VHDL Logical Operators and Signal Assignments for Combinational Logic
In this post, we discuss the VHDL logical operators, when-else statements , with-select statements and instantiation . These basic techniques allow us to model simple digital circuits.
In a previous post in this series, we looked at the way we use the VHDL entity, architecture and library keywords. These are important concepts which provide structure to our code and allow us to define the inputs and outputs of a component.
However, we can't do anything more than define inputs and outputs using this technique. In order to model digital circuits in VHDL, we need to take a closer look at the syntax of the language.
There are two main classes of digital circuit we can model in VHDL – combinational and sequential .
Combinational logic is the simplest of the two, consisting primarily of basic logic gates , such as ANDs, ORs and NOTs. When the circuit input changes, the output changes almost immediately (there is a small delay as signals propagate through the circuit).
Sequential circuits use a clock and require storage elements such as flip flops . As a result, changes in the output are synchronised to the circuit clock and are not immediate. We talk more specifically about modelling combinational logic in this post, whilst sequential logic is discussed in the next post.
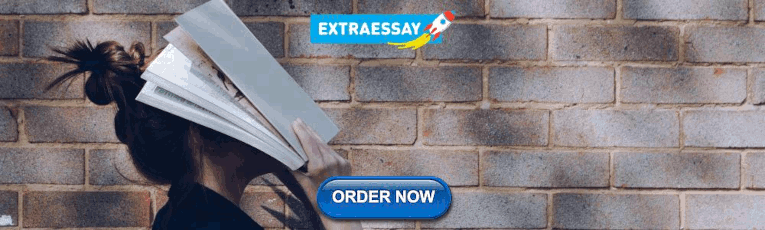
Combinational Logic
The simplest elements to model in VHDL are the basic logic gates – AND, OR, NOR, NAND, NOT and XOR.
Each of these type of gates has a corresponding operator which implements their functionality. Collectively, these are known as logical operators in VHDL.
To demonstrate this concept, let us consider a simple two input AND gate such as that shown below.
The VHDL code shown below uses one of the logical operators to implement this basic circuit.
Although this code is simple, there are a couple of important concepts to consider. The first of these is the VHDL assignment operator (<=) which must be used for all signals. This is roughly equivalent to the = operator in most other programming languages.
In addition to signals, we can also define variables which we use inside of processes. In this case, we would have to use a different assignment operator (:=).
It is not important to understand variables in any detail to model combinational logic but we talk about them in the post on the VHDL process block .
The type of signal used is another important consideration. We talked about the most basic and common VHDL data types in a previous post.
As they represent some quantity or number, types such as real, time or integer are known as scalar types. We can't use the VHDL logical operators with these types and we most commonly use them with std_logic or std_logic_vectors.
Despite these considerations, this code example demonstrates how simple it is to model basic logic gates.
We can change the functionality of this circuit by replacing the AND operator with one of the other VHDL logical operators.
As an example, the VHDL code below models a three input XOR gate.
The NOT operator is slightly different to the other VHDL logical operators as it only has one input. The code snippet below shows the basic syntax for a NOT gate.
- Mixing VHDL Logical Operators
Combinational logic circuits almost always feature more than one type of gate. As a result of this, VHDL allows us to mix logical operators in order to create models of more complex circuits.
To demonstrate this concept, let’s consider a circuit featuring an AND gate and an OR gate. The circuit diagram below shows this circuit.
The code below shows the implementation of this circuit using VHDL.
This code should be easy to understand as it makes use of the logical operators we have already talked about. However, it is important to use brackets when modelling circuits with multiple logic gates, as shown in the above example. Not only does this ensure that the design works as intended, it also makes the intention of the code easier to understand.
- Reduction Functions
We can also use the logical operators on vector types in order to reduce them to a single bit. This is a useful feature as we can determine when all the bits in a vector are either 1 or 0.
We commonly do this for counters where we may want to know when the count reaches its maximum or minimum value.
The logical reduction functions were only introduced in VHDL-2008. Therefore, we can not use the logical operators to reduce vector types to a single bit when working with earlier standards.
The code snippet below shows the most common use cases for the VHDL reduction functions.
Mulitplexors in VHDL
In addition to logic gates, we often use multiplexors (mux for short) in combinational digital circuits. In VHDL, there are two different concurrent statements which we can use to model a mux.
The VHDL with select statement, also commonly referred to as selected signal assignment, is one of these constructs.
The other method we can use to concurrently model a mux is the VHDL when else statement.
In addition to this, we can also use a case statement to model a mux in VHDL . However, we talk about this in more detail in a later post as this method also requires us to have an understanding of the VHDL process block .
Let's look at the VHDL concurrent statements we can use to model a mux in more detail.
VHDL With Select Statement
When we use the with select statement in a VHDL design, we can assign different values to a signal based on the value of some other signal in our design.
The with select statement is probably the most intuitive way of modelling a mux in VHDL.
The code snippet below shows the basic syntax for the with select statement in VHDL.
When we use the VHDL with select statement, the <mux_out> field is assigned data based on the value of the <address> field.
When the <address> field is equal to <address1> then the <mux_out> signal is assigned to <a>, for example.
We use the the others clause at the end of the statement to capture instance when the address is a value other than those explicitly listed.
We can exclude the others clause if we explicitly list all of the possible input combinations.
- With Select Mux Example
Let’s consider a simple four to one multiplexer to give a practical example of the with select statement. The output Q is set to one of the four inputs (A,B, C or D) depending on the value of the addr input signal.
The circuit diagram below shows this circuit.
This circuit is simple to implement using the VHDL with select statement, as shown in the code snippet below.
VHDL When Else Statements
We use the when statement in VHDL to assign different values to a signal based on boolean expressions .
In this case, we actually write a different expression for each of the values which could be assigned to a signal. When one of these conditions evaluates as true, the signal is assigned the value associated with this condition.
The code snippet below shows the basic syntax for the VHDL when else statement.
When we use the when else statement in VHDL, the boolean expression is written after the when keyword. If this condition evaluates as true, then the <mux_out> field is assigned to the value stated before the relevant when keyword.
For example, if the <address> field in the above example is equal to <address1> then the value of <a> is assigned to <mux_out>.
When this condition evaluates as false, the next condition in the sequence is evaluated.
We use the else keyword to separate the different conditions and assignments in our code.
The final else statement captures the instances when the address is a value other than those explicitly listed. We only use this if we haven't explicitly listed all possible combinations of the <address> field.
- When Else Mux Example
Let’s consider the simple four to one multiplexer again in order to give a practical example of the when else statement in VHDL. The output Q is set to one of the four inputs (A,B, C or D) based on the value of the addr signal. This is exactly the same as the previous example we used for the with select statement.
The VHDL code shown below implements this circuit using the when else statement.
- Comparison of Mux Modelling Techniques in VHDL
When we write VHDL code, the with select and when else statements perform the same function. In addition, we will get the same synthesis results from both statements in almost all cases.
In a purely technical sense, there is no major advantage to using one over the other. The choice of which one to use is often a purely stylistic choice.
When we use the with select statement, we can only use a single signal to determine which data will get assigned.
This is in contrast to the when else statements which can also include logical descriptors.
This means we can often write more succinct VHDL code by using the when else statement. This is especially true when we need to use a logic circuit to drive the address bits.
Let's consider the circuit shown below as an example.
To model this using a using a with select statement in VHDL, we would need to write code which specifically models the AND gate.
We must then include the output of this code in the with select statement which models the multiplexer.
The code snippet below shows this implementation.
Although this code would function as needed, using a when else statement would give us more succinct code. Whilst this will have no impact on the way the device works, it is good practice to write clear code. This help to make the design more maintainable for anyone who has to modify it in the future.
The VHDL code snippet below shows the same circuit implemented with a when else statement.
Instantiating Components in VHDL
Up until this point, we have shown how we can use the VHDL language to describe the behavior of circuits.
However, we can also connect a number of previously defined VHDL entity architecture pairs in order to build a more complex circuit.
This is similar to connecting electronic components in a physical circuit.
There are two methods we can use for this in VHDL – component instantiation and direct entity instantiation .
- VHDL Component Instantiation
When using component instantiation in VHDL, we must define a component before it is used.
We can either do this before the main code, in the same way we would declare a signal, or in a separate package.
VHDL packages are similar to headers or libraries in other programming languages and we discuss these in a later post.
When writing VHDL, we declare a component using the syntax shown below. The component name and the ports must match the names in the original entity.
After declaring our component, we can instantiate it within an architecture using the syntax shown below. The <instance_name> must be unique for every instantiation within an architecture.
In VHDL, we use a port map to connect the ports of our component to signals in our architecture.
The signals which we use in our VHDL port map, such as <signal_name1> in the example above, must be declared before they can be used.
As VHDL is a strongly typed language, the signals we use in the port map must also match the type of the port they connect to.
When we write VHDL code, we may also wish to leave some ports unconnected.
For example, we may have a component which models the behaviour of a JK flip flop . However, we only need to use the inverted output in our design meaning. Therefore, we do not want to connect the non-inverted output to a signal in our architecture.
We can use the open keyword to indicate that we don't make a connection to one of the ports.
However, we can only use the open VHDL keyword for outputs.
If we attempt to leave inputs to our components open, our VHDL compiler will raise an error.
- VHDL Direct Entity Instantiation
The second instantiation technique is known as direct entity instantiation.
Using this method we can directly connect the entity in a new design without declaring a component first.
The code snippet below shows how we use direct entity instantiation in VHDL.
As with the component instantiation technique, <instance_name> must be unique for each instantiation in an architecture.
There are two extra requirements for this type of instantiation. We must explicitly state the name of both the library and the architecture which we want to use. This is shown in the example above by the <library_name> and <architecture_name> labels.
Once the component is instantiated within a VHDL architecture, we use a port map to connect signals to the ports. We use the VHDL port map in the same way for both direct entity and component instantiation.
Which types can not be used with the VHDL logical operators?
Scalar types such as integer and real.
Write the code for a 4 input NAND gate
We can use two different types of statement to model multiplexors in VHDL, what are they?
The with select statement and the when else statement
Write the code for an 8 input multiplexor using both types of statement
Write the code to instantiate a two input AND component using both direct entity and component instantiation. Assume that the AND gate is compiled in the work library and the architecture is named rtl.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
Table of Contents
Sign up free for exclusive content.
Don't Miss Out
We are about to launch exclusive video content. Sign up to hear about it first.
Testbenches in VHDL – A complete guide with steps
Whenever we design a circuit or a system, one step that is most important is “testing”. Testing is necessary to verify whether the designed system works as expected or not.
We can, of course, opt to test an IC after fabrication. But that would be, to put it delicately, stupid. If we find some error in an IC after fabrication, we are looking at a great loss because now we have to re-do the entire chip manufacturing process from scratch. Right from designing the circuit to fabrication.
Hence, the chip design methodology has testing after every phase. You can read about the design flow of manufacturing chips and ASICs here . Testbenches are used to test the RTL (Register-transfer logic) that we implement using HDL languages like Verilog and VHDL.
To summarize, we first design the circuit using HDL, then we verify it using a testbench. Only after this can we move on to the next step in the chip design workflow.
What is a testbench?
The testbench is also an HDL code. We write testbenches to inject input sequences to input ports and read values from output ports of the module. The module (or electronic circuit) we are testing is called a DUT or a Device Under Test. Testing of a DUT is very similar to the physical lab testing of digital chips. There we use sequence generators for input and probe the output to a capturing device. And here we do the same thing virtually.
What is a DUT(Device under test)?
How does a testbench work.
A testbench is specific for a DUT. It contains a blank entity and its architecture. Now, you may think “A blank entity,” what does it mean?
The next step is to generate a stimulus, or you may say sequences for inputs. We have two ways to generate an in-program stimulus.
In-program stimulus generation
Using repetitive patterns.
The above lines of code only represent one input combination. We have to repeat this fifteen times more for each set of inputs. That would look fine, but just imagine if we have to write this for a 32×1 MUX. That will make the testbench much longer. There surely has to be a better way right? Yes!
Using vectors
Both methods accomplish the same task. One is just a shorter and easier way of doing it.
Types of testbench in VHDL
The ‘simple testbench’ and the ‘testbench with a process’ types are more suitable for combinational circuits. We will be writing one example of each type for the same DUT so that you can compare them and understand them better.
Let’s write a simple testbench for the above code.
Simple testbench
We start writing the testbench by including the library and using its necessary packages. It is the same as the DUT.
As discussed earlier, testbench is also a VHDL program, so it follows all rules and ethics of VHDL programming.
We declare a component(DUT) and signals in its architecture before begin keyword.
Now we have to port map the internal signals and ports of the DUT to enable the testbench to inject input to the DUT and read its output.
Only after a delay, we give the next set of values. We do this to ensure that we give proper time, for output to become stable and observable.
Testbench with a process
The structure of the testbench always remains the same, just the architecture changes according to what method we use.
After that delay, we verify the output by comparing them with expected values. We do this using the assert statement.
What would happen in the case of an error? Let’s try to assert incorrect values to cause an error. This is the message that gets displayed.
Now let’s look at the other types of testbench that are more suitable for sequential circuits.
Infinite testbench
We use infinite testbenches to test sequential circuits, mainly due to the reason that they allow using a clock.
We can, of course, limit the number of clock cycles for simulation purposes. In fact, we will do that in the next testbench.
The structure of the testbench remains the same from the previous types. The libraries, the usage of packages, creating a blank entity, and declaring components inside the architecture is all done in the same way. Here’s the difference. After declaring the components, we define a constant for storing delay time and some signals.
After that, we create a process (‘clock’ here) to generate a clock of period T/2.
Finite testbench
This injects the stimulus only up to a certain number of times which we specify in the program.
After that, we define some internal signals for data injection. Two constants, one for time and one to keep a record of no. of clocks that makes this testbench finite.
Then again we do port mapping, after the begin keyword.
Now, we create another process to inject inputs. And, it will also stop when ‘i’ will reach the predefined number of the clock cycles.
That sums up all the normal testbenches in VHDL. The comment box below awaits your queries if you have any.
Related courses to Testbenches in VHDL – A complete guide with steps
Verilog course
A free and complete Verilog course for students. Learn everything from scratch including syntax, different modeling styles and testbenches.
CMOS - IC Design Course
Digital electronics course.
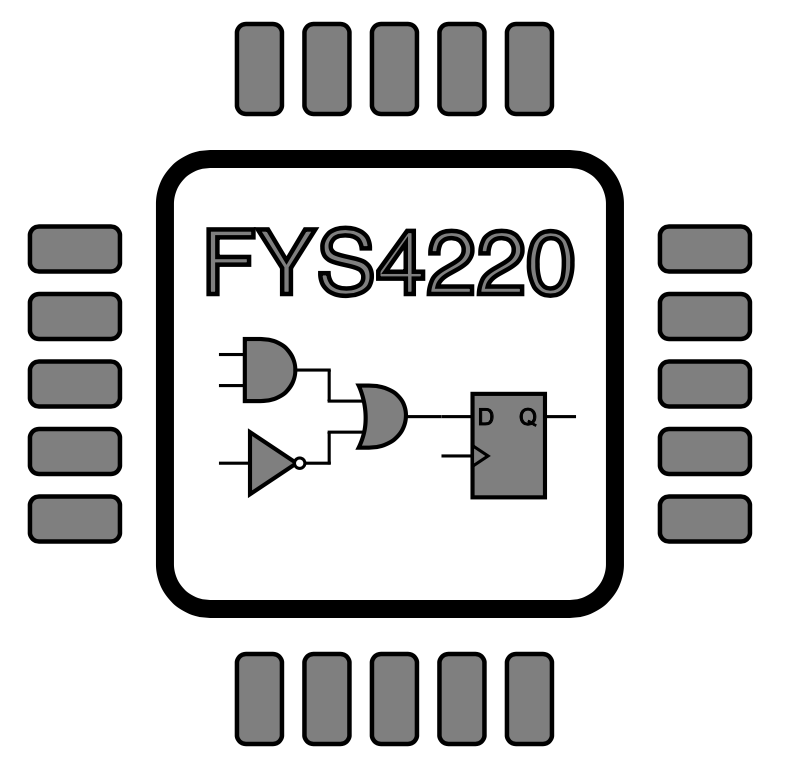
Testbenches
2.9. testbenches #.
For any development it is important to test and verify your solution before it is deployed in the field. This is no different for FPGAs where it is required to simulate your design before downloading and running it on the FPGA. In this section we will introduce the concept of testbenches in VHDL. Testbenches are the standard approach to simulate and verify your HDL design, and are used to apply stimuli to the inputs of a design and observe the outputs to verify correct behaviour. This is illustrated in figure Fig. 2.15 .
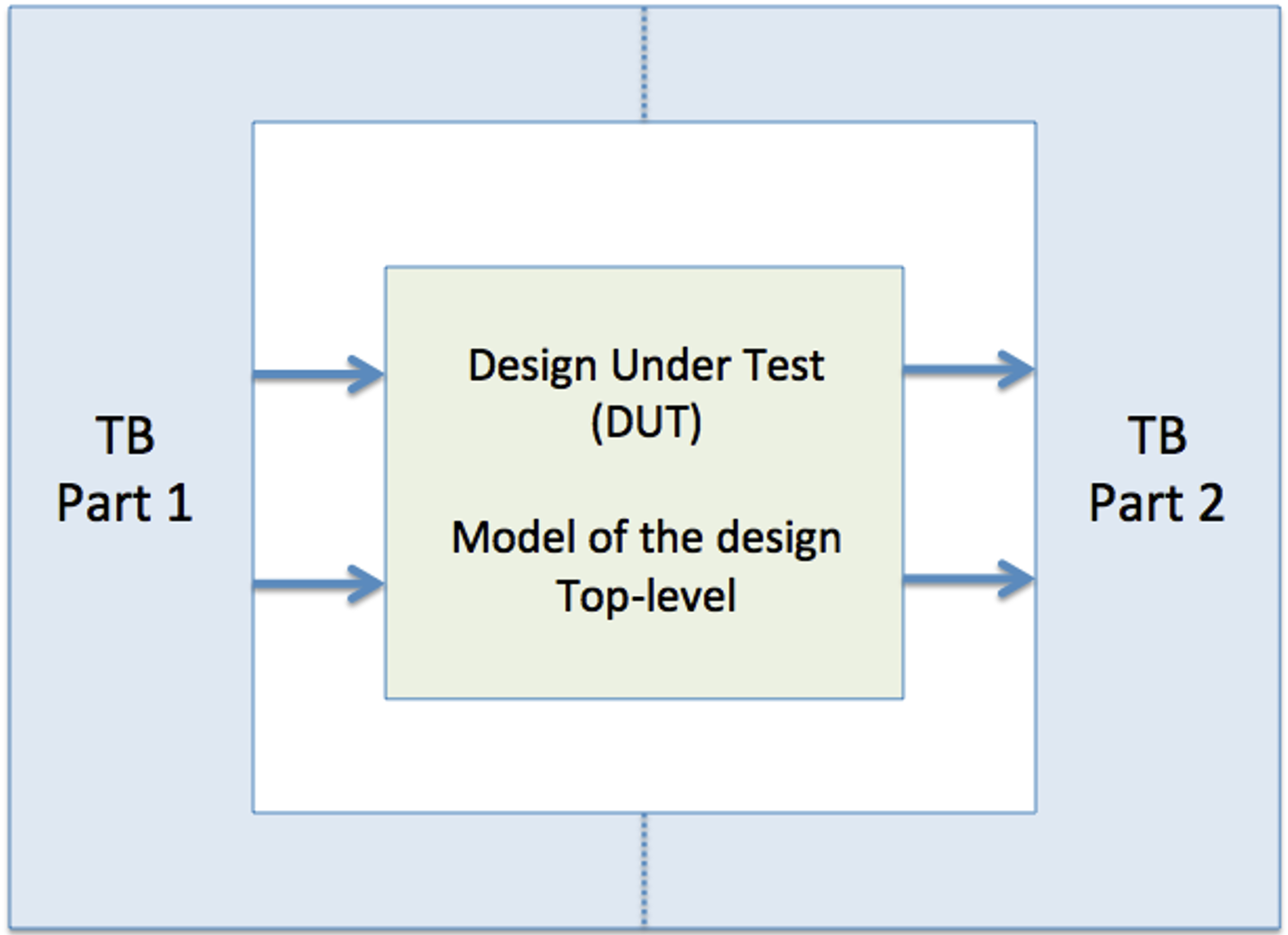
Fig. 2.15 A top-level view on testbenches. #
Testbenches can be written in VHDL. A VHDL testbench is not meant to run on hardware and can therefore take advantage of the full extent of the VHDL language. In contrast, the design under test, which will run on the FPGA, is restricted to VHDL written at the RTL-level level (see section Register Transfer Level (RTL) ).
For a VHDL testbench it is e.g., possible to write a statement like shown below.
This will force a value to the signal A which first is ‘0’ for 20 ns and then ‘1’ for 20 ns as shown in figure Fig. 2.16 .
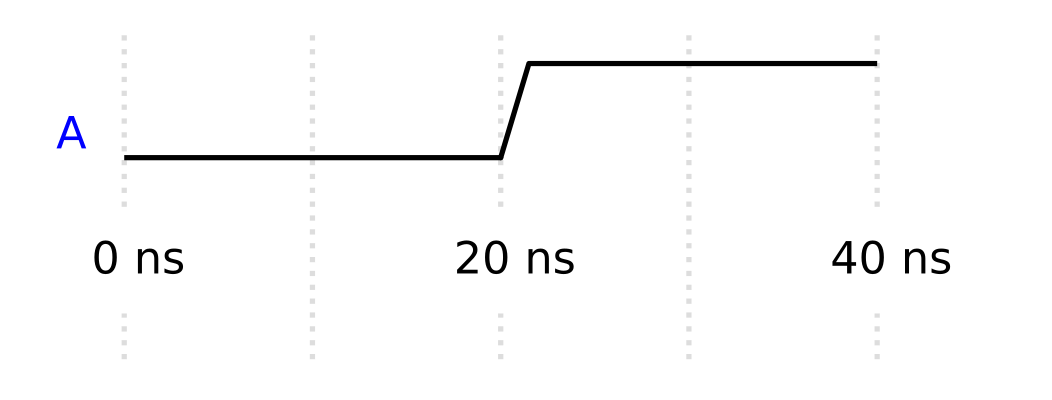
Fig. 2.16 A wave diagram of the code block above. #
This is a simple example of how e.g., an input stimuli can be described using VHDL. However, the synthesis tool is not able to translate the wait for 20 ns statement into any hardware resource on an FPGA. An FPGA does not have any inherently concept or understanding of time specified in this manner. This can only be done by providing the FPGA with a dedicated external clock signal. E.g., a signal that changes between a low and high value at a fixed regular interval. By connecting this clock signal to the internal registers of an FPGA, your design can now be synchronized to this signal, allowing it to update internal states and values on every internal states and values on every clock cycle.
2.9.1. Basic example: half adder #
Consider that you want to verify the correct behaviour of the half adder circuit shown in the code block below.
To build a testbench to verify this design you will make use to the structural description style introduced in section Structural . Since we already have described the half adder as a complete component with an entity and related architecture, we can simply reuse this design in a new VHDL file. The first step is to prepare the testbench VHDL-file. To distinguish the two files and entities, it is common to add tb as a prefix or postfix to the name of the design under test, and use this as the name of the testbench file. In this example add tb as a postfix.
This is the basic skeleton of a general VHDL file. However, different from the VHDL description of the component under test, the testbench has no ports, i.e., the entity declaration is empty. This makes sense if you compare to the diagram in figure Fig. 2.15 . While the component under test may have both inputs and outputs, a testbench is not dependent on outside signal to run. All necessary functionality is generated within the architecture of the testbench. The entity declaration can therefore be left empty.
The testbench can in many ways be compared to a physical test setup on a lab bench, where signal generators are used provide test signals to a component, and an oscilloscope is used to monitor the outputs of the component. The purpose of the VHDL testbench is to act as the signal generator and oscilloscope, and simulate the behaviour of the VHDL design under test.
To include the VHDL design to be tested in the testbench, we can make use of the component and port map statements as shown in section Fig. 2.15 , or we can take advantage of the possibility to do a so called direct instantiation. This uses a slightly different syntax for the port map statement at the benefit of not having to use the component statement.
If not specified, the design will be compiled into a library called work . To reuse this design, we have to specify the name of the entity and the library where it can be found. An entity can be connected to multiple architectures, if this is the case, we also have to specify the name of the architecture, in this case rtl . Otherwise, it will automatically pick the last architecture which was compiled last. It is therefore a good habit to always specify the architecture, even though only one is available.
At the testbench level, we also need to declare signals that will be connected to the design under test, and that will provide stimuli to the inputs, and be monitored for expected values on the outputs.
The next step is to generate the appropriate stimuli to test the design. For this we will make use of the process statement. The process statement is described in more detail in the section VHDL Process . For now you can think of the process as a possibility to describe a sequential behaviour. While all statements inside the architecture of a VHDL file are concurrent statements, including the process statement, statements which are inside a process are read sequentially.
How to end a simulation
The stimuli process in a testbench is meant to run only once. To achieve this the process must use a wait statement at halt the process. Otherwise the process would continue to repeat it self as an infinit loop.
Supplementary suggested reading:
Chapter 5, section 5.7 in LaMeres [ LaM19 ] .
Direct link html-version
Direct link pdf-version Chapter 8, section 8.4 in LaMeres [ LaM19 ] . Direct link html-version Direct link pdf-version
2.9.2. Simulate in Modelsim #
The testbench is now ready to be simulated using Modelsim. A simulation tool like Modelsim can be used to run the test bench and visualize its behaviour in a wave diagram format. Modelsim is a commercial tool, but a free version called Modelsim-Intel FPGA Starter Edition is included when downloading and installing the Quartus Prime Lite Edition. The starter edition is limited to 10000 lines of code. Adding more lines will reduce the performance of the simulator is significantly. An open-source alternative for the VHDL language is GHDL or GHDL on Github . The output of GHDL can be read and viewed by GTKWave . Since Modelsim is already included in Quartus Prime Lite, and since it is one of the most used tool in industry, we will continue to use Modelsim in this course.
In the following description the files are organized as shown below. Design files and testbench files are often separated into different folders. It is also good practice to create a separate folder where the simulations are run.
A new project called half_adder is create in Modelsim (File-> New -> Project) and the folder sim is used as the Project Location as shown in Fig. 2.17
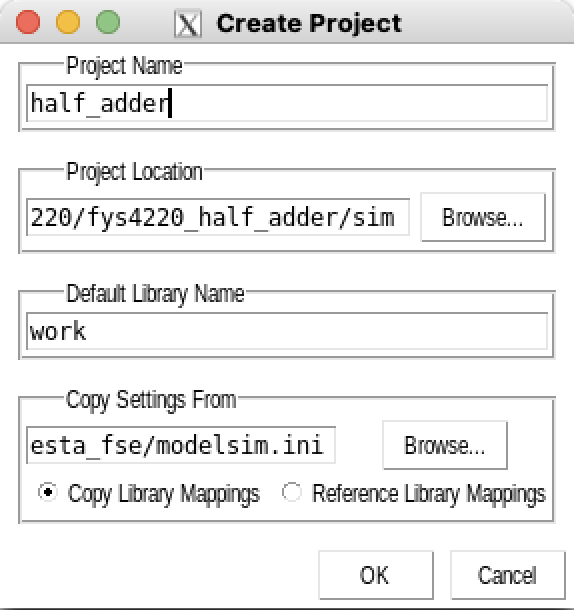
Fig. 2.17 Create Modelsim project. #
The source file and testbench file can be added to the project by choosing “Add Existing File” during the setup of the project, or through the menu bar (Project -> Add to Project -> Existing File).
The files have to be compiled in the correct order. half_adder_tb.vhd is dependent on half_adder.vhd , half_adder.vhd therefore has to be compiled first. To compile right click on the file and choose (Compile -> Compile Selected).
If Modelsim does not report any errors the simulation can now be started choosing (Simulate -> Start Simulation) from the menu and choosing the test bench unit half_adder_tb.vhd under the work library as shown in figure Fig. 2.18 .
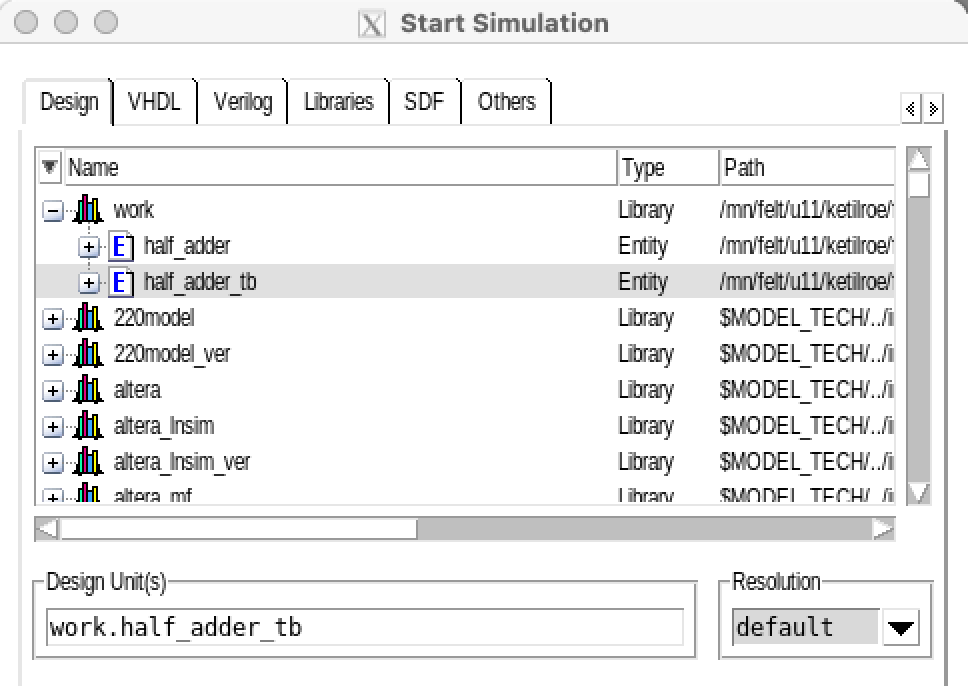
Fig. 2.18 Choose the test bench entity to start the simuliation. #
In Modelsim the wave viewer can be used to visualize the values of the input and outputs signals. If the wave viewer is not opened automatically this can be done by choosing (View -> Wave) from the menu bar. At this point no signals are added to the wave viewer. Mark the relevant signals, right click and choose “Add Wave” as shown in figure Fig. 2.19 .
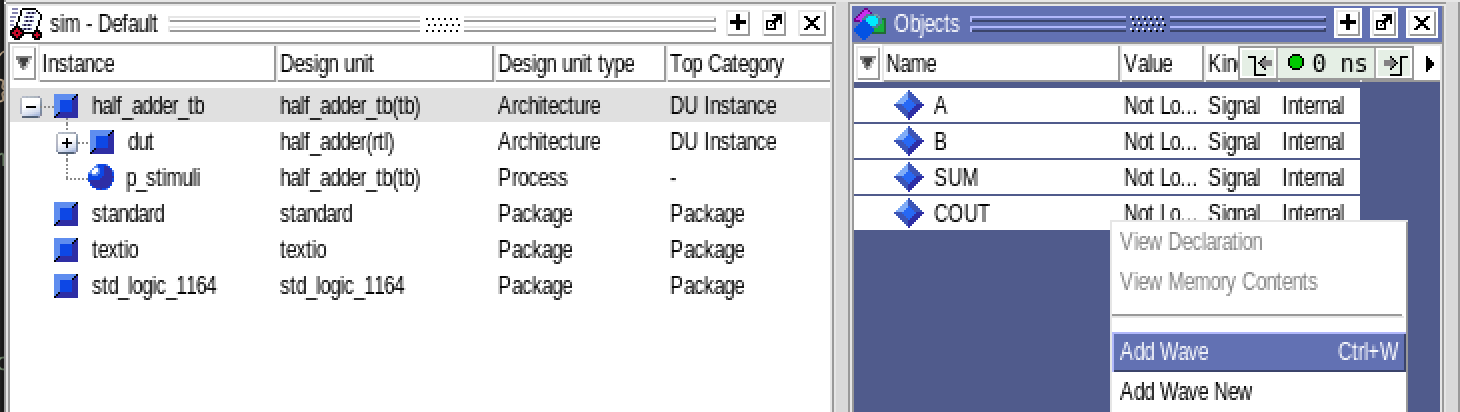
Fig. 2.19 Mark relevant signals to be added to the wave window. #
The simulation can now be run (Simulate -> Run -> Run -All) and you should see a similar results to the wave diagram shown in figure Fig. 2.20 .
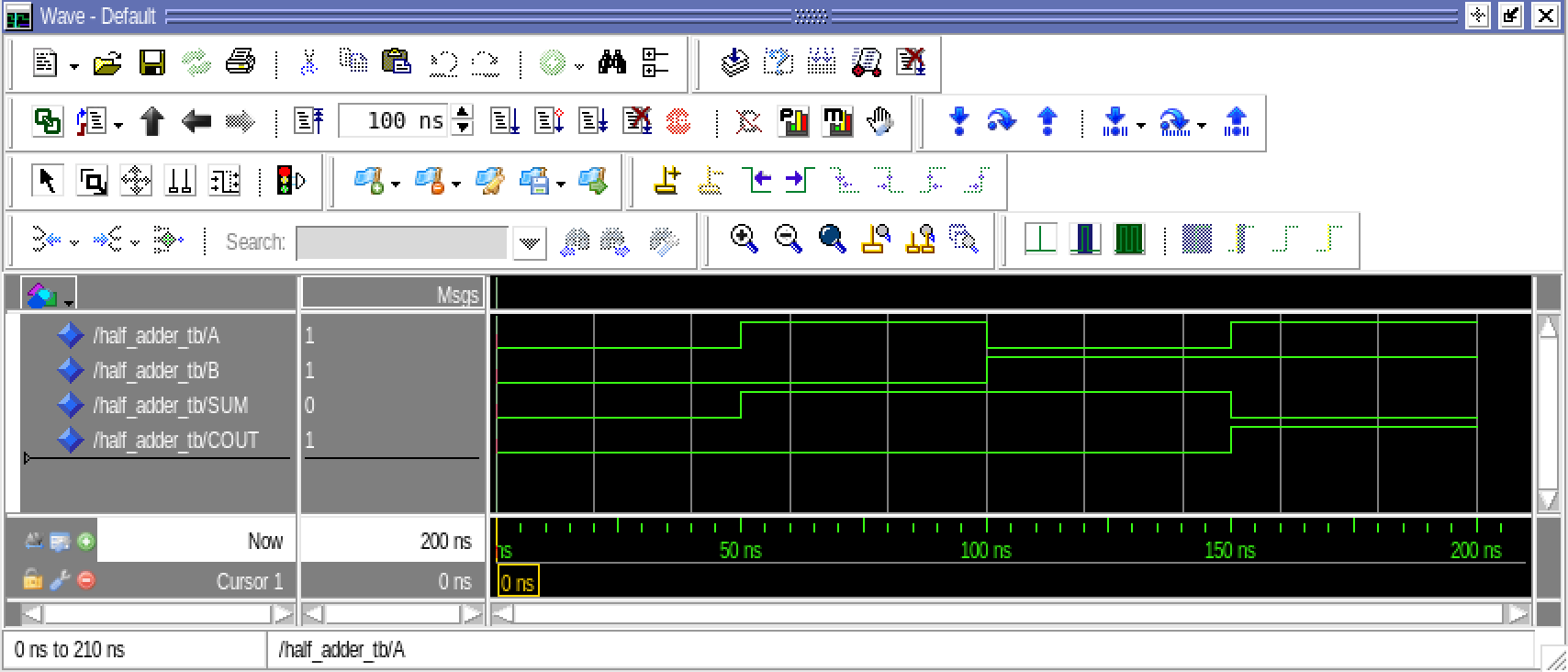
Fig. 2.20 The Modelsim wave viewer showing the result of the simulation of the half adder. #
To verify the correct behaviour of the half adder, we need to manually inspect the wave diagrams in the wave viewer. This is a very basic level of verification where only the stimuli on the inputs are automated.
2.9.3. Self-testing testbenches #
A manual verification of a wave diagram as discussed above will very quickly become impractical and time-consuming as your design’s complexity increases. It is therefore recommended to automate the simulation and verification as much as possible. Ideally, a testbench should run and complete reporting simply if the simulation passed the verification criteria or no. This means that you have to add checking into your testbench. One method is to make use of the assertion and report statements in VHDL.
2.9.3.1. Assert and report #
The basic syntax of the report statement is:
There are four severity levels that can be used (error, warning, note, and failure). Using severity level failure will stop the simulation, while the three others will allow the simulation to continue.
If the severity level is omitted, the report statement is implicitly assumed to be a note .
Given the following lines:
a simulation in Modelsim will return:
Report statements are sequential statements, which means that they can only be used inside a VHDL Process or {ref} vhdl-packages-procedure statement.
The most basic syntax of the assert statement is:
This statement can be used to check if a condition is true or false. If the condition is true, the simulation will continue without prompting a message. If the condition is false, the simulation will prompt a message.
Assertion and report statements can be combined to check if an expected condition is met, or provide feedback in case it is not met.
The combined syntax is shown below followed by a basic example:
When used in the half-adder test bench, the assert statement can be used to verify correct behaviour of the half-adder.
E.g., when the inputs A and B are both ‘0’, we expect the output signal SUM to also be ´0´.
Adding the assertion statement checking for the condition SUM = ‘0’ will report and error if the condition is not met. You can easily test this by either changing your design or by testing for the condition SUM = ‘1’ instead. However, simply testing for the condition will only report the error but not provide any valuable debug information about what has gone wrong expect for the time at which the assertion was activate.
To increase the verbosity level you can add a report statement and also the appropriate severity level. To test this you can change the statement SUM <= A xor B; to SUM <= A or B in your half-adder design and demonstrate the assertion statement as shown below.
Modelsim will then report the following message:
Assert statements can be used both in the concurrent part of the architecture and inside a process.
A note on simulation time and updating of signals
You might have noticed that a “ wait for 1 ns ” statement has been use in the examples above. The purpose of this statement is to allow the simulation time to progress in order for the signal assignments to be updated with their new values. Within a process each signal assignment is scheduled to be updated with its new value only when the process suspends.This can be achieved using a wait statement. When the process hits wait the statement, all the signal assignments up to that point will be updated, triggering the design under test to respond to these events, and eventually leading to that the states and outputs of the design under test also are updated. Adding a “ wait for 1 ns ” statement therefore allows the output SUM and COUT to be updated before the assertion statement is executed.
VHDL 2008 and type convertion
VHDL is a strongly type language. For the report statement this means that the value of SUM which is of the type std_logic needs to be convert to a string. If the testbench is compiled with VHDL 2008, it is possible to use the to_string function. For previous versions, the convertion had to be done using the more cryptic statement std_logic'image(SUM) . Also, this statement did not support convertion from std_logic_vector . For ease of use, it is therefore recommended to use the to_string function supported in VHDL. This also supports std_logic_vector .
In Modelsim you can set the appropriate VHDL version in two ways:
Right click on the file to be compiled (Properties –> VHDL), or
through compile options in the menu bar for project wide setting (Compile –> Compile options)
The assertion statement also supports composite conditions.
A complete stimuli process including assertion statements for the half adder example is shown below.
If the half adder design is correct, Modelsim will report the following when running the process above.
Modifying the half adder design to include and intentional error, e.g., changing the statement SUM <= A xor B; to SUM <= A or B , Modelsim will report the following.
In this case it catches and reports the design error.
2.9.4. Defining a clock source #
For synchronous designs you need to define a clock source for your testbench. A clock is a signal that changes between ‘0’ and ‘1’ at a fixed interval. This can be described using a simple process as shown below.
If the signal is declared with an initial value it can also be described as shown below.
However, for both of these descriptions the clock will start to run immediately and will never end. A recommended solution is therefore to use and enable signal to turn on and of the clock.
2.9.5. Supporting videos #
The following video discusses testbenches in VHDL. The first part of the video until about 14:11, presents some of the basic concepts similar to what has also been introduced in the text on this page. After 14:11 the video continues to cover a few more topics related to more advanced self-testing testbenches. These topics will make more sense as the course progresses and you learn more about VHDL packages and sub-programs. The examples in the video are different from the example shown above – the video was recorded before this page was written. In the future the plan is to update the video to make it more aligned with the content on this page.
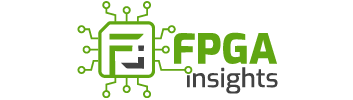
VHDL Testbench: How to Create and Use for Efficient Design Verification
Niranjana R
Updated on: January 24, 2024
VHDL Testbench is a crucial aspect of digital circuit design. It is a simulation environment that allows designers to test their VHDL code before synthesizing it into a hardware device. VHDL Testbenches are used to verify the functional correctness of the design, identify any potential issues, and optimize the design for better performance.
Creating a VHDL Testbench involves creating a stimulus that drives the inputs of the design and monitors the outputs to ensure that they match the expected results. VHDL Testbenches can be created for both combinational and sequential circuits. The test bench should be designed to cover all possible input combinations and edge cases to ensure that the design is robust and reliable.
In this article, we will explore the importance of VHDL Testbenches in digital circuit design and provide a step-by-step guide on how to create a basic VHDL Testbench. We will also discuss the different types of testbenches, stimulus generation methods, and best practices for creating effective VHDL Testbenches.
Table of Contents
Understanding VHDL Testbench
As FPGA designers, we need to ensure that our circuits work as expected before deploying them on hardware. One way to accomplish this is by using VHDL testbenches. A testbench is a VHDL file that provides stimuli to the design under test (DUT) and checks its output against expected results.
The testbench provides the necessary inputs to the DUT, which then produces outputs. The testbench then compares the outputs against the expected results to determine if the DUT is functioning correctly.
The primary goal of the testbench is to automate the testing process and provide a more efficient way to verify the functionality of the DUT. It also allows us to test the DUT under different conditions and scenarios, which can be difficult or impossible to do on hardware.
To create a testbench, we need to define the inputs and outputs of the DUT and initialize them with appropriate values. We also need to provide a clock signal to the DUT and ensure that the inputs are synchronized with the clock.
We can use various VHDL constructs such as if-else statements, loops, and wait statements to define the testbench’s behavior. We can also use assertions to check the correctness of the DUT’s output.
Overall, the test bench is an essential part of the FPGA design process. It allows us to verify the functionality of the DUT and catch any errors before deploying the design on hardware.
VHDL Testbench Components
In VHDL, a testbench is used to verify the functionality of a design through simulation. A testbench is a separate VHDL code that is used to provide stimulus to the design under test (DUT) and check the output response. Several components make up a VHDL testbench.
The entity is the top-level component of the testbench. It is used to define the inputs and outputs of the design under test. The entity of the testbench is different from the entity of the DUT. The inputs of the testbench entity are the stimuli that are applied to the DUT, and the outputs are the responses from the DUT.
The behavior of the testbench is defined in the architecture section of the testbench entity. The behavior can be described using a process or a concurrent statement. A process is used to generate the test vectors, apply them to the DUT, and check the output response. A concurrent statement is used to generate a clock signal or other signals that are required for the test bench.
Test Vector
The test vector is a set of input values that are applied to the DUT. The test vector can be generated using a process or a function. The test vector should cover all possible input combinations to ensure that the DUT is fully tested.
Clock Signal
The clock signal is an important component of the test bench. It is used to synchronize the inputs and outputs of the DUT with the testbench. The clock signal can be generated using a process or a concurrent statement. The clock signal should be generated with a frequency that is appropriate for the DUT.
In summary, a VHDL testbench consists of several components, including the entity, behavior, test vector, and clock signal. The entity defines the inputs and outputs to the DUT, while the behavior describes the testbench’s functionality. The test vector provides the input stimuli to the DUT, and the clock signal synchronizes the inputs and outputs of the DUT with the testbench.
Creating a VHDL Testbench
When designing digital circuits, it is essential to verify their functionality before implementing them in hardware. One way to achieve this is by creating a VHDL testbench. A testbench is an HDL code that simulates the behavior of a design under various input conditions. In this section, we will discuss the code structure, simulation steps, and debugging tips for creating a VHDL testbench.
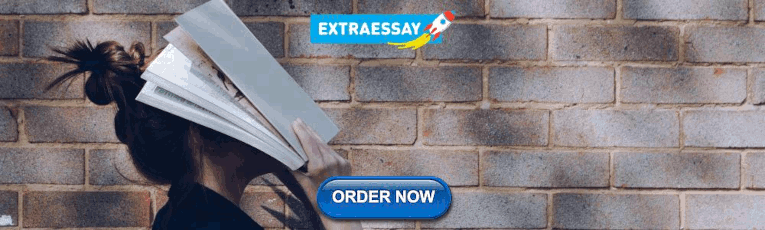
Code Structure
The code structure of a VHDL testbench consists of two main parts: the entity and the architecture. The entity defines the input and output ports of the design under test (DUT), while the architecture contains the testbench code that simulates the DUT. We use the entity to define the inputs and outputs of our design. The testbench code is written using the same VHDL syntax as the design code.
Simulation Steps
The following are the general steps to simulate a VHDL testbench:
- Compile the design and the testbench files using a VHDL simulator.
- Load the compiled design and testbench into the simulator.
- Set the initial values for the input signals of the DUT.
- Simulate a specified amount of time or until a specific condition is met.
- Analyze the simulation results to ensure that the DUT behaves as expected.
Debugging Tips
Debugging a VHDL testbench can be challenging, but the following tips can help:
- Use waveform viewers to visualize the behavior of the signals in the design and testbench.
- Add print statements to the testbench code to output the values of the signals at specific points in time.
- Check the syntax and semantics of the VHDL code for errors.
- Verify that the input signals to the DUT are correct.
- Use assertions to check that the DUT behaves as expected.
In conclusion, creating a VHDL test bench is an essential step in the design process of digital circuits. By following the code structure, simulation steps, and debugging tips outlined in this section, we can ensure that our designs are functional and reliable.
Advanced VHDL Testbench Techniques
When it comes to testing HDL designs, the testbench plays a crucial role in ensuring that the design functions correctly. In this section, we will discuss some advanced techniques that can be used to improve the effectiveness of VHDL test benches.
One technique for improving the efficiency of VHDL test benches is to use data files to store input stimuli. By using data files, we can easily modify the input stimulus without having to modify the testbench code. This can save a lot of time and effort, especially when testing large designs.
Random Stimuli
Another technique for improving VHDL testbenches is to use random stimuli. By generating random input stimuli, we can test the design more comprehensively. This can help to uncover bugs that may not be found using traditional test vectors. However, it is important to ensure that the random stimuli generated are appropriate for the design being tested.
Assertions are another powerful technique for improving VHDL testbenches. Assertions allow us to check that certain conditions are met during simulation. This can help to catch bugs that may not be caught by traditional test vectors. Assertions can also help to reduce the amount of time required for debugging.
In conclusion, by using advanced VHDL testbench techniques such as data files, random stimuli, and assertions, we can improve the efficiency and effectiveness of our test benches. These techniques can help uncover bugs that may not be found using traditional test vectors and can help reduce the amount of time required for debugging.
In conclusion, VHDL test benches are essential tools for verifying the functionality of digital circuits. By creating a testbench, we can simulate the behavior of a circuit and verify its correctness before it is implemented in hardware. This helps to save time and reduce errors in the design process.
Throughout this article, we have covered the basics of VHDL testbenches, including their architecture, key concepts, and a complete example. We have also explored some tips for improving the effectiveness of test benches, such as organizing them effectively and using assertions to validate the expected behavior of the circuit.
Overall, VHDL test benches are a powerful tool for digital circuit design and verification. By using them effectively, we can ensure that our circuits are correct and reliable, and reduce the risk of costly errors in the design process.
Related Articles
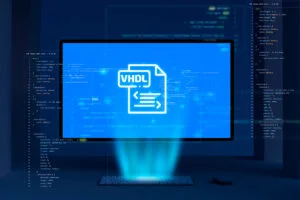
Leave a Comment
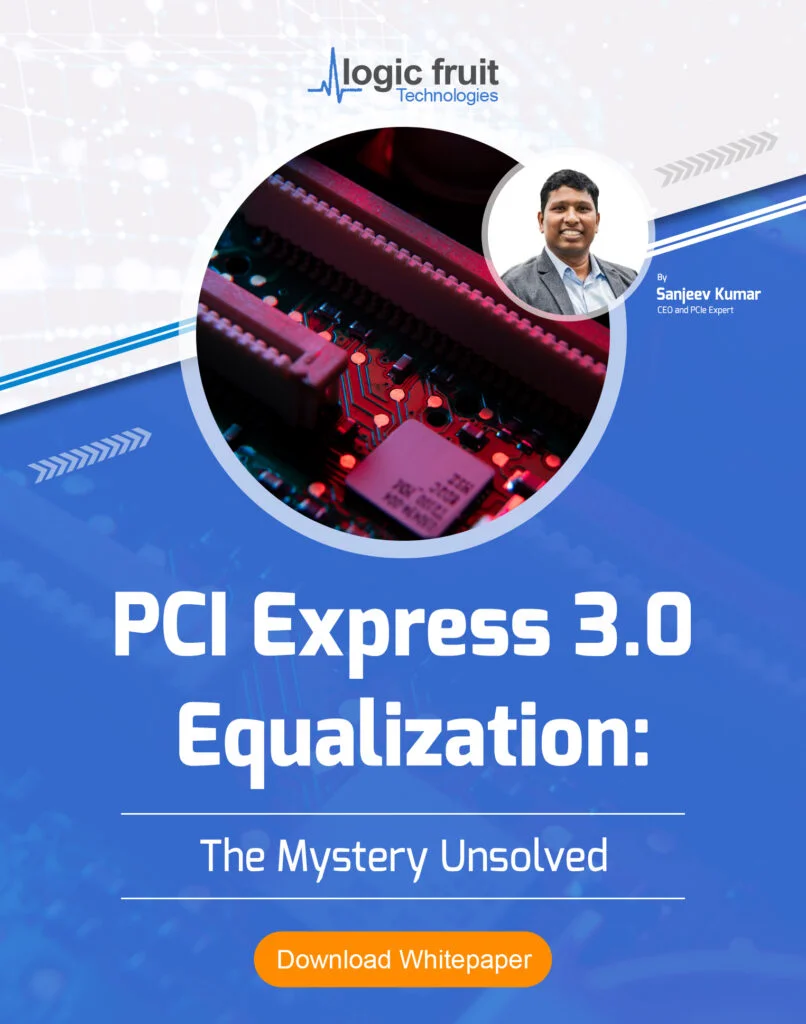
most recent
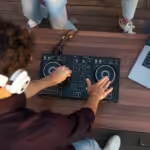
Artificial Intelligence
Ai in music: creating innovative compositions and personalized playlists.
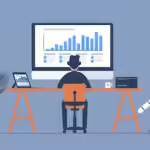
AI in Financial Services – Revolutionizing Banking and Investment Strategies
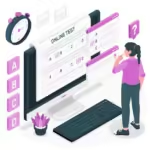
Test & Measurement
Integrating test & measurement in agile development: best practices.
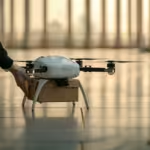
Automated Testing for Drone Technology: Ensuring Safe Flights
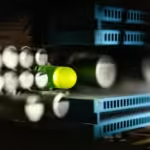
Power Management
Power management in high-performance computing (hpc) systems.
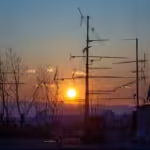
Power Management Strategies for Remote and Off-Grid Locations
Subscribe to get our best viral stories straight into your inbox, related posts.
- Testbench VHDL Example: A Clear and Concise Guide December 3, 2023
- Process: Basic Functional Unit in VHDL June 21, 2023
- VHDL Generic Tutorial: Master Parameterization December 15, 2023
- From Concept to Implementation: Leveraging Procedures in VHDL June 21, 2023
- Exploring Data Types in VHDL: A Comprehensive Guide June 21, 2023
- Mastering VHDL Functions: A Comprehensive Guide for Efficient Programming December 20, 2023
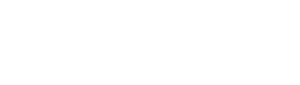
FPGA Insights have a clear mission of supporting students and professionals by creating a valuable repository of FPGA-related knowledge to contribute to the growth and development of the FPGA community and empower individuals to succeed in their projects involving FPGAs. FPGA Insights has been producing FPGA/Verilog/VHDL Guides and blogs with the aim of assisting students and professionals across the globe. The mission of FPGA Insights is to continually create a growing number of FPGA blogs and tutorials to aid professionals in their projects.
© 2024 Fpga Insights. All rights reserved
Beyond Circuit Podcast by Logic Fruit: High-speed video interfaces in Indian Aerospace & Defence.

Variables vs. Signals in VHDL
Variables and Signals in VHDL appears to be very similar. They can both be used to hold any type of data assigned to them. The most obvious difference is that variables use the := assignment symbol whereas signals use the <= assignment symbol. However the differences are more significant than this and must be clearly understood to know when to use which one. If you need a refresher, try this page about VHDL variables .
Signals vs. Variables:
- Variables can only be used inside processes, signals can be used inside or outside processes.
- Any variable that is created in one process cannot be used in another process, signals can be used in multiple processes though they can only be assigned in a single process .
- Variables need to be defined after the keyword process but before the keyword begin . Signals are defined in the architecture before the begin statement.
- Variables are assigned using the := assignment symbol. Signals are assigned using the <= assignment symbol.
- Variables that are assigned immediately take the value of the assignment. Signals depend on if it’s combinational or sequential code to know when the signal takes the value of the assignment.
The most important thing to understand (and the largest source of confusion) is that variables immediately take the value of their assignment, whereas signals depend on if the signal is used in combinational or sequential code . In combinational code, signals immediately take the value of their assignment. In sequential code, signals are used to create flip-flops, which inherently do not immediately take the value of their assignment. They take one clock cycle. In general, I would recommend that beginners avoid using variables. They can cause a lot of confusion and often are hard to synthesize by the tools.
The example below demonstrates how signals behave differently than variables. Notice that r_Count and v_Count appear to be the same, but they actually behave very differently.
Variables can be a bit tricky to display in simulation. If you are using Modelsim, read more about how to see your variables in Modelsim’s waveform window . Look carefully at the waveform above. Do you see how o_var_done pulses every 5th clock cycle, but o_sig_done pulses every 6th clock cycle? Using signals and variables to store data generates very different behavior . Make sure you clearly understand what you code will be generating and make sure that you simulate your code to check that behaves like you want!
Learn Verilog
Leave A Comment Cancel reply
Save my name, email, and website in this browser for the next time I comment.
- Product Manual
- Knowledge Base
- Release Notes
- Tech Articles
- Screencasts
Signal Assignments in VHDL: with/select, when/else and case
Sometimes, there is more than one way to do something in VHDL. OK, most of the time , you can do things in many ways in VHDL. Let’s look at the situation where you want to assign different values to a signal, based on the value of another signal.
With / Select
The most specific way to do this is with as selected signal assignment. Based on several possible values of a , you assign a value to b . No redundancy in the code here. The official name for this VHDL with/select assignment is the selected signal assignment .
When / Else Assignment
The construct of a conditional signal assignment is a little more general. For each option, you have to give a condition. This means that you could write any boolean expression as a condition, which give you more freedom than equality checking. While this construct would give you more freedom, there is a bit more redundancy too. We had to write the equality check ( a = ) on every line. If you use a signal with a long name, this will make your code bulkier. Also, the separator that’s used in the selected signal assignment was a comma. In the conditional signal assignment, you need the else keyword. More code for the same functionality. Official name for this VHDL when/else assignment is the conditional signal assignment
Combinational Process with Case Statement
The most generally usable construct is a process. Inside this process, you can write a case statement, or a cascade of if statements. There is even more redundancy here. You the skeleton code for a process (begin, end) and the sensitivity list. That’s not a big effort, but while I was drafting this, I had put b in the sensitivity list instead of a . Easy to make a small misstake. You also need to specify what happens in the other cases. Of course, you could do the same thing with a bunch of IF-statements, either consecutive or nested, but a case statement looks so much nicer.
While this last code snippet is the largest and perhaps most error-prone, it is probably also the most common. It uses two familiar and often-used constructs: the process and the case statements.
Hard to remember
The problem with the selected and conditional signal assignments is that there is no logic in their syntax. The meaning is almost identical, but the syntax is just different enough to throw you off. I know many engineers who permanenty have a copy of the Doulos Golden Reference Guide to VHDL lying on their desks. Which is good for Doulos, because their name gets mentioned all the time. But most people just memorize one way of getting the job done and stick with it.
- VHDL Pragmas (blog post)
- Records in VHDL: Initialization and Constraining unconstrained fields (blog post)
- Finite State Machine (FSM) encoding in VHDL: binary, one-hot, and others (blog post)
- "Use" and "Library" in VHDL (blog post)
- The scope of VHDL use clauses and VHDL library clauses (blog post)
Stack Exchange Network
Stack Exchange network consists of 183 Q&A communities including Stack Overflow , the largest, most trusted online community for developers to learn, share their knowledge, and build their careers.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
generating clock signal for testbench in VHDL
I'm wondering why the first assignment for a clock signal in VHDL does not work like the second. I'd appreciate any explanations about the simulation behavior of assign statement with delay. Thanks.
First version: (just produces spikes 50 ns apart)
Second version: (produces a square wave of time period 100 ns as expected)

By omitting the "after" clause from the first assignment in your sequence of assignments (known as a waveform), you are implicitly implying "after 1 delta cycle". Delta cycles are the key to how the VHDL simulation is performed. A delta cycle can be thought of as an infinitesimally small delay, but in reality that is a gross simplification. So in your case this infinitesimally small delay is why you don't see the positive duration of the clock cycle last for very long, although this behaviour will vary slightly with different simulators.
I would suggest that you do some further reading on delta cycles and follow it up with reading your simulator's manual to understand their implementation.
A concurrent statement is evaluated every time a signal on the right hand side changed. It may help to think about concurrent statements such as these in their equivalent process form. A concurrent statement is equivalent to a process that is sensitive to the signals it references on the right hand side of the statement. If there are no referenced signals then it is equivalent to a process with an empty sensitivity list and a final wait statement.
The "clk <= '0', not clk after 50ns" example is evaluated every time clk changed, clk will be scheduled with '0' after one delta cycle and "not clk" (ie '1') after 50ns. At 50ns clk has changed and so the statement is evaluated again, at which point clk is scheduled with '0' after one delta cycle and "not clk" 50ns later. Therefore the duration between when clk is '1' and '0' is one delta cycle.
The "clk <= '0', '1' after 50ns" example is only evaluated once because there are no signals on the right hand side for it be sensitive to. Therefore clk is scheduled with '0' after one delta cycle and '1' after 50ns at which point no further changes are scheduled.
- \$\begingroup\$ I understand delta cycles, and know the basic event driven simulation algorithm. This doesn't answer my question. Are you saying the first statement's driver is invoked as soon as a '1' is assigned and assigns a '0' after delta delay? This doesnt make sense, as a statement such as clk <= '0', '1' after 50 ns; works fine. (The '0' is not assigned again) \$\endgroup\$ – Neha Karanjkar Commented May 6, 2013 at 6:00
- \$\begingroup\$ That is exactly what I am saying. I have updated my answer for further clarification. \$\endgroup\$ – Amoch Commented May 6, 2013 at 22:57
- \$\begingroup\$ your edit clarifies it. Thanks for the explanation, this is exactly what I was looking for. \$\endgroup\$ – Neha Karanjkar Commented May 8, 2013 at 15:52
Your Answer
Sign up or log in, post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged vhdl or ask your own question .
- Featured on Meta
- Introducing an accessibility dashboard and some upcoming changes to display...
- We've made changes to our Terms of Service & Privacy Policy - July 2024
- Announcing a change to the data-dump process
Hot Network Questions
- I don't do something AFTER I did something
- If pressure is caused by the weight of water above you, why is pressure said to act in all direction, not just down?
- How to use Mathematica to plot following helix solid geometry?
- Do the merits of an exegesis relying on texts unavailable to the author depend upon whether the unavailable texts were invented or not-yet-existent?
- Can an elf and a firbolg breed?
- Delexing a finitely complete category
- Can I use specific preprocess hooks for a node type or a view mode?
- How to open a single app in a particular language while the system language is English?
- Strategies for handling Maternity leave the last two weeks of the semester
- Refereeing papers by people you are very close to
- How can flipflops sense the edges of the signals?
- Emphasizing the decreasing condition in the Integral Test or in the AST (in Calculus II): is it worth the time?
- Is there a canonical example of the computer misinterpreting a command in any Star Trek franchise?
- Self-employed health insurance deduction and insurance just for my kids
- dealing with the feeling after rejection
- Why is the completely dark disk of the Moon visible on a new moon if the lunar orbit is at an angle to the Earth’s?
- What does "No camping 10-21" mean?
- Does wisdom come with age?
- How do I resolve license terms conflict when forking?
- Combining Regex and Non-Regex in the same function
- Who‘s to say that beliefs held because of rational reasons are indeed more justified than beliefs held because of emotional ones
- Automatically closing a water valve after a few minutes
- Double accentuation (Homeric Greek)
- When can a citizen's arrest of an Interpol fugitive be legal in Washington D.C.?
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Verilog multiple simultaneous independant signal assignments in testbench
In VHDL I can write this in my testbench:
The signal declarations are before the "begin" of the testbench architecture while the portion after the elipse is in the body of the testbench architecture. A much better way is to use a process which ends with "wait" statement when writing testbenches. I understand how to do this in verilog as well as VHDL.
In verilog we can have an initial block that assigns value once. It is also possible to have multiple initial blocks. I have not tried this, but I don't think that it is wise to drive the same signal from multiple initial blocks.
Now my question is, how do I translate the above code for the DUT stimulus into Verilog? I expect that I shall use an assign statement with multiple #delay values. Is that correct? How do I do it?
2 Answers 2
In Verilog 2001 and above, you can initialize variables upon declaration, like VHDL. Another interesting, but perhaps less common way to do this is to use use a fork-join block with blocking assignments. In the following code, each line in the fork-join block is executed independently and concurrently.
Working example on edaplayground .
Also, notice that the clk signal in your code only toggles once. I slightly modified it so that the clock is running endlessly.
- hmm.. I don't see anything similar to fork-join in VHDL. I thought this was something specific to systemverilog. – quantum231 Commented Jun 2, 2014 at 17:50
- As far as I know, there is no fork-join in VHDL, but it existed in Verilog since Verilog-95. SystemVerilog extended it by adding join_any and join_none . Notice that fork-join is a very powerful construct, but it is not synthesizable and may complicate debugging. – Ari Commented Jun 2, 2014 at 18:26
I'm not too familiar with VHDL, but this looks like a test bench stimulus. I generated the runnable testcase for comparison here .
The Verilog equivalent would look something like:
This will generate the same waveform, except count_out is floating instead of all zeros. By the naming convention I consider count_out should be driven by the device-under-test, which needs to be a wire type.
A SystemVerilog may look something like:
Working example of Verilog and System Verilog here

Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged vhdl verilog or ask your own question .
- Featured on Meta
- We've made changes to our Terms of Service & Privacy Policy - July 2024
- Introducing an accessibility dashboard and some upcoming changes to display...
- Tag hover experiment wrap-up and next steps
Hot Network Questions
- Best (safest) order of travel for Russia and the USA (short research trip)
- Design patterns - benefits of using with Apex code
- Arduino Board Getting Too Hot: Need Help Diagnosing Issue (Schematic Provided)
- Is Marisa Tomei in the film the Toxic Avenger?
- Wall of Fire: Taking damage multiple times by using forced movement on a target
- Which Boolean Math mode should I use?
- Flashlight and 6 batteries
- Can a Hall sensor be tested with just basic test gear?
- Emphasizing the decreasing condition in the Integral Test or in the AST (in Calculus II): is it worth the time?
- Solving the "Reverse" Assignment Problem with an Added Constraint?
- Can I continue using technology after it is patented
- Will lights plugged into cigarette lighter drain the battery to the point that the truck won't start?u
- Who‘s to say that beliefs held because of rational reasons are indeed more justified than beliefs held because of emotional ones
- Strategies for handling Maternity leave the last two weeks of the semester
- What type of concept is "mad scientist"?
- Negative Binomial Regression
- Why is the completely dark disk of the Moon visible on a new moon if the lunar orbit is at an angle to the Earth’s?
- Is there such a thing as icing in the propeller?
- Why do I see different declension tables for the same noun in different sources?
- If pressure is caused by the weight of water above you, why is pressure said to act in all direction, not just down?
- How do I rigorously compute probabilities over infinite sequences of coin flips?
- Does wisdom come with age?
- Remove the Freehub Body from a Omnium Cargo Bike
- In Norway, when number ranges are listed 3 times on a sign, what do they mean?
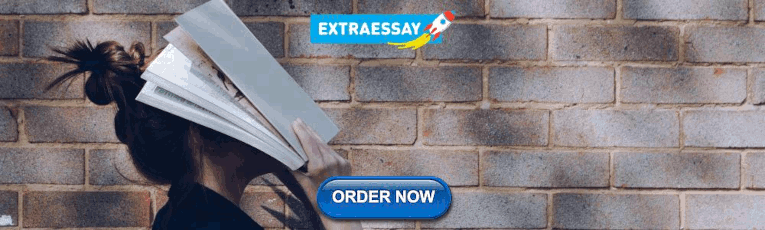
IMAGES
COMMENTS
3. Generate Clock and Reset. The next thing we do when writing a VHDL testbench is generate a clock and a reset signal. We use the after statement to generate the signal concurrently in both instances. We generate the clock by scheduling an inversion every 1 ns, giving a clock frequency of 1GHz.
How can I bring out the internal signals of my VHDL source code to my testbench so that I can view them as waveforms? I use Active HDL. ... When using alias you have to write the assignment in a new statement. ... If you have declared the signals in the testbench but are unable to see any output, you may have a problem in the instantiation ...
The VHDL code shown below uses one of the logical operators to implement this basic circuit. and_out <= a and b; Although this code is simple, there are a couple of important concepts to consider. The first of these is the VHDL assignment operator (<=) which must be used for all signals.
The testbench is also an HDL code. We write testbenches to inject input sequences to input ports and read values from output ports of the module. The module (or electronic circuit) we are testing is called a DUT or a Device Under Test. Testing of a DUT is very similar to the physical lab testing of digital chips.
I want to assign signals of a testbench to a component to which the port have infered constraints. I would like to introduce the problem with a working workbench before moving to a minimally reproducible example. Example with constraint inference. Assume A and B. A.vhdl (the testbench)
If your simulator supports it, you can do what you want as described here. ISim probably does not have any VHDL-2008 support. Without VHDL-2008 your only options are simulator vendor specific functionality, using global signals as in your answer, or with debug ports in your entity. answered Jan 2, 2017 at 15:10.
VHDL test bench (TB) is a piece of code meant to verify the functional correctness of HDL model. The main objectives of TB is to: Instantiate the design under test (DUT) Generate stimulus waveforms for DUT. Generate reference outputs and compare them with the outputs of DUT. Automatically provide a pass or fail indication.
The purpose of the VHDL testbench is to act as the signal generator and oscilloscope, and simulate the behaviour of the VHDL design under test. ... Within a process each signal assignment is scheduled to be updated with its new value only when the process suspends.This can be achieved using a wait statement. When the process hits wait the ...
and write testbench results to another text file, using the VHDL textio package. Operation Address Data. Black: Command from input file. Green: Data read on DOUT. Data read on DOUT. Operations are write (w), read (r), and end (e).
VHDL. VHDL Testbench is a crucial aspect of digital circuit design. It is a simulation environment that allows designers to test their VHDL code before synthesizing it into a hardware device. VHDL Testbenches are used to verify the functional correctness of the design, identify any potential issues, and optimize the design for better performance.
A. Assert. A common way to write a self-checking testbench is with assert statements. Just like in other programming languages, assert statements in simulated VHDL will check a condition and, upon failure of that condition, report a state. Asserts are generally followed by a report statement, which prints a string report.
Variables and Signals in VHDL appears to be very similar. They can both be used to hold any type of data assigned to them. The most obvious difference is that variables use the := assignment symbol whereas signals use the <= assignment symbol. However the differences are more significant than this and must be clearly understood to know when to ...
Assignment. From Last Time. Structural VHDL lab. Outline. VHDL vectors. Test bench styles. Class practice. Coming Up. VHDL Vectors. VHDL vectors are used to group signals together (think buses): ... Test Bench Styles ``Signal generator'' style: LIBRARY ieee; USE ieee.std_logic_1164.ALL; ENTITY tb01 IS END tb01; ARCHITECTURE behavior OF tb01 IS ...
1. Careful with your terminology. When you say a changed in the other "process", that has a specific meaning in VHDL (process is a keyword in VHDL), and your code does not have any processes. Synthesizers will treat your code as: a <= c and d; b <= (c and d) and c; Simulators will typically assign a in a first pass, then assign b on a second ...
The box symbols <<>> denote an external name, and you need to use the full reference to the signal with the correct type. Starting with a . will start from the top of the heirarchy, otherwise its relative to where you currently are, with ^ used to go up a level. Its usual to use an alias for these, so you dont need to keep writing the whole ...
With / Select. The most specific way to do this is with as selected signal assignment. Based on several possible values of a, you assign a value to b. No redundancy in the code here. The official name for this VHDL with/select assignment is the selected signal assignment. with a select b <= "1000" when "00", "0100" when "01", "0010" when "10 ...
The Inside_process and Outside_process versions behave differently. If both designs work, it is mostly out of luck, because in this case Out_signal simply lags half a clock cycle when declared inside the process. Out_signal is assigned when the process triggers, which in this case occurs on rising and falling edges of clk.
I am trying to depict port 3 of microcontroller which acts a I/O and as special functions like timers interrupts in VHDL. The code is as follows: library IEEE; use IEEE.std_logic_1164.all; entity testIO is. port (. p3: inout std_logic; op:out std_logic;
2. I'm wondering why the first assignment for a clock signal in VHDL does not work like the second. I'd appreciate any explanations about the simulation behavior of assign statement with delay. Thanks. First version: (just produces spikes 50 ns apart) clk <= '0', (not clk) after 50 ns; Second version: (produces a square wave of time period 100 ...
alias int1 << signal .tb_top.u_ioc.int1 : std_logic >>; With an earlier VHDL standard you have to use vendor-specific tools, e.g. signal_spy for Questa/ModelSim. Another method would be to write your testbench at a lower level, e.g. connect your sub-components inside the testbench instead of testing the highest-level entity.
The signal declarations are before the "begin" of the testbench architecture while the portion after the elipse is in the body of the testbench architecture. A much better way is to use a process which ends with "wait" statement when writing testbenches. I understand how to do this in verilog as well as VHDL.