introduce your friends
- Could someone tell me what this part of an A320 is called in English?
- Cramer's Rule when the determinant of coefficient matrix is zero?
- What prevents a browser from saving and tracking passwords entered to a site?
- Does the order of ingredients while cooking matter to an extent that it changes the overall taste of the food?
- Too many \setmathfont leads to "Too many symbol fonts declared" error
- How to reply to reviewers who ask for more work by responding that the paper is complete as it stands?
- How can I delete a column from several CSV files?
- I'm trying to remember a novel about an asteroid threatening to destroy the earth. I remember seeing the phrase "SHIVA IS COMING" on the cover
- Command-line script that strips out all comments in given source files
- Is Intuition Indispensable in Mathematics?
- Why do National Geographic and Discovery Channel broadcast fake or pseudoscientific programs?
- How does the summoned monster know who is my enemy?
- What explanations can be offered for the extreme see-sawing in Montana's senate race polling?
- What rule or standard is used to assign a SOT number to a chip housing?
- Raspberry Screen Application
- Who was the "Dutch author", "Bumstone Bumstone"?
- Why does a rolling ball slow down?
Clean One-Line JavaScript Conditionals
August 12, 2021 - 3 minutes read
Writing shorter conditionals in JavaScript, when appropriate, can help keep our code concise yet clean.
I will demonstrate three different shorthand JavaScript conditionals:
Condensed if...else block
One-line ternary expression
One-line binary expression
Link to this section Condensed if…else block
Here’s a standard if...else block in JavaScript:
And here’s one way it can be shortened into a single line:
It’s worth mentioning this method also works for else if and else conditions like this one:
Just place each condition on its one line:
However, there is a caveat to this approach .
Consider the following code:
It won’t work. If you’re going to use this type of one-line conditional as the body of an ES6 arrow function , then you’ll need to enclose it in brackets:
Link to this section One-line ternary expression
JavaScript’s ternary expression is a much more versatile one-line conditional. However, it only supports if and else conditions (but not else if conditions).
Here’s a typical block of code using an if and else condition:
Here’s an example of shortening that block using a ternary expression:
A ternary expression contains two special symbols, “ ? ” and “ : ”.
These two special symbols separate the expression into three parts:
The code before the ? is the condition.
The code between the ? and : is the expression to evaluate if the condition is truthy .
The code after the : is the expression to evaluate if the condition is falsy .
Ternary expressions can be used in variable assignments:
And they can also be interlopated within strings:
Link to this section One-line binary expression
Now this is the one that I’ve found especially useful.
The binary expression only supports if conditions, but neither else if nor else conditions.
Here’s a typical if condition:
And here’s how we can use one of JavaScript’s binary expressions to shorten it:
This binary expression is separated by the “ && ” binary operator into two parts:
The code before the && is the condition.
The code after the && is the expression to evaluate if the condition is truthy.
Link to this section Conclusion
You made it to the end!
I hope you find at least one of these shortened conditionals useful for cleaning and shortening your code.
Personally, I use them all the time.
Get the Reddit app
Chat about javascript and javascript related projects. Yes, typescript counts. Please keep self promotion to a minimum/reasonable level.
Single line if-statements
I tend to use a large variety of styles for a given situation. The traditional block-syntax is great for multiple things that need to happen given a condition.
Ternary is great for assigning one value vs another.
One style I've become a huge proponent of is this style of single line if-statement, which idk if it has a name:
I find this style to read extremely well and makes it very easy to do early-returns on a given function. However, some coworkers point out that this is bad (citing styling issues, primarily), and that I should be using curly-braces for these if-statements. We have had debates over the topic, no party budging.
I've also used the (slightly unintuitive) single line if-statements before and I can understand not liking it. To a novice this is really confusing:
You can do some pretty whacky things with if-statements (not recommended):
What are your thoughts on these different styles? I'm especially curious what people think of the style my coworkers dislike
By continuing, you agree to our User Agreement and acknowledge that you understand the Privacy Policy .
Enter the 6-digit code from your authenticator app
You’ve set up two-factor authentication for this account.
Enter a 6-digit backup code
Create your username and password.
Reddit is anonymous, so your username is what you’ll go by here. Choose wisely—because once you get a name, you can’t change it.
Reset your password
Enter your email address or username and we’ll send you a link to reset your password
Check your inbox
An email with a link to reset your password was sent to the email address associated with your account
Choose a Reddit account to continue
Was this page helpful?
Variable Declaration
let and const are two relatively new concepts for variable declarations in JavaScript. As we mentioned earlier , let is similar to var in some respects, but allows users to avoid some of the common “gotchas” that users run into in JavaScript.
const is an augmentation of let in that it prevents re-assignment to a variable.
With TypeScript being an extension of JavaScript, the language naturally supports let and const . Here we’ll elaborate more on these new declarations and why they’re preferable to var .
If you’ve used JavaScript offhandedly, the next section might be a good way to refresh your memory. If you’re intimately familiar with all the quirks of var declarations in JavaScript, you might find it easier to skip ahead.
var declarations
Declaring a variable in JavaScript has always traditionally been done with the var keyword.
As you might’ve figured out, we just declared a variable named a with the value 10 .
We can also declare a variable inside of a function:
and we can also access those same variables within other functions:
In this above example, g captured the variable a declared in f . At any point that g gets called, the value of a will be tied to the value of a in f . Even if g is called once f is done running, it will be able to access and modify a .
Scoping rules
var declarations have some odd scoping rules for those used to other languages. Take the following example:
Some readers might do a double-take at this example. The variable x was declared within the if block , and yet we were able to access it from outside that block. That’s because var declarations are accessible anywhere within their containing function, module, namespace, or global scope - all which we’ll go over later on - regardless of the containing block. Some people call this var -scoping or function-scoping . Parameters are also function scoped.
These scoping rules can cause several types of mistakes. One problem they exacerbate is the fact that it is not an error to declare the same variable multiple times:
Maybe it was easy to spot out for some experienced JavaScript developers, but the inner for -loop will accidentally overwrite the variable i because i refers to the same function-scoped variable. As experienced developers know by now, similar sorts of bugs slip through code reviews and can be an endless source of frustration.
Variable capturing quirks
Take a quick second to guess what the output of the following snippet is:
For those unfamiliar, setTimeout will try to execute a function after a certain number of milliseconds (though waiting for anything else to stop running).
Ready? Take a look:
Many JavaScript developers are intimately familiar with this behavior, but if you’re surprised, you’re certainly not alone. Most people expect the output to be
Remember what we mentioned earlier about variable capturing? Every function expression we pass to setTimeout actually refers to the same i from the same scope.
Let’s take a minute to consider what that means. setTimeout will run a function after some number of milliseconds, but only after the for loop has stopped executing; By the time the for loop has stopped executing, the value of i is 10 . So each time the given function gets called, it will print out 10 !
A common work around is to use an IIFE - an Immediately Invoked Function Expression - to capture i at each iteration:
This odd-looking pattern is actually pretty common. The i in the parameter list actually shadows the i declared in the for loop, but since we named them the same, we didn’t have to modify the loop body too much.
let declarations
By now you’ve figured out that var has some problems, which is precisely why let statements were introduced. Apart from the keyword used, let statements are written the same way var statements are.
The key difference is not in the syntax, but in the semantics, which we’ll now dive into.
Block-scoping
When a variable is declared using let , it uses what some call lexical-scoping or block-scoping . Unlike variables declared with var whose scopes leak out to their containing function, block-scoped variables are not visible outside of their nearest containing block or for -loop.
Here, we have two local variables a and b . a ’s scope is limited to the body of f while b ’s scope is limited to the containing if statement’s block.
Variables declared in a catch clause also have similar scoping rules.
Another property of block-scoped variables is that they can’t be read or written to before they’re actually declared. While these variables are “present” throughout their scope, all points up until their declaration are part of their temporal dead zone . This is just a sophisticated way of saying you can’t access them before the let statement, and luckily TypeScript will let you know that.
Something to note is that you can still capture a block-scoped variable before it’s declared. The only catch is that it’s illegal to call that function before the declaration. If targeting ES2015, a modern runtime will throw an error; however, right now TypeScript is permissive and won’t report this as an error.
For more information on temporal dead zones, see relevant content on the Mozilla Developer Network .
Re-declarations and Shadowing
With var declarations, we mentioned that it didn’t matter how many times you declared your variables; you just got one.
In the above example, all declarations of x actually refer to the same x , and this is perfectly valid. This often ends up being a source of bugs. Thankfully, let declarations are not as forgiving.
The variables don’t necessarily need to both be block-scoped for TypeScript to tell us that there’s a problem.
That’s not to say that a block-scoped variable can never be declared with a function-scoped variable. The block-scoped variable just needs to be declared within a distinctly different block.
The act of introducing a new name in a more nested scope is called shadowing . It is a bit of a double-edged sword in that it can introduce certain bugs on its own in the event of accidental shadowing, while also preventing certain bugs. For instance, imagine we had written our earlier sumMatrix function using let variables.
This version of the loop will actually perform the summation correctly because the inner loop’s i shadows i from the outer loop.
Shadowing should usually be avoided in the interest of writing clearer code. While there are some scenarios where it may be fitting to take advantage of it, you should use your best judgement.
Block-scoped variable capturing
When we first touched on the idea of variable capturing with var declaration, we briefly went into how variables act once captured. To give a better intuition of this, each time a scope is run, it creates an “environment” of variables. That environment and its captured variables can exist even after everything within its scope has finished executing.
Because we’ve captured city from within its environment, we’re still able to access it despite the fact that the if block finished executing.
Recall that with our earlier setTimeout example, we ended up needing to use an IIFE to capture the state of a variable for every iteration of the for loop. In effect, what we were doing was creating a new variable environment for our captured variables. That was a bit of a pain, but luckily, you’ll never have to do that again in TypeScript.
let declarations have drastically different behavior when declared as part of a loop. Rather than just introducing a new environment to the loop itself, these declarations sort of create a new scope per iteration . Since this is what we were doing anyway with our IIFE, we can change our old setTimeout example to just use a let declaration.
and as expected, this will print out
const declarations
const declarations are another way of declaring variables.
They are like let declarations but, as their name implies, their value cannot be changed once they are bound. In other words, they have the same scoping rules as let , but you can’t re-assign to them.
This should not be confused with the idea that the values they refer to are immutable .
Unless you take specific measures to avoid it, the internal state of a const variable is still modifiable. Fortunately, TypeScript allows you to specify that members of an object are readonly . The chapter on Interfaces has the details.
let vs. const
Given that we have two types of declarations with similar scoping semantics, it’s natural to find ourselves asking which one to use. Like most broad questions, the answer is: it depends.
Applying the principle of least privilege , all declarations other than those you plan to modify should use const . The rationale is that if a variable didn’t need to get written to, others working on the same codebase shouldn’t automatically be able to write to the object, and will need to consider whether they really need to reassign to the variable. Using const also makes code more predictable when reasoning about flow of data.
Use your best judgement, and if applicable, consult the matter with the rest of your team.
The majority of this handbook uses let declarations.
Destructuring
Another ECMAScript 2015 feature that TypeScript has is destructuring. For a complete reference, see the article on the Mozilla Developer Network . In this section, we’ll give a short overview.
Array destructuring
The simplest form of destructuring is array destructuring assignment:
This creates two new variables named first and second . This is equivalent to using indexing, but is much more convenient:
Destructuring works with already-declared variables as well:
And with parameters to a function:
You can create a variable for the remaining items in a list using the syntax ... :
Of course, since this is JavaScript, you can just ignore trailing elements you don’t care about:
Or other elements:
Tuple destructuring
Tuples may be destructured like arrays; the destructuring variables get the types of the corresponding tuple elements:
It’s an error to destructure a tuple beyond the range of its elements:
As with arrays, you can destructure the rest of the tuple with ... , to get a shorter tuple:
Or ignore trailing elements, or other elements:
Object destructuring
You can also destructure objects:
This creates new variables a and b from o.a and o.b . Notice that you can skip c if you don’t need it.
Like array destructuring, you can have assignment without declaration:
Notice that we had to surround this statement with parentheses. JavaScript normally parses a { as the start of block.
You can create a variable for the remaining items in an object using the syntax ... :
Property renaming
You can also give different names to properties:
Here the syntax starts to get confusing. You can read a: newName1 as ” a as newName1 ”. The direction is left-to-right, as if you had written:
Confusingly, the colon here does not indicate the type. The type, if you specify it, still needs to be written after the entire destructuring:
Default values
Default values let you specify a default value in case a property is undefined:
In this example the b? indicates that b is optional, so it may be undefined . keepWholeObject now has a variable for wholeObject as well as the properties a and b , even if b is undefined.
Function declarations
Destructuring also works in function declarations. For simple cases this is straightforward:
But specifying defaults is more common for parameters, and getting defaults right with destructuring can be tricky. First of all, you need to remember to put the pattern before the default value.
The snippet above is an example of type inference, explained earlier in the handbook.
Then, you need to remember to give a default for optional properties on the destructured property instead of the main initializer. Remember that C was defined with b optional:
Use destructuring with care. As the previous example demonstrates, anything but the simplest destructuring expression is confusing. This is especially true with deeply nested destructuring, which gets really hard to understand even without piling on renaming, default values, and type annotations. Try to keep destructuring expressions small and simple. You can always write the assignments that destructuring would generate yourself.
The spread operator is the opposite of destructuring. It allows you to spread an array into another array, or an object into another object. For example:
This gives bothPlus the value [0, 1, 2, 3, 4, 5] . Spreading creates a shallow copy of first and second . They are not changed by the spread.
You can also spread objects:
Now search is { food: "rich", price: "$$", ambiance: "noisy" } . Object spreading is more complex than array spreading. Like array spreading, it proceeds from left-to-right, but the result is still an object. This means that properties that come later in the spread object overwrite properties that come earlier. So if we modify the previous example to spread at the end:
Then the food property in defaults overwrites food: "rich" , which is not what we want in this case.
Object spread also has a couple of other surprising limits. First, it only includes an objects’ own, enumerable properties . Basically, that means you lose methods when you spread instances of an object:
Second, the TypeScript compiler doesn’t allow spreads of type parameters from generic functions. That feature is expected in future versions of the language.
using declarations
using declarations are an upcoming feature for JavaScript that are part of the Stage 3 Explicit Resource Management proposal. A using declaration is much like a const declaration, except that it couples the lifetime of the value bound to the declaration with the scope of the variable.
When control exits the block containing a using declaration, the [Symbol.dispose]() method of the declared value is executed, which allows that value to perform cleanup:
At runtime, this has an effect roughly equivalent to the following:
using declarations are extremely useful for avoiding memory leaks when working with JavaScript objects that hold on to native references like file handles
or scoped operations like tracing
Unlike var , let , and const , using declarations do not support destructuring.
null and undefined
It’s important to note that the value can be null or undefined , in which case nothing is disposed at the end of the block:
which is roughly equivalent to:
This allows you to conditionally acquire resources when declaring a using declaration without the need for complex branching or repetition.
Defining a disposable resource
You can indicate the classes or objects you produce are disposable by implementing the Disposable interface:
await using declarations
Some resources or operations may have cleanup that needs to be performed asynchronously. To accommodate this, the Explicit Resource Management proposal also introduces the await using declaration:
An await using declaration invokes, and awaits , its value’s [Symbol.asyncDispose]() method as control leaves the containing block. This allows for asynchronous cleanup, such as a database transaction performing a rollback or commit, or a file stream flushing any pending writes to storage before it is closed.
As with await , await using can only be used in an async function or method, or at the top level of a module.
Defining an asynchronously disposable resource
Just as using relies on objects that are Disposable , an await using relies on objects that are AsyncDisposable :
await using vs await
The await keyword that is part of the await using declaration only indicates that the disposal of the resource is await -ed. It does not await the value itself:
await using and return
It’s important to note that there is a small caveat with this behavior if you are using an await using declaration in an async function that returns a Promise without first await -ing it:
Because the returned promise isn’t await -ed, it’s possible that the JavaScript runtime may report an unhandled rejection since execution pauses while await -ing the asynchronous disposal of x , without having subscribed to the returned promise. This is not a problem that is unique to await using , however, as this can also occur in an async function that uses try..finally :
To avoid this situation, it is recommended that you await your return value if it may be a Promise :
using and await using in for and for..of statements
Both using and await using can be used in a for statement:
In this case, the lifetime of x is scoped to the entire for statement and is only disposed when control leaves the loop due to break , return , throw , or when the loop condition is false.
In addition to for statements, both declarations can also be used in for..of statements:
Here, x is disposed at the end of each iteration of the loop , and is then reinitialized with the next value. This is especially useful when consuming resources produced one at a time by a generator.
using and await using in older runtimes
using and await using declarations can be used when targeting older ECMAScript editions as long as you are using a compatible polyfill for Symbol.dispose / Symbol.asyncDispose , such as the one provided by default in recent editions of NodeJS.
Nightly Builds
How to use a nightly build of TypeScript
The TypeScript docs are an open source project. Help us improve these pages by sending a Pull Request ❤
Last updated: Aug 26, 2024
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Best Way for Conditional Variable Assignment
Which is the better way for conditional variable assignment?
I actually prefer 2nd one without any specific technical reason. What do you guys think?
- 4 Doesn't the first limit myVariable to the scope it has been assigned in and can't be used outside of the if or the else it has been assigned in. – Tomaltach Commented Oct 13, 2017 at 9:59
- 1 var is funky in javascript and is scoped to the function (or global) not the block. That's another advantage of using let over var . – Jordan Soltman Commented Jun 4, 2019 at 1:27
15 Answers 15

- 5 Is this different than the first way? Isn't it the same thing with different syntax? – Andy Groff Commented Jun 7, 2012 at 6:48
- @Rajkumar, This is no different from the first. I actually want clarification about the over-riding thing. The second way is assigning a variable and then overriding it with something else. Is that a good way to do? – RaviTeja Commented Jun 7, 2012 at 8:13
- 1 yeah.. you can do that. whenever there is a default value.. .second way is good. In second way actually you are assigning a default value false and later on you are modifying it based on conditions. – dku.rajkumar Commented Jun 7, 2012 at 9:59
- What if you have 3? Why no inline ifelse statement in the world? – gunslingor Commented Aug 23, 2022 at 19:43
There are two methods I know of that you can declare a variable's value by conditions.
Method 1: If the condition evaluates to true, the value on the left side of the column would be assigned to the variable. If the condition evaluates to false the condition on the right will be assigned to the variable. You can also nest many conditions into one statement.
Nesting example of method 1: Change variable A value to 0, 1, 2 and a negative value to see how the statement would produce the result.
Method 2: In this method, if the value of the left of the || is equal to zero, false, null, undefined, or an empty string, then the value on the right will be assigned to the variable. If the value on the left of the || does not equal to zero, false, null undefined, or an empty string, then the value on the left will be assigned to the variable.
Although the value on the left can be an undefined value for JS to evaluate the condition but the variable has to be declared otherwise an exception will be produced.
- 1 I like the || because it reminds me of perl in the olden days. – MarkHu Commented Oct 31, 2018 at 18:07
An alternative way of doing this is by leveraging the ability of logical operators to return a value.
let isAnimal = false; let isPlant = true; let thing = isAnimal && 'animal' || isPlant && 'plant' || 'something else'; console.log(thing);
In the code above when one of the flags is true isAnimal or isPlant , the string next to it is returned. This is because both && and || result in the value of one of their operands:
- A && B returns the value A if A can be coerced into false; otherwise, it returns B.
- A || B returns the value A if A can be coerced into true; otherwise, it returns B.
Answer inspired by this article: https://mariusschulz.com/blog/the-and-and-or-operators-in-javascript
PS: Should be used for learning purposes only. Don't make life harder for you and your coworkers by using this method in your production code.

- 1 can anyone explain the PS added at the end? ...Why "learning purposes only" and not for use in production code? My guess is that it's unusual compare to other languages, so it'd maybe cause confusion. – C-Note187 Commented Mar 28, 2022 at 18:41
- 1 @C-Note187 I added the PS because while it's a concise way to do assignment, it also hard to understand what the code does on first glance. So if someone's doing code review or maybe finds this line of code during development, he's going to waste some time trying to understand it. In these cases it would be easier to just use if statements so that whoever is looking at the code can understand the logic quickly, with less mental overhead. – Valentin Commented Apr 1, 2022 at 9:20
- 1 oh, you are the best. I just wanted more than two on conditions and single ternary operator was not enough and I didn't want that long ifs or switch. – irakli2692 Commented Jul 17, 2023 at 18:38
- I think what @Valentin is also getting at with regard to production code is you shouldn't depend on tests that use coerced values but rather do specific tests on variables. – David Welborn Commented Mar 16 at 15:58
You could do a ternary, which is a lot shorter (and no darn curly braces):

Another cool thing is that you can do multiple assignment based on a conditional:
Third way when you are storing only true false in variabel then use
Just for completion, there is another way in addition to all the others mentioned here, which is to use a lookup table.
Say you have many possible values, you could declaratively configure a Map instead of using an if , switch or ternary statement.
This works even for booleans:
For booleans you would probably do it the 'normal' way though with logic operators specifically designed for that. Though sometimes it can be useful, such as:
- portability : you can pass a map around
- configurability : maybe the values come from a property file
- readability : if you don't care it's a boolean or not, you just want to avoid conditional logic and reduce cognitive load that way
Note there is some overlap between the advantages using a lookup map and advantages of using a function variable (closure).
The first solution uses only one assignment instead of 1,5 by average in the second code snippet. On the other hand the first code snippet is less readable as people not familiar with JavaScript might not realize that the scope of a variable is not block oriented by function oriented - on other languages with C-like syntax myVariable would not be accessible outside if and else blocks.
In other words both solutions have disadvantages. What about ternary operator:
or if you don't care about the camel-case (although I understand this is just an example, not a real code);
If you tired of ternary operator then use IIFE
Another way would be to use Immediately Invoked Function Expression . The good thing about it is that it can hold some logic and can be encapsulated from the outside world.
const direction = "n"; const directionFull= (() => { switch(direction ){ case "n": return "north"; case "s": return "south"; case "w": return "west"; case "e": return "east"; } })() console.log(directionFull);
I would prefer 2nd option too, no technical reason but for the sake of easy to read code, readability is very important in code.
If you see the second option, from processing point of view only one check will ever be executed, saved some very minute processing time, so there is only one check in second case.
- one technical reason:there will be only one condition check in second – user1432124 Commented Jun 7, 2012 at 6:47
It depends on the use for me. If I have code that I only want to run if true, but with no extra code for false, I'll use the second. If I want to execute some code on true, and different on false, I use the first. It all depends on use, but the general rule for me is to write once. Keep it clean, keep it simple, and keep it short

Maybe you simply need && operator to check if boolean is true, if it is, assing "myVariable" to true.

If all you need to do is convert a boolean to a string, you should do so explicitly:
If it's important that the first letter be capitalized, as in your example, that is not difficult to do; see e.g. this answer .
Another approach with Map and Object: (Maps are more flexible with key types and Objects are more readable IMHO)
const condition_var = 'e' const options1 = new Map([ ['n','north'],['s','south'],['e','east'],['w','west']]) const myVar1 = options1.get(condition_var) || null const options2 = {n:'north', s:'south', e:'east', w:'west'} const myVar2 = options2[condition_var] || null console.log(myVar1) console.log(myVar2)
If what you're trying to do is set a value if it is not null/undefined, and otherwise use another value, you can use the nullish coalescing operator :
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged javascript or ask your own question .
- The Overflow Blog
- Where does Postgres fit in a world of GenAI and vector databases?
- Featured on Meta
- We've made changes to our Terms of Service & Privacy Policy - July 2024
- Bringing clarity to status tag usage on meta sites
- What does a new user need in a homepage experience on Stack Overflow?
- Feedback requested: How do you use tag hover descriptions for curating and do...
- Staging Ground Reviewer Motivation
Hot Network Questions
- How would you say a couple of letters (as in mail) if they're not necessarily letters?
- Does the order of ingredients while cooking matter to an extent that it changes the overall taste of the food?
- Why doesn't the world fill with time travelers?
- Using Thin Lens Equation to find how far 1972 Blue Marble photo was taken
- Integral concerning the floor function
- Should I report a review I suspect to be AI-generated?
- Chess.com AI says I lost opportunity to win queen but I can't see how
- Safety pictograms
- Is this a new result about hexagon?
- How can judicial independence be jeopardised by politicians' criticism?
- What rule or standard is used to assign a SOT number to a chip housing?
- Distinctive form of "לאהוב ל-" instead of "לאהוב את"
- How do we reconcile the story of the woman caught in adultery in John 8 and the man stoned for picking up sticks on Sabbath in Numbers 15?
- How to remove obligation to run as administrator in Windows?
- My school wants me to download an SSL certificate to connect to WiFi. Can I just avoid doing anything private while on the WiFi?
- Using conditionals within \tl_put_right from latex3 explsyntax
- Two way ANOVA or two way repeat measurement ANOVA
- I'm trying to remember a novel about an asteroid threatening to destroy the earth. I remember seeing the phrase "SHIVA IS COMING" on the cover
- Are quantum states like the W, Bell, GHZ, and Dicke state actually used in quantum computing research?
- My visit is for two weeks but my host bought insurance for two months is it okay
- Expected number of numbers that stay at their place after k swaps
- Is there a phrase for someone who's really bad at cooking?
- How does the summoned monster know who is my enemy?
- Do the amplitude and frequency of gravitational waves emitted by binary stars change as the stars get closer together?
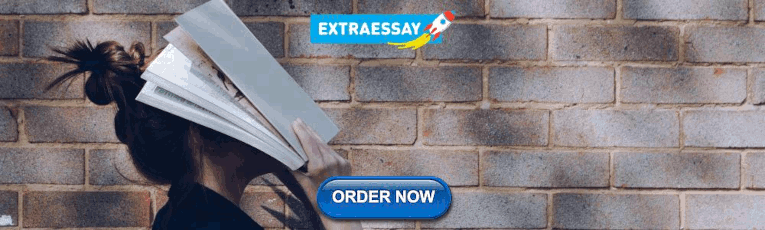
IMAGES
VIDEO
COMMENTS
I know you can set variables with one line if/else statements by doing var variable = (condition) ? (true block) : (else block), but I was wondering if there was a way to put an else if statement in there. Any suggestions would be appreciated, thanks everyone!
If you just want an inline IF (without the ELSE), you can use the logical AND operator: (a < b) && /*your code*/; If you need an ELSE also, use the ternary operation that the other people suggested. edited Jul 15, 2015 at 20:31. answered Jul 14, 2015 at 3:51. Nahn.
Introduction Typescript is a programming language that is a superset of JavaScript. It adds static typing to JavaScript, which helps catch errors at compile-time rather than runtime. One of the powerful features of Typescript is its ability to write concise and readable code. In this article, we will explore how to write a one-line if-else-else […]
TypeScript if statement An if statement executes a statement based on a condition. If the condition is truthy, the if statement will execute the statements inside its body:
When the type on the left of the extends is assignable to the one on the right, then you'll get the type in the first branch (the "true" branch); otherwise you'll get the type in the latter branch (the "false" branch).. From the examples above, conditional types might not immediately seem useful - we can tell ourselves whether or not Dog extends Animal and pick number or string!
In TypeScript, an `else if` statement is a continuation of an `if` statement. It allows the programmer to include additional conditions that will be checked if the previous conditions are not met. It enables us to create complex logical constructs in a clean and efficient manner. ... This is because the assignment `age = 30` changes the age to ...
In the above example, the if condition expression x < y is evaluated to true and so it executes the statement within the curly { } brackets.. if else Condition. An if else condition includes two blocks - if block and an else block. If the if condition evaluates to true, then the if block is executed. Otherwies, the else block is executed.
The TypeScript If statement is a powerful control flow statement that lets you execute a block of code only when a specified condition is true. It's a crucial part of TypeScript as it allows the program to make decisions and execute statements based on those decisions. Syntax of the TypeScript If Statement. Streak.
TypeScript's Logical Toolbox: A Step-by-Step Guide to Logical Statements such as if, else if, and else; Implementation of Logical Operators; and Enhancing Code Efficiency via Conditional Ternary ...
Logical Operators and Assignment. Logical Operators and Assignment are new features in JavaScript for 2020. These are a suite of new operators which edit a JavaScript object. Their goal is to re-use the concept of mathematical operators (e.g. += -= *=) but with logic instead. interface User {. id?: number.
Learn how to write clean single-line if statements in TypeScript with a concise and readable solution to improve your code quality and productivity. ... I'm tired of these old-school single-line statements and looked up one-line solutions in TypeScript. The following one is pretty clean in my eyes:
Handle assignment within one-line if. You can assign a value based on a condition in one line: let variable = condition ? valueIfTrue : valueIfFalse; Use arrow functions for inline execution. Incorporate arrow functions for immediate execution within your one-liner:
A ternary operator should be used in a situation where an if statement would create unneeded clutter (single-line variable assignments and single-line conditionals are great examples) or where an ...
Object property assignment shorthand. In JavaScript and TypeScript, you can assign a property to an object in shorthand by mentioning the variable in the object literal. To do this, the variable must be named with the intended key. See an example of the object property assignment shorthand below: // Longhand const obj = { x: 1, y: 2, z: 3 }
I would propose an alternative answer. I prefer single liners when the condition inside is really a single line and is relatively isolated from the rest of the conditions. One great example is: public void DoSomething(int something) { // Notice how easily we can state in one line that we should exit the method if our int is 0.
Clean One-Line JavaScript Conditionals. August 12, 2021 - 3 minutes read. Writing shorter conditionals in JavaScript, when appropriate, can help keep our code concise yet clean. I will demonstrate three different shorthand JavaScript conditionals: Condensed if...else block. One-line ternary expression. One-line binary expression
The first is an extremely dirty method I don't like, but it still rends down technically to a single expression: it's a bool composed of atleast one function evaluation, possibly 2. So it's still a single action/statement per line. The latter not only has two statements on one line, but also contains branching logic. Yikes.
Now available on Stack Overflow for Teams! AI features where you work: search, IDE, and chat. Learn more Explore Teams
var declarations. Declaring a variable in JavaScript has always traditionally been done with the var keyword. var a = 10; As you might've figured out, we just declared a variable named a with the value 10. We can also declare a variable inside of a function: function f() {.
There are two methods I know of that you can declare a variable's value by conditions. Method 1: If the condition evaluates to true, the value on the left side of the column would be assigned to the variable. If the condition evaluates to false the condition on the right will be assigned to the variable. You can also nest many conditions into ...