Python Variables
In Python, a variable is a container that stores a value. In other words, variable is the name given to a value, so that it becomes easy to refer a value later on.
Unlike C# or Java, it's not necessary to explicitly define a variable in Python before using it. Just assign a value to a variable using the = operator e.g. variable_name = value . That's it.
The following creates a variable with the integer value.
In the above example, we declared a variable named num and assigned an integer value 10 to it. Use the built-in print() function to display the value of a variable on the console or IDLE or REPL .
In the same way, the following declares variables with different types of values.
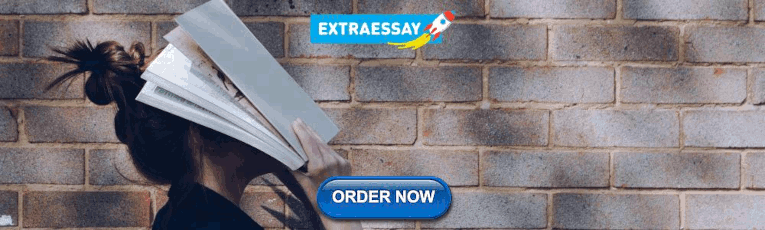
Multiple Variables Assignment
You can declare multiple variables and assign values to each variable in a single statement, as shown below.
In the above example, the first int value 10 will be assigned to the first variable x, the second value to the second variable y, and the third value to the third variable z. Assignment of values to variables must be in the same order in they declared.
You can also declare different types of values to variables in a single statement separated by a comma, as shown below.
Above, the variable x stores 10 , y stores a string 'Hello' , and z stores a boolean value True . The type of variables are based on the types of assigned value.
Assign a value to each individual variable separated by a comma will throw a syntax error, as shown below.
Variables in Python are objects. A variable is an object of a class based on the value it stores. Use the type() function to get the class name (type) of a variable.
In the above example, num is an object of the int class that contains integre value 10 . In the same way, amount is an object of the float class, greet is an object of the str class, isActive is an object of the bool class.
Unlike other programming languages like C# or Java, Python is a dynamically-typed language, which means you don't need to declare a type of a variable. The type will be assigned dynamically based on the assigned value.
The + operator sums up two int variables, whereas it concatenates two string type variables.
Object's Identity
Each object in Python has an id. It is the object's address in memory represented by an integer value. The id() function returns the id of the specified object where it is stored, as shown below.
Variables with the same value will have the same id.
Thus, Python optimize memory usage by not creating separate objects if they point to same value.
Naming Conventions
Any suitable identifier can be used as a name of a variable, based on the following rules:
- The name of the variable should start with either an alphabet letter (lower or upper case) or an underscore (_), but it cannot start with a digit.
- More than one alpha-numeric characters or underscores may follow.
- The variable name can consist of alphabet letter(s), number(s) and underscore(s) only. For example, myVar , MyVar , _myVar , MyVar123 are valid variable names, but m*var , my-var , 1myVar are invalid variable names.
- Variable names in Python are case sensitive. So, NAME , name , nAME , and nAmE are treated as different variable names.
- Variable names cannot be a reserved keywords in Python.
- Compare strings in Python
- Convert file data to list
- Convert User Input to a Number
- Convert String to Datetime in Python
- How to call external commands in Python?
- How to count the occurrences of a list item?
- How to flatten list in Python?
- How to merge dictionaries in Python?
- How to pass value by reference in Python?
- Remove duplicate items from list in Python
- More Python articles
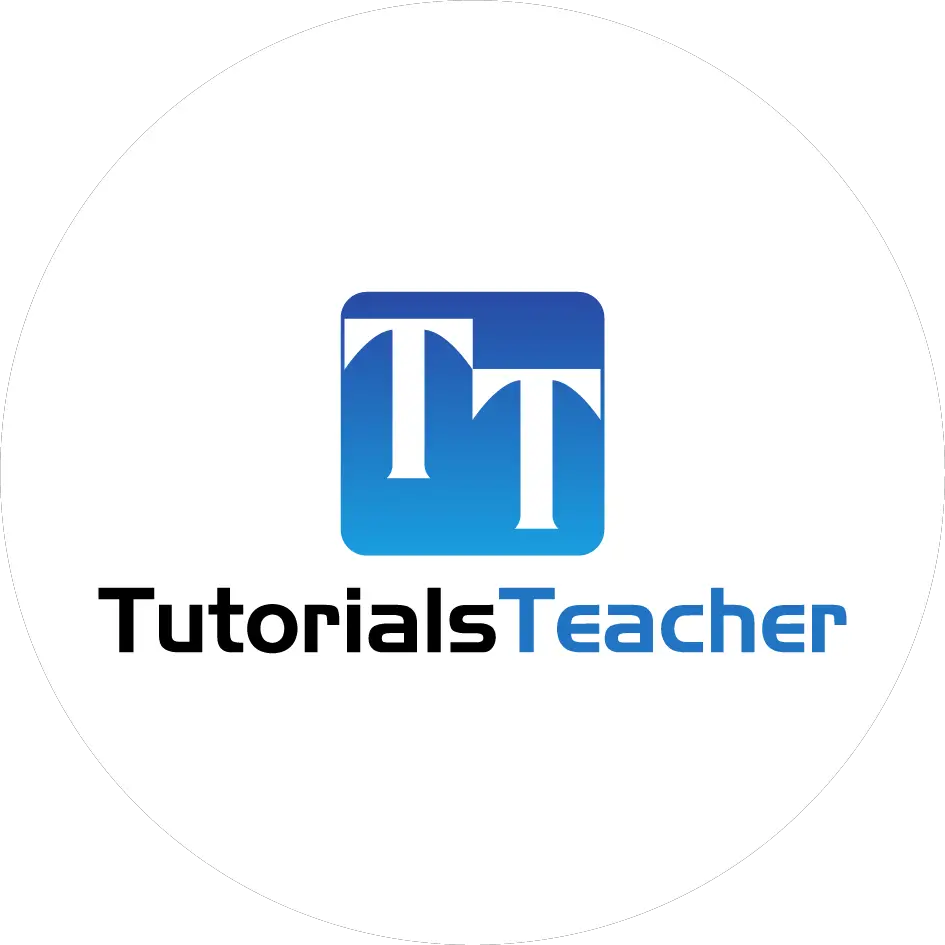
We are a team of passionate developers, educators, and technology enthusiasts who, with their combined expertise and experience, create in -depth, comprehensive, and easy to understand tutorials.We focus on a blend of theoretical explanations and practical examples to encourages hands - on learning. Visit About Us page for more information.
- Python Questions & Answers
- Python Skill Test
- Python Latest Articles
Python Variables – The Complete Beginner's Guide

Variables are an essential part of Python. They allow us to easily store, manipulate, and reference data throughout our projects.
This article will give you all the understanding of Python variables you need to use them effectively in your projects.
If you want the most convenient way to review all the topics covered here, I've put together a helpful cheatsheet for you right here:
Download the Python variables cheatsheet (it takes 5 seconds).
What is a Variable in Python?
So what are variables and why do we need them?
Variables are essential for holding onto and referencing values throughout our application. By storing a value into a variable, you can reuse it as many times and in whatever way you like throughout your project.
You can think of variables as boxes with labels, where the label represents the variable name and the content of the box is the value that the variable holds.
In Python, variables are created the moment you give or assign a value to them.
How Do I Assign a Value to a Variable?
Assigning a value to a variable in Python is an easy process.
You simply use the equal sign = as an assignment operator, followed by the value you want to assign to the variable. Here's an example:
In this example, we've created two variables: country and year_founded. We've assigned the string value "United States" to the country variable and integer value 1776 to the year_founded variable.
There are two things to note in this example:
- Variables in Python are case-sensitive . In other words, watch your casing when creating variables, because Year_Founded will be a different variable than year_founded even though they include the same letters
- Variable names that use multiple words in Python should be separated with an underscore _ . For example, a variable named "site name" should be written as "site_name" . This convention is called snake case (very fitting for the "Python" language).
How Should I Name My Python Variables?
There are some rules to follow when naming Python variables.
Some of these are hard rules that must be followed, otherwise your program will not work, while others are known as conventions . This means, they are more like suggestions.
Variable naming rules
- Variable names must start with a letter or an underscore _ character.
- Variable names can only contain letters, numbers, and underscores.
- Variable names cannot contain spaces or special characters.
Variable naming conventions
- Variable names should be descriptive and not too short or too long.
- Use lowercase letters and underscores to separate words in variable names (known as "snake_case").
What Data Types Can Python Variables Hold?
One of the best features of Python is its flexibility when it comes to handling various data types.
Python variables can hold various data types, including integers, floats, strings, booleans, tuples and lists:
Integers are whole numbers, both positive and negative.
Floats are real numbers or numbers with a decimal point.
Strings are sequences of characters, namely words or sentences.
Booleans are True or False values.
Lists are ordered, mutable collections of values.
Tuples are ordered, immutable collections of values.
There are more data types in Python, but these are the most common ones you will encounter while working with Python variables.
Python is Dynamically Typed
Python is what is known as a dynamically-typed language. This means that the type of a variable can change during the execution of a program.
Another feature of dynamic typing is that it is not necessary to manually declare the type of each variable, unlike other programming languages such as Java.
You can use the type() function to determine the type of a variable. For instance:
What Operations Can Be Performed?
Variables can be used in various operations, which allows us to transform them mathematically (if they are numbers), change their string values through operations like concatenation, and compare values using equality operators.
Mathematic Operations
It's possible to perform basic mathematic operations with variables, such as addition, subtraction, multiplication, and division:
It's also possible to find the remainder of a division operation by using the modulus % operator as well as create exponents using the ** syntax:
String operators
Strings can be added to one another or concatenated using the + operator.
Equality comparisons
Values can also be compared in Python using the < , > , == , and != operators.
These operators, respectively, compare whether values are less than, greater than, equal to, or not equal to each other.
Finally, note that when performing operations with variables, you need to ensure that the types of the variables are compatible with each other.
For example, you cannot directly add a string and an integer. You would need to convert one of the variables to a compatible type using a function like str() or int() .
Variable Scope
The scope of a variable refers to the parts of a program where the variable can be accessed and modified. In Python, there are two main types of variable scope:
Global scope : Variables defined outside of any function or class have a global scope. They can be accessed and modified throughout the program, including within functions and classes.
Local scope : Variables defined within a function or class have a local scope. They can only be accessed and modified within that function or class.
In this example, attempting to access local_var outside of the function_with_local_var function results in a NameError , as the variable is not defined in the global scope.
Don't be afraid to experiment with different types of variables, operations, and scopes to truly grasp their importance and functionality. The more you work with Python variables, the more confident you'll become in applying these concepts.
Finally, if you want to fully learn all of these concepts, I've put together for you a super helpful cheatsheet that summarizes everything we've covered here.
Just click the link below to grab it for free. Enjoy!
Download the Python variables cheatsheet
Become a Professional React Developer
React is hard. You shouldn't have to figure it out yourself.
I've put everything I know about React into a single course, to help you reach your goals in record time:
Introducing: The React Bootcamp
It’s the one course I wish I had when I started learning React.
Click below to try the React Bootcamp for yourself:

Full stack developer sharing everything I know.
If this article was helpful, share it .
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
Declaring Variables and Assigning Values in Python
Variables are an essential component of any programming language. They allow developers to store data in memory for later use and manipulation. In Python, variables provide a way to label and reference data in your code.
This article will provide a comprehensive, step-by-step guide on declaring variables and assigning values in Python. We will cover the following topics:
Table of Contents
Variable names and conventions, declaring and initializing variables, variable types in python, reassigning variables, multiple assignment, constants in python, best practices for using variables.
When declaring a variable in Python, you need to follow certain rules and conventions for naming them:
Case Sensitivity: Variable names are case-sensitive. For example, myvar and myVar would be distinct variables.
Use Meaningful Names: Choose descriptive names that reflect the data the variable represents. For example, student_count rather than sc .
Length: Variable names can be any length, but concise names are preferred for readability.
Allowed Characters: Variable names can only contain alphanumeric characters (a-z, A-Z, 0-9) and underscores (_). They cannot start with a number.
Reserved Words: Avoid using Python’s reserved words like if , else , while etc. as variable names.
Following Python’s PEP 8 style guide for naming conventions will make your code easier to read and maintain.
In Python, variables don’t need explicit declaration to reserve memory space. The variable is automatically created when you first assign it a value.
To declare a variable, simply assign it a value using the equals sign (=).
You can also initialize a variable during declaration by assigning its initial value.
Python is a dynamically typed language, so the data type of a variable is set by the value assigned to it.
In the above examples, count is initialized as an integer, price as a float, website as a string, and rating as a float. The variables can be reassigned to values of any type later on.
The common data types that can be stored in variables include:
- Integers: Whole numbers like 2, 4, -456. No decimal point.
- Floats: Decimal numbers like 1.5, -3.14, 0.456.
- Strings: Text data like “Hello”, ‘World!’, character sequences. Use single or double quotes around strings.
- Booleans: Logical values True and False.
- None Type: Special object None representing absence of value.
Additionally, Python has built-in types like lists, tuples, dictionaries that can also be assigned to variables. Custom classes can also be defined and instantiated as variables.
The value and data type of a variable can be changed after initial assignment in Python. Simply assign a new value to the variable to reinitialize it.
The ability to reassign variables makes Python very flexible. Reusing variable names can help reduce overall memory usage compared to constantly creating new variables.
However, reusing variables for different purposes can make code difficult to understand. Use descriptive names and single responsibility principle to avoid misuse.
Python allows assigning a single value to several variables simultaneously.
This is called multiple assignment and works by assigning the value to the first variable, then linking the rest to it. All variables refer to the same object in memory.
Multiple assignment can be used to swap two variables easily without needing a temporary variable.
Python does not have built-in constant types like other languages. However, by convention capital letters are used to denote constants in Python.
To prevent reassignment of a variable, the constant module can be used:
Constants make code more readable by defining immutable fixed values that have a clear purpose. Use them instead of hardcoding values throughout the code.
Follow these best practices when declaring and using variables in Python:
Use meaningful, descriptive variable names like student_count instead of short names like s_cnt .
Avoid single letter names like x and y except for common math variables.
Declare variables close to where they are first used instead of at the top.
Initialize variables when declaring them to avoid undefined states.
Reuse variables cautiously to avoid overwriting values accidentally.
Use constants like MAX_LENGTH = 100 for fixed values instead of hardcoding.
Limit variable scope by declaring and using them in smallest reasonable scope.
Avoid modifying loop control variables like index inside loop body.
Properly naming and using variables makes programs easier to read and reduces chances of errors. It takes a bit more effort up front, but pays off in maintaining and extending code.
This guide covered key topics related to declaring variables and assigning values in Python, including:
- Naming rules and conventions for Python variable names
- Declaring by simple assignment and initializing variables
- Python’s dynamic typing and common data types for variables
- Reassigning variables to new values and types
- Multiple variable assignment shorthand
- Defining constants using all caps and the constant module
- Best practices for effectively using variables in Python
Variables are the basic building blocks of any Python program. Using them judiciously and following Python’s style guide will lead to code that is more readable, maintainable, and less error-prone. The concepts covered here apply to all Python versions and provide a solid foundation to build programming skills.
- python
- variables-and-operators
Python Programming
Python Variables
Updated on: August 31, 2021 | 19 Comments
A variable is a reserved memory area (memory address) to store value . For example, we want to store an employee’s salary. In such a case, we can create a variable and store salary using it. Using that variable name, you can read or modify the salary amount.
In other words, a variable is a value that varies according to the condition or input pass to the program. Everything in Python is treated as an object so every variable is nothing but an object in Python.
A variable can be either mutable or immutable . If the variable’s value can change, the object is called mutable, while if the value cannot change, the object is called immutable. We will learn the difference between mutable and immutable types in the later section of this article.
Also, Solve :
- Python variables and data type Quiz
- Basic Python exercise for beginners
Table of contents
Creating a variable, changing the value of a variable, integer variable, float variable, complex type, string variable, list type variable, get the data type of variable, delete a variable, variable’s case-sensitive, assigning a value to a constant in python, rules and naming convention for variables and constants, assigning a single value to multiple variables, assigning multiple values to multiple variables, local variable, global variable, object reference, unpack a collection into a variable.
Python programming language is dynamically typed, so there is no need to declare a variable before using it or declare the data type of variable like in other programming languages. The declaration happens automatically when we assign a value to the variable.
Creating a variable and assigning a value
We can assign a value to the variable at that time variable is created. We can use the assignment operator = to assign a value to a variable.
The operand, which is on the left side of the assignment operator, is a variable name. And the operand, which is the right side of the assignment operator, is the variable’s value.
In the above example, “John”, 25, 25800.60 are values that are assigned to name , age , and salary respectively.
Many programming languages are statically typed languages where the variable is initially declared with a specific type, and during its lifetime, it must always have that type.
But in Python, variables are dynamically typed and not subject to the data type restriction. A variable may be assigned to a value of one type , and then later, we can also re-assigned a value of a different type . Let’s see the example.
Create Number, String, List variables
We can create different types of variables as per our requirements. Let’s see each one by one.
A number is a data type to store numeric values. The object for the number will be created when we assign a value to the variable. In Python3, we can use the following three data types to store numeric values.
The int is a data type that returns integer type values (signed integers); they are also called ints or integers . The integer value can be positive or negative without a decimal point.
Note : We used the built-in Python method type() to check the variable type.
Floats are the values with the decimal point dividing the integer and the fractional parts of the number. Use float data type to store decimal values.
In the above example, the variable salary assigned to value 10800.55, which is a float value.
The complex is the numbers that come with the real and imaginary part. A complex number is in the form of a+bj, where a and b contain integers or floating-point values.
In Python, a string is a set of characters represented in quotation marks. Python allows us to define a string in either pair of single or double quotation marks. For example, to store a person’s name we can use a string type.
To retrieve a piece of string from a given string, we can use to slice operator [] or [:] . Slicing provides us the subset of a string with an index starting from index 0 to index end-1.
To concatenate the string, we can use the addition (+) operator.
If we want to represent a group of elements (or value) as a single entity, we should go for the list variable type. For example, we can use them to store student names. In the list, the insertion order of elements is preserved. That means, in which order elements are inserted in the list, the order will be intact.
Read : Complete Guide on Python lists
The list can be accessed in two ways, either positive or negative index. The list has the following characteristics:
- In the list insertion order of elements is preserved.
- Heterogeneous (all types of data types int , float , string ) elements are allowed.
- Duplicates elements are permitted.
- The list is mutable(can change).
- Growable in nature means based on our requirement, we can increase or decrease the list’s size.
- List elements should be enclosed within square brackets [] .
No matter what is stored in a variable (object), a variable can be any type like int , float , str , list , tuple , dict , etc. There is a built-in function called type() to get the data type of any variable.
The type() function has a simple and straightforward syntax.
Syntax of type() :
If we want to get the name of the datatype only as output, then we can use the __name__ attribute along with the type() function. See the following example where __name__ attribute is used.
Use the del keyword to delete the variable. Once we delete the variable, it will not be longer accessible and eligible for the garbage collector.
Now, let’s delete var1 and try to access it again.
Python is a case-sensitive language. If we define a variable with names a = 100 and A =200 then, Python differentiates between a and A . These variables are treated as two different variables (or objects).
Constant is a variable or value that does not change, which means it remains the same and cannot be modified. But in the case of Python, the constant concept is not applicable . By convention, we can use only uppercase characters to define the constant variable if we don’t want to change it.
Example
It is just convention, but we can change the value of MAX_VALUE variable.
As we see earlier, in the case of Python, the constant concept is not applicable. But if we still want to implement it, we can do it using the following way.
The declaration and assignment of constant in Python done with the module. Module means Python file ( .py ) which contains variables, functions, and packages.
So let’s create two modules, constant.py and main.py , respectively.
- In the constant.py file, we will declare two constant variables, PI and TOTAL_AREA .
- import constant module In main.py file.
To create a constant module write the below code in the constant.py file.
Constants are declared with uppercase later variables and separating the words with an underscore.
Create a main.py and write the below code in it.
Note : Constant concept is not available in Python. By convention, we define constants in an uppercase letter to differentiate from variables. But it does not prevent reassignment, which means we can change the value of a constant variable.
A name in a Python program is called an identifier. An identifier can be a variable name, class name, function name, and module name.
There are some rules to define variables in Python.
In Python, there are some conventions and rules to define variables and constants that should follow.
Rule 1 : The name of the variable and constant should have a combination of letters, digits, and underscore symbols.
- Alphabet/letters i.e., lowercase (a to z) or uppercase (A to Z)
- Digits(0 to 9)
- Underscore symbol (_)
Example
Rule 2 : The variable name and constant name should make sense.
Note: we should always create a meaningful variable name so it will be easy to understand. That is, it should be meaningful.
It above example variable x does not make more sense, but student_name is a meaningful variable.
Rule 3: Don’t’ use special symbols in a variable name
For declaring variable and constant, we cannot use special symbols like $, #, @, %, !~, etc. If we try to declare names with a special symbol, Python generates an error
Rule 4: Variable and constant should not start with digit letters.
You will receive an error if you start a variable name with a digit. Let’s verify this using a simple example.
Here Python will generate a syntax error at 1studnet . instead of this, you can declare a variable like studnet_1 = "Jessa"
Rule 5: Identifiers are case sensitive.
Here, Python makes a difference between these variables that is uppercase and lowercase, so that it will create three different variables total , Total , TOTAL .
Rule 6: To declare constant should use capital letters.
Rule 6: Use an underscore symbol for separating the words in a variable name
If we want to declare variable and constant names having two words, use an underscore symbol for separating the words.
Multiple assignments
In Python, there is no restriction to declare a variable before using it in the program. Python allows us to create a variable as and when required.
We can do multiple assignments in two ways, either by assigning a single value to multiple variables or assigning multiple values to multiple variables .
we can assign a single value to multiple variables simultaneously using the assignment operator = .
Now, let’s create an example to assign the single value 10 to all three variables a , b , and c .
In the above example, two integer values 10 and 70 are assigned to variables roll_no and marks , respectively, and string literal, “Jessa,” is assigned to the variable name .
Variable scope
Scope : The scope of a variable refers to the places where we can access a variable.
Depending on the scope, the variable can categorize into two types local variable and the global variable.
A local variable is a variable that is accessible inside a block of code only where it is declared. That means, If we declare a variable inside a method, the scope of the local variable is limited to the method only. So it is not accessible from outside of the method. If we try to access it, we will get an error.
In the above example, we created a function with the name test1 . Inside it, we created a local variable price. Similarly, we created another function with the name test2 and tried to access price, but we got an error "price is not defined" because its scope is limited to function test1() . This error occurs because we cannot access the local variable from outside the code block.
A Global variable is a variable that is defined outside of the method (block of code). That is accessible anywhere in the code file.
In the above example, we created a global variable price and tried to access it in test1 and test2 . In return, we got the same value because the global variable is accessible in the entire file.
Note : You must declare the global variable outside function.
Object/Variable identity and references
In Python, whenever we create an object, a number is given to it and uniquely identifies it. This number is nothing but a memory address of a variable’s value. Once the object is created, the identity of that object never changes.
No two objects will have the same identifier. The Object is for eligible garbage collection when deleted. Python has a built-in function id() to get the memory address of a variable.
For example, consider a library with many books (memory addresses) and many students (objects). At the beginning(when we start The Python program), all books are available. When a new student comes to the library (a new object created), the librarian gives him a book. Every book has a unique number (identity), and that id number tells us which book is delivered to the student (object)
It returns the same address location because both variables share the same value. But if we assign m to some different value, it points to a different object with a different identity.
See the following example
For m = 500 , Python created an integer object with the value 500 and set m as a reference to it. Similarly, n is assigned to an integer object with the value 400 and sets n as a reference to it. Both variables have different identities.
In Python, when we assign a value to a variable, we create an object and reference it.
For example, a=10 , here, an object with the value 10 is created in memory, and reference a now points to the memory address where the object is stored.
Suppose we created a=10 , b=10 , and c=10 , the value of the three variables is the same. Instead of creating three objects, Python creates only one object as 10 with references such as a , b , c .
We can access the memory addresses of these variables using the id() method. a , b refers to the same address in memory, and c , d , e refers to the same address. See the following example for more details.
Here, an object in memory initialized with the value 10 and reference added to it, the reference count increments by ‘1’.
When Python executes the next statement that is b=10 , since it is the same value 10, a new object will not be created because the same object in memory with value 10 available, so it has created another reference, b . Now, the reference count for value 10 is ‘2’.
Again for c=20 , a new object is created with reference ‘c’ since it has a unique value (20). Similarly, for d , and e new objects will not be created because the object ’20’ already available. Now, only the reference count will be incremented.
We can check the reference counts of every object by using the getrefcount function of a sys module. This function takes the object as an input and returns the number of references.
We can pass variable, name, value, function, class as an input to getrefcount() and in return, it will give a reference count for a given object.
See the following image for more details.
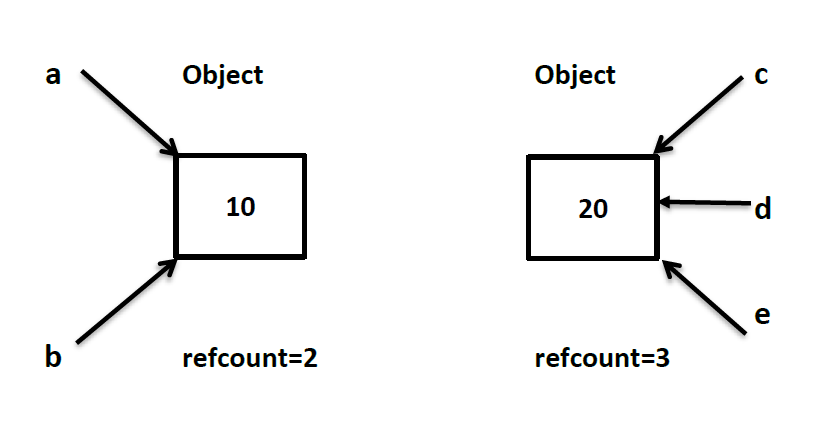
In the above picture, a , b pointing to the same memory address location (i.e., 140722211837248), and c , d , e pointing to the same memory address (i.e., 140722211837568 ). So reference count will be 2 and 3 respectively.
- In Python, we can create a tuple (or list) by packing a group of variables.
- Packing can be used when we want to collect multiple values in a single variable. Generally, this operation is referred to as tuple packing.
Here a , b , c , d are packed in the tuple tuple1 .
Tuple unpacking is the reverse operation of tuple packing . We can unpack tuple and assign tuple values to different variables.
Note: When we are performing unpacking, the number of variables and the number of values should be the same. That is, the number of variables on the left side of the tuple must exactly match a number of values on the right side of the tuple. Otherwise, we will get a ValueError .
Also, See :
- Class Variables
- Instance variables
Did you find this page helpful? Let others know about it. Sharing helps me continue to create free Python resources.
About Vishal

I’m Vishal Hule , the Founder of PYnative.com. As a Python developer, I enjoy assisting students, developers, and learners. Follow me on Twitter .
Related Tutorial Topics:
Python exercises and quizzes.
Free coding exercises and quizzes cover Python basics, data structure, data analytics, and more.
- 15+ Topic-specific Exercises and Quizzes
- Each Exercise contains 10 questions
- Each Quiz contains 12-15 MCQ
Loading comments... Please wait.
About PYnative
PYnative.com is for Python lovers. Here, You can get Tutorials, Exercises, and Quizzes to practice and improve your Python skills .
Explore Python
- Learn Python
- Python Basics
- Python Databases
- Python Exercises
- Python Quizzes
- Online Python Code Editor
- Python Tricks
To get New Python Tutorials, Exercises, and Quizzes
Legal Stuff
We use cookies to improve your experience. While using PYnative, you agree to have read and accepted our Terms Of Use , Cookie Policy , and Privacy Policy .
Copyright © 2018–2024 pynative.com
Variable Assignment
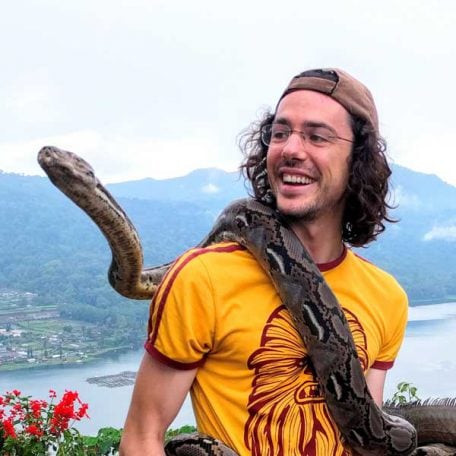
- Discussion (8)
Think of a variable as a name attached to a particular object . In Python, variables need not be declared or defined in advance, as is the case in many other programming languages. To create a variable, you just assign it a value and then start using it. Assignment is done with a single equals sign ( = ).
00:00 Welcome to this first section, where we’ll talk about variable assignments in Python. First of all, I just want to mention that I’m going to be using the IPython shell.
00:09 The reason for that is just that it adds a bit of colors to the prompt and makes it a bit easier to see which types we’re working with, but you don’t need to do this install that I’m going to show you in just a second.
00:19 You can just use your normal Python interpreter and it’s going to work all the same. If you want to install IPython, all you need to do is go to your terminal and type pip3 install ipython , press Enter, and then wait until it installs.
00:36 I already got it installed. And then instead of typing python to get into the interpreter, you’re going to type ipython .
00:46 It gives us the same functionality, only you see there’s some colors involved and it looks a bit nicer. I can do clear and clear my screen. So it’s going to make it a bit easier for you to follow, but that’s all.
00:58 So, first stop: a standard variable assignment in Python. Unlike other languages, in Python this is very simple. We don’t need to declare a variable, all we need to do is give it a name, put the equal sign ( = ) and then the value that we want to assign. That’s it.
01:15 That’s a variable assignment in Python. I just assigned the value 300 to the variable n . So now I can print(n) and get the result.
01:26 Or, since I’m in an interpreter session, I can just put in n and it shows me that the output is going to be 300 . So, that’s the basic, standard variable assignment that you’re going to do many times in Python.
01:38 And it’s nice that you don’t need to declare the variable before. You simply can type it in like this. Now the variable n is referring to the value 300 .
01:48 What happens if I change it? So, I don’t need to stick with 300 through the lifetime of this variable. I can just change it to something else. I can say “Now this is this going to be 400 .”
02:00 Or, in Python, not even the type is fixed, so I can say n = "hello" and change it to a string.
02:10 And this is still all working fine. So you see, it feels very fluid, and this is because Python is a dynamically-typed language, so we don’t need to define types and define variables beforehand and then they’re unchangeable for the rest of the program—but it’s fluid, and we can just start off with n being an integer of the value of 300 and through the lifetime of the program, it can take on a couple of different identities.
02:36 So, apart from the standard variable assignment that we just saw before, n = 300 , we can also use a chained assignment in Python, which makes it quick to assign a couple of variables to the same value.
02:49 And that looks like this.
02:52 I can say n = m = x = y et cetera, and then give it a value. And now all of those variable names point to 400 , so I can say m is 400 , x is 400 , y is 400 , et cetera. That’s what is called a chained assignment in Python.
03:15 Another way is the multiple assignment statement, or multiple assignments, which works a little bit different and there’s something you need to take care of, but I still want to introduce you to it. If you go ahead here, I can assign two values at the same time in one line.
03:32 So I can say a, b = 300, 400 . The comma ( , ) is important, and it’s important that the amount of variables that you’re declaring here on the left side is the same amount of values that you have on the right side.
03:48 I can do this, and now b points to 400 , a points to 300 .
03:54 It doesn’t have to be two, there can be more, but just make sure that every time if you use this multiple assignment statement, that the amount of variables you use left is the same as the amount of values on the right. And as a last point in this section, I want to talk a little bit about variable types.
04:14 I already mentioned that variable types don’t have to be fixed in Python. I can start off with
04:21 n pointing to 300 , which as we know is an integer. Remember, you can always check what the type of a variable is by just saying type() and passing in the variable in there.
04:33 So it gives me as an output that this is an int (integer).
04:37 This is just the same as saying “What’s the type() of 300 or 200 ?” directly— it’s an integer—because all that I’m passing in here is a reference to this object. We’ll talk about this more in the next section.
04:52 But now I can easily change the type of this variable, because all I’m doing is pointing it to a different object. So now n is pointing to a string.
05:01 If I say type(n) now, it will tell me it’s a str (string).
05:08 And the reason for this is that variables in Python are simply references to objects. In the next section, we’ll talk much more what’s important about that and how in Python everything is an object.
05:19 And that it for this section! Let’s do a quick recap. Variable assignments in Python are pretty straightforward. We have the standard variable assignment that just goes <variable name> = <your value> .
05:32 We have another way of doing it that’s called chained assignments, where we can assign a couple of variable names to the same value by just using multiple equal signs.
05:43 Then there’s the multiple assignment statement, which works a little differently, and you have to take care to use commas and the same amount of variable names on the left side as values on the right side.
05:53 It’s going to assign, as expected, n to 300 , m to 400 . And then finally, we talked about variable types, that they are fluid in Python and that you can check what the variable type is by using the type() function.
06:07 And here’s a nice thing to see also, that n is just a pointer to the 300 integer, because we’re going to get the same result if we say type(n) or type(300) .
06:18 They’re both int (integer) objects. And this is a concept that we’re going to talk about more in the upcoming section when we talk about object references. See you over there.
iamscottdavis on Dec. 10, 2019
I installed ipython on my chromebook but it won’t run.
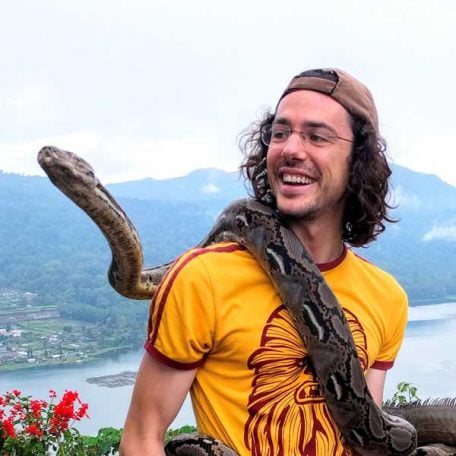
Martin Breuss RP Team on Dec. 10, 2019
You might have to close and re-open your terminal @iamscottdavis
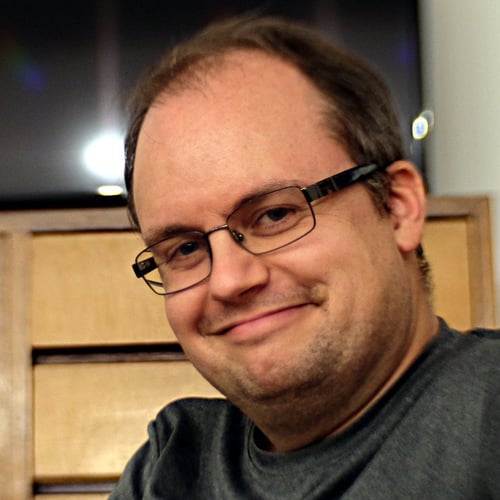
Geir Arne Hjelle RP Team on Dec. 10, 2019
I’ve recently had some weird issues with prompt_toolkit , one of the dependencies of IPython. Maybe that’s what you’re running into?
I got a cryptic error message like TypeError: __init__() got an unexpected keyword argument 'inputhook' . If this is your problem as well, the best solution should be to update to IPython >= 7.10 which should have fixed this. Another workaround is to downgrade prompt_toolkit to version 2.
See some discussion on github.com/ipython/ipython/issues/11975
If you are having other problems, feel free to post your error messages :)
kiran on July 18, 2020
if i declare the variable in any one loop in python.
now my question is a is local/global variable? in C it is local variable but what about python? in Python even i declare a variable with in the loop it become a global variable?
Martin Breuss RP Team on July 18, 2020
In Python, it will keep the last value it got assigned within the loop also outside in the global scope. That is why a is still accessible and has a value in your example, also outside of the loop’s scope.
DoubleA on Jan. 20, 2021
Hey Martin,
Thanks for pulling this stuff together and explaining it so clearly. I came accross some sort of a variation of the multiple assignment you discussed. Basically, it seems that the number of variable names and variables can be diffrent. What I mean is this:
then print(a,b,c) gives me the following output:
Am I right saying that, basically, what happens above is that the variable c having an asterisk before it will get assigned a list of the two values, incl. the excessive (‘string’) one?
Referring back to Kiran’s question:
When I run this code:
I see that the globals() function returns, amongst other things, the value of the variables a , b and the last value of the iterable elem . Both variables a and b appear to be visible in the global scope as key-value pairs of the following dict:
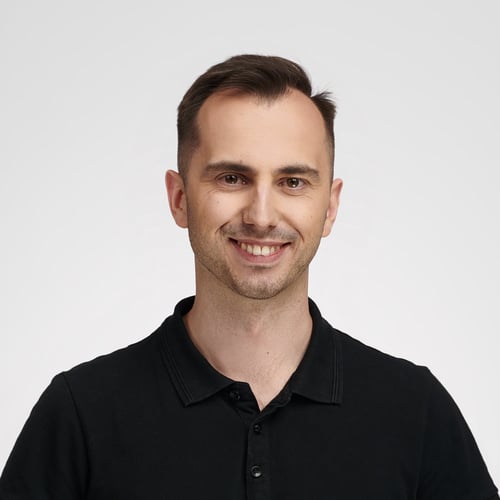
Bartosz Zaczyński RP Team on Jan. 21, 2021
@DoubleA The “starred” expression syntax you were referring to before can be used for extended iterable unpacking .
Become a Member to join the conversation.
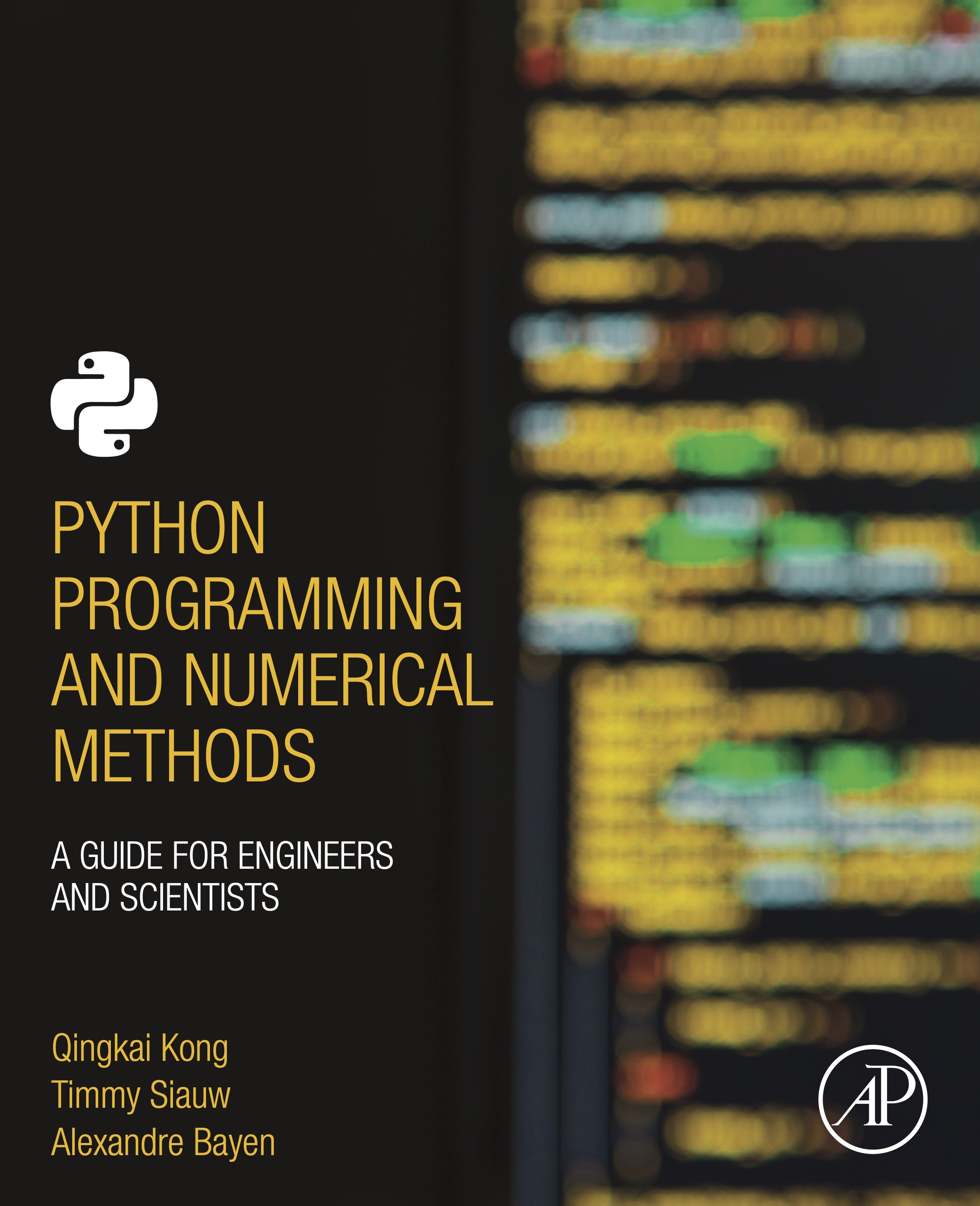
Python Numerical Methods

This notebook contains an excerpt from the Python Programming and Numerical Methods - A Guide for Engineers and Scientists , the content is also available at Berkeley Python Numerical Methods .
The copyright of the book belongs to Elsevier. We also have this interactive book online for a better learning experience. The code is released under the MIT license . If you find this content useful, please consider supporting the work on Elsevier or Amazon !
< 2.0 Variables and Basic Data Structures | Contents | 2.2 Data Structure - Strings >
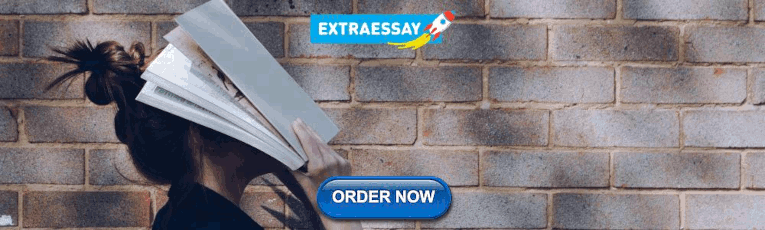
Variables and Assignment ¶
When programming, it is useful to be able to store information in variables. A variable is a string of characters and numbers associated with a piece of information. The assignment operator , denoted by the “=” symbol, is the operator that is used to assign values to variables in Python. The line x=1 takes the known value, 1, and assigns that value to the variable with name “x”. After executing this line, this number will be stored into this variable. Until the value is changed or the variable deleted, the character x behaves like the value 1.
TRY IT! Assign the value 2 to the variable y. Multiply y by 3 to show that it behaves like the value 2.
A variable is more like a container to store the data in the computer’s memory, the name of the variable tells the computer where to find this value in the memory. For now, it is sufficient to know that the notebook has its own memory space to store all the variables in the notebook. As a result of the previous example, you will see the variable “x” and “y” in the memory. You can view a list of all the variables in the notebook using the magic command %whos .
TRY IT! List all the variables in this notebook
Note that the equal sign in programming is not the same as a truth statement in mathematics. In math, the statement x = 2 declares the universal truth within the given framework, x is 2 . In programming, the statement x=2 means a known value is being associated with a variable name, store 2 in x. Although it is perfectly valid to say 1 = x in mathematics, assignments in Python always go left : meaning the value to the right of the equal sign is assigned to the variable on the left of the equal sign. Therefore, 1=x will generate an error in Python. The assignment operator is always last in the order of operations relative to mathematical, logical, and comparison operators.
TRY IT! The mathematical statement x=x+1 has no solution for any value of x . In programming, if we initialize the value of x to be 1, then the statement makes perfect sense. It means, “Add x and 1, which is 2, then assign that value to the variable x”. Note that this operation overwrites the previous value stored in x .
There are some restrictions on the names variables can take. Variables can only contain alphanumeric characters (letters and numbers) as well as underscores. However, the first character of a variable name must be a letter or underscores. Spaces within a variable name are not permitted, and the variable names are case-sensitive (e.g., x and X will be considered different variables).
TIP! Unlike in pure mathematics, variables in programming almost always represent something tangible. It may be the distance between two points in space or the number of rabbits in a population. Therefore, as your code becomes increasingly complicated, it is very important that your variables carry a name that can easily be associated with what they represent. For example, the distance between two points in space is better represented by the variable dist than x , and the number of rabbits in a population is better represented by nRabbits than y .
Note that when a variable is assigned, it has no memory of how it was assigned. That is, if the value of a variable, y , is constructed from other variables, like x , reassigning the value of x will not change the value of y .
EXAMPLE: What value will y have after the following lines of code are executed?
WARNING! You can overwrite variables or functions that have been stored in Python. For example, the command help = 2 will store the value 2 in the variable with name help . After this assignment help will behave like the value 2 instead of the function help . Therefore, you should always be careful not to give your variables the same name as built-in functions or values.
TIP! Now that you know how to assign variables, it is important that you learn to never leave unassigned commands. An unassigned command is an operation that has a result, but that result is not assigned to a variable. For example, you should never use 2+2 . You should instead assign it to some variable x=2+2 . This allows you to “hold on” to the results of previous commands and will make your interaction with Python must less confusing.
You can clear a variable from the notebook using the del function. Typing del x will clear the variable x from the workspace. If you want to remove all the variables in the notebook, you can use the magic command %reset .
In mathematics, variables are usually associated with unknown numbers; in programming, variables are associated with a value of a certain type. There are many data types that can be assigned to variables. A data type is a classification of the type of information that is being stored in a variable. The basic data types that you will utilize throughout this book are boolean, int, float, string, list, tuple, dictionary, set. A formal description of these data types is given in the following sections.
5 Best Ways to Declare a Variable in Python
💡 Problem Formulation: When starting with Python programming, a common task is to store values in variables. Consider the need to track the score in a game or the need to store user input. This article walks through the various methods of declaring variables in Python to store such pieces of information effectively.
Method 1: Basic Variable Assignment
This is the most straightforward method for creating and assigning a variable in Python. You simply declare a variable by typing its name, followed by an equals sign, and the value you wish to assign to it. This method is readable, writable, and often used for its simplicity.
Here’s an example:
The line score = 10 initializes a variable called score with the integer value 10 . This variable can now be used in other parts of a Python program to represent the score in a game.
Method 2: Assignment with Type Annotation (Python 3.6+)
Type annotations allow the programmer to explicitly state the expected data type of a variable. Python 3.6 introduced this capability, which aids in code readability and can help with type checking when using tools like MyPy.
In the example username: str = "PlayerOne" , we declare a variable username with the type annotation str , which indicates it should store a string, and we assign it the value “PlayerOne”. Type annotations are optional in Python but can be very informative.
Method 3: Multiple Assignment
Python allows you to assign multiple variables at once in a single line. This can be cleaner and more efficient when initializing several variables that may relate to each other.
In the snippet x, y, z = 1, 2, 3 , we simultaneously create three variables x , y , and z , assigning them the values 1 , 2 , and 3 respectively. This is a compact way of declaring related variables.
Method 4: Unpacking a Sequence
When you have a list or tuple, you can “unpack” its values into separate variables. This is particularly useful when the sequence size is known, and you want to assign its elements to named variables.
Here, coordinates is a tuple with two elements. The line x, y = coordinates unpacks those elements into variables x and y . This results in x having the value of 10 and y the value of 20 .
Bonus One-Liner Method 5: Variable Assignment with Chained Operators
Python permits chaining assignment operators to assign the same value to multiple variables in a single line. This method is a one-liner that makes the code concise when initial values are the same.
The line a = b = c = 0 initializes the variables a , b , and c to 0 . Now all three variables can be used independently, yet they start with the same initial value.
Summary/Discussion
- Method 1: Basic Variable Assignment. Simple and universally applicable. No inherent weaknesses except lack of type declaration.
- Method 2: Assignment with Type Annotation. Great for code clarity and for use with static type checkers. Not supported in versions of Python before 3.6.
- Method 3: Multiple Assignment. Efficient for declaring multiple variables at once, but may lead to reduced readability if overused.
- Method 4: Unpacking a Sequence. Clean and elegant, provided that the structure of the sequence is known beforehand. Not practical for variable-length sequences.
- Bonus Method 5: Variable Assignment with Chained Operators. Handy for initializing multiple variables with the same value. Can be confusing if used beyond simple initializations.
Python Variables and Assignment
Python variables, variable assignment rules, every value has a type, memory and the garbage collector, variable swap, variable names are superficial labels, assignment = is shallow, decomp by var.
Python Tutorial
File handling, python modules, python numpy, python pandas, python matplotlib, python scipy, machine learning, python mysql, python mongodb, python reference, module reference, python how to, python examples, python variables - assign multiple values, many values to multiple variables.
Python allows you to assign values to multiple variables in one line:
Note: Make sure the number of variables matches the number of values, or else you will get an error.
One Value to Multiple Variables
And you can assign the same value to multiple variables in one line:
Unpack a Collection
If you have a collection of values in a list, tuple etc. Python allows you to extract the values into variables. This is called unpacking .
Unpack a list:
Learn more about unpacking in our Unpack Tuples Chapter.
Video: Python Variable Names

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.

The FinAnalytics

Financial Book
Interview guide, recommendations, understanding variables in python: declaration, assignment, and naming conventions.
Variables are essential elements in programming languages like Python. they serve as containers to store data values that can be manipulated or referenced in a program. Understanding how variables are declared, assigned values, and named according to conventions is crucial for writing clean and readable Python code.
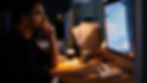
Variable Declaration and Assignment in Python
In Python, variables are declared by simply assigning a value to them. Unlike some other programming languages, Python does not require explicit declaration of variables or specifying their data types. the variable's data type is inferred based on the value assigned to it. this process is known as variable assignment, which can be done using the equals sign "=" (also known as the assignment operator). Once the value is assigned to a variable, it is created, and we can start using it in other statements or expressions.
for instance, the following code snippet creates a variable named " stockPrice " and assigns the value 100 (an integer) to it.

the variable stockPrice is now created and holds the value 100 . You can use it in other expressions or statements as shown below.

Variables in Python can be reassigned to different values, allowing for dynamic changes in a program. A variable's value can be updated by simply assigning a new value to it. You can re-declare a variable by assigning a new value to it. for instance, we can change the value of the " stockPrice " variable to 150 as follows:

You can also assign the values to multiple variables simultaneously using the chaining assignment operation as shown below.

the simultaneous assignment operation in Python provides programmers with a concise and efficient way to assign values to multiple variables in a single line of code.
Naming Conventions for Variables
While naming variables in Python, it is essential to follow proper naming conventions for code clarity and maintainability. Here are some guidelines to follow when naming variables:
Start with a Letter or Underscore: Variable names must begin with a letter (a-z, A-Z) or an underscore (_) character. for example: "stockPrice" or "_stockPrice" are acceptable variable names.
Avoid Starting with a Number: Variable names cannot begin with a number. for example: "1stockPrice" or "1_stockPrice" are invalid variable names.
Use Alphanumeric Characters and Underscores: Variable names can only contain alphanumeric characters (A-Z, a-z, 0-9) and underscores (_). for example: "stockPrice", "stock_Price", "stockPrice_1", and "stockPrice_2" are all valid variable names.
Avoid Whitespace and Special Characters: Variable names should not contain whitespace or special characters such as +, -, etc. for example: "stock price" or "stock-price" are invalid variable names.
Case Sensitive: Variable names are case-sensitive. for example: "StockPrice", "stockPrice", and "Stockprice" are considered distinct variables.
Avoid Python Keywords: Avoid using Python keywords as variable names. for example: keywords such as "str", "is", and "for" cannot be used as variable names as they are reserved keywords in Python.
In addition to these guidelines, professional programmers follow certain conventions to enhance code readability (best practices). these practices include using a name that describes the purpose " stockPrice ", instead of using dummy or temporary names " temp ". It's also common practice to separate words in variable names with underscores " stock_price ", and start variable names with lowercase letters " stockPrice ".
Following these guidelines and practices can make your code more readable and maintainable. Remember that these are good coding practices recommended by professional programmers, which can be applied to any programming language.
- Python Course
- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
Different Forms of Assignment Statements in Python
We use Python assignment statements to assign objects to names. The target of an assignment statement is written on the left side of the equal sign (=), and the object on the right can be an arbitrary expression that computes an object.
There are some important properties of assignment in Python :-
- Assignment creates object references instead of copying the objects.
- Python creates a variable name the first time when they are assigned a value.
- Names must be assigned before being referenced.
- There are some operations that perform assignments implicitly.
Assignment statement forms :-
1. Basic form:
This form is the most common form.
2. Tuple assignment:
When we code a tuple on the left side of the =, Python pairs objects on the right side with targets on the left by position and assigns them from left to right. Therefore, the values of x and y are 50 and 100 respectively.
3. List assignment:
This works in the same way as the tuple assignment.
4. Sequence assignment:
In recent version of Python, tuple and list assignment have been generalized into instances of what we now call sequence assignment – any sequence of names can be assigned to any sequence of values, and Python assigns the items one at a time by position.
5. Extended Sequence unpacking:
It allows us to be more flexible in how we select portions of a sequence to assign.
Here, p is matched with the first character in the string on the right and q with the rest. The starred name (*q) is assigned a list, which collects all items in the sequence not assigned to other names.
This is especially handy for a common coding pattern such as splitting a sequence and accessing its front and rest part.
6. Multiple- target assignment:
In this form, Python assigns a reference to the same object (the object which is rightmost) to all the target on the left.
7. Augmented assignment :
The augmented assignment is a shorthand assignment that combines an expression and an assignment.
There are several other augmented assignment forms:
Please Login to comment...
Similar reads.
- Python Programs
- python-basics
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Comma separated variable assignment
Can someone please explain to me what line 4 is doing?

2 Answers 2
What you describe is tuple assignment :
is equivalent to a = 0 and b = 1 .
It can however have interesting effects if you for instance want to swap values. Like:
will first construct a tuple (b,a) and then untuple it and assign it to a and b . This is thus not equivalent to:
but to (using a temporary):
In general if you have a comma-separated list of variables left of the assignment operator and an expression that generates a tuple, the tuple is unpacked and stored in the values. So:
will assign 1 to a , 'a' to b and None to c . Note that this is not syntactical sugar: the compiler does not look whether the number of variables on the left is the same as the length of the tuple on the right, so you can return tuples from functions, etc. and unpack them in separate variables.

- 2 I've always known it as tuple packing/unpacking, or more generally sequence unpacking . See Tuples and Sequences – Open AI - Opting Out Commented Jan 12, 2017 at 14:37
- Remember functions and class methods can return tuples too. For example: def myfunc(): return 2,4 can be used as a, b = myfunc() which will make a = 2 and b = 4 or myfunc()[0] which will return 2 . – Andrej Žukov-Gregorič Commented Jan 12, 2017 at 15:01
- 1 @AndrejŽukov-Gregorič: that's why in the last code fragment I explicitly first constructed a tuple and stored it into t : such that one can reason this is no syntactical sugar, but actually tuple unpacking. – willeM_ Van Onsem Commented Jan 12, 2017 at 15:03
For the purposes of reading, all it's doing is setting a and b , so that a = 0 and b = 1. Similarly, in line 7, it's setting a to b and b to the sum of a and b .
More specifically, it's setting tuples. Tuples are invariant, in that once they're created, their values can't change. Tuples are pervasive in python - you see them almost everywhere.
Typically, you would expect a tuple to be in parenthesis, e.g. (a, b) = (0, 1) would read more cleanly, but they are such a large feature of python that the parenthesis are optional (unless you're constructing a tuple as an argument to a function, and then you need the extra parenthesis to differentiate between a single tuple and multiple arguments. I.e. you would have to say foo((a, b)) to pass a tuple to foo, as foo(a, b) would pass two arguments to it.)
Tuples can be any length. You can write a, b, c, d, e = 0, 1, 2, 3, 4 , or you can have a function return a tuple, e.g.: ret1, ret2, ret3 = foobar(1)

Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged python python-3.x or ask your own question .
- The Overflow Blog
- Unpacking the 2024 Developer Survey results
- Featured on Meta
- We've made changes to our Terms of Service & Privacy Policy - July 2024
- Introducing an accessibility dashboard and some upcoming changes to display...
- Tag hover experiment wrap-up and next steps
Hot Network Questions
- Compatibility of SD-Card-Readers with iPads or iPhones
- Why did all countries converge on the exact same rules for voting (no poll tax, full suffrage, no maximum age, etc)?
- What happens if your child sells your car?
- Where is the Minor Reflection spell?
- commands execution based on file size fails with no apparent issues
- Does the volume of air in an air tight container affect food shelf life?
- When are these base spaces isomorphic?
- Adjust circle radius by spline parameter
- What is the anti-trust argument made by X Corp's recent lawsuit against advertisers that boycotted X/Twitter
- How do we know that the number of points on a line is uncountable?
- If it's true that a reservation fee for a cruise ship is non-refundable, then what happens if somebody cancels?
- When can a citizen's arrest of an Interpol fugitive be legal in Washington D.C.?
- "Continuity" in a metric space
- How can I append comma except lastline?
- Can a wizard learn a spell from a cleric or druid?
- Is threatening to go to the police blackmailing?
- Typescript Implementation for a fully typed map with all keys from a string union type and values to be function with return type specific to key
- How important is a "no reflection" strategy for 1 Hz systems?
- dealing with the feeling after rejection
- In Europe, are you allowed to enter an intersection on red light in order to allow emergency vehicles to pass?
- Elastic Banach spaces without C[0,1]
- Conservation of energy in a mechanical system with a discontinuous potential function
- Generalized Super-Luhn
- Fantasy book in which a joker wonders what's at the top of a stone column
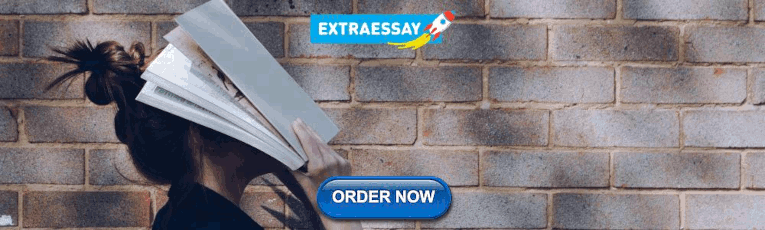
IMAGES
COMMENTS
To create a variable, you just assign it a value and then start using it. Assignment is done with a single equals sign ( = ): Python. >>> n = 300. This is read or interpreted as " n is assigned the value 300 .". Once this is done, n can be used in a statement or expression, and its value will be substituted: Python.
Example Get your own Python Server. x = 5. y = "John". print(x) print(y) Try it Yourself ». Variables do not need to be declared with any particular type, and can even change type after they have been set.
Why not just do this: var = None. Python is dynamic, so you don't need to declare things; they exist automatically in the first scope where they're assigned. So, all you need is a regular old assignment statement as above. This is nice, because you'll never end up with an uninitialized variable.
Python Variable is containers that store values. Python is not "statically typed". We do not need to declare variables before using them or declare their type. A variable is created the moment we first assign a value to it. A Python variable is a name given to a memory location. It is the basic unit of storage in a program.
Just assign a value to a variable using the = operator e.g. variable_name = value. That's it. The following creates a variable with the integer value. Example: Declare a Variable in Python. num = 10. Try it. In the above example, we declared a variable named num and assigned an integer value 10 to it.
In Python, variables are created the moment you give or assign a value to them. How Do I Assign a Value to a Variable? Assigning a value to a variable in Python is an easy process. You simply use the equal sign = as an assignment operator, followed by the value you want to assign to the variable. Here's an example:
Python allows assigning a single value to several variables simultaneously. a = b = c = 10print(a, b, c) # 10 10 10x = y = z = "Hello"print(x, y, z) # Hello Hello Hello. This is called multiple assignment and works by assigning the value to the first variable, then linking the rest to it. All variables refer to the same object in memory.
Here, variable represents a generic Python variable, while expression represents any Python object that you can provide as a concrete value—also known as a literal—or an expression that evaluates to a value. To execute an assignment statement like the above, Python runs the following steps: Evaluate the right-hand expression to produce a concrete value or object.
The declaration and assignment of constant in Python done with the module. Module means Python file (.py) which contains variables, functions, and packages. So let's create two modules, constant.py and main.py, respectively. In the constant.py file, we will declare two constant variables, PI and TOTAL_AREA. import constant module In main.py file.
00:58 So, first stop: a standard variable assignment in Python. Unlike other languages, in Python this is very simple. We don't need to declare a variable, all we need to do is give it a name, put the equal sign (=) and then the value that we want to assign. That's it. 01:15 That's a variable assignment in Python.
Variables and Assignment¶. When programming, it is useful to be able to store information in variables. A variable is a string of characters and numbers associated with a piece of information. The assignment operator, denoted by the "=" symbol, is the operator that is used to assign values to variables in Python.The line x=1 takes the known value, 1, and assigns that value to the variable ...
Method 1: Basic Variable Assignment. This is the most straightforward method for creating and assigning a variable in Python. You simply declare a variable by typing its name, followed by an equals sign, and the value you wish to assign to it. This method is readable, writable, and often used for its simplicity.
Python Variables. A Python variable is a named bit of computer memory, keeping track of a value as the code runs. A variable is created with an "assignment" equal sign =, with the variable's name on the left and the value it should store on the right: x = 42 In the computer's memory, each variable is like a box, identified by the name of the ...
W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more.
Python assigns values from right to left. When assigning multiple variables in a single line, different variable names are provided to the left of the assignment operator separated by a comma. The same goes for their respective values except they should be to the right of the assignment operator. While declaring variables in this fashion one ...
In Python, variables are declared by simply assigning a value to them. Unlike some other programming languages, Python does not require explicit declaration of variables or specifying their data types. the variable's data type is inferred based on the value assigned to it. this process is known as variable assignment, which can be done using the equals sign "=" (also known as the assignment ...
An assignment operator is an operator that is used to assign some value to a variable. Like normally in Python, we write "a = 5" to assign value 5 to variable 'a'. Augmented assignment operators have a special role to play in Python programming. It basically combines the functioning of the arithmetic or bitwise operator with the assignment operator
See PEP 526 -- Syntax for Variable Annotations, and What's new in Python 3.6: Just as for function annotations, the Python interpreter does not attach any particular meaning to variable annotations and only stores them in the __annotations__ attribute of a class or module.
Mass variable declaration and assignment in Python. Ask Question Asked 13 years, 3 months ago. Modified 4 years, 3 months ago. Viewed 4k times ... Python Variable Declaration. 0. Variable assignment in python. 1. Assign Many Variables at Once, Python. 2. Pythonic Variable Assignment. 1.
Edit: Python 3.5 introduced type hints which introduced a way to specify the type of a variable. This answer was written before this feature became available. There is no way to declare variables in Python, since neither "declaration" nor "variables" in the C sense exist. This will bind the three names to the same object: x = y = z = 0
a = b. b = t. In general if you have a comma-separated list of variables left of the assignment operator and an expression that generates a tuple, the tuple is unpacked and stored in the values. So: t = (1,'a',None) a,b,c = t. will assign 1 to a, 'a' to b and None to c. Note that this is not syntactical sugar: the compiler does not look whether ...